Added support for T-Display-S3-MIDI
418
example/T-Display-S3-MIDI/T-Display-S3-MIDI.cpp
Normal file
@ -0,0 +1,418 @@
|
||||
/*
|
||||
* @Description: This program is used to test the T-Display-S3-MIDI program and consists
|
||||
of 3 parts, 1.PCF8575_Test, 2.MPR121_Test, 3.PCM5102A_Test
|
||||
|
||||
When the program is freshly burned, you can see the "START" text in red. By touching
|
||||
any of the TOUCH0 (0-11) or TOUCH1 (0-10) pins of the MPR121 touch sensor, the system is
|
||||
activated. At this point, the "START" text changes to purple, indicating that the PCF8575
|
||||
and MPR121 modes are being tested. Next, when you touch the touch IO pins, you can
|
||||
observe that the GPIO level of PCF8575 on the screen flips (one flip per touch). The touch
|
||||
range includes TOUCH0 (0-11) and TOUCH1 (0-3). If this occurs, it indicates a successful
|
||||
test of PCF8575 and MPR121; otherwise, it fails.
|
||||
When touching the 11th pin of TOUCH1, the system can be switched to test PCM5102A
|
||||
mode.If an SD card is present, it will scan all files inside and display them on the T-Display-S3
|
||||
screen. It will then proceed to play the ".mp3" file. Otherwise, the screen will display
|
||||
"Failed to open directory" (requiring a mode switch to attempt playback again). Any other
|
||||
situation will be considered a test failure.
|
||||
|
||||
* @version: V1.0.0
|
||||
* @Author: LILYGO_L
|
||||
* @Date: 2023-06-12 16:16:37
|
||||
* @LastEditors: LILYGO_L
|
||||
* @LastEditTime: 2023-07-10 09:56:52
|
||||
*/
|
||||
|
||||
#include "Arduino.h"
|
||||
#include "PCF8575.h"
|
||||
#include "TFT_eSPI.h"
|
||||
#include "pin_config.h"
|
||||
#include "Adafruit_MPR121.h"
|
||||
#include "FS.h"
|
||||
#include "SPI.h"
|
||||
#include "SD.h"
|
||||
#include "Audio.h"
|
||||
|
||||
TFT_eSPI tft;
|
||||
Audio audio;
|
||||
|
||||
int32_t Windows = 0;
|
||||
|
||||
// Function interrupt
|
||||
void PCF8575_MPR121_External_Interrupt();
|
||||
|
||||
// Set IIC address
|
||||
PCF8575 PCF8575_Class(T_DISPLAY_PCF8575_IIC_ADDRESS, PCF8575_SDA, PCF8575_SCL, T_DISPLAY_PCF8575_INTERRUPTPIN, PCF8575_MPR121_External_Interrupt);
|
||||
bool PCF8575_External_Interrupt_Flag = false;
|
||||
|
||||
Adafruit_MPR121 Touch_Sensor1 = Adafruit_MPR121();
|
||||
Adafruit_MPR121 Touch_Sensor2 = Adafruit_MPR121();
|
||||
bool MPR121_External_Interrupt_Flag = false;
|
||||
|
||||
uint16_t touched1 = 0;
|
||||
uint16_t touched2 = 0;
|
||||
uint16_t MPR121_IRQ_Number = 0;
|
||||
|
||||
File File_CurrentFile;
|
||||
File File_Root;
|
||||
bool File_Scan_Flag = true;
|
||||
|
||||
void PCF8575_MPR121_External_Interrupt()
|
||||
{
|
||||
// Interrupt called (No Serial no read no wire in this function, and DEBUG disabled on PCF library)
|
||||
PCF8575_External_Interrupt_Flag = true;
|
||||
MPR121_External_Interrupt_Flag = true;
|
||||
}
|
||||
|
||||
const char *PCF8575_TFT_Show(uint8_t Num)
|
||||
{
|
||||
if (Num == 0)
|
||||
{
|
||||
return "LOW";
|
||||
}
|
||||
else
|
||||
{
|
||||
return "HIGH";
|
||||
}
|
||||
}
|
||||
|
||||
/**
|
||||
* @brief PCF8575 test program, detect the mode of IO ports, and measure the voltage
|
||||
level of the corresponding IO ports using a multimeter.
|
||||
|
||||
* @return
|
||||
* @Date 2023-07-13 10:06:19
|
||||
*/
|
||||
void PCF8575_Test(void)
|
||||
{
|
||||
tft.fillScreen(TFT_BLACK);
|
||||
|
||||
tft.setTextColor(TFT_VIOLET);
|
||||
tft.drawString("START", 0, 5, 4);
|
||||
tft.setTextColor(TFT_BROWN);
|
||||
// tft.drawString("|---PCF8575_MPR121---|", 100, 10, 2);
|
||||
tft.drawString("|---TDS3_MIDI_Driver---|", 90, 10, 2);
|
||||
tft.drawString("LILYGO", 260, 5, 1);
|
||||
tft.drawString("V1.0.0", 260, 17, 1);
|
||||
tft.setTextColor(TFT_WHITE);
|
||||
|
||||
PCF8575::DigitalInput readAllPin = PCF8575_Class.digitalReadAll();
|
||||
delay(50); // Anti-jamming machine delay
|
||||
|
||||
tft.drawString("READ VALUE FROM PCF8575: ", 5, 30, 2);
|
||||
|
||||
tft.setTextColor(TFT_PINK);
|
||||
tft.drawString("PORT 0", 50, 50, 1);
|
||||
tft.setTextColor(TFT_WHITE);
|
||||
|
||||
tft.setTextColor(TFT_PINK);
|
||||
tft.drawString("PORT 1", 210, 50, 1);
|
||||
tft.setTextColor(TFT_WHITE);
|
||||
|
||||
tft.drawString("GPIO_00", 20, 60, 1);
|
||||
tft.drawString("->", 75, 60, 1);
|
||||
tft.setTextColor(TFT_BLACK);
|
||||
tft.drawString("LOW", 100, 60, 1);
|
||||
tft.drawString("HIGH", 100, 60, 1);
|
||||
tft.setTextColor(TFT_WHITE);
|
||||
tft.drawString(PCF8575_TFT_Show(readAllPin.p0), 100, 60, 1);
|
||||
|
||||
tft.drawString("GPIO_01", 20, 74, 1);
|
||||
tft.drawString("->", 75, 74, 1);
|
||||
tft.setTextColor(TFT_BLACK);
|
||||
tft.drawString("LOW", 100, 74, 1);
|
||||
tft.drawString("HIGH", 100, 74, 1);
|
||||
tft.setTextColor(TFT_WHITE);
|
||||
tft.drawString(PCF8575_TFT_Show(readAllPin.p1), 100, 74, 1);
|
||||
|
||||
tft.drawString("GPIO_02", 20, 88, 1);
|
||||
tft.drawString("->", 75, 88, 1);
|
||||
tft.setTextColor(TFT_BLACK);
|
||||
tft.drawString("LOW", 100, 88, 1);
|
||||
tft.drawString("HIGH", 100, 88, 1);
|
||||
tft.setTextColor(TFT_WHITE);
|
||||
tft.drawString(PCF8575_TFT_Show(readAllPin.p2), 100, 88, 1);
|
||||
|
||||
tft.drawString("GPIO_03", 20, 102, 1);
|
||||
tft.drawString("->", 75, 102, 1);
|
||||
tft.setTextColor(TFT_BLACK);
|
||||
tft.drawString("LOW", 100, 102, 1);
|
||||
tft.drawString("HIGH", 100, 102, 1);
|
||||
tft.setTextColor(TFT_WHITE);
|
||||
tft.drawString(PCF8575_TFT_Show(readAllPin.p3), 100, 102, 1);
|
||||
|
||||
tft.drawString("GPIO_04", 20, 116, 1);
|
||||
tft.drawString("->", 75, 116, 1);
|
||||
tft.setTextColor(TFT_BLACK);
|
||||
tft.drawString("LOW", 100, 116, 1);
|
||||
tft.drawString("HIGH", 100, 116, 1);
|
||||
tft.setTextColor(TFT_WHITE);
|
||||
tft.drawString(PCF8575_TFT_Show(readAllPin.p4), 100, 116, 1);
|
||||
|
||||
tft.drawString("GPIO_05", 20, 130, 1);
|
||||
tft.drawString("->", 75, 130, 1);
|
||||
tft.setTextColor(TFT_BLACK);
|
||||
tft.drawString("LOW", 100, 130, 1);
|
||||
tft.drawString("HIGH", 100, 130, 1);
|
||||
tft.setTextColor(TFT_WHITE);
|
||||
tft.drawString(PCF8575_TFT_Show(readAllPin.p5), 100, 130, 1);
|
||||
|
||||
tft.drawString("GPIO_06", 20, 144, 1);
|
||||
tft.drawString("->", 75, 144, 1);
|
||||
tft.setTextColor(TFT_BLACK);
|
||||
tft.drawString("LOW", 100, 144, 1);
|
||||
tft.drawString("HIGH", 100, 144, 1);
|
||||
tft.setTextColor(TFT_WHITE);
|
||||
tft.drawString(PCF8575_TFT_Show(readAllPin.p6), 100, 144, 1);
|
||||
|
||||
tft.drawString("GPIO_07", 20, 158, 1);
|
||||
tft.drawString("->", 75, 158, 1);
|
||||
tft.setTextColor(TFT_BLACK);
|
||||
tft.drawString("LOW", 100, 158, 1);
|
||||
tft.drawString("HIGH", 100, 158, 1);
|
||||
tft.setTextColor(TFT_WHITE);
|
||||
tft.drawString(PCF8575_TFT_Show(readAllPin.p7), 100, 158, 1);
|
||||
|
||||
tft.drawString("GPIO_08", 20 + 160, 60, 1);
|
||||
tft.drawString("->", 75 + 160, 60, 1);
|
||||
tft.setTextColor(TFT_BLACK);
|
||||
tft.drawString("LOW", 100 + 160, 60, 1);
|
||||
tft.drawString("HIGH", 100 + 160, 60, 1);
|
||||
tft.setTextColor(TFT_WHITE);
|
||||
tft.drawString(PCF8575_TFT_Show(readAllPin.p8), 100 + 160, 60, 1);
|
||||
|
||||
tft.drawString("GPIO_09", 20 + 160, 74, 1);
|
||||
tft.drawString("->", 75 + 160, 74, 1);
|
||||
tft.setTextColor(TFT_BLACK);
|
||||
tft.drawString("LOW", 100 + 160, 74, 1);
|
||||
tft.drawString("HIGH", 100 + 160, 74, 1);
|
||||
tft.setTextColor(TFT_WHITE);
|
||||
tft.drawString(PCF8575_TFT_Show(readAllPin.p9), 100 + 160, 74, 1);
|
||||
|
||||
tft.drawString("GPIO_10", 20 + 160, 88, 1);
|
||||
tft.drawString("->", 75 + 160, 88, 1);
|
||||
tft.setTextColor(TFT_BLACK);
|
||||
tft.drawString("LOW", 100 + 160, 88, 1);
|
||||
tft.drawString("HIGH", 100 + 160, 88, 1);
|
||||
tft.setTextColor(TFT_WHITE);
|
||||
tft.drawString(PCF8575_TFT_Show(readAllPin.p10), 100 + 160, 88, 1);
|
||||
|
||||
tft.drawString("GPIO_11", 20 + 160, 102, 1);
|
||||
tft.drawString("->", 75 + 160, 102, 1);
|
||||
tft.setTextColor(TFT_BLACK);
|
||||
tft.drawString("LOW", 100 + 160, 102, 1);
|
||||
tft.drawString("HIGH", 100 + 160, 102, 1);
|
||||
tft.setTextColor(TFT_WHITE);
|
||||
tft.drawString(PCF8575_TFT_Show(readAllPin.p11), 100 + 160, 102, 1);
|
||||
|
||||
tft.drawString("GPIO_12", 20 + 160, 116, 1);
|
||||
tft.drawString("->", 75 + 160, 116, 1);
|
||||
tft.setTextColor(TFT_BLACK);
|
||||
tft.drawString("LOW", 100 + 160, 116, 1);
|
||||
tft.drawString("HIGH", 100 + 160, 116, 1);
|
||||
tft.setTextColor(TFT_WHITE);
|
||||
tft.drawString(PCF8575_TFT_Show(readAllPin.p12), 100 + 160, 116, 1);
|
||||
|
||||
tft.drawString("GPIO_13", 20 + 160, 130, 1);
|
||||
tft.drawString("->", 75 + 160, 130, 1);
|
||||
tft.setTextColor(TFT_BLACK);
|
||||
tft.drawString("LOW", 100 + 160, 130, 1);
|
||||
tft.drawString("HIGH", 100 + 160, 130, 1);
|
||||
tft.setTextColor(TFT_WHITE);
|
||||
tft.drawString(PCF8575_TFT_Show(readAllPin.p13), 100 + 160, 130, 1);
|
||||
|
||||
tft.drawString("GPIO_14", 20 + 160, 144, 1);
|
||||
tft.drawString("->", 75 + 160, 144, 1);
|
||||
tft.setTextColor(TFT_BLACK);
|
||||
tft.drawString("LOW", 100 + 160, 144, 1);
|
||||
tft.drawString("HIGH", 100 + 160, 144, 1);
|
||||
tft.setTextColor(TFT_WHITE);
|
||||
tft.drawString(PCF8575_TFT_Show(readAllPin.p14), 100 + 160, 144, 1);
|
||||
|
||||
tft.drawString("GPIO_15", 20 + 160, 158, 1);
|
||||
tft.drawString("->", 75 + 160, 158, 1);
|
||||
tft.setTextColor(TFT_BLACK);
|
||||
tft.drawString("LOW", 100 + 160, 158, 1);
|
||||
tft.drawString("HIGH", 100 + 160, 158, 1);
|
||||
tft.setTextColor(TFT_WHITE);
|
||||
tft.drawString(PCF8575_TFT_Show(readAllPin.p15), 100 + 160, 158, 1);
|
||||
}
|
||||
|
||||
/**
|
||||
* @brief MPR121 test program for detecting whether touch IO is triggered and recording
|
||||
the IO port number after the trigger for linkage debugging with PCF8575.
