mirror of
https://github.com/php/php-src.git
synced 2024-09-22 02:17:32 +00:00
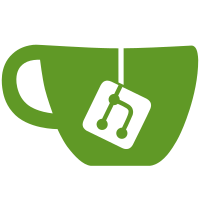
This deprecates passing null to non-nullable scale arguments of internal functions, with the eventual goal of making the behavior consistent with userland functions, where null is never accepted for non-nullable arguments. This change is expected to cause quite a lot of fallout. In most cases, calling code should be adjusted to avoid passing null. In some cases, PHP should be adjusted to make some function arguments nullable. I have already fixed a number of functions before landing this, but feel free to file a bug if you encounter a function that doesn't accept null, but probably should. (The rule of thumb for this to be applicable is that the function must have special behavior for 0 or "", which is distinct from the natural behavior of the parameter.) RFC: https://wiki.php.net/rfc/deprecate_null_to_scalar_internal_arg Closes GH-6475.
134 lines
2.9 KiB
PHP
134 lines
2.9 KiB
PHP
--TEST--
|
|
Test join() function : usage variations - unexpected values for 'glue' argument
|
|
--FILE--
|
|
<?php
|
|
/*
|
|
* testing join() by passing different unexpected value for glue argument
|
|
*/
|
|
|
|
echo "*** Testing join() : usage variations ***\n";
|
|
// initialize all required variables
|
|
$pieces = array("element1", "element2");
|
|
|
|
// get a resource variable
|
|
$fp = fopen(__FILE__, "r");
|
|
|
|
// define a class
|
|
class test
|
|
{
|
|
var $t = 10;
|
|
function __toString() {
|
|
return "testObject";
|
|
}
|
|
}
|
|
|
|
// array with different values
|
|
$values = array (
|
|
|
|
// integer values
|
|
0,
|
|
1,
|
|
12345,
|
|
-2345,
|
|
|
|
// float values
|
|
10.5,
|
|
-10.5,
|
|
10.1234567e10,
|
|
10.7654321E-10,
|
|
.5,
|
|
|
|
// array values
|
|
array(),
|
|
array(0),
|
|
array(1),
|
|
array(1, 2),
|
|
array('color' => 'red', 'item' => 'pen'),
|
|
|
|
// boolean values
|
|
true,
|
|
false,
|
|
TRUE,
|
|
FALSE,
|
|
|
|
// objects
|
|
new test(),
|
|
|
|
// empty string
|
|
"",
|
|
'',
|
|
|
|
// resource variable
|
|
$fp,
|
|
);
|
|
|
|
|
|
// loop through each element of the array and check the working of join()
|
|
// when $glue argument is supplied with different values
|
|
echo "\n--- Testing join() by supplying different values for 'glue' argument ---\n";
|
|
$counter = 1;
|
|
for($index = 0; $index < count($values); $index ++) {
|
|
echo "-- Iteration $counter --\n";
|
|
$glue = $values [$index];
|
|
|
|
try {
|
|
var_dump(join($glue, $pieces));
|
|
} catch (TypeError $exception) {
|
|
echo $exception->getMessage() . "\n";
|
|
}
|
|
|
|
$counter++;
|
|
}
|
|
|
|
echo "Done\n";
|
|
?>
|
|
--EXPECT--
|
|
*** Testing join() : usage variations ***
|
|
|
|
--- Testing join() by supplying different values for 'glue' argument ---
|
|
-- Iteration 1 --
|
|
string(17) "element10element2"
|
|
-- Iteration 2 --
|
|
string(17) "element11element2"
|
|
-- Iteration 3 --
|
|
string(21) "element112345element2"
|
|
-- Iteration 4 --
|
|
string(21) "element1-2345element2"
|
|
-- Iteration 5 --
|
|
string(20) "element110.5element2"
|
|
-- Iteration 6 --
|
|
string(21) "element1-10.5element2"
|
|
-- Iteration 7 --
|
|
string(28) "element1101234567000element2"
|
|
-- Iteration 8 --
|
|
string(29) "element11.07654321E-9element2"
|
|
-- Iteration 9 --
|
|
string(19) "element10.5element2"
|
|
-- Iteration 10 --
|
|
join(): Argument #1 ($separator) must be of type string, array given
|
|
-- Iteration 11 --
|
|
join(): Argument #1 ($separator) must be of type string, array given
|
|
-- Iteration 12 --
|
|
join(): Argument #1 ($separator) must be of type string, array given
|
|
-- Iteration 13 --
|
|
join(): Argument #1 ($separator) must be of type string, array given
|
|
-- Iteration 14 --
|
|
join(): Argument #1 ($separator) must be of type string, array given
|
|
-- Iteration 15 --
|
|
string(17) "element11element2"
|
|
-- Iteration 16 --
|
|
string(16) "element1element2"
|
|
-- Iteration 17 --
|
|
string(17) "element11element2"
|
|
-- Iteration 18 --
|
|
string(16) "element1element2"
|
|
-- Iteration 19 --
|
|
string(26) "element1testObjectelement2"
|
|
-- Iteration 20 --
|
|
string(16) "element1element2"
|
|
-- Iteration 21 --
|
|
string(16) "element1element2"
|
|
-- Iteration 22 --
|
|
join(): Argument #1 ($separator) must be of type array|string, resource given
|
|
Done
|