mirror of
https://github.com/librenms/librenms.git
synced 2024-09-21 18:38:25 +00:00
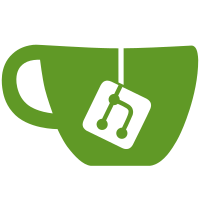
* Processor Tests! * Capture data from live devices easily. * fix up some stuff, remove powerconnect things as they seem to be just broken. * formatting, fix missing assignment add netonix processor data * fix multi-line, always add sysDescr and sysObjectID ios cpm test file * revert composer change and fix whitespace issues * add help text * missed help text * tighter debug output * handle empty strings properly and mibs with numbers * use keys for sorting as intended * fix type with empty data * oops :) * whitespace fix * try installing fping * Fix TestCase collision + cleanup * mark TestCase as abstract don't run two instances of snmpsim * better database dumps, improved capture * style fixes * fix quotes add a few more tables * add --prefer-new, properly merge data * Support separate discovery and poller data. But don't waste space if they aren't needed. * refactor to use class collects all code in one place for reusability * reorganize * Print out when not saving. * Support for running multiple (or all) modules at once. * tidy * Change unit test to a generic name and test all modules we have data for. * Add documentation and a few more tidies * whitespace fixes * Fix tests, add a couple more modules, more docs * More docs updates * Fix scrutinizer issues * add bgp-peers
100 lines
3.5 KiB
PHP
100 lines
3.5 KiB
PHP
<?php
|
|
/**
|
|
* RrdtoolTest.php
|
|
*
|
|
* Tests functionality of our rrdtool wrapper
|
|
*
|
|
* This program is free software: you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation, either version 3 of the License, or
|
|
* (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE.See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program. If not, see <http://www.gnu.org/licenses/>.
|
|
*
|
|
* @package LibreNMS
|
|
* @link http://librenms.org
|
|
* @copyright 2016 Tony Murray
|
|
* @author Tony Murray <murraytony@gmail.com>
|
|
*/
|
|
|
|
namespace LibreNMS\Tests;
|
|
|
|
class RrdtoolTest extends TestCase
|
|
{
|
|
|
|
public function testBuildCommandLocal()
|
|
{
|
|
global $config;
|
|
$config['rrdcached'] = '';
|
|
$config['rrdtool_version'] = '1.4';
|
|
$config['rrd_dir'] = '/opt/librenms/rrd';
|
|
|
|
$cmd = rrdtool_build_command('create', '/opt/librenms/rrd/f', 'o');
|
|
$this->assertEquals('create /opt/librenms/rrd/f o', $cmd);
|
|
|
|
$cmd = rrdtool_build_command('tune', '/opt/librenms/rrd/f', 'o');
|
|
$this->assertEquals('tune /opt/librenms/rrd/f o', $cmd);
|
|
|
|
$cmd = rrdtool_build_command('update', '/opt/librenms/rrd/f', 'o');
|
|
$this->assertEquals('update /opt/librenms/rrd/f o', $cmd);
|
|
|
|
|
|
$config['rrdtool_version'] = '1.6';
|
|
|
|
$cmd = rrdtool_build_command('create', '/opt/librenms/rrd/f', 'o');
|
|
$this->assertEquals('create /opt/librenms/rrd/f o -O', $cmd);
|
|
|
|
$cmd = rrdtool_build_command('tune', '/opt/librenms/rrd/f', 'o');
|
|
$this->assertEquals('tune /opt/librenms/rrd/f o', $cmd);
|
|
|
|
$cmd = rrdtool_build_command('update', '/opt/librenms/rrd/f', 'o');
|
|
$this->assertEquals('update /opt/librenms/rrd/f o', $cmd);
|
|
}
|
|
|
|
public function testBuildCommandRemote()
|
|
{
|
|
global $config;
|
|
$config['rrdcached'] = 'server:42217';
|
|
$config['rrdtool_version'] = '1.4';
|
|
$config['rrd_dir'] = '/opt/librenms/rrd';
|
|
|
|
$cmd = rrdtool_build_command('create', '/opt/librenms/rrd/f', 'o');
|
|
$this->assertEquals('create /opt/librenms/rrd/f o', $cmd);
|
|
|
|
$cmd = rrdtool_build_command('tune', '/opt/librenms/rrd/f', 'o');
|
|
$this->assertEquals('tune /opt/librenms/rrd/f o', $cmd);
|
|
|
|
$cmd = rrdtool_build_command('update', '/opt/librenms/rrd/f', 'o');
|
|
$this->assertEquals('update f o --daemon server:42217', $cmd);
|
|
|
|
|
|
$config['rrdtool_version'] = '1.6';
|
|
|
|
$cmd = rrdtool_build_command('create', '/opt/librenms/rrd/f', 'o');
|
|
$this->assertEquals('create f o -O --daemon server:42217', $cmd);
|
|
|
|
$cmd = rrdtool_build_command('tune', '/opt/librenms/rrd/f', 'o');
|
|
$this->assertEquals('tune f o --daemon server:42217', $cmd);
|
|
|
|
$cmd = rrdtool_build_command('update', '/opt/librenms/rrd/f', 'o');
|
|
$this->assertEquals('update f o --daemon server:42217', $cmd);
|
|
}
|
|
|
|
public function testBuildCommandException()
|
|
{
|
|
global $config;
|
|
$config['rrdcached'] = '';
|
|
$config['rrdtool_version'] = '1.4';
|
|
|
|
$this->setExpectedException('LibreNMS\Exceptions\FileExistsException');
|
|
// use this file, since it is guaranteed to exist
|
|
rrdtool_build_command('create', __FILE__, 'o');
|
|
}
|
|
}
|