mirror of
https://github.com/php/php-src.git
synced 2024-09-21 18:07:23 +00:00
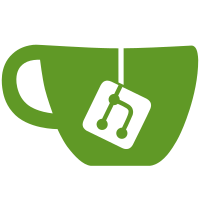
sigsetjmp use setjmp. Windows is of course weird in that it seems to have sigsetjmp but not sigjmp_buf (??) so force it to use setjmp in config.w32.h.in
282 lines
6.2 KiB
C
282 lines
6.2 KiB
C
/*
|
|
+----------------------------------------------------------------------+
|
|
| Zend Engine |
|
|
+----------------------------------------------------------------------+
|
|
| Copyright (c) 1998-2008 Zend Technologies Ltd. (http://www.zend.com) |
|
|
+----------------------------------------------------------------------+
|
|
| This source file is subject to version 2.00 of the Zend license, |
|
|
| that is bundled with this package in the file LICENSE, and is |
|
|
| available through the world-wide-web at the following url: |
|
|
| http://www.zend.com/license/2_00.txt. |
|
|
| If you did not receive a copy of the Zend license and are unable to |
|
|
| obtain it through the world-wide-web, please send a note to |
|
|
| license@zend.com so we can mail you a copy immediately. |
|
|
+----------------------------------------------------------------------+
|
|
| Authors: Andi Gutmans <andi@zend.com> |
|
|
| Zeev Suraski <zeev@zend.com> |
|
|
+----------------------------------------------------------------------+
|
|
*/
|
|
|
|
/* $Id$ */
|
|
|
|
#ifndef ZEND_GLOBALS_H
|
|
#define ZEND_GLOBALS_H
|
|
|
|
|
|
#include <setjmp.h>
|
|
|
|
#include "zend_globals_macros.h"
|
|
|
|
#include "zend_stack.h"
|
|
#include "zend_ptr_stack.h"
|
|
#include "zend_hash.h"
|
|
#include "zend_llist.h"
|
|
#include "zend_objects.h"
|
|
#include "zend_objects_API.h"
|
|
#include "zend_modules.h"
|
|
|
|
/* Define ZTS if you want a thread-safe Zend */
|
|
/*#undef ZTS*/
|
|
|
|
#ifdef ZTS
|
|
|
|
BEGIN_EXTERN_C()
|
|
ZEND_API extern int compiler_globals_id;
|
|
ZEND_API extern int executor_globals_id;
|
|
END_EXTERN_C()
|
|
|
|
#endif
|
|
|
|
#define SYMTABLE_CACHE_SIZE 32
|
|
|
|
|
|
#include "zend_compile.h"
|
|
|
|
/* excpt.h on Digital Unix 4.0 defines function_table */
|
|
#undef function_table
|
|
|
|
#define ZEND_EARLY_BINDING_COMPILE_TIME 0
|
|
#define ZEND_EARLY_BINDING_DELAYED 1
|
|
#define ZEND_EARLY_BINDING_DELAYED_ALL 2
|
|
|
|
typedef struct _zend_declarables {
|
|
zval ticks;
|
|
} zend_declarables;
|
|
|
|
typedef struct _zend_vm_stack *zend_vm_stack;
|
|
|
|
struct _zend_compiler_globals {
|
|
zend_stack bp_stack;
|
|
zend_stack switch_cond_stack;
|
|
zend_stack foreach_copy_stack;
|
|
zend_stack object_stack;
|
|
zend_stack declare_stack;
|
|
|
|
zend_class_entry *active_class_entry;
|
|
|
|
/* variables for list() compilation */
|
|
zend_llist list_llist;
|
|
zend_llist dimension_llist;
|
|
zend_stack list_stack;
|
|
|
|
zend_stack function_call_stack;
|
|
|
|
char *compiled_filename;
|
|
|
|
int zend_lineno;
|
|
int comment_start_line;
|
|
char *heredoc;
|
|
int heredoc_len;
|
|
|
|
zend_op_array *active_op_array;
|
|
|
|
HashTable *function_table; /* function symbol table */
|
|
HashTable *class_table; /* class table */
|
|
|
|
HashTable filenames_table;
|
|
|
|
HashTable *auto_globals;
|
|
|
|
zend_bool in_compilation;
|
|
zend_bool short_tags;
|
|
zend_bool asp_tags;
|
|
zend_bool allow_call_time_pass_reference;
|
|
|
|
zend_declarables declarables;
|
|
|
|
zend_bool unclean_shutdown;
|
|
|
|
zend_bool ini_parser_unbuffered_errors;
|
|
|
|
zend_llist open_files;
|
|
|
|
long catch_begin;
|
|
|
|
struct _zend_ini_parser_param *ini_parser_param;
|
|
|
|
int interactive;
|
|
|
|
zend_uint start_lineno;
|
|
zend_bool increment_lineno;
|
|
|
|
znode implementing_class;
