mirror of
https://github.com/librenms/librenms.git
synced 2024-09-21 10:28:13 +00:00
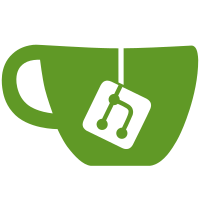
* PHP-Flasher for toast messages Allows customized template removes dependency on unmaintained package using dev stability no solution for javascript toasts yet Use DI in places it makes sense allow html in flashes Use "template.librenms" as a default notification style merge toast containers toastr needs to be second because it will find the containr made by flasher, but the inverse is not true upgrade php-flasher to add custom options and persistent notifications Add dark theme * update composer.lock
130 lines
4.4 KiB
PHP
130 lines
4.4 KiB
PHP
<?php
|
|
/**
|
|
* Checks.php
|
|
*
|
|
* Pre-flight checks at various stages of booting
|
|
*
|
|
* This program is free software: you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation, either version 3 of the License, or
|
|
* (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE.See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program. If not, see <https://www.gnu.org/licenses/>.
|
|
*
|
|
* @link https://www.librenms.org
|
|
*
|
|
* @copyright 2018 Tony Murray
|
|
* @author Tony Murray <murraytony@gmail.com>
|
|
*/
|
|
|
|
namespace App;
|
|
|
|
use App\Models\Device;
|
|
use App\Models\Notification;
|
|
use App\Models\User;
|
|
use Cache;
|
|
use Carbon\Carbon;
|
|
use Illuminate\Support\Facades\Auth;
|
|
use LibreNMS\Config;
|
|
|
|
class Checks
|
|
{
|
|
/**
|
|
* Post boot Toast messages
|
|
*/
|
|
public static function postAuth()
|
|
{
|
|
// limit popup messages frequency
|
|
if (Cache::get('checks_popup_timeout') || ! Auth::check()) {
|
|
return;
|
|
}
|
|
|
|
Cache::put('checks_popup_timeout', true, Config::get('checks_popup_timer', 5) * 60);
|
|
|
|
/** @var User $user */
|
|
$user = Auth::user();
|
|
|
|
if ($user->isAdmin()) {
|
|
$notifications = Notification::isUnread($user)->where('severity', '>', \LibreNMS\Enum\Alert::OK)->get();
|
|
foreach ($notifications as $notification) {
|
|
flash()
|
|
->using('template.librenms')
|
|
->title($notification->title)
|
|
->addWarning("<a href='notifications/'>$notification->body</a>");
|
|
}
|
|
|
|
$warn_sec = Config::get('rrd.step', 300) * 3;
|
|
if (Device::isUp()->where('last_polled', '<=', Carbon::now()->subSeconds($warn_sec))->exists()) {
|
|
$warn_min = $warn_sec / 60;
|
|
flash()
|
|
->using('template.librenms')
|
|
->title('Devices unpolled')
|
|
->addWarning('<a href="poller/log?filter=unpolled/">It appears as though you have some devices that haven\'t completed polling within the last ' . $warn_min . ' minutes, you may want to check that out :)</a>');
|
|
}
|
|
|
|
// Directory access checks
|
|
$rrd_dir = Config::get('rrd_dir');
|
|
if (! is_dir($rrd_dir)) {
|
|
flash()->addError("RRD Directory is missing ($rrd_dir). Graphing may fail. <a href=" . url('validate') . '>Validate your install</a>');
|
|
}
|
|
|
|
$temp_dir = Config::get('temp_dir');
|
|
if (! is_dir($temp_dir)) {
|
|
flash()->addError("Temp Directory is missing ($temp_dir). Graphing may fail. <a href=" . url('validate') . '>Validate your install</a>');
|
|
} elseif (! is_writable($temp_dir)) {
|
|
flash()->addError("Temp Directory is not writable ($temp_dir). Graphing may fail. <a href='" . url('validate') . "'>Validate your install</a>");
|
|
}
|
|
}
|
|
}
|
|
|
|
/**
|
|
* Check the script is running as the right user (works before config is available)
|
|
*/
|
|
public static function runningUser()
|
|
{
|
|
if (function_exists('posix_getpwuid') && posix_getpwuid(posix_geteuid())['name'] !== get_current_user()) {
|
|
if (get_current_user() == 'root') {
|
|
self::printMessage(
|
|
'Error: lnms file is owned by root, it should be owned and ran by a non-privileged user.',
|
|
null,
|
|
true
|
|
);
|
|
}
|
|
|
|
self::printMessage(
|
|
'Error: You must run lnms as the user ' . get_current_user(),
|
|
null,
|
|
true
|
|
);
|
|
}
|
|
}
|
|
|
|
private static function printMessage($title, $content, $exit = false)
|
|
{
|
|
$content = (array) $content;
|
|
|
|
if (PHP_SAPI == 'cli') {
|
|
$format = "%s\n\n%s\n\n";
|
|
$message = implode(PHP_EOL, $content);
|
|
} else {
|
|
$format = "<h3 style='color: firebrick;'>%s</h3><p>%s</p>";
|
|
$message = '';
|
|
foreach ($content as $line) {
|
|
$message .= "<p style='margin:0.5em'>$line</p>\n";
|
|
}
|
|
}
|
|
|
|
printf($format, $title, $message);
|
|
|
|
if ($exit) {
|
|
exit(1);
|
|
}
|
|
}
|
|
}
|