Boards with an I2S Audio Chip
You can use many boards or modules which are using I2S based audio codecs that need to be configured. In this case you usually have both a DAC and an ADC available which needs to be configured via I2C before it can be used.
The I2SCodecStream and AudioBoardStream classes can used both as audio source and audio sink. The latter provides some additional button handling functionality.
This project provides many examples for the AudioKit. But you can also use these examples with other boards. You would just need to change the device in the constructor.
Further informatation can be found in this wiki.
Dependencies: Details how to install the necessary HAL library can be found in the arduino-audio-driver project
ESP32-A1S Based Audio Boards (AudioKit, Lyrat)
There are several ESP32-A1S based Audio Boards: The AI-Thinker AudioKit is a popular example, the Espressif LyraT is another. The big advantage is that you don't need to bother about the wiring and you can just use the built in SD drive, microphone and amplifier.
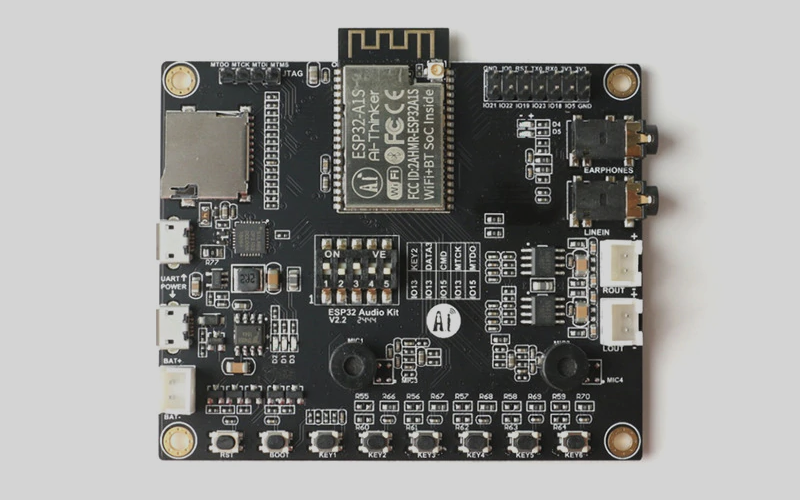
Example use:
#include "AudioTools.h"
#include "AudioLibs/I2SCodecStream.h"
I2SCodecStream i2s(LyratV43); // or LyratV42,AudioKitAC101,AudioKitEs8388V1,AudioKitEs8388V2
void setup() {
// setup i2s and codec
auto cfg = i2s.defaultConfig();
cfg.sample_rate = 44100;