|
||||
|
||||
* @return
|
||||
* @Date 2023-07-13 11:32:22
|
||||
*/
|
||||
void MPR121_Test(void)
|
||||
{
|
||||
for (uint8_t i = 0; i < 12; i++)
|
||||
{
|
||||
if (touched1 & (1 << i))
|
||||
{
|
||||
for (uint8_t i = 0; i < 16; i++)
|
||||
{
|
||||
PCF8575_Class.pinMode(i, OUTPUT); // Write to output
|
||||
}
|
||||
PCF8575_Class.digitalWrite(i, !PCF8575_Class.digitalRead(i));
|
||||
for (uint8_t i = 0; i < 16; i++)
|
||||
{
|
||||
PCF8575_Class.pinMode(i, INPUT);
|
||||
}
|
||||
}
|
||||
else if (touched2 & (1 << i))
|
||||
{
|
||||
for (uint8_t i = 0; i < 16; i++)
|
||||
{
|
||||
PCF8575_Class.pinMode(i, OUTPUT);
|
||||
}
|
||||
PCF8575_Class.digitalWrite(i + 12, !PCF8575_Class.digitalRead(i + 12));
|
||||
for (uint8_t i = 0; i < 16; i++)
|
||||
{
|
||||
PCF8575_Class.pinMode(i, INPUT);
|
||||
}
|
||||
}
|
||||
}
|
||||
}
|
||||
|
||||
/**
|
||||
* @brief Recursively fetch all files
|
||||
* @return
|
||||
* @Date 2023-07-13 11:36:10
|
||||
*/
|
||||
void getNextFile()
|
||||
{
|
||||
String fileName;
|
||||
if (File_Scan_Flag)
|
||||
{
|
||||
File_Scan_Flag = false;
|
||||
File_Root = SD.open("/");
|
||||
if (!File_Root)
|
||||
{
|
||||
tft.println("Failed to open directory");
|
||||
tft.setCursor(0, 0);
|
||||
return;
|
||||
}
|
||||
}
|
||||
|
||||
File_CurrentFile = File_Root.openNextFile();
|
||||
if (File_CurrentFile)
|
||||
{
|
||||
if (File_CurrentFile.isDirectory())
|
||||
{
|
||||
tft.print("DIR: ");
|
||||
tft.println(File_CurrentFile.name());
|
||||
getNextFile(); // Recursively call to print all file names under the directory
|
||||
}
|
||||
else
|
||||
{
|
||||
fileName = File_CurrentFile.name();
|
||||
fileName.toLowerCase();
|
||||
tft.println("Filename: " + String(fileName));
|
||||
if (fileName.indexOf(".mp3") < 0)
|
||||
{
|
||||
getNextFile(); // If the .mp3 file is less than 3, continue looking for .mp3 file
|
||||
}
|
||||
}
|
||||
}
|
||||
else
|
||||
{
|
||||
File_Scan_Flag = true;
|
||||
getNextFile(); // Returns to the previous root directory until all file searches are complete
|
||||
}
|
||||
}
|
||||
|
||||
/**
|
||||
* @brief Automatically select to play the next song
|
||||
* @return
|
||||
* @Date 2023-07-13 11:35:12
|
||||
*/
|
||||
void playNextAudio()
|
||||
{
|
||||
String sdfile;
|
||||
getNextFile();
|
||||
if (File_CurrentFile)
|
||||
{
|
||||
sdfile = File_CurrentFile.name();
|
||||
audio.connecttoSD(sdfile.c_str());
|
||||
tft.println("\nStart playing: " + sdfile);
|
||||
tft.setCursor(0, 0);
|
||||
}
|
||||
}
|
||||
|
||||
void setup()
|
||||
{
|
||||
Serial.begin(115200);
|
||||
Serial.println("Hello T-Display-S3");
|
||||
|
||||
tft.begin();
|
||||
tft.setRotation(3);
|
||||
// tft.setSwapBytes(true); // Make the picture color by RGB->BGRs
|
||||
tft.fillScreen(TFT_BLACK);
|
||||
|
||||
ledcAttachPin(TFT_BL, 1); // assign TFT_BL pin to channel 1
|
||||
ledcSetup(1, 12000, 8); // 12 kHz PWM, 8-bit resolution
|
||||
ledcWrite(1, 255); // brightness 0 - 255
|
||||
|
||||
// SD SPI
|
||||
SPI.begin(PIN_SD_CLK, PIN_SD_MISO, PIN_SD_MOSI, PIN_SD_CS); // SPI boots
|
||||
|
||||
PCF8575_Class.begin();
|
||||
for (uint8_t i = 0; i < 16; i++)
|
||||
{
|
||||
PCF8575_Class.pinMode(i, INPUT); // Set the IO mode for all PCF8575s
|
||||
}
|
||||
|
||||
Touch_Sensor1.begin(MPR121_ADDR1); // MPR121 address 1
|
||||
Touch_Sensor2.begin(MPR121_ADDR2); // MPR121 address 2
|
||||
|
||||
// PCM5102A IIS
|
||||
audio.setPinout(PCM5102A_BCK, PCM5102A_LRCK, PCM5102A_DIN);
|
||||
audio.setVolume(10); // 0...21,Volume setting
|
||||
|
||||
tft.setTextColor(TFT_RED);
|
||||
tft.drawString("START", 0, 5, 4);
|
||||
tft.drawString("Press any MPR121 touch button to activate", 0, 80, 2);
|
||||
tft.setTextColor(TFT_BROWN);
|
||||
tft.drawString("|---TDS3_MIDI_Driver---|", 90, 10, 2);
|
||||
tft.drawString("LILYGO", 260, 5, 1);
|
||||
tft.drawString("V1.0.0", 260, 17, 1);
|
||||
tft.setTextColor(TFT_WHITE);
|
||||
}
|
||||
|
||||
void loop()
|
||||
{
|
||||
if (MPR121_External_Interrupt_Flag == true)
|
||||
{
|
||||
MPR121_External_Interrupt_Flag = false;
|
||||
|
||||
delay(100); // Anti-jamming machine delay
|
||||
touched1 = Touch_Sensor1.touched(); // Get touch data
|
||||
touched2 = Touch_Sensor2.touched();
|
||||
|
||||
if (touched2 & (1 << 11))
|
||||
{
|
||||
tft.fillScreen(TFT_BLACK);
|
||||
SD.end();
|
||||
SD.begin(PIN_SD_CS, SPI, 40000000);
|
||||
|
||||
playNextAudio();
|
||||
Windows = !Windows;
|
||||
}
|
||||
else
|
||||
{
|
||||
MPR121_Test();
|
||||
}
|
||||
}
|
||||
|
||||
if (PCF8575_External_Interrupt_Flag == true && Windows == 0)
|
||||
{
|
||||
PCF8575_External_Interrupt_Flag = false;
|
||||
PCF8575_Test();
|
||||
}
|
||||
|
||||
if (Windows == 1)
|
||||
{
|
||||
audio.loop();
|
||||
}
|
||||
}
|
71
example/T-Display-S3-MIDI/pin_config.h
Normal file
@ -0,0 +1,71 @@
|
||||
/*
|
||||
* @Description: None
|
||||
* @version: None
|
||||
* @Author: None
|
||||
* @Date: 2023-06-05 13:01:59
|
||||
* @LastEditors: None
|
||||
* @LastEditTime: 2023-07-01 13:36:19
|
||||
*/
|
||||
#pragma once
|
||||
|
||||
/*ESP32S3*/
|
||||
#define PIN_LCD_BL 38
|
||||
|
||||
#define PIN_LCD_D0 39
|
||||
#define PIN_LCD_D1 40
|
||||
#define PIN_LCD_D2 41
|
||||
#define PIN_LCD_D3 42
|
||||
#define PIN_LCD_D4 45
|
||||
#define PIN_LCD_D5 46
|
||||
#define PIN_LCD_D6 47
|
||||
#define PIN_LCD_D7 48
|
||||
|
||||
#define PIN_POWER_ON 15
|
||||
|
||||
#define PIN_LCD_RES 5
|
||||
#define PIN_LCD_CS 6
|
||||
#define PIN_LCD_DC 7
|
||||
#define PIN_LCD_WR 8
|
||||
#define PIN_LCD_RD 9
|
||||
|
||||
#define PIN_BUTTON_1 0
|
||||
#define PIN_BUTTON_2 14
|
||||
#define PIN_BAT_VOLT 4
|
||||
|
||||
#define PIN_IIC_SCL 17
|
||||
#define PIN_IIC_SDA 18
|
||||
|
||||
#define PIN_TOUCH_INT 16
|
||||
#define PIN_TOUCH_RES 21
|
||||
|
||||
/* External expansion */
|
||||
//SD
|
||||
#define PIN_SD_CS 16
|
||||
#define PIN_SD_CLK 21
|
||||
#define PIN_SD_MISO 3
|
||||
#define PIN_SD_MOSI 10
|
||||
|
||||
// MPR121_PIN
|
||||
#define MPR121_ADDR1 0x5A
|
||||
#define MPR121_ADDR2 0x5B // VCC
|
||||
#define MPR121_IRQ_PIN 2
|
||||
#define MPR121_SDA 18
|
||||
#define MPR121_SCL 17
|
||||
|
||||
// PCF8575_PIN
|
||||
#define T_DISPLAY_PCF8575_IIC_ADDRESS 0x20
|
||||
#define PCF8575_IRQ_PIN 2
|
||||
#define PCF8575_SDA 18
|
||||
#define PCF8575_SCL 17
|
||||
#define T_DISPLAY_PCF8575_INTERRUPTPIN 2
|
||||
|
||||
// PCM5102A_PIN
|
||||
#define PCM5102A_LRCK 13
|
||||
#define PCM5102A_DIN 12
|
||||
#define PCM5102A_BCK 11
|
||||
|
||||
// XL95x5_PIN
|
||||
#define T_DISPLAY_XL95x5_IIC_ADDRESS 0X20
|
||||
#define T_DISPLAY_XL95x5_SDA 18 // Default configuration
|
||||
#define T_DISPLAY_XL95x5_SCL 17
|
||||
#define T_DISPLAY_XL95x5_INTERRUPTPIN 2
|
17
lib/ESP32-audioI2S-3.0.0/.github/stale.yml
vendored
Normal file
@ -0,0 +1,17 @@
|
||||
# Number of days of inactivity before an issue becomes stale
|
||||
daysUntilStale: 60
|
||||
# Number of days of inactivity before a stale issue is closed
|
||||
daysUntilClose: 7
|
||||
# Issues with these labels will never be considered stale
|
||||
exemptLabels:
|
||||
- pinned
|
||||
- security
|
||||
# Label to use when marking an issue as stale
|
||||
staleLabel: wontfix
|
||||
# Comment to post when marking an issue as stale. Set to `false` to disable
|
||||
markComment: >
|
||||
This issue has been automatically marked as stale because it has not had
|
||||
recent activity. It will be closed if no further activity occurs. Thank you
|
||||
for your contributions.
