mirror of
https://github.com/php/php-src.git
synced 2024-10-17 14:32:37 +00:00
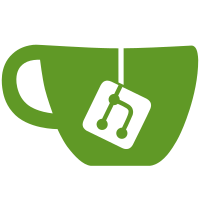
proto bool PDOStatement::closeCursor() Closes the cursor, leaving the statement ready for re-execution. The purpose of the function is to free up the connection to the server so that other queries may be issued, but leaving the statement in a state that it can be re-executed. This is implemented either as an optional driver specific method (allowing for maximum efficiency), or as the generic PDO fallback if no driver specific function is installed. The PDO generic fallback is semantically the same as writing the following code in your PHP script: do { while ($stmt->fetch()) ; if (!$stmt->nextRowset()) break; } while (true);
199 lines
3.5 KiB
PHP
199 lines
3.5 KiB
PHP
--TEST--
|
|
PDO Common: PDO_FETCH_BOUND
|
|
--SKIPIF--
|
|
<?php # vim:ft=php
|
|
if (!extension_loaded('pdo')) die('skip');
|
|
$dir = getenv('REDIR_TEST_DIR');
|
|
if (false == $dir) die('skip no driver');
|
|
require_once $dir . 'pdo_test.inc';
|
|
PDOTest::skip();
|
|
?>
|
|
--FILE--
|
|
<?php
|
|
require getenv('REDIR_TEST_DIR') . 'pdo_test.inc';
|
|
$db = PDOTest::factory();
|
|
|
|
$db->exec('CREATE TABLE test(idx int NOT NULL PRIMARY KEY, txt VARCHAR(20))');
|
|
$db->exec('INSERT INTO test VALUES(0, \'String0\')');
|
|
$db->exec('INSERT INTO test VALUES(1, \'String1\')');
|
|
$db->exec('INSERT INTO test VALUES(2, \'String2\')');
|
|
|
|
$stmt1 = $db->prepare('SELECT COUNT(idx) FROM test');
|
|
$stmt2 = $db->prepare('SELECT idx, txt FROM test ORDER by idx');
|
|
|
|
$stmt1->execute();
|
|
var_dump($stmt1->fetchColumn());
|
|
$stmt1 = null;
|
|
|
|
$stmt2->execute();
|
|
$cont = $stmt2->fetchAll(PDO_FETCH_COLUMN|PDO_FETCH_UNIQUE);
|
|
var_dump($cont);
|
|
|
|
echo "===WHILE===\n";
|
|
|
|
$stmt2->bindColumn('idx', $idx);
|
|
$stmt2->bindColumn('txt', $txt);
|
|
$stmt2->execute();
|
|
|
|
while($stmt2->fetch(PDO_FETCH_BOUND)) {
|
|
var_dump(array($idx=>$txt));
|
|
}
|
|
|
|
echo "===ALONE===\n";
|
|
|
|
$stmt3 = $db->prepare('SELECT txt FROM test WHERE idx=:inp');
|
|
$stmt3->bindParam(':inp', $idx); /* by foreign name */
|
|
|
|
$stmt4 = $db->prepare('SELECT idx FROM test WHERE txt=:txt');
|
|
$stmt4->bindParam(':txt', $txt); /* using same name */
|
|
|
|
foreach($cont as $idx => $txt)
|
|
{
|
|
var_dump(array($idx=>$txt));
|
|
var_dump($stmt3->execute());
|
|
|
|
if ($idx == 0) {
|
|
/* portability-wise, you may only bindColumn()s
|
|
* after execute() has been called at least once */
|
|
