mirror of
https://github.com/twbs/bootstrap.git
synced 2024-09-22 19:17:16 +00:00
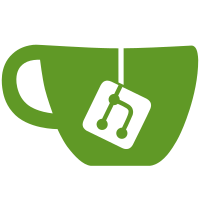
Changes in the Gruntfile: * Use two spaces for consistency with the rest of the codebase. * Tasks are now organize based on task type. since lodash templates are used across tasks/targets, this makes it easier to follow. * Use `src-dest` files format when only src-dest pairing is necessary. This saves a line over the files-object format, but more importantly it allows use the src or dest in lodash templates (e.g. `<%= concat.bootstrap.src %>`), which isn't possible in the files-object or files-array formats. * Make the explicit order of the javascript files more obvious in concat task , maybe just a tiny bit more usable and easier to customize - but really just OCD * Use `<%= pkg.name %>` variable for dest CSS, both for consistency with how javascript targets are defined, and to make it just little easier for devs to customize bootstrap. ran the build to test, everything seems good.
145 lines
3.2 KiB
JavaScript
145 lines
3.2 KiB
JavaScript
/* jshint node: true */
|
|
|
|
module.exports = function(grunt) {
|
|
"use strict";
|
|
|
|
// Project configuration.
|
|
grunt.initConfig({
|
|
|
|
// Metadata.
|
|
pkg: grunt.file.readJSON('package.json'),
|
|
banner: '/**\n' +
|
|
'* <%= pkg.name %>.js v<%= pkg.version %> by @fat and @mdo\n' +
|
|
'* Copyright <%= grunt.template.today("yyyy") %> <%= pkg.author %>\n' +
|
|
'* <%= _.pluck(pkg.licenses, "url").join(", ") %>\n' +
|
|
'*/\n',
|
|
jqueryCheck: 'if (!jQuery) { throw new Error(\"Bootstrap requires jQuery\") }\n\n',
|
|
|
|
// Task configuration.
|
|
clean: {
|
|
dist: ['dist']
|
|
},
|
|
|
|
jshint: {
|
|
options: {
|
|
jshintrc: 'js/.jshintrc'
|
|
},
|
|
gruntfile: {
|
|
src: 'Gruntfile.js'
|
|
},
|
|
src: {
|
|
src: ['js/*.js']
|
|
},
|
|
test: {
|
|
src: ['js/tests/unit/*.js']
|
|
}
|
|
},
|
|
concat: {
|
|
options: {
|
|
banner: '<%= banner %><%= jqueryCheck %>',
|
|
stripBanners: false
|
|
},
|
|
bootstrap: {
|
|
src: [
|
|
'js/transition.js',
|
|
'js/alert.js',
|
|
'js/button.js',
|
|
'js/carousel.js',
|
|
'js/collapse.js',
|
|
'js/dropdown.js',
|
|
'js/modal.js',
|
|
'js/tooltip.js',
|
|
'js/popover.js',
|
|
'js/scrollspy.js',
|
|
'js/tab.js',
|
|
'js/affix.js'
|
|
],
|
|
dest: 'dist/js/<%= pkg.name %>.js'
|
|
}
|
|
},
|
|
uglify: {
|
|
options: {
|
|
banner: '<%= banner %>'
|
|
},
|
|
bootstrap: {
|
|
src: ['<%= concat.bootstrap.dest %>'],
|
|
dest: 'dist/js/<%= pkg.name %>.min.js'
|
|
}
|
|
},
|
|
|
|
recess: {
|
|
options: {
|
|
compile: true
|
|
},
|
|
bootstrap: {
|
|
src: ['less/bootstrap.less'],
|
|
dest: 'dist/css/<%= pkg.name %>.css'
|
|
},
|
|
min: {
|
|
options: {
|
|
compress: true
|
|
},
|
|
src: ['less/bootstrap.less'],
|
|
dest: 'dist/css/<%= pkg.name %>.min.css'
|
|
}
|
|
},
|
|
|
|
qunit: {
|
|
options: {
|
|
inject: 'js/tests/unit/phantom.js'
|
|
},
|
|
files: ['js/tests/*.html']
|
|
},
|
|
connect: {
|
|
server: {
|
|
options: {
|
|
port: 3000,
|
|
base: '.'
|
|
}
|
|
}
|
|
},
|
|
|
|
watch: {
|
|
src: {
|
|
files: '<%= jshint.src.src %>',
|
|
tasks: ['jshint:src', 'qunit']
|
|
},
|
|
test: {
|
|
files: '<%= jshint.test.src %>',
|
|
tasks: ['jshint:test', 'qunit']
|
|
},
|
|
recess: {
|
|
files: 'less/*.less',
|
|
tasks: ['recess']
|
|
}
|
|
}
|
|
});
|
|
|
|
|
|
// These plugins provide necessary tasks.
|
|
grunt.loadNpmTasks('grunt-contrib-clean');
|
|
grunt.loadNpmTasks('grunt-contrib-concat');
|
|
grunt.loadNpmTasks('grunt-contrib-connect');
|
|
grunt.loadNpmTasks('grunt-contrib-jshint');
|
|
grunt.loadNpmTasks('grunt-contrib-qunit');
|
|
grunt.loadNpmTasks('grunt-contrib-uglify');
|
|
grunt.loadNpmTasks('grunt-contrib-watch');
|
|
grunt.loadNpmTasks('grunt-recess');
|
|
|
|
|
|
// Test task.
|
|
grunt.registerTask('test', ['jshint', 'qunit']);
|
|
|
|
// JS distribution task.
|
|
grunt.registerTask('dist-js', ['concat', 'uglify']);
|
|
|
|
// CSS distribution task.
|
|
grunt.registerTask('dist-css', ['recess']);
|
|
|
|
// Full distribution task.
|
|
grunt.registerTask('dist', ['clean', 'dist-css', 'dist-js']);
|
|
|
|
// Default task.
|
|
grunt.registerTask('default', ['test', 'dist']);
|
|
};
|