|
||||
# Comment to post when closing a stale issue. Set to `false` to disable
|
||||
closeComment: false
|
674
lib/ESP32-audioI2S-3.0.0/LICENSE
Normal file
@ -0,0 +1,674 @@
|
||||
GNU GENERAL PUBLIC LICENSE
|
||||
Version 3, 29 June 2007
|
||||
|
||||
Copyright (C) 2007 Free Software Foundation, Inc. <https://fsf.org/>
|
||||
Everyone is permitted to copy and distribute verbatim copies
|
||||
of this license document, but changing it is not allowed.
|
||||
|
||||
Preamble
|
||||
|
||||
The GNU General Public License is a free, copyleft license for
|
||||
software and other kinds of works.
|
||||
|
||||
The licenses for most software and other practical works are designed
|
||||
to take away your freedom to share and change the works. By contrast,
|
||||
the GNU General Public License is intended to guarantee your freedom to
|
||||
share and change all versions of a program--to make sure it remains free
|
||||
software for all its users. We, the Free Software Foundation, use the
|
||||
GNU General Public License for most of our software; it applies also to
|
||||
any other work released this way by its authors. You can apply it to
|
||||
your programs, too.
|
||||
|
||||
When we speak of free software, we are referring to freedom, not
|
||||
price. Our General Public Licenses are designed to make sure that you
|
||||
have the freedom to distribute copies of free software (and charge for
|
||||
them if you wish), that you receive source code or can get it if you
|
||||
want it, that you can change the software or use pieces of it in new
|
||||
free programs, and that you know you can do these things.
|
||||
|
||||
To protect your rights, we need to prevent others from denying you
|
||||
these rights or asking you to surrender the rights. Therefore, you have
|
||||
certain responsibilities if you distribute copies of the software, or if
|
||||
you modify it: responsibilities to respect the freedom of others.
|
||||
|
||||
For example, if you distribute copies of such a program, whether
|
||||
gratis or for a fee, you must pass on to the recipients the same
|
||||
freedoms that you received. You must make sure that they, too, receive
|
||||
or can get the source code. And you must show them these terms so they
|
||||
know their rights.
|
||||
|
||||
Developers that use the GNU GPL protect your rights with two steps:
|
||||
(1) assert copyright on the software, and (2) offer you this License
|
||||
giving you legal permission to copy, distribute and/or modify it.
|
||||
|
||||
For the developers' and authors' protection, the GPL clearly explains
|
||||
that there is no warranty for this free software. For both users' and
|
||||
authors' sake, the GPL requires that modified versions be marked as
|
||||
changed, so that their problems will not be attributed erroneously to
|
||||
authors of previous versions.
|
||||
|
||||
Some devices are designed to deny users access to install or run
|
||||
modified versions of the software inside them, although the manufacturer
|
||||
can do so. This is fundamentally incompatible with the aim of
|
||||
protecting users' freedom to change the software. The systematic
|
||||
pattern of such abuse occurs in the area of products for individuals to
|
||||
use, which is precisely where it is most unacceptable. Therefore, we
|
||||
have designed this version of the GPL to prohibit the practice for those
|
||||
products. If such problems arise substantially in other domains, we
|
||||
stand ready to extend this provision to those domains in future versions
|
||||
of the GPL, as needed to protect the freedom of users.
|
||||
|
||||
Finally, every program is threatened constantly by software patents.
|
||||
States should not allow patents to restrict development and use of
|
||||
software on general-purpose computers, but in those that do, we wish to
|
||||
avoid the special danger that patents applied to a free program could
|
||||
make it effectively proprietary. To prevent this, the GPL assures that
|
||||
patents cannot be used to render the program non-free.
|
||||
|
||||
The precise terms and conditions for copying, distribution and
|
||||
modification follow.
|
||||
|
||||
TERMS AND CONDITIONS
|
||||
|
||||
0. Definitions.
|
||||
|
||||
"This License" refers to version 3 of the GNU General Public License.
|
||||
|
||||
"Copyright" also means copyright-like laws that apply to other kinds of
|
||||
works, such as semiconductor masks.
|
||||
|
||||
"The Program" refers to any copyrightable work licensed under this
|
||||
License. Each licensee is addressed as "you". "Licensees" and
|
||||
"recipients" may be individuals or organizations.
|
||||
|
||||
To "modify" a work means to copy from or adapt all or part of the work
|
||||
in a fashion requiring copyright permission, other than the making of an
|
||||
exact copy. The resulting work is called a "modified version" of the
|
||||
earlier work or a work "based on" the earlier work.
|
||||
|
||||
A "covered work" means either the unmodified Program or a work based
|
||||
on the Program.
|
||||
|
||||
To "propagate" a work means to do anything with it that, without
|
||||
permission, would make you directly or secondarily liable for
|
||||
infringement under applicable copyright law, except executing it on a
|
||||
computer or modifying a private copy. Propagation includes copying,
|
||||
distribution (with or without modification), making available to the
|
||||
public, and in some countries other activities as well.
|
||||
|
||||
To "convey" a work means any kind of propagation that enables other
|
||||
parties to make or receive copies. Mere interaction with a user through
|
||||
a computer network, with no transfer of a copy, is not conveying.
|
||||
|
||||
An interactive user interface displays "Appropriate Legal Notices"
|
||||
to the extent that it includes a convenient and prominently visible
|
||||
feature that (1) displays an appropriate copyright notice, and (2)
|
||||
tells the user that there is no warranty for the work (except to the
|
||||
extent that warranties are provided), that licensees may convey the
|
||||
work under this License, and how to view a copy of this License. If
|
||||
the interface presents a list of user commands or options, such as a
|
||||
menu, a prominent item in the list meets this criterion.
|
||||
|
||||
1. Source Code.
|
||||
|
||||
The "source code" for a work means the preferred form of the work
|
||||
for making modifications to it. "Object code" means any non-source
|
||||
form of a work.
|
||||
|
||||
A "Standard Interface" means an interface that either is an official
|
||||
standard defined by a recognized standards body, or, in the case of
|
||||
interfaces specified for a particular programming language, one that
|
||||
is widely used among developers working in that language.
|
||||
|
||||
The "System Libraries" of an executable work include anything, other
|
||||
than the work as a whole, that (a) is included in the normal form of
|
||||
packaging a Major Component, but which is not part of that Major
|
||||
Component, and (b) serves only to enable use of the work with that
|
||||
Major Component, or to implement a Standard Interface for which an
|
||||
implementation is available to the public in source code form. A
|
||||
"Major Component", in this context, means a major essential component
|
||||
(kernel, window system, and so on) of the specific operating system
|
||||
(if any) on which the executable work runs, or a compiler used to
|
||||
produce the work, or an object code interpreter used to run it.
|
||||
|
||||
The "Corresponding Source" for a work in object code form means all
|
||||
the source code needed to generate, install, and (for an executable
|
||||
work) run the object code and to modify the work, including scripts to
|
||||
control those activities. However, it does not include the work's
|
||||
System Libraries, or general-purpose tools or generally available free
|
||||
programs which are used unmodified in performing those activities but
|
||||
which are not part of the work. For example, Corresponding Source
|
||||
includes interface definition files associated with source files for
|
||||
the work, and the source code for shared libraries and dynamically
|
||||
linked subprograms that the work is specifically designed to require,
|
||||
such as by intimate data communication or control flow between those
|
||||
subprograms and other parts of the work.
|
||||
|
||||
The Corresponding Source need not include anything that users
|
||||
can regenerate automatically from other parts of the Corresponding
|
||||
Source.
|
||||
|
||||
The Corresponding Source for a work in source code form is that
|
||||
same work.
|
||||
|
||||
2. Basic Permissions.
|
||||
|
||||
All rights granted under this License are granted for the term of
|
||||
copyright on the Program, and are irrevocable provided the stated
|
||||
conditions are met. This License explicitly affirms your unlimited
|
||||
permission to run the unmodified Program. The output from running a
|
||||
covered work is covered by this License only if the output, given its
|
||||
content, constitutes a covered work. This License acknowledges your
|
||||
rights of fair use or other equivalent, as provided by copyright law.
|
||||
|
||||
You may make, run and propagate covered works that you do not
|
||||
convey, without conditions so long as your license otherwise remains
|
||||
in force. You may convey covered works to others for the sole purpose
|
||||
of having them make modifications exclusively for you, or provide you
|
||||
with facilities for running those works, provided that you comply with
|
||||
the terms of this License in conveying all material for which you do
|
||||
not control copyright. Those thus making or running the covered works
|
||||
for you must do so exclusively on your behalf, under your direction
|
||||
and control, on terms that prohibit them from making any copies of
|
||||
your copyrighted material outside their relationship with you.
|
||||
|
||||
Conveying under any other circumstances is permitted solely under
|
||||
the conditions stated below. Sublicensing is not allowed; section 10
|
||||
makes it unnecessary.
