mirror of
https://github.com/librenms/librenms.git
synced 2024-09-21 10:28:13 +00:00
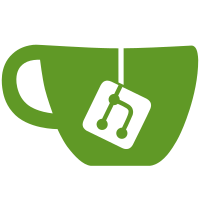
* Split out single device polling to a Laravel Job Probably totally broken :) * Add dispatch option to device:poll * Fix up submodules * Add missing logger parameter * Apply fixes from StyleCI * Update module format in test helper * Apply fixes from StyleCI * Use Log facade to match other code --------- Co-authored-by: Tony Murray <murrant@users.noreply.github.com>
88 lines
2.8 KiB
PHP
88 lines
2.8 KiB
PHP
<?php
|
|
/**
|
|
* Module.php
|
|
*
|
|
* -Description-
|
|
*
|
|
* This program is free software: you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation, either version 3 of the License, or
|
|
* (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE.See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program. If not, see <https://www.gnu.org/licenses/>.
|
|
*
|
|
* @link https://www.librenms.org
|
|
*
|
|
* @copyright 2022 Tony Murray
|
|
* @author Tony Murray <murraytony@gmail.com>
|
|
*/
|
|
|
|
namespace LibreNMS\Util;
|
|
|
|
use App\Models\Device;
|
|
use LibreNMS\Config;
|
|
use LibreNMS\Modules\LegacyModule;
|
|
use LibreNMS\Polling\ModuleStatus;
|
|
|
|
class Module
|
|
{
|
|
public static function exists(string $module_name): bool
|
|
{
|
|
if (class_exists(StringHelpers::toClass($module_name, '\\LibreNMS\\Modules\\'))) {
|
|
return true;
|
|
}
|
|
|
|
return Config::has('discovery_modules.' . $module_name) || Config::has('poller_modules.' . $module_name);
|
|
}
|
|
|
|
public static function fromName(string $module_name): \LibreNMS\Interfaces\Module
|
|
{
|
|
$module_class = StringHelpers::toClass($module_name, '\\LibreNMS\\Modules\\');
|
|
|
|
return class_exists($module_class) ? new $module_class : new LegacyModule($module_name);
|
|
}
|
|
|
|
public static function legacyDiscoveryExists(string $module_name): bool
|
|
{
|
|
return is_file(base_path("includes/discovery/$module_name.inc.php"));
|
|
}
|
|
|
|
public static function legacyPollingExists(string $module_name): bool
|
|
{
|
|
return is_file(base_path("includes/polling/$module_name.inc.php"));
|
|
}
|
|
|
|
public static function pollingStatus(string $module_name, Device $device, ?bool $manual = null): ModuleStatus
|
|
{
|
|
return new ModuleStatus(
|
|
Config::get("poller_modules.$module_name", false),
|
|
Config::get("os.{$device->os}.poller_modules.$module_name"),
|
|
$device->getAttrib("poll_$module_name"),
|
|
$manual,
|
|
);
|
|
}
|
|
|
|
public static function parseUserOverrides(array $overrides): array
|
|
{
|
|
$modules = [];
|
|
|
|
foreach ($overrides as $index => $module) {
|
|
// parse submodules (only supported by some modules)
|
|
if (str_contains($module, '/')) {
|
|
[$module, $submodule] = explode('/', $module, 2);
|
|
$modules[$module][] = $submodule;
|
|
} elseif (self::exists($module)) {
|
|
$modules[$module] = true;
|
|
}
|
|
}
|
|
|
|
return $modules;
|
|
}
|
|
}
|