mirror of
https://github.com/NginxProxyManager/nginx-proxy-manager.git
synced 2024-09-21 11:47:09 +00:00
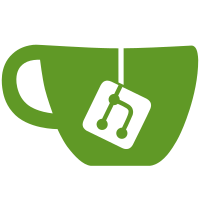
- Added a script to install every single plugin, used in development and debugging - Improved certbot plugin install commands - Adjusted some version for plugins to install properly - It's noted that some plugins require deps that do not match other plugins, however these use cases should be extremely rare
100 lines
2.6 KiB
JavaScript
100 lines
2.6 KiB
JavaScript
const _ = require('lodash');
|
|
const util = require('util');
|
|
|
|
module.exports = {
|
|
|
|
PermissionError: function (message, previous) {
|
|
Error.captureStackTrace(this, this.constructor);
|
|
this.name = this.constructor.name;
|
|
this.previous = previous;
|
|
this.message = 'Permission Denied';
|
|
this.public = true;
|
|
this.status = 403;
|
|
},
|
|
|
|
ItemNotFoundError: function (id, previous) {
|
|
Error.captureStackTrace(this, this.constructor);
|
|
this.name = this.constructor.name;
|
|
this.previous = previous;
|
|
this.message = 'Item Not Found - ' + id;
|
|
this.public = true;
|
|
this.status = 404;
|
|
},
|
|
|
|
AuthError: function (message, previous) {
|
|
Error.captureStackTrace(this, this.constructor);
|
|
this.name = this.constructor.name;
|
|
this.previous = previous;
|
|
this.message = message;
|
|
this.public = true;
|
|
this.status = 401;
|
|
},
|
|
|
|
InternalError: function (message, previous) {
|
|
Error.captureStackTrace(this, this.constructor);
|
|
this.name = this.constructor.name;
|
|
this.previous = previous;
|
|
this.message = message;
|
|
this.status = 500;
|
|
this.public = false;
|
|
},
|
|
|
|
InternalValidationError: function (message, previous) {
|
|
Error.captureStackTrace(this, this.constructor);
|
|
this.name = this.constructor.name;
|
|
this.previous = previous;
|
|
this.message = message;
|
|
this.status = 400;
|
|
this.public = false;
|
|
},
|
|
|
|
ConfigurationError: function (message, previous) {
|
|
Error.captureStackTrace(this, this.constructor);
|
|
this.name = this.constructor.name;
|
|
this.previous = previous;
|
|
this.message = message;
|
|
this.status = 400;
|
|
this.public = true;
|
|
},
|
|
|
|
CacheError: function (message, previous) {
|
|
Error.captureStackTrace(this, this.constructor);
|
|
this.name = this.constructor.name;
|
|
this.message = message;
|
|
this.previous = previous;
|
|
this.status = 500;
|
|
this.public = false;
|
|
},
|
|
|
|
ValidationError: function (message, previous) {
|
|
Error.captureStackTrace(this, this.constructor);
|
|
this.name = this.constructor.name;
|
|
this.previous = previous;
|
|
this.message = message;
|
|
this.public = true;
|
|
this.status = 400;
|
|
},
|
|
|
|
AssertionFailedError: function (message, previous) {
|
|
Error.captureStackTrace(this, this.constructor);
|
|
this.name = this.constructor.name;
|
|
this.previous = previous;
|
|
this.message = message;
|
|
this.public = false;
|
|
this.status = 400;
|
|
},
|
|
|
|
CommandError: function (stdErr, code, previous) {
|
|
Error.captureStackTrace(this, this.constructor);
|
|
this.name = this.constructor.name;
|
|
this.previous = previous;
|
|
this.message = stdErr;
|
|
this.code = code;
|
|
this.public = false;
|
|
},
|
|
};
|
|
|
|
_.forEach(module.exports, function (error) {
|
|
util.inherits(error, Error);
|
|
});
|