mirror of
https://github.com/php/php-src.git
synced 2024-09-22 10:27:25 +00:00
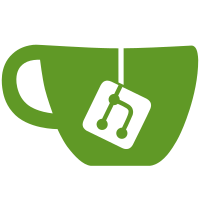
* Modify php_hash_ops to contain the algorithm name and serialize and unserialize methods. * Implement __serialize and __unserialize magic methods on HashContext. Note that serialized HashContexts are not necessarily portable between PHP versions or from architecture to architecture. (Most are, though Keccak and slow SHA3s are not.) An exception is thrown when an unsupported serialization is attempted. Because of security concerns, HASH_HMAC contexts are not currently serializable; attempting to serialize one throws an exception. Serialization exposes the state of HashContext memory, so ensure that memory is zeroed before use by allocating it with a new php_hash_alloc_context function. Performance impact is negligible. Some hash internal states have logical pointers into a buffer, or sponge, that absorbs input provided in bytes rather than chunks. The unserialize functions for these hash functions must validate that the logical pointers are all within bounds, lest future hash operations cause out-of-bounds memory accesses. * Adler32, CRC32, FNV, joaat: simple state, no buffer positions * Gost, MD2, SHA3, Snefru, Tiger, Whirlpool: buffer positions must be validated * MD4, MD5, SHA1, SHA2, haval, ripemd: buffer positions encoded bitwise, forced to within bounds on use; no need to validate
57 lines
2.1 KiB
C
57 lines
2.1 KiB
C
/*
|
|
+----------------------------------------------------------------------+
|
|
| Copyright (c) The PHP Group |
|
|
+----------------------------------------------------------------------+
|
|
| This source file is subject to version 3.01 of the PHP license, |
|
|
| that is bundled with this package in the file LICENSE, and is |
|
|
| available through the world-wide-web at the following url: |
|
|
| http://www.php.net/license/3_01.txt |
|
|
| If you did not receive a copy of the PHP license and are unable to |
|
|
| obtain it through the world-wide-web, please send a note to |
|
|
| license@php.net so we can mail you a copy immediately. |
|
|
+----------------------------------------------------------------------+
|
|
| Author: Sara Golemon <pollita@php.net> |
|
|
+----------------------------------------------------------------------+
|
|
*/
|
|
|
|
#ifndef PHP_HASH_HAVAL_H
|
|
#define PHP_HASH_HAVAL_H
|
|
|
|
#include "ext/standard/basic_functions.h"
|
|
/* HAVAL context. */
|
|
typedef struct {
|
|
uint32_t state[8];
|
|
uint32_t count[2];
|
|
unsigned char buffer[128];
|
|
|
|
char passes;
|
|
short output;
|
|
void (*Transform)(uint32_t state[8], const unsigned char block[128]);
|
|
} PHP_HAVAL_CTX;
|
|
#define PHP_HAVAL_SPEC "l8l2b128"
|
|
|
|
#define PHP_HASH_HAVAL_INIT_DECL(p,b) PHP_HASH_API void PHP_##p##HAVAL##b##Init(PHP_HAVAL_CTX *); \
|
|
PHP_HASH_API void PHP_HAVAL##b##Final(unsigned char*, PHP_HAVAL_CTX *);
|
|
|
|
PHP_HASH_API void PHP_HAVALUpdate(PHP_HAVAL_CTX *, const unsigned char *, size_t);
|
|
|
|
PHP_HASH_HAVAL_INIT_DECL(3,128)
|
|
PHP_HASH_HAVAL_INIT_DECL(3,160)
|
|
PHP_HASH_HAVAL_INIT_DECL(3,192)
|
|
PHP_HASH_HAVAL_INIT_DECL(3,224)
|
|
PHP_HASH_HAVAL_INIT_DECL(3,256)
|
|
|
|
PHP_HASH_HAVAL_INIT_DECL(4,128)
|
|
PHP_HASH_HAVAL_INIT_DECL(4,160)
|
|
PHP_HASH_HAVAL_INIT_DECL(4,192)
|
|
PHP_HASH_HAVAL_INIT_DECL(4,224)
|
|
PHP_HASH_HAVAL_INIT_DECL(4,256)
|
|
|
|
PHP_HASH_HAVAL_INIT_DECL(5,128)
|
|
PHP_HASH_HAVAL_INIT_DECL(5,160)
|
|
PHP_HASH_HAVAL_INIT_DECL(5,192)
|
|
PHP_HASH_HAVAL_INIT_DECL(5,224)
|
|
PHP_HASH_HAVAL_INIT_DECL(5,256)
|
|
|
|
#endif
|