|
||||
|
||||
3. Protecting Users' Legal Rights From Anti-Circumvention Law.
|
||||
|
||||
No covered work shall be deemed part of an effective technological
|
||||
measure under any applicable law fulfilling obligations under article
|
||||
11 of the WIPO copyright treaty adopted on 20 December 1996, or
|
||||
similar laws prohibiting or restricting circumvention of such
|
||||
measures.
|
||||
|
||||
When you convey a covered work, you waive any legal power to forbid
|
||||
circumvention of technological measures to the extent such circumvention
|
||||
is effected by exercising rights under this License with respect to
|
||||
the covered work, and you disclaim any intention to limit operation or
|
||||
modification of the work as a means of enforcing, against the work's
|
||||
users, your or third parties' legal rights to forbid circumvention of
|
||||
technological measures.
|
||||
|
||||
4. Conveying Verbatim Copies.
|
||||
|
||||
You may convey verbatim copies of the Program's source code as you
|
||||
receive it, in any medium, provided that you conspicuously and
|
||||
appropriately publish on each copy an appropriate copyright notice;
|
||||
keep intact all notices stating that this License and any
|
||||
non-permissive terms added in accord with section 7 apply to the code;
|
||||
keep intact all notices of the absence of any warranty; and give all
|
||||
recipients a copy of this License along with the Program.
|
||||
|
||||
You may charge any price or no price for each copy that you convey,
|
||||
and you may offer support or warranty protection for a fee.
|
||||
|
||||
5. Conveying Modified Source Versions.
|
||||
|
||||
You may convey a work based on the Program, or the modifications to
|
||||
produce it from the Program, in the form of source code under the
|
||||
terms of section 4, provided that you also meet all of these conditions:
|
||||
|
||||
a) The work must carry prominent notices stating that you modified
|
||||
it, and giving a relevant date.
|
||||
|
||||
b) The work must carry prominent notices stating that it is
|
||||
released under this License and any conditions added under section
|
||||
7. This requirement modifies the requirement in section 4 to
|
||||
"keep intact all notices".
|
||||
|
||||
c) You must license the entire work, as a whole, under this
|
||||
License to anyone who comes into possession of a copy. This
|
||||
License will therefore apply, along with any applicable section 7
|
||||
additional terms, to the whole of the work, and all its parts,
|
||||
regardless of how they are packaged. This License gives no
|
||||
permission to license the work in any other way, but it does not
|
||||
invalidate such permission if you have separately received it.
|
||||
|
||||
d) If the work has interactive user interfaces, each must display
|
||||
Appropriate Legal Notices; however, if the Program has interactive
|
||||
interfaces that do not display Appropriate Legal Notices, your
|
||||
work need not make them do so.
|
||||
|
||||
A compilation of a covered work with other separate and independent
|
||||
works, which are not by their nature extensions of the covered work,
|
||||
and which are not combined with it such as to form a larger program,
|
||||
in or on a volume of a storage or distribution medium, is called an
|
||||
"aggregate" if the compilation and its resulting copyright are not
|
||||
used to limit the access or legal rights of the compilation's users
|
||||
beyond what the individual works permit. Inclusion of a covered work
|
||||
in an aggregate does not cause this License to apply to the other
|
||||
parts of the aggregate.
|
||||
|
||||
6. Conveying Non-Source Forms.
|
||||
|
||||
You may convey a covered work in object code form under the terms
|
||||
of sections 4 and 5, provided that you also convey the
|
||||
machine-readable Corresponding Source under the terms of this License,
|
||||
in one of these ways:
|
||||
|
||||
a) Convey the object code in, or embodied in, a physical product
|
||||
(including a physical distribution medium), accompanied by the
|
||||
Corresponding Source fixed on a durable physical medium
|
||||
customarily used for software interchange.
|
||||
|
||||
b) Convey the object code in, or embodied in, a physical product
|
||||
(including a physical distribution medium), accompanied by a
|
||||
written offer, valid for at least three years and valid for as
|
||||
long as you offer spare parts or customer support for that product
|
||||
model, to give anyone who possesses the object code either (1) a
|
||||
copy of the Corresponding Source for all the software in the
|
||||
product that is covered by this License, on a durable physical
|
||||
medium customarily used for software interchange, for a price no
|
||||
more than your reasonable cost of physically performing this
|
||||
conveying of source, or (2) access to copy the
|
||||
Corresponding Source from a network server at no charge.
|
||||
|
||||
c) Convey individual copies of the object code with a copy of the
|
||||
written offer to provide the Corresponding Source. This
|
||||
alternative is allowed only occasionally and noncommercially, and
|
||||
only if you received the object code with such an offer, in accord
|
||||
with subsection 6b.
|
||||
|
||||
d) Convey the object code by offering access from a designated
|
||||
place (gratis or for a charge), and offer equivalent access to the
|
||||
Corresponding Source in the same way through the same place at no
|
||||
further charge. You need not require recipients to copy the
|
||||
Corresponding Source along with the object code. If the place to
|
||||
copy the object code is a network server, the Corresponding Source
|
||||
may be on a different server (operated by you or a third party)
|
||||
that supports equivalent copying facilities, provided you maintain
|
||||
clear directions next to the object code saying where to find the
|
||||
Corresponding Source. Regardless of what server hosts the
|
||||
Corresponding Source, you remain obligated to ensure that it is
|
||||
available for as long as needed to satisfy these requirements.
|
||||
|
||||
e) Convey the object code using peer-to-peer transmission, provided
|
||||
you inform other peers where the object code and Corresponding
|
||||
Source of the work are being offered to the general public at no
|
||||
charge under subsection 6d.
|
||||
|
||||
A separable portion of the object code, whose source code is excluded
|
||||
from the Corresponding Source as a System Library, need not be
|
||||
included in conveying the object code work.
|
||||
|
||||
A "User Product" is either (1) a "consumer product", which means any
|
||||
tangible personal property which is normally used for personal, family,
|
||||
or household purposes, or (2) anything designed or sold for incorporation
|
||||
into a dwelling. In determining whether a product is a consumer product,
|
||||
doubtful cases shall be resolved in favor of coverage. For a particular
|
||||
product received by a particular user, "normally used" refers to a
|
||||
typical or common use of that class of product, regardless of the status
|
||||
of the particular user or of the way in which the particular user
|
||||
actually uses, or expects or is expected to use, the product. A product
|
||||
is a consumer product regardless of whether the product has substantial
|
||||
commercial, industrial or non-consumer uses, unless such uses represent
|
||||
the only significant mode of use of the product.
|
||||
|
||||
"Installation Information" for a User Product means any methods,
|
||||
procedures, authorization keys, or other information required to install
|
||||
and execute modified versions of a covered work in that User Product from
|
||||
a modified version of its Corresponding Source. The information must
|
||||
suffice to ensure that the continued functioning of the modified object
|
||||
code is in no case prevented or interfered with solely because
|
||||
modification has been made.
|
||||
|
||||
If you convey an object code work under this section in, or with, or
|
||||
specifically for use in, a User Product, and the conveying occurs as
|
||||
part of a transaction in which the right of possession and use of the
|
||||
User Product is transferred to the recipient in perpetuity or for a
|
||||
fixed term (regardless of how the transaction is characterized), the
|
||||
Corresponding Source conveyed under this section must be accompanied
|
||||
by the Installation Information. But this requirement does not apply
|
||||
if neither you nor any third party retains the ability to install
|
||||
modified object code on the User Product (for example, the work has
|
||||
been installed in ROM).
|
||||
|
||||
The requirement to provide Installation Information does not include a
|
||||
requirement to continue to provide support service, warranty, or updates
|
||||
for a work that has been modified or installed by the recipient, or for
|
||||
the User Product in which it has been modified or installed. Access to a
|
||||
network may be denied when the modification itself materially and
|
||||
adversely affects the operation of the network or violates the rules and
|
||||
protocols for communication across the network.
|
||||
|
||||
Corresponding Source conveyed, and Installation Information provided,
|
||||
in accord with this section must be in a format that is publicly
|
||||
documented (and with an implementation available to the public in
|
||||
source code form), and must require no special password or key for
|
||||
unpacking, reading or copying.
|
||||
|
||||
7. Additional Terms.
|
||||
|
||||
"Additional permissions" are terms that supplement the terms of this
|
||||
License by making exceptions from one or more of its conditions.
|
||||
Additional permissions that are applicable to the entire Program shall
|
||||
be treated as though they were included in this License, to the extent
|
||||
that they are valid under applicable law. If additional permissions
|
||||
apply only to part of the Program, that part may be used separately
|
||||
under those permissions, but the entire Program remains governed by
|
||||
this License without regard to the additional permissions.
|
||||
|
||||
When you convey a copy of a covered work, you may at your option
|
||||
remove any additional permissions from that copy, or from any part of
|
||||
it. (Additional permissions may be written to require their own
|
||||
removal in certain cases when you modify the work.) You may place
|
||||
additional permissions on material, added by you to a covered work,
|
||||
for which you have or can give appropriate copyright permission.
|
||||
|
||||
Notwithstanding any other provision of this License, for material you
|
||||
add to a covered work, you may (if authorized by the copyright holders of
|
||||
that material) supplement the terms of this License with terms:
|
||||
|
||||
a) Disclaiming warranty or limiting liability differently from the
|
||||
terms of sections 15 and 16 of this License; or
|
||||
|
||||
b) Requiring preservation of specified reasonable legal notices or
|
||||
author attributions in that material or in the Appropriate Legal
|
||||
Notices displayed by works containing it; or
|
||||
|
||||
c) Prohibiting misrepresentation of the origin of that material, or
|
||||
requiring that modified versions of such material be marked in
|
||||
reasonable ways as different from the original version; or
|
||||
|
||||
d) Limiting the use for publicity purposes of names of licensors or
|
||||
authors of the material; or
|
||||
|
||||
e) Declining to grant rights under trademark law for use of some
|
||||
trade names, trademarks, or service marks; or
|
||||
|
||||
f) Requiring indemnification of licensors and authors of that
|
||||
material by anyone who conveys the material (or modified versions of
|
||||
it) with contractual assumptions of liability to the recipient, for
|
||||
any liability that these contractual assumptions directly impose on
|
||||
those licensors and authors.
|
||||
|
||||
All other non-permissive additional terms are considered "further
|
||||
restrictions" within the meaning of section 10. If the Program as you
|
||||
received it, or any part of it, contains a notice stating that it is
|
||||
governed by this License along with a term that is a further
|
||||
restriction, you may remove that term. If a license document contains
|
||||
a further restriction but permits relicensing or conveying under this
|
||||
License, you may add to a covered work material governed by the terms
|
||||
of that license document, provided that the further restriction does
|
||||
not survive such relicensing or conveying.
|
||||
|
||||
If you add terms to a covered work in accord with this section, you
|
||||
must place, in the relevant source files, a statement of the
|
||||
additional terms that apply to those files, or a notice indicating
|
||||
where to find the applicable terms.
|
||||
|
||||
Additional terms, permissive or non-permissive, may be stated in the
|
||||
form of a separately written license, or stated as exceptions;
|
||||
the above requirements apply either way.
|
||||
|
||||
8. Termination.
|
||||
|
||||
You may not propagate or modify a covered work except as expressly
|
||||
provided under this License. Any attempt otherwise to propagate or
|
||||
modify it is void, and will automatically terminate your rights under
|
||||
this License (including any patent licenses granted under the third
|
||||
paragraph of section 11).