cfg.bits_per_sample = 16;
cfg.channels = 1;
i2s.begin();
// set volume
i2s.setVolume(0.5);
}
ES8388 Audio Codec Module
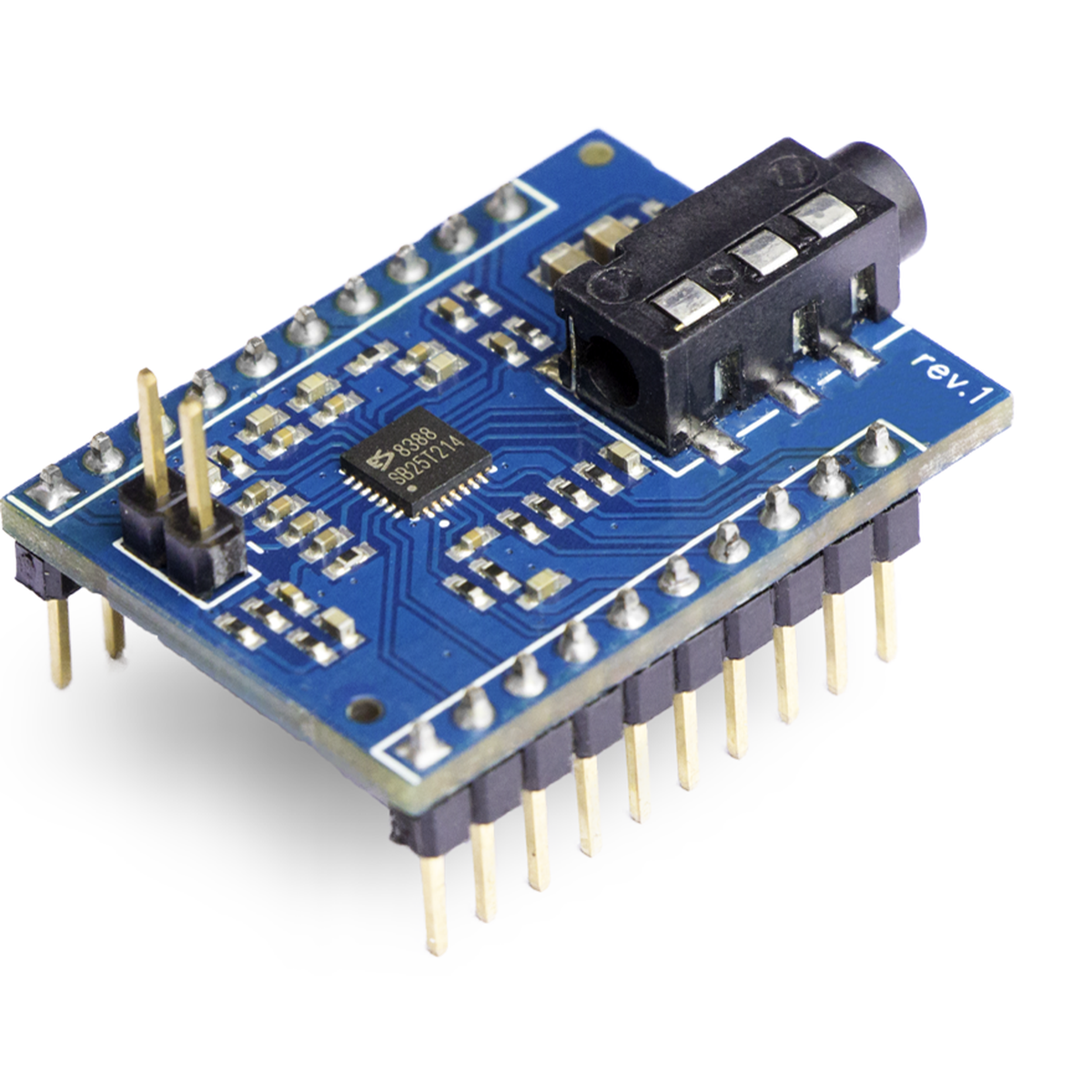
There are no predefined pins, so you need to define them yourself:
#include "AudioTools.h"
#include "AudioLibs/I2SCodecStream.h"
I2SCodecStream i2s(GenericES8388);
void setup() {
// setup I2C
Wire.begin();
// setup i2s and codec
auto cfg = i2s.defaultConfig();
cfg.sample_rate = 44100;
cfg.bits_per_sample = 16;
cfg.channels = 2;
config.pin_bck = 14; // define your i2s pins
config.pin_ws = 15;
config.pin_data = 22;
i2s.begin();
// set volume
i2s.setVolume(0.5);
}
WM8960 Audio Codec Modules
There are no predefined pins, so you need to define them yourself:
#include "AudioTools.h"
#include "AudioLibs/I2SCodecStream.h"
I2SCodecStream i2s(GenericWM8960);
void setup() {
// setup I2C
Wire.begin();
// setup i2s and codec
auto cfg = i2s.defaultConfig();
cfg.sample_rate = 44100;
cfg.bits_per_sample = 16;
cfg.channels = 2;
config.pin_bck = 14; // define your i2s pins
config.pin_ws = 15;
config.pin_data = 22;
i2s.begin();
// set volume
i2s.setVolume(0.5);
}
PI WM8960 Hat
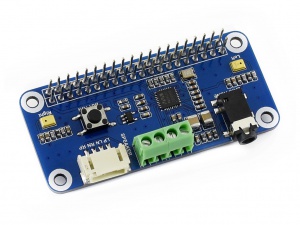
Pins
Func | Module | Description |
---|---|---|
5V | 5V | Power positive (5V power input) |
GND | GND | Power Ground |
SDA | GPIO2 | I2C data input |
SCL | GPIO3 | I2C clock Input |
