mirror of
https://github.com/golang/go.git
synced 2024-09-21 10:28:27 +00:00
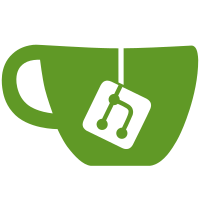
Use OTAILCALL in wrapper if the receiver and method are both pointers and it is not going to be inlined, similar to how it is done in reflectdata.methodWrapper. Currently tail call may be used for functions with identical argument types. This change updates wrappers where both wrapper and the wrapped method's receiver are pointers. In this case, we have the same signature for the wrapper and the wrapped method (modulo the receiver's pointed-to types), and do not need any local variables in the generated wrapper (on stack) because the arguments are immediately passed to the wrapped method in place (without need to move some value passed to other register or to change any argument/return passed through stack). Thus, the wrapper does not need its own stack frame. This applies to promoted methods, e.g. when we have some struct type U with an embedded type *T and construct a wrapper like func (recv *U) M(arg int) bool { return recv.T.M(i) } See also test/abi/method_wrapper.go for a running example. Code size difference measured with this change (tried for x86_64): etcd binary: .text section size: 21472251 -> 21432350 (0.2%) total binary size: 32226640 -> 32191136 (0.1%) compile binary: .text section size: 17419073 -> 17413929 (0.03%) total binary size: 26744743 -> 26737567 (0.03%) Change-Id: I9bbe730568f6def21a8e61118a6b6f503d98049c Reviewed-on: https://go-review.googlesource.com/c/go/+/578235 LUCI-TryBot-Result: Go LUCI <golang-scoped@luci-project-accounts.iam.gserviceaccount.com> Reviewed-by: Cherry Mui <cherryyz@google.com> Reviewed-by: Dmitri Shuralyov <dmitshur@google.com> Reviewed-by: Keith Randall <khr@golang.org> Reviewed-by: Keith Randall <khr@google.com>
30 lines
805 B
Go
30 lines
805 B
Go
// errorcheck -0 -d=tailcall=1
|
|
|
|
// Copyright 2024 The Go Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
package p
|
|
|
|
// Test that when generating wrappers for methods, we generate a tail call to the pointer version of
|
|
// the method, if that method is not inlineable. We use go:noinline here to force the non-inlineability
|
|
// condition.
|
|
|
|
//go:noinline
|
|
func (f *Foo) Get2Vals() [2]int { return [2]int{f.Val, f.Val + 1} }
|
|
func (f *Foo) Get3Vals() [3]int { return [3]int{f.Val, f.Val + 1, f.Val + 2} }
|
|
|
|
type Foo struct{ Val int }
|
|
|
|
type Bar struct { // ERROR "tail call emitted for the method \(\*Foo\).Get2Vals wrapper"
|
|
int64
|
|
*Foo // needs a method wrapper
|
|
string
|
|
}
|
|
|
|
var i any
|
|
|
|
func init() {
|
|
i = Bar{1, nil, "first"}
|
|
}
|