mirror of
https://github.com/immich-app/immich.git
synced 2024-09-22 02:57:26 +00:00
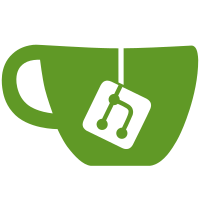
* feat(server): better error messages * chore: open api * chore: remove debug log * fix: syntax error * fix: e2e test
10 KiB
Generated
10 KiB
Generated
openapi.api.APIKeyApi
Load the API package
import 'package:openapi/api.dart';
All URIs are relative to /api
Method | HTTP request | Description |
---|---|---|
createApiKey | POST /api-key | |
deleteApiKey | DELETE /api-key/{id} | |
getApiKey | GET /api-key/{id} | |
getApiKeys | GET /api-key | |
updateApiKey | PUT /api-key/{id} |
createApiKey
APIKeyCreateResponseDto createApiKey(aPIKeyCreateDto)
Example
import 'package:openapi/api.dart';
// TODO Configure API key authorization: cookie
//defaultApiClient.getAuthentication<ApiKeyAuth>('cookie').apiKey = 'YOUR_API_KEY';
// uncomment below to setup prefix (e.g. Bearer) for API key, if needed
//defaultApiClient.getAuthentication<ApiKeyAuth>('cookie').apiKeyPrefix = 'Bearer';
// TODO Configure API key authorization: api_key
//defaultApiClient.getAuthentication<ApiKeyAuth>('api_key').apiKey = 'YOUR_API_KEY';
// uncomment below to setup prefix (e.g. Bearer) for API key, if needed
//defaultApiClient.getAuthentication<ApiKeyAuth>('api_key').apiKeyPrefix = 'Bearer';
// TODO Configure HTTP Bearer authorization: bearer
// Case 1. Use String Token
//defaultApiClient.getAuthentication<HttpBearerAuth>('bearer').setAccessToken('YOUR_ACCESS_TOKEN');
// Case 2. Use Function which generate token.
// String yourTokenGeneratorFunction() { ... }
//defaultApiClient.getAuthentication<HttpBearerAuth>('bearer').setAccessToken(yourTokenGeneratorFunction);
final api_instance = APIKeyApi();
final aPIKeyCreateDto = APIKeyCreateDto(); // APIKeyCreateDto |
try {
final result = api_instance.createApiKey(aPIKeyCreateDto);
print(result);
} catch (e) {
print('Exception when calling APIKeyApi->createApiKey: $e\n');
}
Parameters
Name | Type | Description | Notes |
---|---|---|---|
aPIKeyCreateDto | APIKeyCreateDto |
Return type
Authorization
HTTP request headers
- Content-Type: application/json
- Accept: application/json
[Back to top] [Back to API list] [Back to Model list] [Back to README]
deleteApiKey
deleteApiKey(id)
Example
import 'package:openapi/api.dart';
// TODO Configure API key authorization: cookie
//defaultApiClient.getAuthentication<ApiKeyAuth>('cookie').apiKey = 'YOUR_API_KEY';
// uncomment below to setup prefix (e.g. Bearer) for API key, if needed
//defaultApiClient.getAuthentication<ApiKeyAuth>('cookie').apiKeyPrefix = 'Bearer';
// TODO Configure API key authorization: api_key
//defaultApiClient.getAuthentication<ApiKeyAuth>('api_key').apiKey = 'YOUR_API_KEY';
// uncomment below to setup prefix (e.g. Bearer) for API key, if needed
//defaultApiClient.getAuthentication<ApiKeyAuth>('api_key').apiKeyPrefix = 'Bearer';
// TODO Configure HTTP Bearer authorization: bearer
// Case 1. Use String Token
//defaultApiClient.getAuthentication<HttpBearerAuth>('bearer').setAccessToken('YOUR_ACCESS_TOKEN');
// Case 2. Use Function which generate token.
// String yourTokenGeneratorFunction() { ... }
//defaultApiClient.getAuthentication<HttpBearerAuth>('bearer').setAccessToken(yourTokenGeneratorFunction);
final api_instance = APIKeyApi();
final id = 38400000-8cf0-11bd-b23e-10b96e4ef00d; // String |
try {
api_instance.deleteApiKey(id);
} catch (e) {
print('Exception when calling APIKeyApi->deleteApiKey: $e\n');
}
Parameters
Name | Type | Description | Notes |
---|---|---|---|
id | String |
Return type
void (empty response body)
Authorization
HTTP request headers
- Content-Type: Not defined
- Accept: Not defined
[Back to top] [Back to API list] [Back to Model list] [Back to README]
getApiKey
APIKeyResponseDto getApiKey(id)
Example
import 'package:openapi/api.dart';
// TODO Configure API key authorization: cookie
//defaultApiClient.getAuthentication<ApiKeyAuth>('cookie').apiKey = 'YOUR_API_KEY';
// uncomment below to setup prefix (e.g. Bearer) for API key, if needed
//defaultApiClient.getAuthentication<ApiKeyAuth>('cookie').apiKeyPrefix = 'Bearer';
// TODO Configure API key authorization: api_key
//defaultApiClient.getAuthentication<ApiKeyAuth>('api_key').apiKey = 'YOUR_API_KEY';
// uncomment below to setup prefix (e.g. Bearer) for API key, if needed
//defaultApiClient.getAuthentication<ApiKeyAuth>('api_key').apiKeyPrefix = 'Bearer';
// TODO Configure HTTP Bearer authorization: bearer
// Case 1. Use String Token
//defaultApiClient.getAuthentication<HttpBearerAuth>('bearer').setAccessToken('YOUR_ACCESS_TOKEN');
// Case 2. Use Function which generate token.