|
||||
|
||||
However, if you cease all violation of this License, then your
|
||||
license from a particular copyright holder is reinstated (a)
|
||||
provisionally, unless and until the copyright holder explicitly and
|
||||
finally terminates your license, and (b) permanently, if the copyright
|
||||
holder fails to notify you of the violation by some reasonable means
|
||||
prior to 60 days after the cessation.
|
||||
|
||||
Moreover, your license from a particular copyright holder is
|
||||
reinstated permanently if the copyright holder notifies you of the
|
||||
violation by some reasonable means, this is the first time you have
|
||||
received notice of violation of this License (for any work) from that
|
||||
copyright holder, and you cure the violation prior to 30 days after
|
||||
your receipt of the notice.
|
||||
|
||||
Termination of your rights under this section does not terminate the
|
||||
licenses of parties who have received copies or rights from you under
|
||||
this License. If your rights have been terminated and not permanently
|
||||
reinstated, you do not qualify to receive new licenses for the same
|
||||
material under section 10.
|
||||
|
||||
9. Acceptance Not Required for Having Copies.
|
||||
|
||||
You are not required to accept this License in order to receive or
|
||||
run a copy of the Program. Ancillary propagation of a covered work
|
||||
occurring solely as a consequence of using peer-to-peer transmission
|
||||
to receive a copy likewise does not require acceptance. However,
|
||||
nothing other than this License grants you permission to propagate or
|
||||
modify any covered work. These actions infringe copyright if you do
|
||||
not accept this License. Therefore, by modifying or propagating a
|
||||
covered work, you indicate your acceptance of this License to do so.
|
||||
|
||||
10. Automatic Licensing of Downstream Recipients.
|
||||
|
||||
Each time you convey a covered work, the recipient automatically
|
||||
receives a license from the original licensors, to run, modify and
|
||||
propagate that work, subject to this License. You are not responsible
|
||||
for enforcing compliance by third parties with this License.
|
||||
|
||||
An "entity transaction" is a transaction transferring control of an
|
||||
organization, or substantially all assets of one, or subdividing an
|
||||
organization, or merging organizations. If propagation of a covered
|
||||
work results from an entity transaction, each party to that
|
||||
transaction who receives a copy of the work also receives whatever
|
||||
licenses to the work the party's predecessor in interest had or could
|
||||
give under the previous paragraph, plus a right to possession of the
|
||||
Corresponding Source of the work from the predecessor in interest, if
|
||||
the predecessor has it or can get it with reasonable efforts.
|
||||
|
||||
You may not impose any further restrictions on the exercise of the
|
||||
rights granted or affirmed under this License. For example, you may
|
||||
not impose a license fee, royalty, or other charge for exercise of
|
||||
rights granted under this License, and you may not initiate litigation
|
||||
(including a cross-claim or counterclaim in a lawsuit) alleging that
|
||||
any patent claim is infringed by making, using, selling, offering for
|
||||
sale, or importing the Program or any portion of it.
|
||||
|
||||
11. Patents.
|
||||
|
||||
A "contributor" is a copyright holder who authorizes use under this
|
||||
License of the Program or a work on which the Program is based. The
|
||||
work thus licensed is called the contributor's "contributor version".
|
||||
|
||||
A contributor's "essential patent claims" are all patent claims
|
||||
owned or controlled by the contributor, whether already acquired or
|
||||
hereafter acquired, that would be infringed by some manner, permitted
|
||||
by this License, of making, using, or selling its contributor version,
|
||||
but do not include claims that would be infringed only as a
|
||||
consequence of further modification of the contributor version. For
|
||||
purposes of this definition, "control" includes the right to grant
|
||||
patent sublicenses in a manner consistent with the requirements of
|
||||
this License.
|
||||
|
||||
Each contributor grants you a non-exclusive, worldwide, royalty-free
|
||||
patent license under the contributor's essential patent claims, to
|
||||
make, use, sell, offer for sale, import and otherwise run, modify and
|
||||
propagate the contents of its contributor version.
|
||||
|
||||
In the following three paragraphs, a "patent license" is any express
|
||||
agreement or commitment, however denominated, not to enforce a patent
|
||||
(such as an express permission to practice a patent or covenant not to
|
||||
sue for patent infringement). To "grant" such a patent license to a
|
||||
party means to make such an agreement or commitment not to enforce a
|
||||
patent against the party.
|
||||
|
||||
If you convey a covered work, knowingly relying on a patent license,
|
||||
and the Corresponding Source of the work is not available for anyone
|
||||
to copy, free of charge and under the terms of this License, through a
|
||||
publicly available network server or other readily accessible means,
|
||||
then you must either (1) cause the Corresponding Source to be so
|
||||
available, or (2) arrange to deprive yourself of the benefit of the
|
||||
patent license for this particular work, or (3) arrange, in a manner
|
||||
consistent with the requirements of this License, to extend the patent
|
||||
license to downstream recipients. "Knowingly relying" means you have
|
||||
actual knowledge that, but for the patent license, your conveying the
|
||||
covered work in a country, or your recipient's use of the covered work
|
||||
in a country, would infringe one or more identifiable patents in that
|
||||
country that you have reason to believe are valid.
|
||||
|
||||
If, pursuant to or in connection with a single transaction or
|
||||
arrangement, you convey, or propagate by procuring conveyance of, a
|
||||
covered work, and grant a patent license to some of the parties
|
||||
receiving the covered work authorizing them to use, propagate, modify
|
||||
or convey a specific copy of the covered work, then the patent license
|
||||
you grant is automatically extended to all recipients of the covered
|
||||
work and works based on it.
|
||||
|
||||
A patent license is "discriminatory" if it does not include within
|
||||
the scope of its coverage, prohibits the exercise of, or is
|
||||
conditioned on the non-exercise of one or more of the rights that are
|
||||
specifically granted under this License. You may not convey a covered
|
||||
work if you are a party to an arrangement with a third party that is
|
||||
in the business of distributing software, under which you make payment
|
||||
to the third party based on the extent of your activity of conveying
|
||||
the work, and under which the third party grants, to any of the
|
||||
parties who would receive the covered work from you, a discriminatory
|
||||
patent license (a) in connection with copies of the covered work
|
||||
conveyed by you (or copies made from those copies), or (b) primarily
|
||||
for and in connection with specific products or compilations that
|
||||
contain the covered work, unless you entered into that arrangement,
|
||||
or that patent license was granted, prior to 28 March 2007.
|
||||
|
||||
Nothing in this License shall be construed as excluding or limiting
|
||||
any implied license or other defenses to infringement that may
|
||||
otherwise be available to you under applicable patent law.
|
||||
|
||||
12. No Surrender of Others' Freedom.
|
||||
|
||||
If conditions are imposed on you (whether by court order, agreement or
|
||||
otherwise) that contradict the conditions of this License, they do not
|
||||
excuse you from the conditions of this License. If you cannot convey a
|
||||
covered work so as to satisfy simultaneously your obligations under this
|
||||
License and any other pertinent obligations, then as a consequence you may
|
||||
not convey it at all. For example, if you agree to terms that obligate you
|
||||
to collect a royalty for further conveying from those to whom you convey
|
||||
the Program, the only way you could satisfy both those terms and this
|
||||
License would be to refrain entirely from conveying the Program.
|
||||
|
||||
13. Use with the GNU Affero General Public License.
|
||||
|
||||
Notwithstanding any other provision of this License, you have
|
||||
permission to link or combine any covered work with a work licensed
|
||||
under version 3 of the GNU Affero General Public License into a single
|
||||
combined work, and to convey the resulting work. The terms of this
|
||||
License will continue to apply to the part which is the covered work,
|
||||
but the special requirements of the GNU Affero General Public License,
|
||||
section 13, concerning interaction through a network will apply to the
|
||||
combination as such.
|
||||
|
||||
14. Revised Versions of this License.
|
||||
|
||||
The Free Software Foundation may publish revised and/or new versions of
|
||||
the GNU General Public License from time to time. Such new versions will
|
||||
be similar in spirit to the present version, but may differ in detail to
|
||||
address new problems or concerns.
|
||||
|
||||
Each version is given a distinguishing version number. If the
|
||||
Program specifies that a certain numbered version of the GNU General
|
||||
Public License "or any later version" applies to it, you have the
|
||||
option of following the terms and conditions either of that numbered
|
||||
version or of any later version published by the Free Software
|
||||
Foundation. If the Program does not specify a version number of the
|
||||
GNU General Public License, you may choose any version ever published
|
||||
by the Free Software Foundation.
|
||||
|
||||
If the Program specifies that a proxy can decide which future
|
||||
versions of the GNU General Public License can be used, that proxy's
|
||||
public statement of acceptance of a version permanently authorizes you
|
||||
to choose that version for the Program.
|
||||
|
||||
Later license versions may give you additional or different
|
||||
permissions. However, no additional obligations are imposed on any
|
||||
author or copyright holder as a result of your choosing to follow a
|
||||
later version.
|
||||
|
||||
15. Disclaimer of Warranty.
|
||||
|
||||
THERE IS NO WARRANTY FOR THE PROGRAM, TO THE EXTENT PERMITTED BY
|
||||
APPLICABLE LAW. EXCEPT WHEN OTHERWISE STATED IN WRITING THE COPYRIGHT
|
||||
HOLDERS AND/OR OTHER PARTIES PROVIDE THE PROGRAM "AS IS" WITHOUT WARRANTY
|
||||
OF ANY KIND, EITHER EXPRESSED OR IMPLIED, INCLUDING, BUT NOT LIMITED TO,
|
||||
THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR
|
||||
PURPOSE. THE ENTIRE RISK AS TO THE QUALITY AND PERFORMANCE OF THE PROGRAM
|
||||
IS WITH YOU. SHOULD THE PROGRAM PROVE DEFECTIVE, YOU ASSUME THE COST OF
|
||||
ALL NECESSARY SERVICING, REPAIR OR CORRECTION.
|
||||
|
||||
16. Limitation of Liability.
|
||||
|
||||
IN NO EVENT UNLESS REQUIRED BY APPLICABLE LAW OR AGREED TO IN WRITING
|
||||
WILL ANY COPYRIGHT HOLDER, OR ANY OTHER PARTY WHO MODIFIES AND/OR CONVEYS
|
||||
THE PROGRAM AS PERMITTED ABOVE, BE LIABLE TO YOU FOR DAMAGES, INCLUDING ANY
|
||||
GENERAL, SPECIAL, INCIDENTAL OR CONSEQUENTIAL DAMAGES ARISING OUT OF THE
|
||||
USE OR INABILITY TO USE THE PROGRAM (INCLUDING BUT NOT LIMITED TO LOSS OF
|
||||
DATA OR DATA BEING RENDERED INACCURATE OR LOSSES SUSTAINED BY YOU OR THIRD
|
||||
PARTIES OR A FAILURE OF THE PROGRAM TO OPERATE WITH ANY OTHER PROGRAMS),
|
||||
EVEN IF SUCH HOLDER OR OTHER PARTY HAS BEEN ADVISED OF THE POSSIBILITY OF
|
||||
SUCH DAMAGES.
|
||||
|
||||
17. Interpretation of Sections 15 and 16.
|
||||
|
||||
If the disclaimer of warranty and limitation of liability provided
|
||||
above cannot be given local legal effect according to their terms,
|
||||
reviewing courts shall apply local law that most closely approximates
|
||||
an absolute waiver of all civil liability in connection with the
|
||||
Program, unless a warranty or assumption of liability accompanies a
|
||||
copy of the Program in return for a fee.
