mirror of
https://github.com/php/php-src.git
synced 2024-09-22 02:17:32 +00:00
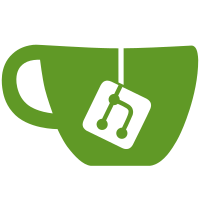
Writing to a proprety that hasn't been declared is deprecated, unless the class uses the #[AllowDynamicProperties] attribute or defines __get()/__set(). RFC: https://wiki.php.net/rfc/deprecate_dynamic_properties
86 lines
1.8 KiB
PHP
86 lines
1.8 KiB
PHP
--TEST--
|
|
Unsetting and recreating private properties.
|
|
--FILE--
|
|
<?php
|
|
class C {
|
|
private $p = 'test';
|
|
function unsetPrivate() {
|
|
unset($this->p);
|
|
}
|
|
function setPrivate() {
|
|
$this->p = 'changed';
|
|
}
|
|
}
|
|
|
|
#[AllowDynamicProperties]
|
|
class D extends C {
|
|
function setP() {
|
|
$this->p = 'changed in D';
|
|
}
|
|
}
|
|
|
|
echo "Unset and recreate a superclass's private property:\n";
|
|
$d = new D;
|
|
$d->unsetPrivate();
|
|
$d->setPrivate();
|
|
var_dump($d);
|
|
|
|
echo "\nUnset superclass's private property, and recreate it as public in subclass:\n";
|
|
$d = new D;
|
|
$d->unsetPrivate();
|
|
$d->setP();
|
|
var_dump($d);
|
|
|
|
echo "\nUnset superclass's private property, and recreate it as public at global scope:\n";
|
|
$d = new D;
|
|
$d->unsetPrivate();
|
|
$d->p = 'this will create a public property';
|
|
var_dump($d);
|
|
|
|
|
|
echo "\n\nUnset and recreate a private property:\n";
|
|
$c = new C;
|
|
$c->unsetPrivate();
|
|
$c->setPrivate();
|
|
var_dump($c);
|
|
|
|
echo "\nUnset a private property, and attempt to recreate at global scope (expecting failure):\n";
|
|
$c = new C;
|
|
$c->unsetPrivate();
|
|
$c->p = 'this will fail';
|
|
var_dump($c);
|
|
?>
|
|
===DONE===
|
|
--EXPECTF--
|
|
Unset and recreate a superclass's private property:
|
|
object(D)#%d (1) {
|
|
["p":"C":private]=>
|
|
string(7) "changed"
|
|
}
|
|
|
|
Unset superclass's private property, and recreate it as public in subclass:
|
|
object(D)#%d (1) {
|
|
["p"]=>
|
|
string(12) "changed in D"
|
|
}
|
|
|
|
Unset superclass's private property, and recreate it as public at global scope:
|
|
object(D)#%d (1) {
|
|
["p"]=>
|
|
string(34) "this will create a public property"
|
|
}
|
|
|
|
|
|
Unset and recreate a private property:
|
|
object(C)#%d (1) {
|
|
["p":"C":private]=>
|
|
string(7) "changed"
|
|
}
|
|
|
|
Unset a private property, and attempt to recreate at global scope (expecting failure):
|
|
|
|
Fatal error: Uncaught Error: Cannot access private property C::$p in %s:%d
|
|
Stack trace:
|
|
#0 {main}
|
|
thrown in %s on line %d
|