mirror of
https://github.com/php/php-src.git
synced 2024-09-21 18:07:23 +00:00
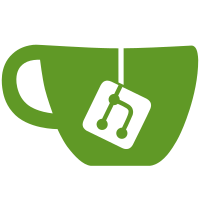
Support acquiring a Closure to a callable using the syntax func(...), $obj->method(...), etc. This is essentially a shortcut for Closure::fromCallable(). RFC: https://wiki.php.net/rfc/first_class_callable_syntax Closes GH-7019. Co-Authored-By: Nikita Popov <nikita.ppv@gmail.com>
58 lines
1.2 KiB
PHP
58 lines
1.2 KiB
PHP
--TEST--
|
|
Trying to acquire callable to something that's not callable
|
|
--FILE--
|
|
<?php
|
|
|
|
class Test {
|
|
private static function privateMethod() {}
|
|
|
|
public function instanceMethod() {}
|
|
}
|
|
|
|
try {
|
|
$fn = 123;
|
|
$fn(...);
|
|
} catch (Error $e) {
|
|
echo $e->getMessage(), "\n";
|
|
}
|
|
try {
|
|
does_not_exist(...);
|
|
} catch (Error $e) {
|
|
echo $e->getMessage(), "\n";
|
|
}
|
|
try {
|
|
stdClass::doesNotExist(...);
|
|
} catch (Error $e) {
|
|
echo $e->getMessage(), "\n";
|
|
}
|
|
try {
|
|
(new stdClass)->doesNotExist(...);
|
|
} catch (Error $e) {
|
|
echo $e->getMessage(), "\n";
|
|
}
|
|
try {
|
|
[new stdClass, 'doesNotExist'](...);
|
|
} catch (Error $e) {
|
|
echo $e->getMessage(), "\n";
|
|
}
|
|
try {
|
|
Test::privateMethod(...);
|
|
} catch (Error $e) {
|
|
echo $e->getMessage(), "\n";
|
|
}
|
|
try {
|
|
Test::instanceMethod(...);
|
|
} catch (Error $e) {
|
|
echo $e->getMessage(), "\n";
|
|
}
|
|
|
|
?>
|
|
--EXPECT--
|
|
Value of type int is not callable
|
|
Call to undefined function does_not_exist()
|
|
Call to undefined method stdClass::doesNotExist()
|
|
Call to undefined method stdClass::doesNotExist()
|
|
Call to undefined method stdClass::doesNotExist()
|
|
Call to private method Test::privateMethod() from global scope
|
|
Non-static method Test::instanceMethod() cannot be called statically
|