mirror of
https://github.com/php/php-src.git
synced 2024-10-05 16:56:11 +00:00
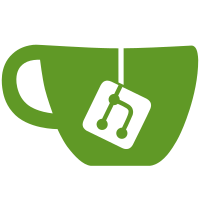
Add is_url field to wrapper structure; the stream wrapper openers will disallow opening is is_url && !PG(allow_url_fopen). Add infrastructure for stat($url) and opendir($url). Tidy up/centralize code that locates and instantiates wrappers for the various operations. Implement opendir for plain files. Make the PHP opendir and dir functions use the streams implementations. Add modelines for syntax highlighting the pear scripts in vim
226 lines
4.2 KiB
PHP
226 lines
4.2 KiB
PHP
#!@prefix@/bin/php -Cq
|
|
<?php // -*- PHP -*-
|
|
main($argc, $argv, $_ENV);
|
|
|
|
// {{{ main()
|
|
|
|
function main(&$argc, &$argv, &$env)
|
|
{
|
|
global $debug;
|
|
$debug = false;
|
|
$file = check_options($argc, $argv, $env);
|
|
parse_package_file($file);
|
|
make_makefile_in($env);
|
|
}
|
|
|
|
// }}}
|
|
// {{{ check_options()
|
|
|
|
function check_options($argc, $argv, $env)
|
|
{
|
|
global $debug;
|
|
array_shift($argv);
|
|
while ($argv[0]{0} == '-' && $argv[0] != '-') {
|
|
$opt = array_shift($argv);
|
|
switch ($opt) {
|
|
case '--': {
|
|
break 2;
|
|
}
|
|
case '-d': {
|
|
$debug = true;
|
|
break;
|
|
}
|
|
default: {
|
|
die("pearize: unrecognized option `$opt'\n");
|
|
}
|
|
}
|
|
}
|
|
$file = array_shift($argv);
|
|
if (empty($file)) {
|
|
$file = "package.xml";
|
|
} elseif ($file == '-') {
|
|
$file = "php://stdin";
|
|
}
|
|
return $file;
|
|
}
|
|
|
|
// }}}
|
|
// {{{ make_makefile_in()
|
|
|
|
function make_makefile_in(&$env)
|
|
{
|
|
global $libdata, $debug;
|
|
if (sizeof($libdata) > 1) {
|
|
die("No support yet for multiple libraries in one package.\n");
|
|
}
|
|
|
|
if ($debug) {
|
|
$wp = fopen("php://stdout", "w");
|
|
} else {
|
|
$wp = @fopen("Makefile.in", "w");
|
|
}
|
|
if (is_resource($wp)) {
|
|
print "Creating Makefile.in...";
|
|
flush();
|
|
} else {
|
|
die("Could not create Makefile.in in current directory.\n");
|
|
}
|
|
|
|
$who = $env["USER"];
|
|
$when = gmdate('Y-m-d h:i');
|
|
fwrite($wp, "# This file was generated by `pearize' by $who at $when GMT\n\n");
|
|
|
|
foreach ($libdata as $lib => $info) {
|
|
extract($info);
|
|
fwrite($wp, "\
|
|
INCLUDES = $includes
|
|
LTLIBRARY_NAME = lib{$lib}.la
|
|
LTLIBRARY_SOURCES = $sources
|
|
LTLIBRARY_SHARED_NAME = {$lib}.la
|
|
LTLIBRARY_SHARED_LIBADD = $libadd
|
|
");
|
|
}
|
|
|
|
if (sizeof($libdata) > 0) {
|
|
fwrite($wp, "include \$(top_srcdir)/build/dynlib.mk\n");
|
|
}
|
|
fclose($wp);
|
|
print "done.\n";
|
|
}
|
|
|
|
// }}}
|
|
// {{{ parse_package_file()
|
|
|
|
function parse_package_file($file)
|
|
{
|
|
global $in_file, $curlib, $curelem, $libdata, $cdata;
|
|
global $currinstalldir, $baseinstalldir;
