mirror of
https://github.com/librenms/librenms.git
synced 2024-09-22 10:58:42 +00:00
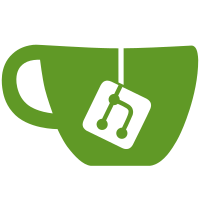
While adding configurable port mapping in 2c9df26bbf
I broke the query for known ports from the database so discovery.php considered
ALL ports to be new every time it ran. Whoops.
This went undetected for so long as there is a unique constraint on the ports
table (device_id+ifIndex) which prevented ports from being added again and
again.
Signed-off-by: Maximilian Wilhelm <max@rfc2324.org>
107 lines
3.9 KiB
PHP
107 lines
3.9 KiB
PHP
<?php
|
|
|
|
// Build SNMP Cache Array
|
|
$port_stats = array();
|
|
$port_stats = snmpwalk_cache_oid($device, 'ifDescr', $port_stats, 'IF-MIB');
|
|
$port_stats = snmpwalk_cache_oid($device, 'ifName', $port_stats, 'IF-MIB');
|
|
$port_stats = snmpwalk_cache_oid($device, 'ifType', $port_stats, 'IF-MIB');
|
|
|
|
// End Building SNMP Cache Array
|
|
d_echo($port_stats);
|
|
|
|
|
|
// By default libreNMS uses the ifIndex to associate ports on devices with ports discoverd/polled
|
|
// before and stored in the database. On Linux boxes this is a problem as ifIndexes may be
|
|
// unstable between reboots or (re)configuration of tunnel interfaces (think: GRE/OpenVPN/Tinc/...)
|
|
// The port association configuration allows to choose between association via ifIndex, ifName,
|
|
// or maybe other means in the future. The default port association mode still is ifIndex for
|
|
// compatibility reasons.
|
|
$port_association_mode = $config['default_port_association_mode'];
|
|
if ($device['port_association_mode'])
|
|
$port_association_mode = get_port_assoc_mode_name ($device['port_association_mode']);
|
|
|
|
// Build array of ports in the database and an ifIndex/ifName -> port_id map
|
|
$ports_mapped = get_ports_mapped ($device['device_id']);
|
|
$ports_db = $ports_mapped['ports'];
|
|
|
|
//
|
|
// Rename any old RRD files still named after the previous ifIndex based naming schema.
|
|
foreach ($ports_mapped['maps']['ifIndex'] as $ifIndex => $port_id) {
|
|
foreach (array ('', 'adsl', 'dot3') as $suffix) {
|
|
$suffix_tmp = '';
|
|
if ($suffix)
|
|
$suffix_tmp = "-$suffix";
|
|
|
|
$old_rrd_path = trim ($config['rrd_dir']) . '/' . $device['hostname'] . "/port-$ifIndex$suffix_tmp.rrd";
|
|
$new_rrd_path = get_port_rrdfile_path ($device['hostname'], $port_id, $suffix);
|
|
|
|
if (is_file ($old_rrd_path)) {
|
|
rename ($old_rrd_path, $new_rrd_path);
|
|
}
|
|
}
|
|
}
|
|
|
|
|
|
// New interface detection
|
|
foreach ($port_stats as $ifIndex => $port) {
|
|
// Store ifIndex in port entry and prefetch ifName as we'll need it multiple times
|
|
$port['ifIndex'] = $ifIndex;
|
|
$ifName = $port['ifName'];
|
|
|
|
// Get port_id according to port_association_mode used for this device
|
|
$port_id = get_port_id ($ports_mapped, $port, $port_association_mode);
|
|
|
|
if (is_port_valid($port, $device)) {
|
|
|
|
// Port newly discovered?
|
|
if (! is_array($ports_db[$port_id])) {
|
|
$port_id = dbInsert(array('device_id' => $device['device_id'], 'ifIndex' => $ifIndex, 'ifName' => $ifName), 'ports');
|
|
$ports[$port_id] = dbFetchRow('SELECT * FROM `ports` WHERE `device_id` = ? AND `port_id` = ?', array($device['device_id'], $port_id));
|
|
echo 'Adding: '.$ifName.'('.$ifIndex.')('.$port_id.')';
|
|
}
|
|
|
|
// Port re-discovered after previous deletion?
|
|
else if ($ports_db[$port_id]['deleted'] == '1') {
|
|
dbUpdate(array('deleted' => '0'), 'ports', '`port_id` = ?', array($ports_db[$port_id]));
|
|
$ports_db[$port_id]['deleted'] = '0';
|
|
echo 'U';
|
|
}
|
|
else {
|
|
echo '.';
|
|
}
|
|
|
|
// We've seen it. Remove it from the cache.
|
|
unset($ports_l[$ifIndex]);
|
|
}
|
|
|
|
// Port vanished (mark as deleted)
|
|
else {
|
|
if (is_array($ports_db[$port_id])) {
|
|
if ($ports_db[$port_id]['deleted'] != '1') {
|
|
dbUpdate(array('deleted' => '1'), 'ports', '`port_id` = ?', array($ports_db[$port_id]));
|
|
$ports_db[$port_id]['deleted'] = '1';
|
|
echo '-';
|
|
}
|
|
}
|
|
|
|
echo 'X';
|
|
}//end if
|
|
}//end foreach
|
|
|
|
// End New interface detection
|
|
// Interface Deletion
|
|
// If it's in our $ports_l list, that means it's not been seen. Mark it deleted.
|
|
foreach ($ports_l as $ifIndex => $port_id) {
|
|
if ($ports_db[$ifIndex]['deleted'] == '0') {
|
|
dbUpdate(array('deleted' => '1'), 'ports', '`port_id` = ?', array($port_id));
|
|
echo '-'.$ifIndex;
|
|
}
|
|
}
|
|
|
|
// End interface deletion
|
|
echo "\n";
|
|
|
|
// Clear Variables Here
|
|
unset($port_stats);
|
|
unset($ports_db);
|