mirror of
https://github.com/librenms/librenms.git
synced 2024-09-21 18:38:25 +00:00
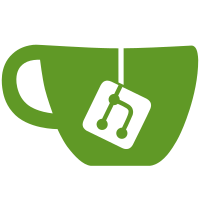
* WIP Sqlite Down methods don't work either, avoid them with refresh for now. WIP persistent support WIP db_schema WIP db_schema 2 Update new migrations... revert dump_db_schema changes for now, too much difference. fix migrations on mysql fix up some more items, this should be our target schema lots of index renames and a two misc changes index rename WIP index rename WIP another round fix up new schema changes try case insensitive fix Trying tests WIP fix down methods DBSetupTest working (uses mysql) Test sqlite migrations work * Properly validate sqlite output * revert glue changes, should be separate PR * remove dusk workaround * remove unused variables * import * sqlite capitalization * Revert some refresh tests * testing_mysql back to testing
187 lines
6.4 KiB
PHP
187 lines
6.4 KiB
PHP
<?php
|
|
|
|
/*
|
|
| !!!! DO NOT EDIT THIS FILE !!!!
|
|
|
|
|
| You can change settings by setting them in the environment or .env
|
|
| If there is something you need to change, but is not available as an environment setting,
|
|
| request an environment variable to be created upstream or send a pull request.
|
|
*/
|
|
|
|
use Illuminate\Support\Str;
|
|
use LibreNMS\Config;
|
|
|
|
$fallback_db_config = Config::getDatabaseSettings();
|
|
|
|
return [
|
|
|
|
/*
|
|
|--------------------------------------------------------------------------
|
|
| Default Database Connection Name
|
|
|--------------------------------------------------------------------------
|
|
|
|
|
| Here you may specify which of the database connections below you wish
|
|
| to use as your default connection for all database work. Of course
|
|
| you may use many connections at once using the Database library.
|
|
|
|
|
*/
|
|
|
|
'default' => env('DB_CONNECTION', env('DBTEST') ? 'testing' : 'mysql'),
|
|
|
|
/*
|
|
|--------------------------------------------------------------------------
|
|
| Database Connections
|
|
|--------------------------------------------------------------------------
|
|
|
|
|
| Here are each of the database connections setup for your application.
|
|
| Of course, examples of configuring each database platform that is
|
|
| supported by Laravel is shown below to make development simple.
|
|
|
|
|
|
|
|
| All database work in Laravel is done through the PHP PDO facilities
|
|
| so make sure you have the driver for your particular database of
|
|
| choice installed on your machine before you begin development.
|
|
|
|
|
*/
|
|
|
|
'connections' => [
|
|
|
|
'sqlite' => [
|
|
'driver' => 'sqlite',
|
|
'url' => env('DATABASE_URL'),
|
|
'database' => env('DB_DATABASE', storage_path('librenms.sqlite')),
|
|
'prefix' => '',
|
|
'foreign_key_constraints' => env('DB_FOREIGN_KEYS', true),
|
|
],
|
|
|
|
'mysql' => [
|
|
'driver' => 'mysql',
|
|
'url' => env('DATABASE_URL'),
|
|
'host' => env('DB_HOST', $fallback_db_config['db_host']),
|
|
'port' => env('DB_PORT', $fallback_db_config['db_port']),
|
|
'database' => env('DB_DATABASE', $fallback_db_config['db_name']),
|
|
'username' => env('DB_USERNAME', $fallback_db_config['db_user']),
|
|
'password' => env('DB_PASSWORD', $fallback_db_config['db_pass']),
|
|
'unix_socket' => env('DB_SOCKET', $fallback_db_config['db_socket']),
|
|
'charset' => 'utf8',
|
|
'collation' => 'utf8_unicode_ci',
|
|
'prefix' => '',
|
|
'prefix_indexes' => true,
|
|
'strict' => true,