CLK | GPIO18 | I2S bit clock input |
LRCLK | GPIO19 | I2S frame clock input |
DAC | GPIO21 | I2S serial data output |
ADC | GPIO20 | I2S serial data input |
Button | GPIO17 | Configurable Button |
Rasperry PI Zero Module Pins
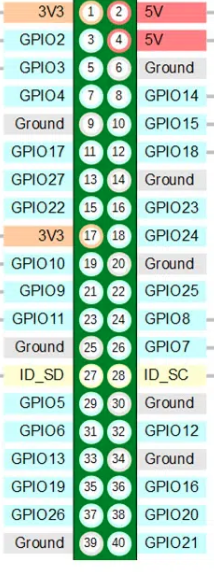
Connections
I suggest to use Wire.begin(19,21); to avoid any conflicts with the I2S pins used by the AudioTools:
Func | Module | e.g ESP32 |
---|---|---|
VCC | 3.3V | 3.3V |
GND | GND | GND |
SDA | GPIO 2/SDA | GPIO 19 |
SCL | GPIO 3/SCL | GPIO 21 |
CLK | GPIO 18 | GPIO 14 |
LRCLK | GPIO 19 | GPIO 15 |
DAC | GPIO 21 | GPIO 22 |
ADC | GPIO 20 | GPIO 32 |
Button | GPIO 17 | - |
WM8960 Audio Board
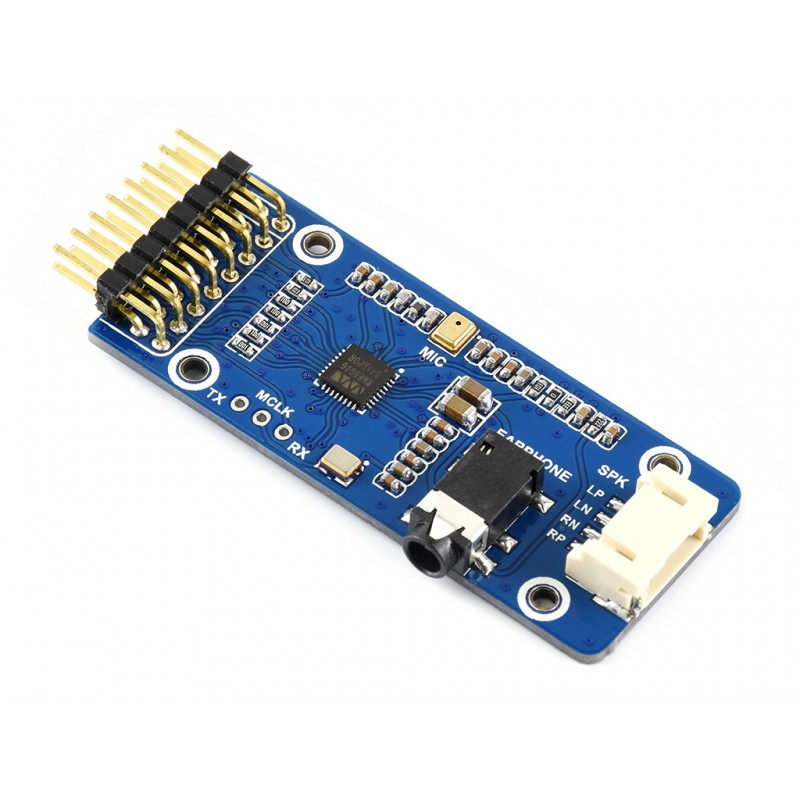
I suggest to use Wire.begin(19,21); to avoid any conflicts with the I2S pins used by the AudioTools:
Func | Module | e.g. ESP32 |
---|---|---|
VCC | 3.3V | |
GND | GND | GND |
SDA | SDA | GPIO 19 |
SCL | SCL | GPIO 21 |
CLK | CLK | GPIO 14 |
LRCLK | WS | GPIO 15 |
DAC | RXSDA | GPIO 22 |
ADC | TXSDA | GPIO 32 |
Boards using SPI
VS1053 Audio Codec Modules
Not all Microcontrollers support I2S and many do not have enough memory or speed to do audio decoding. In this case you can use a VS1053 based module which can decode Ogg Vorbis, MP3, AAC, WMA, FLAC and MIDI Audio. The boards are using SPI and most boards provide also a built in SD drive.