|
|
|
|
zend_uint access_type;
|
|
|
|
char *doc_comment;
|
|
zend_uint doc_comment_len;
|
|
|
|
zend_uint compiler_options; /* set of ZEND_COMPILE_* constants */
|
|
|
|
zval *current_namespace;
|
|
HashTable *current_import;
|
|
|
|
#ifdef ZTS
|
|
HashTable **static_members;
|
|
int last_static_member;
|
|
#endif
|
|
};
|
|
|
|
|
|
struct _zend_executor_globals {
|
|
zval **return_value_ptr_ptr;
|
|
|
|
zval uninitialized_zval;
|
|
zval *uninitialized_zval_ptr;
|
|
|
|
zval error_zval;
|
|
zval *error_zval_ptr;
|
|
|
|
zend_ptr_stack arg_types_stack;
|
|
|
|
/* symbol table cache */
|
|
HashTable *symtable_cache[SYMTABLE_CACHE_SIZE];
|
|
HashTable **symtable_cache_limit;
|
|
HashTable **symtable_cache_ptr;
|
|
|
|
zend_op **opline_ptr;
|
|
|
|
HashTable *active_symbol_table;
|
|
HashTable symbol_table; /* main symbol table */
|
|
|
|
HashTable included_files; /* files already included */
|
|
|
|
JMP_BUF *bailout;
|
|
|
|
int error_reporting;
|
|
int orig_error_reporting;
|
|
int exit_status;
|
|
|
|
zend_op_array *active_op_array;
|
|
|
|
HashTable *function_table; /* function symbol table */
|
|
HashTable *class_table; /* class table */
|
|
HashTable *zend_constants; /* constants table */
|
|
|
|
zend_class_entry *scope;
|
|
zend_class_entry *called_scope; /* Scope of the calling class */
|
|
|
|
zval *This;
|
|
|
|
long precision;
|
|
|
|
int ticks_count;
|
|
|
|
zend_bool in_execution;
|
|
HashTable *in_autoload;
|
|
zend_function *autoload_func;
|
|
zend_bool full_tables_cleanup;
|
|
|
|
/* for extended information support */
|
|
zend_bool no_extensions;
|
|
|
|
#ifdef ZEND_WIN32
|
|
zend_bool timed_out;
|
|
#endif
|
|
|
|
HashTable regular_list;
|
|
HashTable persistent_list;
|
|
|
|
zend_vm_stack argument_stack;
|
|
|
|
int user_error_handler_error_reporting;
|
|
zval *user_error_handler;
|
|
zval *user_error_handler_old;
|
|
zval *user_exception_handler;
|
|
zend_stack user_error_handlers_error_reporting;
|
|
zend_ptr_stack user_error_handlers;
|
|
zend_ptr_stack user_exception_handlers;
|
|
|
|
zend_error_handling_t error_handling;
|
|
zend_class_entry *exception_class;
|
|
|
|
/* timeout support */
|
|
int timeout_seconds;
|
|
|
|
int lambda_count;
|
|
|
|
HashTable *ini_directives;
|
|
HashTable *modified_ini_directives;
|
|
|
|
zend_objects_store objects_store;
|
|
zval *exception;
|
|
zend_op *opline_before_exception;
|
|
zend_op exception_op[3];
|
|
|
|
struct _zend_execute_data *current_execute_data;
|
|
|
|
struct _zend_module_entry *current_module;
|
|
|
|
zend_property_info std_property_info;
|
|
|
|
zend_bool active;
|
|
|
|
void *reserved[ZEND_MAX_RESERVED_RESOURCES];
|
|
};
|
|
|
|
struct _zend_ini_scanner_globals {
|
|
zend_file_handle *yy_in;
|
|
zend_file_handle *yy_out;
|
|
|
|
unsigned int yy_leng;
|
|
unsigned char *yy_start;
|
|
unsigned char *yy_text;
|
|
unsigned char *yy_cursor;
|
|
unsigned char *yy_marker;
|
|
unsigned char *yy_limit;
|
|
int yy_state;
|
|
zend_stack state_stack;
|
|
|
|
char *filename;
|
|
int lineno;
|
|
|
|
/* Modes are: ZEND_INI_SCANNER_NORMAL, ZEND_INI_SCANNER_RAW */
|
|
int scanner_mode;
|
|
};
|
|
|
|
struct _zend_php_scanner_globals {
|
|
zend_file_handle *yy_in;
|
|
zend_file_handle *yy_out;
|
|
|
|
unsigned int yy_leng;
|
|
unsigned char *yy_start;
|
|
unsigned char *yy_text;
|
|
unsigned char *yy_cursor;
|
|
unsigned char *yy_marker;
|
|
unsigned char *yy_limit;
|
|
int yy_state;
|
|
zend_stack state_stack;
|
|
|
|
zend_llist used_state_stacks;
|
|
};
|
|
|
|
#endif /* ZEND_GLOBALS_H */
|
|
|
|
/*
|
|
* Local variables:
|
|
* tab-width: 4
|
|
* c-basic-offset: 4
|
|
* indent-tabs-mode: t
|
|
* End:
|
|
*/
|