mirror of
https://github.com/php/php-src.git
synced 2024-09-21 09:57:23 +00:00
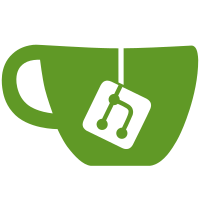
Cf. <https://github.com/php/php-src/pull/10220#issuecomment-1383739816>. This reverts commit45a128c9de
. This reverts commit1eb71c3f15
. This reverts commit492523a779
. This reverts commitc7a4633891
. This reverts commit308adb915c
. This reverts commitcd27d5e07f
. This reverts commitc5933409b4
. This reverts commit46371f4eb3
. This reverts commit623e2e9fc6
. This reverts commite7434c1247
. This reverts commitd28d323ca2
. This reverts commit1a067b84ee
. This reverts commita55c0c5fc3
. This reverts commitb5aeb3a4d4
. This reverts commitf061a035e4
. This reverts commitb088575119
. This reverts commitb1d48774a7
. This reverts commit94f9a20ce6
. This reverts commit4831e48708
. This reverts commitcd985de190
. This reverts commit9521d21681
. This reverts commitd6136151e9
.
98 lines
3.7 KiB
C
98 lines
3.7 KiB
C
/*
|
|
+----------------------------------------------------------------------+
|
|
| Zend OPcache |
|
|
+----------------------------------------------------------------------+
|
|
| Copyright (c) The PHP Group |
|
|
+----------------------------------------------------------------------+
|
|
| This source file is subject to version 3.01 of the PHP license, |
|
|
| that is bundled with this package in the file LICENSE, and is |
|
|
| available through the world-wide-web at the following url: |
|
|
| https://www.php.net/license/3_01.txt |
|
|
| If you did not receive a copy of the PHP license and are unable to |
|
|
| obtain it through the world-wide-web, please send a note to |
|
|
| license@php.net so we can mail you a copy immediately. |
|
|
+----------------------------------------------------------------------+
|
|
| Authors: Andi Gutmans <andi@php.net> |
|
|
| Zeev Suraski <zeev@php.net> |
|
|
| Stanislav Malyshev <stas@zend.com> |
|
|
| Dmitry Stogov <dmitry@php.net> |
|
|
+----------------------------------------------------------------------+
|
|
*/
|
|
|
|
#ifndef ZEND_ACCELERATOR_HASH_H
|
|
#define ZEND_ACCELERATOR_HASH_H
|
|
|
|
#include "zend.h"
|
|
|
|
/*
|
|
zend_accel_hash - is a hash table allocated in shared memory and
|
|
distributed across simultaneously running processes. The hash tables have
|
|
fixed sizen selected during construction by zend_accel_hash_init(). All the
|
|
hash entries are preallocated in the 'hash_entries' array. 'num_entries' is
|
|
initialized by zero and grows when new data is added.
|
|
zend_accel_hash_update() just takes the next entry from 'hash_entries'
|
|
array and puts it into appropriate place of 'hash_table'.
|
|
Hash collisions are resolved by separate chaining with linked lists,
|
|
however, entries are still taken from the same 'hash_entries' array.
|
|
'key' and 'data' passed to zend_accel_hash_update() must be already
|
|
allocated in shared memory. Few keys may be resolved to the same data.
|
|
using 'indirect' entries, that point to other entries ('data' is actually
|
|
a pointer to another zend_accel_hash_entry).
|
|
zend_accel_hash_update() requires exclusive lock, however,
|
|
zend_accel_hash_find() does not.
|
|
*/
|
|
|
|
typedef struct _zend_accel_hash_entry zend_accel_hash_entry;
|
|
|
|
struct _zend_accel_hash_entry {
|
|
zend_ulong hash_value;
|
|
zend_string *key;
|
|
zend_accel_hash_entry *next;
|
|
void *data;
|
|
bool indirect;
|
|
};
|
|
|
|
typedef struct _zend_accel_hash {
|
|
zend_accel_hash_entry **hash_table;
|
|
zend_accel_hash_entry *hash_entries;
|
|
uint32_t num_entries;
|
|
uint32_t max_num_entries;
|
|
uint32_t num_direct_entries;
|
|
} zend_accel_hash;
|
|
|
|
BEGIN_EXTERN_C()
|
|
|
|
void zend_accel_hash_init(zend_accel_hash *accel_hash, uint32_t hash_size);
|
|
void zend_accel_hash_clean(zend_accel_hash *accel_hash);
|
|
|
|
zend_accel_hash_entry* zend_accel_hash_update(
|
|
zend_accel_hash *accel_hash,
|
|
zend_string *key,
|
|
bool indirect,
|
|
void *data);
|
|
|
|
void* zend_accel_hash_find(
|
|
zend_accel_hash *accel_hash,
|
|
zend_string *key);
|
|
|
|
zend_accel_hash_entry* zend_accel_hash_find_entry(
|
|
zend_accel_hash *accel_hash,
|
|
zend_string *key);
|
|
|
|
int zend_accel_hash_unlink(
|
|
zend_accel_hash *accel_hash,
|
|
zend_string *key);
|
|
|
|
static inline bool zend_accel_hash_is_full(zend_accel_hash *accel_hash)
|
|
{
|
|
if (accel_hash->num_entries == accel_hash->max_num_entries) {
|
|
return 1;
|
|
} else {
|
|
return 0;
|
|
}
|
|
}
|
|
|
|
END_EXTERN_C()
|
|
|
|
#endif /* ZEND_ACCELERATOR_HASH_H */
|