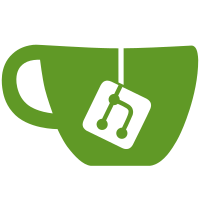
This also updates the spec for `NodePackageImporter` to correctly document indicate that errors should be thrown during construction. See sass/dart-sass#2178
7.4 KiB
Compile API
These APIs are the entrypoints for compiling Sass to CSS.
import {RawSourceMap} from 'source-map-js'; // https://www.npmjs.com/package/source-map-js
import {Options, StringOptions} from './options';
Table of Contents
Types
CompileResult
The object returned by the compiler when a Sass compilation succeeds.
export interface CompileResult {
css: string;
loadedUrls: URL[];
sourceMap?: RawSourceMap;
}
Compiler
The class returned by creating a synchronous compiler with initCompiler()
.
If the class is directly constructed (as opposed to the Compiler being created
via initCompiler
), throw an error.
Only synchronous compilation methods compile()
and compileString()
must
be included, and must have identical semantics to the Sass interface.
export class Compiler {
private constructor();
compile(path: string, options?: Options<'sync'>): CompileResult;
compileString(source: string, options?: StringOptions<'sync'>): CompileResult;
dispose()
When dispose
is invoked on a Compiler:
- Any subsequent invocations of
compile
andcompileString
must throw an error.
dispose(): void;
}
AsyncCompiler
The object returned by creating an asynchronous compiler with
initAsyncCompiler()
. If the class is directly constructed (as opposed to the
AsyncCompiler being created via initAsyncCompiler
), throw an error.
Only asynchronous compilation methods compileAsync()
and
compileStringAsync()
must be included, and must have identical semantics to
the Sass interface.
export class AsyncCompiler {
private constructor();
compileAsync(
path: string,
options?: Options<'async'>
): Promise<CompileResult>;
compileStringAsync(
source: string,
options?: StringOptions<'async'>
): Promise<CompileResult>;
dispose()
When dispose
is invoked on an Async Compiler:
-
Any subsequent invocations of
compileAsync
andcompileStringAsync
must throw an error. -
Any compilations that have not yet been settled must be allowed to settle, and not be cancelled.
-
Resolves a Promise when all compilations have been settled, and disposal is complete.
dispose(): Promise<void>;
}
Functions
compile
Compiles the Sass file at path
:
-
If any item in
options.importers
is an instance of theNodePackageImporter
class:-
If no filesystem is available, throw an error.
This primarily refers to a browser environment, but applies to other sandboxed JavaScript environments as well.
-
Let
pkgImporter
be a Node Package Importer with an associatedentryPointURL
of the absolute file URL for the object'sentryPointDirectory
. -
Replace the item with
pkgImporter
in a copy ofoptions.importers
.
-
-
If any object in
options.importers
has bothfindFileUrl
andcanonicalize
fields, throw an error. -
Let
css
be the result of compilingpath
withoptions.importers
asimporters
andoptions.loadPaths
asload-paths
. The compiler must respect the configuration specified by theoptions
object. -
If the compilation succeeds, return a
CompileResult
object composed as follows:-
Set
CompileResult.css
tocss
. -
Set
CompileResult.loadedUrls
to a list of unique canonical URLs of source files loaded during the compilation. The order of URLs is not guaranteed. -
If
options.sourceMap
istrue
, setCompileResult.sourceMap
to a sourceMap object describing how sections of the Sass input correspond to sections of the CSS output.The structure of the sourceMap can vary from implementation to implementation.
-
-
Otherwise, throw an
Exception
.
export function compile(path: string, options?: Options<'sync'>): CompileResult;
compileAsync
Like compile
, but runs asynchronously.
The compiler must support asynchronous plugins when running in this mode.
export function compileAsync(
path: string,
options?: Options<'async'>
): Promise<CompileResult>;
compileString
Compiles the Sass source
:
-
If any item in
options.importers
is an instance of theNodePackageImporter
class:-
If no filesystem is available, throw an error.
This primarily refers to a browser environment, but applies to other sandboxed JavaScript environments as well.
-
Let
pkgImporter
be a Node Package Importer with an associatedentryPointURL
of the absolute file URL for the object'sentryPointDirectory
. -
Replace the item with
pkgImporter
in a copy ofoptions.importers
.
-
-
If
options.importer
or any object inoptions.importers
has bothfindFileUrl
andcanonicalize
fields, throw an error. -
Let
css
be the result of compiling a string with:options.source
asstring
;options.syntax
assyntax
, or "scss" ifoptions.syntax
is not set;options.url
asurl
;options.importer
asimporter
;options.importers
asimporters
;options.loadPaths
asload-paths
.
The compiler must respect the configuration specified by the
options
object. -
If the compilation succeeds, return a
CompileResult
object composed as follows:-
Set
CompileResult.css
tocss
. -
Set
CompileResult.loadedUrls
to a list of unique canonical URLs of source files loaded during the compilation. The order of URLs is not guaranteed.- If
options.url
is set, include it in the list. - Otherwise, do not include a URL for
source
.
- If
-
If
options.sourceMap
istrue
, setCompileResult.sourceMap
to a sourceMap object describing how sections of the Sass input correspond to sections of the CSS output.The structure of the sourceMap can vary from implementation to implementation.
-
-
If the compilation fails, throw an
Exception
.
export function compileString(
source: string,
options?: StringOptions<'sync'>
): CompileResult;
compileStringAsync
Like compileString
, but runs asynchronously.
The compiler must support asynchronous plugins when running in this mode.
export function compileStringAsync(
source: string,
options?: StringOptions<'async'>
): Promise<CompileResult>;
initCompiler
Returns a synchronous Compiler.
export function initCompiler(): Compiler;
initAsyncCompiler
Resolves with an Async Compiler.
export function initAsyncCompiler(): Promise<AsyncCompiler>;