|
||||
|
||||
END OF TERMS AND CONDITIONS
|
||||
|
||||
How to Apply These Terms to Your New Programs
|
||||
|
||||
If you develop a new program, and you want it to be of the greatest
|
||||
possible use to the public, the best way to achieve this is to make it
|
||||
free software which everyone can redistribute and change under these terms.
|
||||
|
||||
To do so, attach the following notices to the program. It is safest
|
||||
to attach them to the start of each source file to most effectively
|
||||
state the exclusion of warranty; and each file should have at least
|
||||
the "copyright" line and a pointer to where the full notice is found.
|
||||
|
||||
<one line to give the program's name and a brief idea of what it does.>
|
||||
Copyright (C) <year> <name of author>
|
||||
|
||||
This program is free software: you can redistribute it and/or modify
|
||||
it under the terms of the GNU General Public License as published by
|
||||
the Free Software Foundation, either version 3 of the License, or
|
||||
(at your option) any later version.
|
||||
|
||||
This program is distributed in the hope that it will be useful,
|
||||
but WITHOUT ANY WARRANTY; without even the implied warranty of
|
||||
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
||||
GNU General Public License for more details.
|
||||
|
||||
You should have received a copy of the GNU General Public License
|
||||
along with this program. If not, see <https://www.gnu.org/licenses/>.
|
||||
|
||||
Also add information on how to contact you by electronic and paper mail.
|
||||
|
||||
If the program does terminal interaction, make it output a short
|
||||
notice like this when it starts in an interactive mode:
|
||||
|
||||
<program> Copyright (C) <year> <name of author>
|
||||
This program comes with ABSOLUTELY NO WARRANTY; for details type `show w'.
|
||||
This is free software, and you are welcome to redistribute it
|
||||
under certain conditions; type `show c' for details.
|
||||
|
||||
The hypothetical commands `show w' and `show c' should show the appropriate
|
||||
parts of the General Public License. Of course, your program's commands
|
||||
might be different; for a GUI interface, you would use an "about box".
|
||||
|
||||
You should also get your employer (if you work as a programmer) or school,
|
||||
if any, to sign a "copyright disclaimer" for the program, if necessary.
|
||||
For more information on this, and how to apply and follow the GNU GPL, see
|
||||
<https://www.gnu.org/licenses/>.
|
||||
|
||||
The GNU General Public License does not permit incorporating your program
|
||||
into proprietary programs. If your program is a subroutine library, you
|
||||
may consider it more useful to permit linking proprietary applications with
|
||||
the library. If this is what you want to do, use the GNU Lesser General
|
||||
Public License instead of this License. But first, please read
|
||||
<https://www.gnu.org/licenses/why-not-lgpl.html>.
|
103
lib/ESP32-audioI2S-3.0.0/README.md
Normal file
@ -0,0 +1,103 @@
|
||||
# ESP32-audioI2S
|
||||
Plays mp3, m4a and wav files from SD card via I2S with external hardware.
|
||||
HELIX-mp3 and -aac decoder is included.
|
||||
Works with MAX98357A (3 Watt amplifier with DAC), connected three lines (DOUT, BLCK, LRC) to I2S.
|
||||
For stereo are two MAX98357A necessary. AudioI2S works with UDA1334A (Adafruit I2S Stereo Decoder Breakout Board), PCM5102A and CS4344.
|
||||
Other HW may work but not tested. Plays also icy-streams and GoogleTTS. Can be compiled with Arduino IDE. [WIKI](https://github.com/schreibfaul1/ESP32-audioI2S/wiki)
|
||||
|
||||
```` c++
|
||||
#include "Arduino.h"
|
||||
#include "WiFi.h"
|
||||
#include "Audio.h"
|
||||
#include "SD.h"
|
||||
#include "FS.h"
|
||||
|
||||
// Digital I/O used
|
||||
#define SD_CS 5
|
||||
#define SPI_MOSI 23
|
||||
#define SPI_MISO 19
|
||||
#define SPI_SCK 18
|
||||
#define I2S_DOUT 25
|
||||
#define I2S_BCLK 27
|
||||
#define I2S_LRC 26
|
||||
|
||||
Audio audio;
|
||||
|
||||
String ssid = "*******";
|
||||
String password = "*******";
|
||||
|
||||
void setup() {
|
||||
pinMode(SD_CS, OUTPUT); digitalWrite(SD_CS, HIGH);
|
||||
SPI.begin(SPI_SCK, SPI_MISO, SPI_MOSI);
|
||||
Serial.begin(115200);
|
||||
SD.begin(SD_CS);
|
||||
WiFi.disconnect();
|
||||
WiFi.mode(WIFI_STA);
|
||||
WiFi.begin(ssid.c_str(), password.c_str());
|
||||
while (WiFi.status() != WL_CONNECTED) delay(1500);
|
||||
audio.setPinout(I2S_BCLK, I2S_LRC, I2S_DOUT);
|
||||
audio.setVolume(21); // default 0...21
|
||||
// or alternative
|
||||
// audio.setVolumeSteps(64); // max 255
|
||||
// audio.setVolume(63);
|
||||
//
|
||||
audio.connecttohost("http://iskatel.hostingradio.ru:8015/iskatel-320.aac"); // aac
|
||||
// audio.connecttohost("http://mcrscast.mcr.iol.pt/cidadefm"); // mp3
|
||||
// audio.connecttohost("http://www.wdr.de/wdrlive/media/einslive.m3u"); // m3u
|
||||
// audio.connecttohost("https://stream.srg-ssr.ch/rsp/aacp_48.asx"); // asx
|
||||
// audio.connecttohost("http://tuner.classical102.com/listen.pls"); // pls
|
||||
// audio.connecttohost("http://stream.radioparadise.com/flac"); // flac
|
||||
// audio.connecttohost("http://stream.sing-sing-bis.org:8000/singsingFlac"); // flac (ogg)
|
||||
// audio.connecttohost("http://s1.knixx.fm:5347/dein_webradio_vbr.opus"); // opus (ogg)
|
||||
// audio.connecttohost("http://26373.live.streamtheworld.com:3690/XHQQ_FMAAC/HLSTS/playlist.m3u8"); // HLS
|
||||
// audio.connecttohost("http://eldoradolive02.akamaized.net/hls/live/2043453/eldorado/master.m3u8"); // HLS (ts)
|
||||
// audio.connecttoFS(SD, "/test.wav"); // SD
|
||||
// audio.connecttoFS(SD_MMC, "/test.wav"); // SD_MMC
|
||||
// audio.connecttoFS(SPIFFS, "/test.wav"); // SPIFFS
|
||||
// audio.connecttospeech("Wenn die Hunde schlafen, kann der Wolf gut Schafe stehlen.", "de"); // Google TTS
|
||||
}
|
||||
|
||||
void loop()
|
||||
{
|
||||
audio.loop();
|
||||
}
|
||||
|
||||
// optional
|
||||
void audio_info(const char *info){
|
||||
Serial.print("info "); Serial.println(info);
|
||||
}
|
||||
void audio_id3data(const char *info){ //id3 metadata
|
||||
Serial.print("id3data ");Serial.println(info);
|
||||
}
|
||||
void audio_eof_mp3(const char *info){ //end of file
|
||||
Serial.print("eof_mp3 ");Serial.println(info);
|
||||
}
|
||||
void audio_showstation(const char *info){
|
||||
Serial.print("station ");Serial.println(info);
|
||||
}
|
||||
void audio_showstreamtitle(const char *info){
|
||||
Serial.print("streamtitle ");Serial.println(info);
|
||||
}
|
||||
void audio_bitrate(const char *info){
|
||||
Serial.print("bitrate ");Serial.println(info);
|
||||
}
|
||||
void audio_commercial(const char *info){ //duration in sec
|
||||
Serial.print("commercial ");Serial.println(info);
|
||||
}
|
||||
void audio_icyurl(const char *info){ //homepage
|
||||
Serial.print("icyurl ");Serial.println(info);
|
||||
}
|
||||
void audio_lasthost(const char *info){ //stream URL played
|
||||
Serial.print("lasthost ");Serial.println(info);
|
||||
}
|
||||
void audio_eof_speech(const char *info){
|
||||
Serial.print("eof_speech ");Serial.println(info);
|
||||
}
|
||||
|
||||
````
|
||||
Breadboard
|
||||

|
||||
Wiring
|
||||

|
||||
Impulse diagram
|
||||

|
BIN
lib/ESP32-audioI2S-3.0.0/additional_info/Arduino Library.png
Normal file
After Width: | Height: | Size: 60 KiB |
BIN
lib/ESP32-audioI2S-3.0.0/additional_info/Audio Duration.png
Normal file
After Width: | Height: | Size: 58 KiB |
BIN
lib/ESP32-audioI2S-3.0.0/additional_info/Breadboard.jpg
Normal file
After Width: | Height: | Size: 1.3 MiB |
BIN
lib/ESP32-audioI2S-3.0.0/additional_info/DAC CS4344.jpg
Normal file
After Width: | Height: | Size: 58 KiB |
BIN
lib/ESP32-audioI2S-3.0.0/additional_info/DAC PCM5102A.jpg
Normal file
After Width: | Height: | Size: 38 KiB |
After Width: | Height: | Size: 309 KiB |
BIN
lib/ESP32-audioI2S-3.0.0/additional_info/ESP32_I2S_PCM5102A.JPG
Normal file
After Width: | Height: | Size: 107 KiB |
After Width: | Height: | Size: 84 KiB |
BIN
lib/ESP32-audioI2S-3.0.0/additional_info/ESP32_I2S_UDA1334A.JPG
Normal file
After Width: | Height: | Size: 337 KiB |
BIN
lib/ESP32-audioI2S-3.0.0/additional_info/Impulsdiagramm.jpg
Normal file
After Width: | Height: | Size: 50 KiB |
BIN
lib/ESP32-audioI2S-3.0.0/additional_info/Partition Scheme.png
Normal file
After Width: | Height: | Size: 102 KiB |
BIN
lib/ESP32-audioI2S-3.0.0/additional_info/Testfiles/320k_test.mp3
Normal file
@ -0,0 +1,5 @@
|
||||
#EXTM3U
|
||||
#EXTINF:18,Bjarne Liller - Olsen Banden (Titelmusik der Olsenbande) - Olsen-Banden.mp3
|
||||
https://raw.githubusercontent.com/schreibfaul1/ESP32-audioI2S/master/additional_info/Testfiles/Olsen-Banden.mp3
|
||||
#EXTINF:10,Santiano-Wellermann - Santiano-Wellerman.flac
|
||||
https://raw.githubusercontent.com/schreibfaul1/ESP32-audioI2S/master/additional_info/Testfiles/Santiano-Wellerman.flac
|
BIN
lib/ESP32-audioI2S-3.0.0/additional_info/lowpass.png
Normal file
After Width: | Height: | Size: 31 KiB |
BIN
lib/ESP32-audioI2S-3.0.0/additional_info/minimal.JPG
Normal file
After Width: | Height: | Size: 36 KiB |
BIN
lib/ESP32-audioI2S-3.0.0/additional_info/with PSRAM.png
Normal file
After Width: | Height: | Size: 12 KiB |
BIN
lib/ESP32-audioI2S-3.0.0/additional_info/without PSRAM.png
Normal file
After Width: | Height: | Size: 14 KiB |
After Width: | Height: | Size: 46 KiB |
39
lib/ESP32-audioI2S-3.0.0/examples/DLNA/include/README
Normal file
@ -0,0 +1,39 @@
|
||||
|
||||
This directory is intended for project header files.
