mirror of
https://github.com/librenms/librenms.git
synced 2024-09-21 18:38:25 +00:00
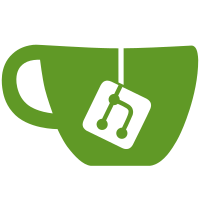
* Add Laravel to LibreNMS. * Try to set permissions during initial install and first composer update to Laravel. * Fix composer.lock Fix missing db config keys * Start building v1 layout Port ajax_setresolution, inject csrf into jquery ajax calls Layout works, building menu Partially done. * Fix device group list remove stupid count relationships * Print messages for common boot errors. Don't log to laravel.log file. Log to error_log until booted, then librenms.log * Fix up some issues with Config loading Start of custom directives * Custom blade directives: config, notconfig, admin * Preflight checks Only load config files once. * Update the composer.lock for php 5.6 * Menu through routing * Start of alert menu * Better alert scopes * reduce cruft in models * Alerting menu more or less working :D * Fix style * Improved preflight * Fix chicken-eggs! * Remove examples * Better alert_rule status queries Debugbar * fix app.env check * User Menu * Settings bar (dropped refresh) Search JS * Toastr messages * Rename preflight * Use hasAccess(User) on most models. Add port counts * Missed a Preflight -> Checks rename * Fix some formatting * Boot Eloquent outside of Laravel Use Eloquent for Config and Plugins so we don't have to connect with dbFacile inside Laravel. Move locate_binary() into Config class * Config WIP * Try to fix a lot of config loading issues. * Improve menu for non-admins removing unneeded menus url() for all in menu * Only use eloquent if it exists * Include APP_URL in initial .env settings * Implement Legacy User Provider * Helper class for using Eloquent outside of Laravel. Allows access to DB style queries too and checking the connection status. * Fix up tests * Fix device groups query * Checking Travis * copy config.test.php earlier * dbFacile check config before connecting Don't use exception to check if eloquent is connected, it gets grabbed by the exception handler. Ignore missing config.php error. * Fix config load with database is not migrated yet. * Remove Config::load() from early boot. * Use laravel config settings to init db (this prefers .env settings) Fix bgp vars not set in menu add _ide_helper.php to .gitignore * Restrict dependencies to versions that support php 5.6 * Update ConfigTest * Fix a couple of installation issues * Add unique NODE_ID to .env * Correct handling of title image * Fix database config not loading. Thanks @laf * Don't prepend / * add class_exists checks for development service providers * Fix config value casting * Don't use functions that may not exist * Update dbFacile.php * d_echo may not be defined when Config used called. * Add SELinux configuration steps More detailed permissions check. Check all and give complete corrective commands in one step. * Ignore node_modules directory * Re-add accidetal removal
91 lines
2.3 KiB
PHP
91 lines
2.3 KiB
PHP
<?php
|
|
/**
|
|
* app/Models/AlertRule.php
|
|
*
|
|
* Model for access to alert_rules table data
|
|
*
|
|
* This program is free software: you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation, either version 3 of the License, or
|
|
* (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE.See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program. If not, see <http://www.gnu.org/licenses/>.
|
|
*
|
|
* @package LibreNMS
|
|
* @link http://librenms.org
|
|
* @copyright 2016 Neil Lathwood
|
|
* @author Neil Lathwood <neil@lathwood.co.uk>
|
|
*/
|
|
|
|
namespace App\Models;
|
|
|
|
use Illuminate\Database\Eloquent\Builder;
|
|
|
|
class AlertRule extends BaseModel
|
|
{
|
|
public $timestamps = false;
|
|
|
|
// ---- Query scopes ----
|
|
|
|
/**
|
|
* @param Builder $query
|
|
* @return Builder
|
|
*/
|
|
public function scopeEnabled($query)
|
|
{
|
|
return $query->where('disabled', 0);
|
|
}
|
|
|
|
/**
|
|
* Scope for only alert rules that are currently in alarm
|
|
*
|
|
* @param Builder $query
|
|
* @return Builder
|
|
*/
|
|
public function scopeIsActive($query)
|
|
{
|
|
return $query->enabled()
|
|
->join('alerts', 'alerts.rule_id', 'alert_rules.id')
|
|
->where('alerts.state', 1);
|
|
}
|
|
|
|
/**
|
|
* Scope to filter rules for devices permitted to user
|
|
* (do not use for admin and global read-only users)
|
|
*
|
|
* @param $query
|
|
* @param User $user
|
|
* @return mixed
|
|
*/
|
|
public function scopeHasAccess($query, User $user)
|
|
{
|
|
if ($user->hasGlobalRead()) {
|
|
return $query;
|
|
}
|
|
|
|
if (!$this->isJoined($query, 'alerts')) {
|
|
$query->join('alerts', 'alerts.rule_id', 'alert_rules.id');
|
|
}
|
|
|
|
return $this->hasDeviceAccess($query, $user, 'alerts');
|
|
}
|
|
|
|
// ---- Define Relationships ----
|
|
|
|
public function alerts()
|
|
{
|
|
return $this->hasMany('App\Models\Alert', 'rule_id');
|
|
}
|
|
|
|
public function devices()
|
|
{
|
|
return $this->belongsToMany('App\Models\Device', 'alert_device_map', 'device_id', 'device_id', 'devices');
|
|
}
|
|
}
|