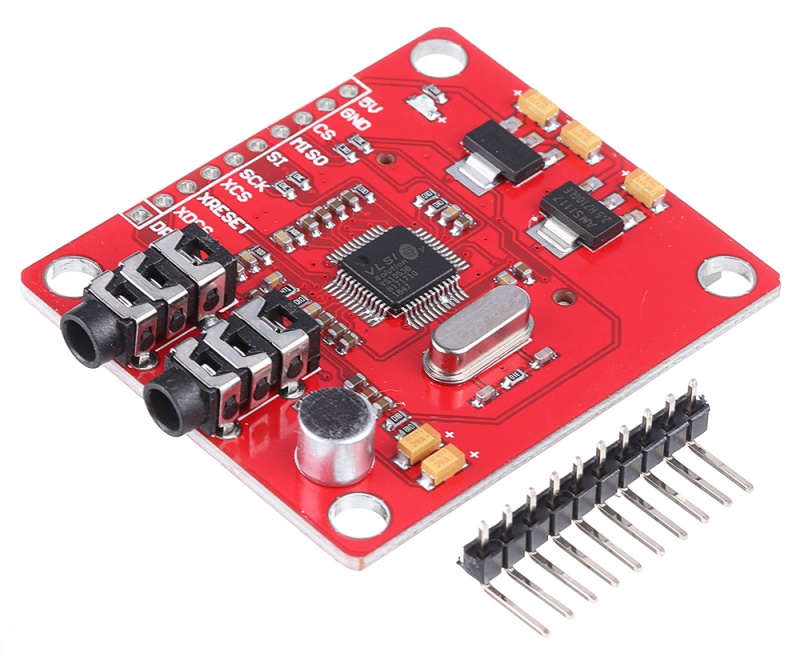
The VS1053Stream class can be used as audio sink and you can use any SD library to access the audio files.
Here are some examples.
Dependencies: Details how to install the required HAL library can be found in the arduino-vs1053 project
Analog Boards
ESP32 Arduino LVGL WIFI&Bluetooth Development Board 2.4inch LCD TFT Module
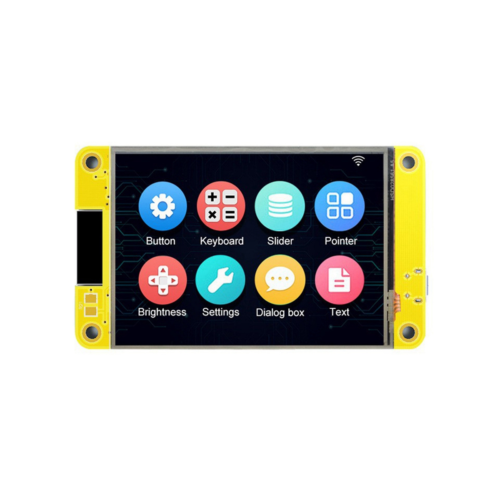
This is a cool ESP32 based board that comes with
- a 2.4inch LCD TFT Module with capacitive touch
- a built in mono amplifier
- a SD drive
Just connect a speaker to the SPEAK pins and select the AnalogAudioStream as output device!