mirror of
https://github.com/librenms/librenms.git
synced 2024-09-21 18:38:25 +00:00
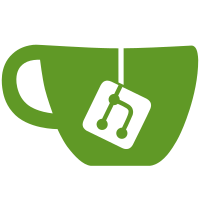
* initial work on add the ability to save/fetch app data * update to use get_app_data for ZFS * update the poller for the new app_data stuff * ZFS now logs changes to pools * add schema update for app_data stuff * small formatting fix * add a missing \ * now adds a column * sql-schema is no longer used, so remove the file that was added here * misc cleanups * rename the method in database/migrations/2022_07_03_1947_add_app_data.php * hopefully fix the migration bit * add the column to misc/db_schema.yaml * more misc small DB fixes * update the test as the json column uses collat of utf8mb4_bin * revert the last change and try manually setting it to what is expected * remove a extra ; * update suricata as well * correct the instance -> instances in one location to prevent the old instance list from being stomped * remove a extra ; * update fail2ban to use it as well * remove two unused functions as suricata and fail2ban no longer use components * style cleanup * postgres poller updated to use it * update html side of the postgres bits * chronyd now uses app data bits now as well * portactivity now uses it as well * style fix * sort the returned arrays from app_data * correct log message for port activity * collocation change * try re-ordering it * add in the new data column to the tests * remove a extra , * hmm... ->collate('utf8mb4_unicode_ci') is not usable as apparently collate does not exist * change the column type from json to longtext * mv chronyd stuff while I sort out the rest of the tests... damn thing is always buggy * hmm... fix a missing line then likely move stuff back * style fix * add fillable * add the expexcted data for fail2ban json * escape a " I missed * add data for portactivity * add suricata app data * add app data to zfs legacy test * put the moved tests back into place and update zfs-v1 test * add app data for chronyd test * add app data for fail2ban legacy test * update zfs v1 app data * add some notes on application dev work * add Developing/Application-Notes.md to mkdocs.yml * add data column to it * added various suggestions from bennet-esyoil * convert from isset to sizeof * type fix * fully remove the old save app data function and move it into a helper function... the other still needs cleaned up prior to removal * update docs * get_app_data is fully removed now as well * a few style fixes * add $casts * update chronyd test * attempt to fix the data * more doc cleanup and try changing the cast * style fix * revert the changes to the chronyd test * apply a few of murrant's suggestions * document working with ->data as json and non-josn * remove two no-longer used in this PR exceptions * ->data now operates transparently * style fix * update data tests * fix json * test fix * update the app notes to reflect how app data now works * app test fix * app data fix for linux_lsi * json fix * minor doc cleanup * remove duplicate querty and use json_decode instead * style fix * modelize the app poller * use a anon func instead of foreach * test update * style cleanup * style cleanup * another test cleanup * more test cleanup * reverse the test changes and add in some more glue code * revert one of the test changes * another small test fix * Make things use models Left some array access, but those will still work just fine. * missed chronyd and portactivity * rename poll to avoid make it any confusion * Remove extra save and fix timestamp * save any changes made to app->data * nope, that was not it * What are magic methods and how do they work? * fix two typos * update linux_lsi test * change quote type Co-authored-by: Tony Murray <murraytony@gmail.com>
51 lines
1.3 KiB
PHP
51 lines
1.3 KiB
PHP
<?php
|
|
/**
|
|
* Applications.php
|
|
*
|
|
* -Description-
|
|
*
|
|
* This program is free software: you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation, either version 3 of the License, or
|
|
* (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE.See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program. If not, see <https://www.gnu.org/licenses/>.
|
|
*
|
|
* @link https://www.librenms.org
|
|
*
|
|
* @copyright 2018 Tony Murray
|
|
* @author Tony Murray <murraytony@gmail.com>
|
|
*/
|
|
|
|
namespace App\Models;
|
|
|
|
use LibreNMS\Util\StringHelpers;
|
|
|
|
class Application extends DeviceRelatedModel
|
|
{
|
|
public $timestamps = false;
|
|
protected $primaryKey = 'app_id';
|
|
protected $fillable = ['data'];
|
|
protected $casts = [
|
|
'data' => 'array',
|
|
];
|
|
|
|
// ---- Helper Functions ----
|
|
|
|
public function displayName()
|
|
{
|
|
return StringHelpers::niceCase($this->app_type);
|
|
}
|
|
|
|
public function getShowNameAttribute()
|
|
{
|
|
return $this->displayName();
|
|
}
|
|
}
|