|
||||
|
||||
A header file is a file containing C declarations and macro definitions
|
||||
to be shared between several project source files. You request the use of a
|
||||
header file in your project source file (C, C++, etc) located in `src` folder
|
||||
by including it, with the C preprocessing directive `#include'.
|
||||
|
||||
```src/main.c
|
||||
|
||||
#include "header.h"
|
||||
|
||||
int main (void)
|
||||
{
|
||||
...
|
||||
}
|
||||
```
|
||||
|
||||
Including a header file produces the same results as copying the header file
|
||||
into each source file that needs it. Such copying would be time-consuming
|
||||
and error-prone. With a header file, the related declarations appear
|
||||
in only one place. If they need to be changed, they can be changed in one
|
||||
place, and programs that include the header file will automatically use the
|
||||
new version when next recompiled. The header file eliminates the labor of
|
||||
finding and changing all the copies as well as the risk that a failure to
|
||||
find one copy will result in inconsistencies within a program.
|
||||
|
||||
In C, the usual convention is to give header files names that end with `.h'.
|
||||
It is most portable to use only letters, digits, dashes, and underscores in
|
||||
header file names, and at most one dot.
|
||||
|
||||
Read more about using header files in official GCC documentation:
|
||||
|
||||
* Include Syntax
|
||||
* Include Operation
|
||||
* Once-Only Headers
|
||||
* Computed Includes
|
||||
|
||||
https://gcc.gnu.org/onlinedocs/cpp/Header-Files.html
|
46
lib/ESP32-audioI2S-3.0.0/examples/DLNA/lib/README
Normal file
@ -0,0 +1,46 @@
|
||||
|
||||
This directory is intended for project specific (private) libraries.
|
||||
PlatformIO will compile them to static libraries and link into executable file.
|
||||
|
||||
The source code of each library should be placed in a an own separate directory
|
||||
("lib/your_library_name/[here are source files]").
|
||||
|
||||
For example, see a structure of the following two libraries `Foo` and `Bar`:
|
||||
|
||||
|--lib
|
||||
| |
|
||||
| |--Bar
|
||||
| | |--docs
|
||||
| | |--examples
|
||||
| | |--src
|
||||
| | |- Bar.c
|
||||
| | |- Bar.h
|
||||
| | |- library.json (optional, custom build options, etc) https://docs.platformio.org/page/librarymanager/config.html
|
||||
| |
|
||||
| |--Foo
|
||||
| | |- Foo.c
|
||||
| | |- Foo.h
|
||||
| |
|
||||
| |- README --> THIS FILE
|
||||
|
|
||||
|- platformio.ini
|
||||
|--src
|
||||
|- main.c
|
||||
|
||||
and a contents of `src/main.c`:
|
||||
```
|
||||
#include <Foo.h>
|
||||
#include <Bar.h>
|
||||
|
||||
int main (void)
|
||||
{
|
||||
...
|
||||
}
|
||||
|
||||
```
|
||||
|
||||
PlatformIO Library Dependency Finder will find automatically dependent
|
||||
libraries scanning project source files.
|
||||
|
||||
More information about PlatformIO Library Dependency Finder
|
||||
- https://docs.platformio.org/page/librarymanager/ldf.html
|
721
lib/ESP32-audioI2S-3.0.0/examples/DLNA/lib/websrv/websrv.cpp
Normal file
@ -0,0 +1,721 @@
|
||||
/*
|
||||
* websrv.cpp
|
||||
*
|
||||
* Created on: 09.07.2017
|
||||
* updated on: 19.10.2022
|
||||
* Author: Wolle
|
||||
*/
|
||||
|
||||
#include "websrv.h"
|
||||
|
||||
//--------------------------------------------------------------------------------------------------------------
|
||||
WebSrv::WebSrv(String Name, String Version){
|
||||
_Name=Name; _Version=Version;
|
||||
method = HTTP_NONE;
|
||||
}
|
||||
//--------------------------------------------------------------------------------------------------------------
|
||||
void WebSrv::show_not_found(){
|
||||
cmdclient.print("HTTP/1.1 404 Not Found\n\n");
|
||||
return;
|
||||
}
|
||||
//--------------------------------------------------------------------------------------------------------------
|
||||
String WebSrv::calculateWebSocketResponseKey(String sec_WS_key){
|
||||
// input Sec-WebSocket-Key from client
|
||||
// output Sec-WebSocket-Accept-Key (used in response message to client)
|
||||
unsigned char sha1_result[20];
|
||||
String concat = sec_WS_key + WS_sec_conKey;
|
||||
mbedtls_sha1_ret((unsigned char*)concat.c_str(), concat.length(), (unsigned char*) sha1_result );
|
||||
return base64::encode(sha1_result, 20);
|
||||
}
|
||||
//--------------------------------------------------------------------------------------------------------------
|
||||
void WebSrv::printWebSocketHeader(String wsRespKey){
|
||||
String wsHeader = (String)"HTTP/1.1 101 Switching Protocols\r\n" +
|
||||
"Upgrade: websocket\r\n" +
|
||||
"Connection: Upgrade\r\n" +
|
||||
"Sec-WebSocket-Accept: " + wsRespKey + "\r\n" +
|
||||
"Access-Control-Allow-Origin: \r\n\r\n";
|
||||
// "Sec-WebSocket-Protocol: chat\r\n\r\n";
|
||||
//log_i("wsheader %s", wsHeader.c_str());
|
||||
webSocketClient.print(wsHeader) ; // header sent
|
||||
}
|
||||
//--------------------------------------------------------------------------------------------------------------
|
||||
void WebSrv::show(const char* pagename, int16_t len){
|
||||
uint TCPCHUNKSIZE = 1024; // Max number of bytes per write
|
||||
size_t pagelen=0, res=0; // Size of requested page
|
||||
const unsigned char* p;
|
||||
p = reinterpret_cast<const unsigned char*>(pagename);
|
||||
if(len==-1){
|
||||
pagelen=strlen(pagename);
|
||||
}
|
||||
else{
|
||||
if(len>0) pagelen = len;
|
||||
}
|
||||
while((*p=='\n') && (pagelen>0)){ // If page starts with newline:
|
||||
p++; // Skip first character
|
||||
pagelen--;
|
||||
}
|
||||
// HTTP header
|
||||
String httpheader="";
|
||||
httpheader += "HTTP/1.1 200 OK\r\n";
|
||||
httpheader += "Connection: close\r\n";
|
||||
httpheader += "Content-type: text/html\r\n";
|
||||
httpheader += "Content-Length: " + String(pagelen, 10) + "\r\n";
|
||||
httpheader += "Server: " + _Name+ "\r\n";
|
||||
httpheader += "Cache-Control: max-age=86400\r\n";
|
||||
httpheader += "Last-Modified: " + _Version + "\r\n\r\n";
|
||||
|
||||
cmdclient.print(httpheader) ; // header sent
|
||||
|
||||
sprintf(buff, "Length of page is %d", pagelen);
|
||||
if(WEBSRV_onInfo) WEBSRV_onInfo(buff);
|
||||
// The content of the HTTP response follows the header:
|
||||
|
||||
while(pagelen){ // Loop through the output page
|
||||
if (pagelen <= TCPCHUNKSIZE){ // Near the end?
|
||||
res=cmdclient.write(p, pagelen); // Yes, send last part
|
||||
if(res!=pagelen){
|
||||
log_e("write error in webpage");
|
||||
cmdclient.clearWriteError();
|
||||
return;
|
||||
}
|
||||
pagelen = 0;
|
||||
}
|
||||
else{
|
||||
|
||||
res=cmdclient.write(p, TCPCHUNKSIZE); // Send part of the page
|
||||
|
||||
if(res!=TCPCHUNKSIZE){
|
||||
log_e("write error in webpage");
|
||||
cmdclient.clearWriteError();
|
||||
return;
|
||||
}
|
||||
p += TCPCHUNKSIZE; // Update startpoint and rest of bytes
|
||||
pagelen -= TCPCHUNKSIZE;
|
||||
}
|
||||
}
|
||||
return;
|
||||
}
|
||||
//--------------------------------------------------------------------------------------------------------------
|
||||
boolean WebSrv::streamfile(fs::FS &fs,const char* path){ // transfer file from SD to webbrowser
|
||||
size_t bytesPerTransaction = 1024;
|
||||
uint8_t transBuf[bytesPerTransaction], i=0;
|
||||
size_t wIndex = 0, res=0, leftover=0;
|
||||
if(!cmdclient.connected()){log_e("not connected"); return false;}
|
||||
while(path[i] != 0){ // protect SD for invalid signs to avoid a crash!!
