mirror of
https://github.com/librenms/librenms.git
synced 2024-09-21 18:38:25 +00:00
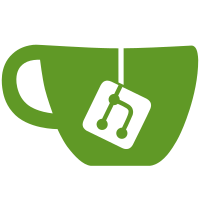
* Update manifest and add service worker cleanup icons a bit * Push notifications WIP * navigate working * cleanup * acknowledge wired up * Set VAPID keys on composer install * Component to control notification permissions. * Allow all user option to validate * Enable on browser load if transport exists. * Check for transport before showing user permissions translations * Documentation * style fixes * access via the attribute model * fix alerting test * update schema * cleanup subscription on disable * non-configurable db and table for webpush subscriptions (respect system connection) * revert AlertTransport change hopefully phpstan can figure it out * phpstan fixes * Support custom details display * Match transport names to brand's preferred display * less duplicate id errors * Tests are done in Laravel code now so remove legacy function usage... could be better, but ok * Style fixes * Style fixes 2 * Fix alert test * Doc updates requires HTTPS and GMP * unregister subscription when permission is set to denied * cleanup after user deletion * delete the right thing * fix whitespace * update install docs to include php-gmp * suggest ext-gmp * update javascript * Update functions.php Co-authored-by: Jellyfrog <Jellyfrog@users.noreply.github.com>
82 lines
2.1 KiB
PHP
82 lines
2.1 KiB
PHP
<?php
|
|
/**
|
|
* app/Models/Alert.php
|
|
*
|
|
* Model for access to alerts table data
|
|
*
|
|
* This program is free software: you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation, either version 3 of the License, or
|
|
* (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE.See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program. If not, see <https://www.gnu.org/licenses/>.
|
|
*
|
|
* @link https://www.librenms.org
|
|
*
|
|
* @copyright 2016 Neil Lathwood
|
|
* @author Neil Lathwood <neil@lathwood.co.uk>
|
|
*/
|
|
|
|
namespace App\Models;
|
|
|
|
use Illuminate\Database\Eloquent\Builder;
|
|
use Illuminate\Database\Eloquent\Model;
|
|
use Illuminate\Database\Eloquent\Relations\BelongsTo;
|
|
use Illuminate\Database\Eloquent\Relations\BelongsToMany;
|
|
use LibreNMS\Enum\AlertState;
|
|
|
|
class Alert extends Model
|
|
{
|
|
public $timestamps = false;
|
|
public $casts = [
|
|
'info' => 'array',
|
|
];
|
|
|
|
// ---- Query scopes ----
|
|
|
|
/**
|
|
* Only select active alerts
|
|
*
|
|
* @param Builder $query
|
|
* @return Builder
|
|
*/
|
|
public function scopeActive($query)
|
|
{
|
|
return $query->where('state', '=', AlertState::ACTIVE);
|
|
}
|
|
|
|
/**
|
|
* Only select active alerts
|
|
*
|
|
* @param Builder $query
|
|
* @return Builder
|
|
*/
|
|
public function scopeAcknowledged($query)
|
|
{
|
|
return $query->where('state', '=', AlertState::ACKNOWLEDGED);
|
|
}
|
|
|
|
// ---- Define Relationships ----
|
|
|
|
public function device(): BelongsTo
|
|
{
|
|
return $this->belongsTo(\App\Models\Device::class, 'device_id');
|
|
}
|
|
|
|
public function rule(): BelongsTo
|
|
{
|
|
return $this->belongsTo(\App\Models\AlertRule::class, 'rule_id', 'id');
|
|
}
|
|
|
|
public function users(): BelongsToMany
|
|
{
|
|
return $this->belongsToMany(\App\Models\User::class, 'devices_perms', 'device_id', 'user_id');
|
|
}
|
|
}
|