$stmt3->bindColumn('txt', $col1);
|
|
}
|
|
var_dump($stmt3->fetch(PDO_FETCH_BOUND));
|
|
$stmt3->closeCursor();
|
|
|
|
var_dump($stmt4->execute());
|
|
if ($idx == 0) {
|
|
/* portability-wise, you may only bindColumn()s
|
|
* after execute() has been called at least once */
|
|
$stmt4->bindColumn('idx', $col2);
|
|
}
|
|
var_dump($stmt4->fetch(PDO_FETCH_BOUND));
|
|
$stmt4->closeCursor();
|
|
var_dump(array($col2=>$col1));
|
|
}
|
|
|
|
echo "===REBIND/SAME===\n";
|
|
|
|
$stmt4->bindColumn('idx', $col1);
|
|
|
|
foreach($cont as $idx => $txt)
|
|
{
|
|
var_dump(array($idx=>$txt));
|
|
var_dump($stmt3->execute());
|
|
var_dump($stmt3->fetch(PDO_FETCH_BOUND));
|
|
$stmt3->closeCursor();
|
|
var_dump($col1);
|
|
var_dump($stmt4->execute());
|
|
var_dump($stmt4->fetch(PDO_FETCH_BOUND));
|
|
$stmt4->closeCursor();
|
|
var_dump($col1);
|
|
}
|
|
|
|
echo "===REBIND/CONFLICT===\n";
|
|
|
|
$stmt2->bindColumn('idx', $col1);
|
|
$stmt2->bindColumn('txt', $col1);
|
|
$stmt2->execute();
|
|
|
|
while($stmt2->fetch(PDO_FETCH_BOUND))
|
|
{
|
|
var_dump($col1);
|
|
}
|
|
|
|
|
|
?>
|
|
--EXPECT--
|
|
string(1) "3"
|
|
array(3) {
|
|
[0]=>
|
|
string(7) "String0"
|
|
[1]=>
|
|
string(7) "String1"
|
|
[2]=>
|
|
string(7) "String2"
|
|
}
|
|
===WHILE===
|
|
array(1) {
|
|
[0]=>
|
|
string(7) "String0"
|
|
}
|
|
array(1) {
|
|
[1]=>
|
|
string(7) "String1"
|
|
}
|
|
array(1) {
|
|
[2]=>
|
|
string(7) "String2"
|
|
}
|
|
===ALONE===
|
|
array(1) {
|
|
[0]=>
|
|
string(7) "String0"
|
|
}
|
|
bool(true)
|
|
bool(true)
|
|
bool(true)
|
|
bool(true)
|
|
array(1) {
|
|
[0]=>
|
|
string(7) "String0"
|
|
}
|
|
array(1) {
|
|
[1]=>
|
|
string(7) "String1"
|
|
}
|
|
bool(true)
|
|
bool(true)
|
|
bool(true)
|
|
bool(true)
|
|
array(1) {
|
|
[1]=>
|
|
string(7) "String1"
|
|
}
|
|
array(1) {
|
|
[2]=>
|
|
string(7) "String2"
|
|
}
|
|
bool(true)
|
|
bool(true)
|
|
bool(true)
|
|
bool(true)
|
|
array(1) {
|
|
[2]=>
|
|
string(7) "String2"
|
|
}
|
|
===REBIND/SAME===
|
|
array(1) {
|
|
[0]=>
|
|
string(7) "String0"
|
|
}
|
|
bool(true)
|
|
bool(true)
|
|
string(7) "String0"
|
|
bool(true)
|
|
bool(true)
|
|
string(1) "0"
|
|
array(1) {
|
|
[1]=>
|
|
string(7) "String1"
|
|
}
|
|
bool(true)
|
|
bool(true)
|
|
string(7) "String1"
|
|
bool(true)
|
|
bool(true)
|
|
string(1) "1"
|
|
array(1) {
|
|
[2]=>
|
|
string(7) "String2"
|
|
}
|
|
bool(true)
|
|
bool(true)
|
|
string(7) "String2"
|
|
bool(true)
|
|
bool(true)
|
|
string(1) "2"
|
|
===REBIND/CONFLICT===
|
|
string(7) "String0"
|
|
string(7) "String1"
|
|
string(7) "String2"
|