mirror of
https://github.com/librenms/librenms.git
synced 2024-09-21 18:38:25 +00:00
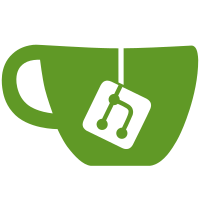
* Move assets to 5.7 location * Add 5.7 SVGs * add cache data dir * update QUEUE_DRIVER -> QUEUE_CONNECTION * Update trusted proxy config * update composer.json * 5.5 command loading * @php and @endphp can't be inline * Laravel 5.6 logging, Nice! * Update blade directives * improved redirects * remove unneeded service providers * Improved debugbar loading * no need to emulate renderable exceptions anymore * merge updated 5.7 files (WIP) * Enable CSRF * database_path() call causes issue in init.php * fix old testcase name * generic phpunit 7 fixes * add missed file_get_contents Keep migrations table content * fix duplicate key * Drop old php versions from travis-ci * remove hhvm * fix code climate message * remove use of deprecated function assertInternalType * Disable CSRF, we'll enable it separately. All forms need to be updated to work. * Update document references
100 lines
3.5 KiB
PHP
100 lines
3.5 KiB
PHP
<?php
|
|
/**
|
|
* RrdtoolTest.php
|
|
*
|
|
* Tests functionality of our rrdtool wrapper
|
|
*
|
|
* This program is free software: you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation, either version 3 of the License, or
|
|
* (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE.See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program. If not, see <http://www.gnu.org/licenses/>.
|
|
*
|
|
* @package LibreNMS
|
|
* @link http://librenms.org
|
|
* @copyright 2016 Tony Murray
|
|
* @author Tony Murray <murraytony@gmail.com>
|
|
*/
|
|
|
|
namespace LibreNMS\Tests;
|
|
|
|
class RrdtoolTest extends TestCase
|
|
{
|
|
|
|
public function testBuildCommandLocal()
|
|
{
|
|
global $config;
|
|
$config['rrdcached'] = '';
|
|
$config['rrdtool_version'] = '1.4';
|
|
$config['rrd_dir'] = '/opt/librenms/rrd';
|
|
|
|
$cmd = rrdtool_build_command('create', '/opt/librenms/rrd/f', 'o');
|
|
$this->assertEquals('create /opt/librenms/rrd/f o', $cmd);
|
|
|
|
$cmd = rrdtool_build_command('tune', '/opt/librenms/rrd/f', 'o');
|
|
$this->assertEquals('tune /opt/librenms/rrd/f o', $cmd);
|
|
|
|
$cmd = rrdtool_build_command('update', '/opt/librenms/rrd/f', 'o');
|
|
$this->assertEquals('update /opt/librenms/rrd/f o', $cmd);
|
|
|
|
|
|
$config['rrdtool_version'] = '1.6';
|
|
|
|
$cmd = rrdtool_build_command('create', '/opt/librenms/rrd/f', 'o');
|
|
$this->assertEquals('create /opt/librenms/rrd/f o -O', $cmd);
|
|
|
|
$cmd = rrdtool_build_command('tune', '/opt/librenms/rrd/f', 'o');
|
|
$this->assertEquals('tune /opt/librenms/rrd/f o', $cmd);
|
|
|
|
$cmd = rrdtool_build_command('update', '/opt/librenms/rrd/f', 'o');
|
|
$this->assertEquals('update /opt/librenms/rrd/f o', $cmd);
|
|
}
|
|
|
|
public function testBuildCommandRemote()
|
|
{
|
|
global $config;
|
|
$config['rrdcached'] = 'server:42217';
|
|
$config['rrdtool_version'] = '1.4';
|
|
$config['rrd_dir'] = '/opt/librenms/rrd';
|
|
|
|
$cmd = rrdtool_build_command('create', '/opt/librenms/rrd/f', 'o');
|
|
$this->assertEquals('create /opt/librenms/rrd/f o', $cmd);
|
|
|
|
$cmd = rrdtool_build_command('tune', '/opt/librenms/rrd/f', 'o');
|
|
$this->assertEquals('tune /opt/librenms/rrd/f o', $cmd);
|
|
|
|
$cmd = rrdtool_build_command('update', '/opt/librenms/rrd/f', 'o');
|
|
$this->assertEquals('update f o --daemon server:42217', $cmd);
|
|
|
|
|
|
$config['rrdtool_version'] = '1.6';
|
|
|
|
$cmd = rrdtool_build_command('create', '/opt/librenms/rrd/f', 'o');
|
|
$this->assertEquals('create f o -O --daemon server:42217', $cmd);
|
|
|
|
$cmd = rrdtool_build_command('tune', '/opt/librenms/rrd/f', 'o');
|
|
$this->assertEquals('tune f o --daemon server:42217', $cmd);
|
|
|
|
$cmd = rrdtool_build_command('update', '/opt/librenms/rrd/f', 'o');
|
|
$this->assertEquals('update f o --daemon server:42217', $cmd);
|
|
}
|
|
|
|
public function testBuildCommandException()
|
|
{
|
|
global $config;
|
|
$config['rrdcached'] = '';
|
|
$config['rrdtool_version'] = '1.4';
|
|
|
|
$this->expectException('LibreNMS\Exceptions\FileExistsException');
|
|
// use this file, since it is guaranteed to exist
|
|
rrdtool_build_command('create', __FILE__, 'o');
|
|
}
|
|
}
|