|
|
'engine' => null,
|
|
'options' => extension_loaded('pdo_mysql') ? array_filter([
|
|
PDO::MYSQL_ATTR_SSL_CA => env('MYSQL_ATTR_SSL_CA'),
|
|
]) : [],
|
|
],
|
|
|
|
'testing' => [
|
|
'driver' => env('DB_TEST_DRIVER', 'mysql'),
|
|
'host' => env('DB_TEST_HOST', 'localhost'),
|
|
'port' => env('DB_TEST_PORT', ''),
|
|
'database' => env('DB_TEST_DATABASE', 'librenms_phpunit_78hunjuybybh'),
|
|
'username' => env('DB_TEST_USERNAME', 'root'),
|
|
'password' => env('DB_TEST_PASSWORD', ''),
|
|
'unix_socket' => env('DB_TEST_SOCKET', ''),
|
|
'charset' => 'utf8',
|
|
'collation' => 'utf8_unicode_ci',
|
|
'prefix' => '',
|
|
'strict' => true,
|
|
'engine' => null,
|
|
],
|
|
|
|
'pgsql' => [
|
|
'driver' => 'pgsql',
|
|
'url' => env('DATABASE_URL'),
|
|
'host' => env('DB_HOST', '127.0.0.1'),
|
|
'port' => env('DB_PORT', '5432'),
|
|
'database' => env('DB_DATABASE', 'forge'),
|
|
'username' => env('DB_USERNAME', 'forge'),
|
|
'password' => env('DB_PASSWORD', ''),
|
|
'charset' => 'utf8',
|
|
'prefix' => '',
|
|
'prefix_indexes' => true,
|
|
'schema' => 'public',
|
|
'sslmode' => 'prefer',
|
|
],
|
|
|
|
'sqlsrv' => [
|
|
'driver' => 'sqlsrv',
|
|
'url' => env('DATABASE_URL'),
|
|
'host' => env('DB_HOST', 'localhost'),
|
|
'port' => env('DB_PORT', '1433'),
|
|
'database' => env('DB_DATABASE', 'forge'),
|
|
'username' => env('DB_USERNAME', 'forge'),
|
|
'password' => env('DB_PASSWORD', ''),
|
|
'charset' => 'utf8',
|
|
'prefix' => '',
|
|
'prefix_indexes' => true,
|
|
],
|
|
|
|
'testing_memory' => [
|
|
'driver' => 'sqlite',
|
|
'database' => ':memory:',
|
|
'prefix' => '',
|
|
'foreign_key_constraints' => true,
|
|
],
|
|
|
|
'testing_persistent' => [
|
|
'driver' => 'sqlite',
|
|
'database' => storage_path('testing.sqlite'),
|
|
'prefix' => '',
|
|
'foreign_key_constraints' => true,
|
|
]
|
|
|
|
],
|
|
|
|
/*
|
|
|--------------------------------------------------------------------------
|
|
| Migration Repository Table
|
|
|--------------------------------------------------------------------------
|
|
|
|
|
| This table keeps track of all the migrations that have already run for
|
|
| your application. Using this information, we can determine which of
|
|
| the migrations on disk haven't actually been run in the database.
|
|
|
|
|
*/
|
|
|
|
'migrations' => 'migrations',
|
|
|
|
/*
|
|
|--------------------------------------------------------------------------
|
|
| Redis Databases
|
|
|--------------------------------------------------------------------------
|
|
|
|
|
| Redis is an open source, fast, and advanced key-value store that also
|
|
| provides a richer body of commands than a typical key-value system
|
|
| such as APC or Memcached. Laravel makes it easy to dig right in.
|
|
|
|
|
*/
|
|
|
|
'redis' => [
|
|
|
|
'client' => env('REDIS_CLIENT', 'predis'),
|
|
'options' => [
|
|
'cluster' => env('REDIS_CLUSTER', 'predis'),
|
|
'prefix' => env('REDIS_PREFIX', Str::slug(env('APP_NAME', 'laravel'), '_').'_database_'),
|
|
],
|
|
|
|
'default' => [
|
|
'url' => env('REDIS_URL'),
|
|
'host' => env('REDIS_HOST', '127.0.0.1'),
|
|
'password' => env('REDIS_PASSWORD', null),
|
|
'port' => env('REDIS_PORT', 6379),
|
|
'database' => env('REDIS_DB', 0),
|
|
],
|
|
|
|
'cache' => [
|
|
'url' => env('REDIS_URL'),
|
|
'host' => env('REDIS_HOST', '127.0.0.1'),
|
|
'password' => env('REDIS_PASSWORD', null),
|
|
'port' => env('REDIS_PORT', 6379),
|
|
'database' => env('REDIS_CACHE_DB', 1),
|
|
],
|
|
|
|
],
|
|
|
|
];
|