|
|
|
|
$in_file = false;
|
|
$curlib = '';
|
|
$curelem = '';
|
|
$libdata = array();
|
|
$cdata = array();
|
|
$baseinstalldir = array();
|
|
$currinstalldir = array();
|
|
|
|
$xp = xml_parser_create();
|
|
xml_set_element_handler($xp, "start_handler", "end_handler");
|
|
xml_set_character_data_handler($xp, "cdata_handler");
|
|
xml_parser_set_option($xp, XML_OPTION_CASE_FOLDING, false);
|
|
|
|
$fp = @fopen($file, "r");
|
|
if (!is_resource($fp)) {
|
|
die("Could not open file `$file'.\n");
|
|
}
|
|
while (!feof($fp)) {
|
|
xml_parse($xp, fread($fp, 2048), feof($fp));
|
|
}
|
|
xml_parser_free($xp);
|
|
}
|
|
|
|
// }}}
|
|
// {{{ start_handler()
|
|
|
|
function start_handler($xp, $elem, $attrs)
|
|
{
|
|
global $cdata, $in_file, $curelem, $curfile, $filerole;
|
|
global $baseinstalldir, $currinstalldir;
|
|
switch ($elem) {
|
|
case "file": {
|
|
$curfile = '';
|
|
$filerole = $attrs['role'];
|
|
switch ($filerole) {
|
|
case "ext": {
|
|
$in_file = true;
|
|
$cdata = array();
|
|
break;
|
|
}
|
|
case "php": default: {
|
|
break;
|
|
}
|
|
}
|
|
break;
|
|
}
|
|
case "dir": {
|
|
$cdir = $currinstalldir[sizeof($currinstalldir)-1];
|
|
$bdir = $baseinstalldir[sizeof($baseinstalldir)-1];
|
|
array_push($currinstalldir, "$cdir/{$attrs[name]}");
|
|
if (isset($attrs["baseinstalldir"])) {
|
|
array_push($baseinstalldir, "$bdir/{$attrs[baseinstalldir]}");
|
|
} else {
|
|
array_push($baseinstalldir, $bdir);
|
|
}
|
|
break;
|
|
}
|
|
case "includes":
|
|
case "libname":
|
|
case "libadd":
|
|
case "sources": {
|
|
$curelem = $elem;
|
|
break;
|
|
}
|
|
}
|
|
}
|
|
|
|
// }}}
|
|
// {{{ end_handler()
|
|
|
|
function end_handler($xp, $elem)
|
|
{
|
|
global $in_file, $curlib, $curelem, $libdata, $cdata;
|
|
global $baseinstalldir, $currinstalldir;
|
|
switch ($elem) {
|
|
case "file": {
|
|
if ($in_file === true) {
|
|
$libname = trim($cdata['libname']);
|
|
$libdata[$libname] = array(
|
|
"sources" => trim($cdata['sources']),
|
|
"includes" => trim($cdata['includes']),
|
|
"libadd" => trim($cdata['libadd']),
|
|
);
|
|
$in_file = false;
|
|
}
|
|
break;
|
|
}
|
|
case "dir": {
|
|
array_pop($currinstalldir);
|
|
array_pop($baseinstalldir);
|
|
break;
|
|
}
|
|
}
|
|
}
|
|
|
|
// }}}
|
|
// {{{ cdata_handler()
|
|
|
|
function cdata_handler($xp, $data)
|
|
{
|
|
global $curelem, $cdata, $curfile;
|
|
switch ($curelem) {
|
|
case "file": {
|
|
$curfile .= $data;
|
|
break;
|
|
}
|
|
case "includes":
|
|
case "libadd":
|
|
case "libname":
|
|
case "sources": {
|
|
$cdata[$curelem] .= $data;
|
|
break;
|
|
}
|
|
}
|
|
}
|
|
|
|
// }}}
|
|
|
|
/*
|
|
* Local variables:
|
|
* tab-width: 4
|
|
* c-basic-offset: 4
|
|
* indent-tabs-mode: t
|
|
* End:
|
|
*/
|
|
// vim600:syn=php
|
|
?>
|