// String yourTokenGeneratorFunction() { ... }
//defaultApiClient.getAuthentication<HttpBearerAuth>('bearer').setAccessToken(yourTokenGeneratorFunction);
final api_instance = APIKeyApi();
final id = 38400000-8cf0-11bd-b23e-10b96e4ef00d; // String |
try {
final result = api_instance.getApiKey(id);
print(result);
} catch (e) {
print('Exception when calling APIKeyApi->getApiKey: $e\n');
}
Parameters
Name | Type | Description | Notes |
---|---|---|---|
id | String |
Return type
Authorization
HTTP request headers
- Content-Type: Not defined
- Accept: application/json
[Back to top] [Back to API list] [Back to Model list] [Back to README]
getApiKeys
List getApiKeys()
Example
import 'package:openapi/api.dart';
// TODO Configure API key authorization: cookie
//defaultApiClient.getAuthentication<ApiKeyAuth>('cookie').apiKey = 'YOUR_API_KEY';
// uncomment below to setup prefix (e.g. Bearer) for API key, if needed
//defaultApiClient.getAuthentication<ApiKeyAuth>('cookie').apiKeyPrefix = 'Bearer';
// TODO Configure API key authorization: api_key
//defaultApiClient.getAuthentication<ApiKeyAuth>('api_key').apiKey = 'YOUR_API_KEY';
// uncomment below to setup prefix (e.g. Bearer) for API key, if needed
//defaultApiClient.getAuthentication<ApiKeyAuth>('api_key').apiKeyPrefix = 'Bearer';
// TODO Configure HTTP Bearer authorization: bearer
// Case 1. Use String Token
//defaultApiClient.getAuthentication<HttpBearerAuth>('bearer').setAccessToken('YOUR_ACCESS_TOKEN');
// Case 2. Use Function which generate token.
// String yourTokenGeneratorFunction() { ... }
//defaultApiClient.getAuthentication<HttpBearerAuth>('bearer').setAccessToken(yourTokenGeneratorFunction);
final api_instance = APIKeyApi();
try {
final result = api_instance.getApiKeys();
print(result);
} catch (e) {
print('Exception when calling APIKeyApi->getApiKeys: $e\n');
}
Parameters
This endpoint does not need any parameter.
Return type
Authorization
HTTP request headers
- Content-Type: Not defined
- Accept: application/json
[Back to top] [Back to API list] [Back to Model list] [Back to README]
updateApiKey
APIKeyResponseDto updateApiKey(id, aPIKeyUpdateDto)
Example
import 'package:openapi/api.dart';
// TODO Configure API key authorization: cookie
//defaultApiClient.getAuthentication<ApiKeyAuth>('cookie').apiKey = 'YOUR_API_KEY';
// uncomment below to setup prefix (e.g. Bearer) for API key, if needed
//defaultApiClient.getAuthentication<ApiKeyAuth>('cookie').apiKeyPrefix = 'Bearer';
// TODO Configure API key authorization: api_key
//defaultApiClient.getAuthentication<ApiKeyAuth>('api_key').apiKey = 'YOUR_API_KEY';
// uncomment below to setup prefix (e.g. Bearer) for API key, if needed
//defaultApiClient.getAuthentication<ApiKeyAuth>('api_key').apiKeyPrefix = 'Bearer';
// TODO Configure HTTP Bearer authorization: bearer
// Case 1. Use String Token
//defaultApiClient.getAuthentication<HttpBearerAuth>('bearer').setAccessToken('YOUR_ACCESS_TOKEN');
// Case 2. Use Function which generate token.
// String yourTokenGeneratorFunction() { ... }
//defaultApiClient.getAuthentication<HttpBearerAuth>('bearer').setAccessToken(yourTokenGeneratorFunction);
final api_instance = APIKeyApi();
final id = 38400000-8cf0-11bd-b23e-10b96e4ef00d; // String |
final aPIKeyUpdateDto = APIKeyUpdateDto(); // APIKeyUpdateDto |
try {
final result = api_instance.updateApiKey(id, aPIKeyUpdateDto);
print(result);
} catch (e) {
print('Exception when calling APIKeyApi->updateApiKey: $e\n');
}
Parameters
Name | Type | Description | Notes |
---|---|---|---|
id | String | ||
aPIKeyUpdateDto | APIKeyUpdateDto |
Return type
Authorization
HTTP request headers
- Content-Type: application/json
- Accept: application/json
[Back to top] [Back to API list] [Back to Model list] [Back to README]