mirror of
https://github.com/php/php-src.git
synced 2024-09-21 18:07:23 +00:00
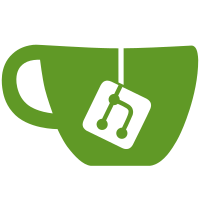
First, the limitation already doesn't trigger if you copy the whole file (i.e. use copy() or stream_copy_to_stream() and don't specify a length). This happens because length will be 0 at the time of the check and only later calculated based on the file size. This means that we're already completely blowing the length limit for what is likely the most common case, and it doesn't seem like anyone complained about that. Second, the premise of the code comment ("to avoid runaway swapping") seems incorrect to me. Because this performs a file-backed non-private mmap, no swap backing is needed for the mapping. Concerns over "memory usage" are also misplaced, as this is a virtual mapping.
59 lines
2.1 KiB
C
59 lines
2.1 KiB
C
/*
|
|
+----------------------------------------------------------------------+
|
|
| PHP Version 7 |
|
|
+----------------------------------------------------------------------+
|
|
| Copyright (c) The PHP Group |
|
|
+----------------------------------------------------------------------+
|
|
| This source file is subject to version 3.01 of the PHP license, |
|
|
| that is bundled with this package in the file LICENSE, and is |
|
|
| available through the world-wide-web at the following url: |
|
|
| http://www.php.net/license/3_01.txt |
|
|
| If you did not receive a copy of the PHP license and are unable to |
|
|
| obtain it through the world-wide-web, please send a note to |
|
|
| license@php.net so we can mail you a copy immediately. |
|
|
+----------------------------------------------------------------------+
|
|
| Author: Wez Furlong <wez@thebrainroom.com> |
|
|
+----------------------------------------------------------------------+
|
|
*/
|
|
|
|
/* Memory Mapping interface for streams */
|
|
#include "php.h"
|
|
#include "php_streams_int.h"
|
|
|
|
PHPAPI char *_php_stream_mmap_range(php_stream *stream, size_t offset, size_t length, php_stream_mmap_access_t mode, size_t *mapped_len)
|
|
{
|
|
php_stream_mmap_range range;
|
|
|
|
range.offset = offset;
|
|
range.length = length;
|
|
range.mode = mode;
|
|
range.mapped = NULL;
|
|
|
|
if (PHP_STREAM_OPTION_RETURN_OK == php_stream_set_option(stream, PHP_STREAM_OPTION_MMAP_API, PHP_STREAM_MMAP_MAP_RANGE, &range)) {
|
|
if (mapped_len) {
|
|
*mapped_len = range.length;
|
|
}
|
|
return range.mapped;
|
|
}
|
|
return NULL;
|
|
}
|
|
|
|
PHPAPI int _php_stream_mmap_unmap(php_stream *stream)
|
|
{
|
|
return php_stream_set_option(stream, PHP_STREAM_OPTION_MMAP_API, PHP_STREAM_MMAP_UNMAP, NULL) == PHP_STREAM_OPTION_RETURN_OK;
|
|
}
|
|
|
|
PHPAPI int _php_stream_mmap_unmap_ex(php_stream *stream, zend_off_t readden)
|
|
{
|
|
int ret = 1;
|
|
|
|
if (php_stream_seek(stream, readden, SEEK_CUR) != 0) {
|
|
ret = 0;
|
|
}
|
|
if (php_stream_mmap_unmap(stream) == 0) {
|
|
ret = 0;
|
|
}
|
|
|
|
return ret;
|
|
}
|