mirror of
https://github.com/golang/go.git
synced 2024-09-22 19:08:30 +00:00
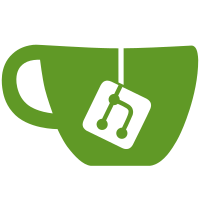
This commit adds a new cmd/compile flag -spectre, which accepts a comma-separated list of possible Spectre mitigations to apply, or the empty string (none), or "all". The only known mitigation right now is "index", which uses conditional moves to ensure that x86-64 CPUs do not speculate past index bounds checks. Speculating past index bounds checks may be problematic on systems running privileged servers that accept requests from untrusted users who can execute their own programs on the same machine. (And some more constraints that make it even more unlikely in practice.) The cases this protects against are analogous to the ones Microsoft explains in the "Array out of bounds load/store feeding ..." sections here: https://docs.microsoft.com/en-us/cpp/security/developer-guidance-speculative-execution?view=vs-2019#array-out-of-bounds-load-feeding-an-indirect-branch Change-Id: Ib7532d7e12466b17e04c4e2075c2a456dc98f610 Reviewed-on: https://go-review.googlesource.com/c/go/+/222660 Reviewed-by: Keith Randall <khr@golang.org>
39 lines
722 B
Go
39 lines
722 B
Go
// +build amd64
|
|
// asmcheck -gcflags=-spectre=index
|
|
|
|
// Copyright 2020 The Go Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
package codegen
|
|
|
|
func IndexArray(x *[10]int, i int) int {
|
|
// amd64:`CMOVQCC`
|
|
return x[i]
|
|
}
|
|
|
|
func IndexString(x string, i int) byte {
|
|
// amd64:`CMOVQCC`
|
|
return x[i]
|
|
}
|
|
|
|
func IndexSlice(x []float64, i int) float64 {
|
|
// amd64:`CMOVQCC`
|
|
return x[i]
|
|
}
|
|
|
|
func SliceArray(x *[10]int, i, j int) []int {
|
|
// amd64:`CMOVQHI`
|
|
return x[i:j]
|
|
}
|
|
|
|
func SliceString(x string, i, j int) string {
|
|
// amd64:`CMOVQHI`
|
|
return x[i:j]
|
|
}
|
|
|
|
func SliceSlice(x []float64, i, j int) []float64 {
|
|
// amd64:`CMOVQHI`
|
|
return x[i:j]
|
|
}
|