mirror of
https://github.com/immich-app/immich.git
synced 2024-09-22 02:57:26 +00:00
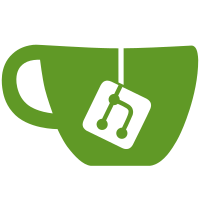
* add library validation api * chore: open api * show warning i UI * add flex row * fix e2e * tests * fix tests * enforce path validation * enforce validation on refresh * return 400 on bad import path * add limits to import paths * set response code to 200 * fix e2e * fix lint * fix test * restore e2e folder * fix import * use startsWith * icon color * notify user of failed validation * add parent div to validation * add docs to the import validation * improve library troubleshooting docs * fix button alignment --------- Co-authored-by: Alex Tran <alex.tran1502@gmail.com>
182 lines
4.6 KiB
TypeScript
182 lines
4.6 KiB
TypeScript
import { UserAvatarColor, UserEntity } from '@app/infra/entities';
|
|
import { authStub } from './auth.stub';
|
|
|
|
export const userDto = {
|
|
user1: {
|
|
email: 'user1@immich.app',
|
|
password: 'Password123',
|
|
name: 'User 1',
|
|
},
|
|
user2: {
|
|
email: 'user2@immich.app',
|
|
password: 'Password123',
|
|
name: 'User 2',
|
|
},
|
|
user3: {
|
|
email: 'user3@immich.app',
|
|
password: 'Password123',
|
|
name: 'User 3',
|
|
},
|
|
userWithQuota: {
|
|
email: 'quota-user@immich.app',
|
|
password: 'Password123',
|
|
name: 'User with quota',
|
|
quotaSizeInBytes: 42,
|
|
},
|
|
};
|
|
|
|
export const userStub = {
|
|
admin: Object.freeze<UserEntity>({
|
|
...authStub.admin.user,
|
|
password: 'admin_password',
|
|
name: 'admin_name',
|
|
storageLabel: 'admin',
|
|
externalPath: null,
|
|
oauthId: '',
|
|
shouldChangePassword: false,
|
|
profileImagePath: '',
|
|
createdAt: new Date('2021-01-01'),
|
|
deletedAt: null,
|
|
updatedAt: new Date('2021-01-01'),
|
|
tags: [],
|
|
assets: [],
|
|
memoriesEnabled: true,
|
|
avatarColor: UserAvatarColor.PRIMARY,
|
|
quotaSizeInBytes: null,
|
|
quotaUsageInBytes: 0,
|
|
}),
|
|
user1: Object.freeze<UserEntity>({
|
|
...authStub.user1.user,
|
|
password: 'immich_password',
|
|
name: 'immich_name',
|
|
storageLabel: null,
|
|
externalPath: null,
|
|
oauthId: '',
|
|
shouldChangePassword: false,
|
|
profileImagePath: '',
|
|
createdAt: new Date('2021-01-01'),
|
|
deletedAt: null,
|
|
updatedAt: new Date('2021-01-01'),
|
|
tags: [],
|
|
assets: [],
|
|
memoriesEnabled: true,
|
|
avatarColor: UserAvatarColor.PRIMARY,
|
|
quotaSizeInBytes: null,
|
|
quotaUsageInBytes: 0,
|
|
}),
|
|
user2: Object.freeze<UserEntity>({
|
|
...authStub.user2.user,
|
|
password: 'immich_password',
|
|
name: 'immich_name',
|
|
storageLabel: null,
|
|
externalPath: null,
|
|
oauthId: '',
|
|
shouldChangePassword: false,
|
|
profileImagePath: '',
|
|
createdAt: new Date('2021-01-01'),
|
|
deletedAt: null,
|
|
updatedAt: new Date('2021-01-01'),
|
|
tags: [],
|
|
assets: [],
