mirror of
https://github.com/librenms/librenms.git
synced 2024-09-21 10:28:13 +00:00
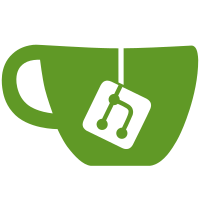
* Install bouncer * Seeder and level migration * Display and edit roles * remove unused deluser page * Update Radius and SSO to assign roles * update AlertUtil direct level check to use roles instead * rewrite ircbot auth handling * Remove legacy auth getUserlist and getUserlevel methods, add getRoles Set roles in LegacyUserProvider * Small cleanups * centralize role sync code show roles on user preferences page * VueSelect component WIP and a little docs * WIP * SelectControllers id and text fields. * LibrenmsSelect component extracted from SettingSelectDynamic * Handle multiple selections * allow type coercion * full width settings * final style adjustments * Final compiled assets update * Style fixes * Fix SSO tests * Lint cleanups * small style fix * don't use json yet * Update baseline for usptream package issues * Change schema, not 100% sure it is correct not sure why xor doesn't work
64 lines
1.4 KiB
PHP
64 lines
1.4 KiB
PHP
<?php
|
|
|
|
namespace App\Policies;
|
|
|
|
use App\Models\User;
|
|
use Illuminate\Auth\Access\HandlesAuthorization;
|
|
|
|
class UserPolicy
|
|
{
|
|
use HandlesAuthorization;
|
|
|
|
/**
|
|
* Determine whether the user can view the user.
|
|
*
|
|
* @param User $user
|
|
* @param User $target
|
|
*/
|
|
public function view(User $user, User $target): ?bool
|
|
{
|
|
return $target->is($user) ?: null; // allow users to view themselves
|
|
}
|
|
|
|
/**
|
|
* Determine whether the user can create users.
|
|
*
|
|
* @param User $user
|
|
*/
|
|
public function create(User $user): ?bool
|
|
{
|
|
// if not mysql, forbid, otherwise defer to bouncer
|
|
if (\LibreNMS\Config::get('auth_mechanism') != 'mysql') {
|
|
return false;
|
|
}
|
|
|
|
return null;
|
|
}
|
|
|
|
/**
|
|
* Determine whether the user can update the user.
|
|
*
|
|
* @param User $user
|
|
* @param User $target
|
|
*/
|
|
public function update(User $user, User $target = null): ?bool
|
|
{
|
|
if ($target == null) {
|
|
return null;
|
|
}
|
|
|
|
return $target->is($user) ?: null; // allow user to update self or defer to bouncer
|
|
}
|
|
|
|
/**
|
|
* Determine whether the user can delete the user.
|
|
*
|
|
* @param User $user
|
|
* @param User $target
|
|
*/
|
|
public function delete(User $user, User $target): ?bool
|
|
{
|
|
return $target->is($user) ? false : null; // do not allow users to delete themselves or defer to bouncer
|
|
}
|
|
}
|