mirror of
https://github.com/librenms/librenms.git
synced 2024-09-21 10:28:13 +00:00
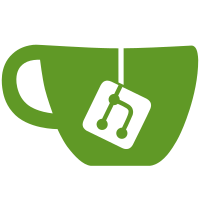
* WIP Device Ports porting to Laravel * WIP port links * Port Links WIP * Port Links * in_array -> isset * Add request to DeviceTab data * Add initial Pagination * Missing select component * Collapsed and expandable port neighbors New expandable component * Port sorting * Fix port transfer * Use menu entries to filter ports * Add translatable strings * style fixes and cleanup * update css * graph views and tidy controller basic port link view * cleanup * port row blade to reuse in legacy port view * Legacy tab url handling work properly in subdirectory remove includes from sub tab directory to prevent oddity * fallback to detail list when the view doesn't exist * Use named variable to simplify * Fix issue from file that was a symlink * Submenu handle sub items and query string urls * extract pageLinks to improve readability * fix typo * Apply fixes from StyleCI * phpstan was not happy using the relationship HasMany query * Don't allow *bps etc to be on a second line * Improve table on small screens * Fix sort --------- Co-authored-by: Tony Murray <murrant@users.noreply.github.com>
55 lines
1.6 KiB
PHP
55 lines
1.6 KiB
PHP
<?php
|
|
/**
|
|
* Ipv4Network.php
|
|
*
|
|
* -Description-
|
|
*
|
|
* This program is free software: you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation, either version 3 of the License, or
|
|
* (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE.See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program. If not, see <https://www.gnu.org/licenses/>.
|
|
*
|
|
* @link https://www.librenms.org
|
|
*
|
|
* @copyright 2018 Tony Murray
|
|
* @author Tony Murray <murraytony@gmail.com>
|
|
*/
|
|
|
|
namespace App\Models;
|
|
|
|
use Illuminate\Database\Eloquent\Factories\HasFactory;
|
|
use Illuminate\Database\Eloquent\Model;
|
|
use Illuminate\Database\Eloquent\Relations\HasMany;
|
|
use Illuminate\Database\Eloquent\Relations\HasManyThrough;
|
|
|
|
class Ipv4Network extends Model
|
|
{
|
|
use HasFactory;
|
|
|
|
public $timestamps = false;
|
|
protected $primaryKey = 'ipv4_network_id';
|
|
protected $fillable = [
|
|
'ipv4_network',
|
|
'context_name',
|
|
];
|
|
// ---- Define Relationships ----
|
|
|
|
public function ipv4(): HasMany
|
|
{
|
|
return $this->hasMany(Ipv4Address::class, 'ipv4_network_id');
|
|
}
|
|
|
|
public function connectedPorts(): HasManyThrough
|
|
{
|
|
return $this->hasManyThrough(Port::class, Ipv4Address::class, 'ipv4_network_id', 'port_id', 'ipv4_network_id', 'port_id');
|
|
}
|
|
}
|