mirror of
https://github.com/librenms/librenms.git
synced 2024-09-21 10:28:13 +00:00
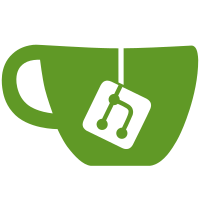
* Apply code style * Remove explicit call to register policies * Shift core files * Shift config files * Default config files In an effort to make upgrading the constantly changing config files easier, Shift defaulted them and merged your true customizations - where ENV variables may not be used. * Bump Laravel dependencies * Add type hints for Laravel 10 * Shift cleanup * wip * wip * sync translation * Sync back config * Public Path Binding * QueryException * monolog * db::raw * monolog * db::raw * fix larastan collections * fix phpstan bug looping forever * larastan errors * larastan: fix column type * styleci * initialize array * fixes * fixes --------- Co-authored-by: Shift <shift@laravelshift.com>
95 lines
2.8 KiB
PHP
95 lines
2.8 KiB
PHP
<?php
|
|
|
|
namespace LibreNMS\OS;
|
|
|
|
use LibreNMS\Device\WirelessSensor;
|
|
use LibreNMS\Interfaces\Discovery\Sensors\WirelessClientsDiscovery;
|
|
use LibreNMS\Interfaces\Polling\Sensors\WirelessClientsPolling;
|
|
use LibreNMS\OS;
|
|
|
|
class Stellar extends OS implements
|
|
WirelessClientsDiscovery,
|
|
WirelessClientsPolling
|
|
{
|
|
public function discoverWirelessClients()
|
|
{
|
|
$sensors = [];
|
|
$device = $this->getDeviceArray();
|
|
|
|
$ssid = [];
|
|
$ssid_data = $this->getCacheTable('apWlanEssid', $device['hardware']);
|
|
|
|
foreach ($ssid_data as $ssid_entry) {
|
|
if ($ssid_entry['apWlanEssid'] == 'athmon2') {
|
|
continue;
|
|
} elseif (array_key_exists($ssid_entry['apWlanEssid'], $ssid)) {
|
|
continue;
|
|
} else {
|
|
$ssid[$ssid_entry['apWlanEssid']] = 0;
|
|
}
|
|
}
|
|
|
|
$client_ws_data = $this->getCacheTable('apClientWlanService', $device['hardware']);
|
|
|
|
if (empty($client_ws_data)) {
|
|
$total_clients = 0;
|
|
} else {
|
|
$total_clients = count($client_ws_data);
|
|
}
|
|
|
|
$combined_oid = sprintf('%s::%s', $device['hardware'], 'apClientWlanService');
|
|
$oid = snmp_translate($combined_oid, 'ALL', 'nokia/stellar', '-On');
|
|
|
|
if (empty($oid)) {
|
|
return $sensors;
|
|
}
|
|
|
|
foreach ($client_ws_data as $client_entry) {
|
|
$ssid[$client_entry['apClientWlanService']] += 1;
|
|
}
|
|
|
|
foreach ($ssid as $key => $value) {
|
|
$sensors[] = new WirelessSensor('clients', $this->getDeviceId(), $oid, 'stellar', $key, 'SSID ' . $key . ' Clients', $value);
|
|
}
|
|
|
|
$sensors[] = new WirelessSensor('clients', $this->getDeviceId(), $oid, 'stellar', 'total-clients', 'Total Clients', $total_clients);
|
|
|
|
return $sensors;
|
|
}
|
|
|
|
/**
|
|
* Poll wireless clients
|
|
* The returned array should be sensor_id => value pairs
|
|
*
|
|
* @param array $sensors Array of sensors needed to be polled
|
|
* @return array of polled data
|
|
*/
|
|
public function pollWirelessClients(array $sensors)
|
|
{
|
|
$data = [];
|
|
if (! empty($sensors)) {
|
|
$device = $this->getDeviceArray();
|
|
|
|
$client_ws_data = $this->getCacheTable('apClientWlanService', $device['hardware']);
|
|
|
|
if (empty($client_ws_data)) {
|
|
$total_clients = 0;
|
|
} else {
|
|
$total_clients = count($client_ws_data);
|
|
}
|
|
|
|
foreach ($sensors as $sensor) {
|
|
foreach ($client_ws_data as $cliententry) {
|
|
if ($cliententry['apClientWlanService'] == $sensor['sensor_index']) {
|
|
$data[$sensor['sensor_id']] += 1;
|
|
}
|
|
}
|
|
}
|
|
|
|
$data[$sensors[0]['sensor_id']] = $total_clients;
|
|
}
|
|
|
|
return $data;
|
|
}
|
|
}
|