|
|
memoriesEnabled: true,
|
|
avatarColor: UserAvatarColor.PRIMARY,
|
|
quotaSizeInBytes: null,
|
|
quotaUsageInBytes: 0,
|
|
}),
|
|
storageLabel: Object.freeze<UserEntity>({
|
|
...authStub.user1.user,
|
|
password: 'immich_password',
|
|
name: 'immich_name',
|
|
storageLabel: 'label-1',
|
|
externalPath: null,
|
|
oauthId: '',
|
|
shouldChangePassword: false,
|
|
profileImagePath: '',
|
|
createdAt: new Date('2021-01-01'),
|
|
deletedAt: null,
|
|
updatedAt: new Date('2021-01-01'),
|
|
tags: [],
|
|
assets: [],
|
|
memoriesEnabled: true,
|
|
avatarColor: UserAvatarColor.PRIMARY,
|
|
quotaSizeInBytes: null,
|
|
quotaUsageInBytes: 0,
|
|
}),
|
|
externalPath1: Object.freeze<UserEntity>({
|
|
...authStub.user1.user,
|
|
password: 'immich_password',
|
|
name: 'immich_name',
|
|
storageLabel: 'label-1',
|
|
externalPath: '/data/user1',
|
|
oauthId: '',
|
|
shouldChangePassword: false,
|
|
profileImagePath: '',
|
|
createdAt: new Date('2021-01-01'),
|
|
deletedAt: null,
|
|
updatedAt: new Date('2021-01-01'),
|
|
tags: [],
|
|
assets: [],
|
|
memoriesEnabled: true,
|
|
avatarColor: UserAvatarColor.PRIMARY,
|
|
quotaSizeInBytes: null,
|
|
quotaUsageInBytes: 0,
|
|
}),
|
|
externalPath2: Object.freeze<UserEntity>({
|
|
...authStub.user1.user,
|
|
password: 'immich_password',
|
|
name: 'immich_name',
|
|
storageLabel: 'label-1',
|
|
externalPath: '/data/user2',
|
|
oauthId: '',
|
|
shouldChangePassword: false,
|
|
profileImagePath: '',
|
|
createdAt: new Date('2021-01-01'),
|
|
deletedAt: null,
|
|
updatedAt: new Date('2021-01-01'),
|
|
tags: [],
|
|
assets: [],
|
|
memoriesEnabled: true,
|
|
avatarColor: UserAvatarColor.PRIMARY,
|
|
quotaSizeInBytes: null,
|
|
quotaUsageInBytes: 0,
|
|
}),
|
|
externalPathRoot: Object.freeze<UserEntity>({
|
|
...authStub.user1.user,
|
|
password: 'immich_password',
|
|
name: 'immich_name',
|
|
storageLabel: 'label-1',
|
|
externalPath: '/',
|
|
oauthId: '',
|
|
shouldChangePassword: false,
|
|
profileImagePath: '',
|
|
createdAt: new Date('2021-01-01'),
|
|
deletedAt: null,
|
|
updatedAt: new Date('2021-01-01'),
|
|
tags: [],
|
|
assets: [],
|
|
memoriesEnabled: true,
|
|
avatarColor: UserAvatarColor.PRIMARY,
|
|
quotaSizeInBytes: null,
|
|
quotaUsageInBytes: 0,
|
|
}),
|
|
profilePath: Object.freeze<UserEntity>({
|
|
...authStub.user1.user,
|
|
password: 'immich_password',
|
|
name: 'immich_name',
|
|
storageLabel: 'label-1',
|
|
externalPath: null,
|
|
oauthId: '',
|
|
shouldChangePassword: false,
|
|
profileImagePath: '/path/to/profile.jpg',
|
|
createdAt: new Date('2021-01-01'),
|
|
deletedAt: null,
|
|
updatedAt: new Date('2021-01-01'),
|
|
tags: [],
|
|
assets: [],
|
|
memoriesEnabled: true,
|
|
avatarColor: UserAvatarColor.PRIMARY,
|
|
quotaSizeInBytes: null,
|
|
quotaUsageInBytes: 0,
|
|
}),
|
|
};
|