mirror of
https://github.com/php/php-src.git
synced 2024-09-21 18:07:23 +00:00
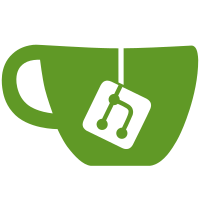
Shift pointers by ZEND_MM_ALIGNMENT_LOG2 to avoid the noticeable performance degradation caused by hash table collisions. in `EG(weakrefs)` and zend_weakmap->ht On 64-bit platforms, pointers are usually aligned to at least 8 bytes, so only one in 8 hash buckets were actually getting used. (With the metadata needed to track allocations, alignment might be at least 16 bytes in practice) Address review comments, add optimization Make it public for any extensions that need to work with EG(weakrefs) for instrumentation, debugging, etc. (e.g. zend_test) PHP 8.1 and previous releases would use the raw pointer value as a hash key instead.
69 lines
2.7 KiB
C
69 lines
2.7 KiB
C
/*
|
|
+----------------------------------------------------------------------+
|
|
| Copyright (c) The PHP Group |
|
|
+----------------------------------------------------------------------+
|
|
| This source file is subject to version 2.00 of the Zend license, |
|
|
| that is bundled with this package in the file LICENSE, and is |
|
|
| available through the world-wide-web at the following url: |
|
|
| http://www.zend.com/license/2_00.txt. |
|
|
| If you did not receive a copy of the Zend license and are unable to |
|
|
| obtain it through the world-wide-web, please send a note to |
|
|
| license@zend.com so we can mail you a copy immediately. |
|
|
+----------------------------------------------------------------------+
|
|
| Authors: krakjoe@php.net |
|
|
+----------------------------------------------------------------------+
|
|
*/
|
|
|
|
#ifndef ZEND_WEAKREFS_H
|
|
#define ZEND_WEAKREFS_H
|
|
|
|
#include "zend_alloc.h"
|
|
|
|
BEGIN_EXTERN_C()
|
|
|
|
extern ZEND_API zend_class_entry *zend_ce_weakref;
|
|
|
|
void zend_register_weakref_ce(void);
|
|
|
|
void zend_weakrefs_init(void);
|
|
void zend_weakrefs_shutdown(void);
|
|
|
|
ZEND_API void zend_weakrefs_notify(zend_object *object);
|
|
|
|
ZEND_API zval *zend_weakrefs_hash_add(HashTable *ht, zend_object *key, zval *pData);
|
|
ZEND_API zend_result zend_weakrefs_hash_del(HashTable *ht, zend_object *key);
|
|
static zend_always_inline void *zend_weakrefs_hash_add_ptr(HashTable *ht, zend_object *key, void *ptr) {
|
|
zval tmp, *zv;
|
|
ZVAL_PTR(&tmp, ptr);
|
|
if ((zv = zend_weakrefs_hash_add(ht, key, &tmp))) {
|
|
return Z_PTR_P(zv);
|
|
} else {
|
|
return NULL;
|
|
}
|
|
}
|
|
|
|
/* Because php uses the raw numbers as a hash function, raw pointers will lead to hash collisions.
|
|
* We have a guarantee that the lowest ZEND_MM_ALIGNED_OFFSET_LOG2 bits of a pointer are zero.
|
|
*
|
|
* E.g. On most 64-bit platforms, pointers are aligned to 8 bytes, so the least significant 3 bits are always 0 and can be discarded.
|
|
*
|
|
* NOTE: This function is only used for EG(weakrefs) and zend_weakmap->ht.
|
|
* It is not used for the HashTable instances associated with ZEND_WEAKREF_TAG_HT tags (created in zend_weakref_register, which uses ZEND_WEAKREF_ENCODE instead).
|
|
* The ZEND_WEAKREF_TAG_HT instances are used to disambiguate between multiple weak references to the same zend_object.
|
|
*/
|
|
static zend_always_inline zend_ulong zend_object_to_weakref_key(const zend_object *object)
|
|
{
|
|
ZEND_ASSERT(((uintptr_t)object) % ZEND_MM_ALIGNMENT == 0);
|
|
return ((uintptr_t) object) >> ZEND_MM_ALIGNMENT_LOG2;
|
|
}
|
|
|
|
static zend_always_inline zend_object *zend_weakref_key_to_object(zend_ulong key)
|
|
{
|
|
return (zend_object *) (((uintptr_t) key) << ZEND_MM_ALIGNMENT_LOG2);
|
|
}
|
|
|
|
END_EXTERN_C()
|
|
|
|
#endif
|
|
|