|
||||
if(path[i] < 32)return false;
|
||||
i++;
|
||||
}
|
||||
if(!fs.exists(path)) return false;
|
||||
File file = fs.open(path, "r");
|
||||
if(!file){
|
||||
sprintf(buff, "Failed to open file for reading %s", path);
|
||||
if(WEBSRV_onInfo) WEBSRV_onInfo(buff);
|
||||
show_not_found();
|
||||
return false;
|
||||
}
|
||||
sprintf(buff, "Length of file %s is %d", path, file.size());
|
||||
if(WEBSRV_onInfo) WEBSRV_onInfo(buff);
|
||||
|
||||
// HTTP header
|
||||
String httpheader="";
|
||||
httpheader += "HTTP/1.1 200 OK\r\n";
|
||||
httpheader += "Connection: close\r\n";
|
||||
httpheader += "Content-type: " + getContentType(String(path)) +"\r\n";
|
||||
httpheader += "Content-Length: " + String(file.size(),10) + "\r\n";
|
||||
httpheader += "Server: " + _Name+ "\r\n";
|
||||
httpheader += "Cache-Control: max-age=86400\r\n";
|
||||
httpheader += "Last-Modified: " + _Version + "\r\n\r\n";
|
||||
|
||||
cmdclient.print(httpheader) ; // header sent
|
||||
|
||||
while(wIndex+bytesPerTransaction < file.size()){
|
||||
file.read(transBuf, bytesPerTransaction);
|
||||
res=cmdclient.write(transBuf, bytesPerTransaction);
|
||||
wIndex+=res;
|
||||
if(res!=bytesPerTransaction){
|
||||
log_i("write error %s", path);
|
||||
cmdclient.clearWriteError();
|
||||
return false;
|
||||
}
|
||||
}
|
||||
leftover=file.size()-wIndex;
|
||||
file.read(transBuf, leftover);
|
||||
res=cmdclient.write(transBuf, leftover);
|
||||
wIndex+=res;
|
||||
if(res!=leftover){
|
||||
log_i("write error %s", path);
|
||||
cmdclient.clearWriteError();
|
||||
return false;
|
||||
}
|
||||
if(wIndex!=file.size()) log_e("file %s not correct sent", path);
|
||||
file.close();
|
||||
return true;
|
||||
}
|
||||
//--------------------------------------------------------------------------------------------------------------
|
||||
boolean WebSrv::send(String msg, uint8_t opcode) { // sends text messages via websocket
|
||||
return send(msg.c_str(), opcode);
|
||||
}
|
||||
//--------------------------------------------------------------------------------------------------------------
|
||||
boolean WebSrv::send(const char *msg, uint8_t opcode) { // sends text messages via websocket
|
||||
uint8_t headerLen = 2;
|
||||
|
||||
if(!hasclient_WS) {
|
||||
// log_e("can't send, websocketserver not connected");
|
||||
return false;
|
||||
}
|
||||
size_t msgLen = strlen(msg);
|
||||
|
||||
if(msgLen > UINT16_MAX) {
|
||||
log_e("send: message too long, greather than 64kB");
|
||||
return false;
|
||||
}
|
||||
|
||||
uint8_t fin = 1;
|
||||
uint8_t rsv1 = 0;
|
||||
uint8_t rsv2 = 0;
|
||||
uint8_t rsv3 = 0;
|
||||
uint8_t mask = 0;
|
||||
|
||||
buff[0] = (128 * fin) + (64 * rsv1) + (32 * rsv2) + (16 * rsv3) + opcode;
|
||||
if(msgLen < 126) {
|
||||
buff[1] = (128 * mask) + msgLen;
|
||||
}
|
||||
else {
|
||||
headerLen = 4;
|
||||
buff[1] = (128 * mask) + 126;
|
||||
buff[2] = (msgLen >> 8) & 0xFF;
|
||||
buff[3] = msgLen & 0xFF;
|
||||
}
|
||||
|
||||
webSocketClient.write(buff, headerLen);
|
||||
webSocketClient.write(msg, msgLen);
|
||||
|
||||
return true;
|
||||
}
|
||||
//--------------------------------------------------------------------------------------------------------------
|
||||
void WebSrv::sendPing(){ // heartbeat, keep alive via websockets
|
||||
|
||||
if(!hasclient_WS) {
|
||||
return;
|
||||
}
|
||||
uint8_t fin = 1;
|
||||
uint8_t rsv1 = 0;
|
||||
uint8_t rsv2 = 0;
|
||||
uint8_t rsv3 = 0;
|
||||
uint8_t mask = 0;
|
||||
|
||||
buff[0] = (128 * fin) + (64 * rsv1) + (32 * rsv2) + (16 * rsv3) + Ping_Frame;
|
||||
buff[1] = (128 * mask) + 0;
|
||||
webSocketClient.write(buff,2);
|
||||
}
|
||||
//--------------------------------------------------------------------------------------------------------------
|
||||
void WebSrv::sendPong(){ // heartbeat, keep alive via websockets
|
||||
|
||||
if(!hasclient_WS) {
|
||||
return;
|
||||
}
|
||||
uint8_t fin = 1;
|
||||
uint8_t rsv1 = 0;
|
||||
uint8_t rsv2 = 0;
|
||||
uint8_t rsv3 = 0;
|
||||
uint8_t mask = 0;
|
||||
|
||||
buff[0] = (128 * fin) + (64 * rsv1) + (32 * rsv2) + (16 * rsv3) + Pong_Frame;
|
||||
buff[1] = (128 * mask) + 0;
|
||||
webSocketClient.write(buff,2);
|
||||
}
|
||||
//--------------------------------------------------------------------------------------------------------------
|
||||
boolean WebSrv::uploadB64image(fs::FS &fs,const char* path, uint32_t contentLength){ // transfer imagefile from webbrowser to SD
|
||||
size_t bytesPerTransaction = 1024;
|
||||
uint8_t tBuf[bytesPerTransaction];
|
||||
uint16_t av, i, j;
|
||||
uint32_t len = contentLength;
|
||||
boolean f_werror=false;
|
||||
String str="";
|
||||
int n=0;
|
||||
File file;
|
||||
fs.remove(path); // Remove a previous version, otherwise data is appended the file again
|
||||
file = fs.open(path, FILE_WRITE); // Open the file for writing (create it, if doesn't exist)
|
||||
|
||||
log_i("ContentLength %i", contentLength);
|
||||
str = str + cmdclient.readStringUntil(','); // data:image/jpeg;base64,
|
||||
len -= str.length();
|
||||
while(cmdclient.available()){
|
||||
av=cmdclient.available();
|
||||
if(av==0) break;
|
||||
if(av>bytesPerTransaction) av=bytesPerTransaction;
|
||||
if(av>len) av=len;
|
||||
len -= av;
|
||||
i=0; j=0;
|
||||
cmdclient.read(tBuf, av); // b64 decode
|
||||
while(i<av){
|
||||
if(tBuf[i]==0)break; // ignore all other stuff
|
||||
n=B64index[tBuf[i]]<<18 | B64index[tBuf[i+1]]<<12 | B64index[tBuf[i+2]]<<6 | B64index[tBuf[i+3]];
|
||||
tBuf[j ]= n>>16;
|
||||
tBuf[j+1]= n>>8 & 0xFF;
|
||||
tBuf[j+2]= n & 0xFF;
|
||||
i+=4;
|
||||
j+=3;
|
||||
}
|
||||
if(tBuf[j]=='=') j--;
|
||||
if(tBuf[j]=='=') j--; // remove =
|
||||
|
||||
if(file.write(tBuf, j)!=j) f_werror=true; // write error?
|
||||
if(len == 0) break;
|
||||
}
|
||||
cmdclient.readStringUntil('\n'); // read the remains, first \n
|
||||
cmdclient.readStringUntil('\n'); // read the remains webkit\n
|
||||
file.close();
|
||||
if(f_werror) {
|
||||
sprintf(buff, "File: %s write error", path);
|
||||
if(WEBSRV_onInfo) WEBSRV_onInfo(buff);
|
||||
return false;
|
||||
}
|
||||
sprintf(buff, "File: %s written, FileSize: %d", path, contentLength);
|
||||
//log_i(buff);
|
||||
if(WEBSRV_onInfo) WEBSRV_onInfo(buff);
|
||||
return true;
|
||||
}
|
||||
//--------------------------------------------------------------------------------------------------------------
|
||||
boolean WebSrv::uploadfile(fs::FS &fs,const char* path, uint32_t contentLength){ // transfer file from webbrowser to sd
|
||||
size_t bytesPerTransaction = 1024;
|
||||
uint8_t tBuf[bytesPerTransaction];
|
||||
uint16_t av;
|
||||
uint32_t len = contentLength;
|
||||
boolean f_werror=false;
|
||||
String str="";
|
||||
File file;
|
||||
if(fs.exists(path)) fs.remove(path); // Remove a previous version, otherwise data is appended the file again
|
||||
|
||||
file = fs.open(path, FILE_WRITE); // Open the file for writing in SD (create it, if doesn't exist)
|
||||
while(cmdclient.available()){
|
||||
av=cmdclient.available();
|
||||
if(av>bytesPerTransaction) av=bytesPerTransaction;
|
||||
if(av>len) av=len;
|
||||
len -= av;
|
||||
cmdclient.read(tBuf, av);
|
||||
if(file.write(tBuf, av)!=av) f_werror=true; // write error?
|
||||
if(len == 0) break;
|
||||
}
|
||||
cmdclient.readStringUntil('\n'); // read the remains, first \n
|
||||
cmdclient.readStringUntil('\n'); // read the remains webkit\n
|
||||
file.close();
|
||||
if(f_werror) {
|
||||
sprintf(buff, "File: %s write error", path);
|
||||
if(WEBSRV_onInfo) WEBSRV_onInfo(buff);
|
||||
return false;
|
||||
}
|
||||
sprintf(buff, "File: %s written, FileSize %d: ", path, contentLength);
|
||||
if(WEBSRV_onInfo) WEBSRV_onInfo(buff);
|
||||
return true;
|
||||
}
|
||||
//--------------------------------------------------------------------------------------------------------------
|
||||
void WebSrv::begin(uint16_t http_port, uint16_t websocket_port) {
|
||||
cmdserver.begin(http_port);
|
||||
webSocketServer.begin(websocket_port);
|
||||
}
|
||||
//--------------------------------------------------------------------------------------------------------------
|
||||
void WebSrv::stop() {
|
||||
cmdclient.stop();
|
||||
webSocketClient.stop();
|
||||
}
|
||||
//--------------------------------------------------------------------------------------------------------------
|
||||
String WebSrv::getContentType(String filename){
|
||||
if (filename.endsWith(".html")) return "text/html" ;
|
||||
else if (filename.endsWith(".htm" )) return "text/html";
|
||||
else if (filename.endsWith(".css" )) return "text/css";
|
||||
else if (filename.endsWith(".txt" )) return "text/plain";
|
||||
else if (filename.endsWith(".js" )) return "application/javascript";
|
||||
else if (filename.endsWith(".json")) return "application/json";
|
||||
else if (filename.endsWith(".svg" )) return "image/svg+xml";
|
||||
else if (filename.endsWith(".ttf" )) return "application/x-font-ttf";
|
||||
else if (filename.endsWith(".otf" )) return "application/x-font-opentype";
|
||||
else if (filename.endsWith(".xml" )) return "text/xml";
|
||||
else if (filename.endsWith(".pdf" )) return "application/pdf";
|
||||
else if (filename.endsWith(".png" )) return "image/png" ;
|
||||
else if (filename.endsWith(".bmp" )) return "image/bmp" ;
|
||||
else if (filename.endsWith(".gif" )) return "image/gif" ;
|
||||
else if (filename.endsWith(".jpg" )) return "image/jpeg" ;
|
||||
else if (filename.endsWith(".ico" )) return "image/x-icon" ;
|
||||
else if (filename.endsWith(".css" )) return "text/css" ;
|
||||
else if (filename.endsWith(".zip" )) return "application/x-zip" ;
|
||||
else if (filename.endsWith(".gz" )) return "application/x-gzip" ;
|
||||
else if (filename.endsWith(".xls" )) return "application/msexcel" ;
|
||||
else if (filename.endsWith(".mp3" )) return "audio/mpeg" ;
|
||||
return "text/plain" ;
|
||||
}
|
||||
//--------------------------------------------------------------------------------------------------------------
|
||||
boolean WebSrv::handlehttp() { // HTTPserver, message received
|
||||
bool wswitch=true;
|
||||
int16_t inx0, inx1, inx2, inx3; // Pos. of search string in currenLine
|
||||
String currentLine = ""; // Build up to complete line
|
||||
String ct; // contentType
|
||||
uint32_t contentLength = 0; // contentLength
|
||||
uint8_t count = 0;
|
||||
|
||||
if (!cmdclient.connected()){
|
||||
log_e("cmdclient schould be connected but is not!");
|
||||
return false;
|
||||
}
|
||||
while (wswitch==true){ // first while
|
||||
if(!cmdclient.available()){
|
||||
log_e("Command client schould be available but is not!");
|
||||
return false;
|
||||
}
|
||||
currentLine = cmdclient.readStringUntil('\n');
|
||||
// log_i("currLine %s", currentLine.c_str());
|
||||
// If the current line is blank, you got two newline characters in a row.
|
||||
// that's the end of the client HTTP request, so send a response:
|
||||
if (currentLine.length() == 1) { // contains '\n' only
|
||||
wswitch=false; // use second while
|
||||
if (http_cmd.length()) {
|
||||
if(WEBSRV_onInfo) WEBSRV_onInfo(URLdecode(http_cmd).c_str());
|
||||
if(WEBSRV_onCommand) WEBSRV_onCommand(URLdecode(http_cmd), URLdecode(http_param), URLdecode(http_arg));
|
||||
}
|
||||
else if(http_rqfile.length()){
|
||||
if(WEBSRV_onInfo) WEBSRV_onInfo(URLdecode(http_rqfile).c_str());
|
||||
if(WEBSRV_onCommand) WEBSRV_onCommand(URLdecode(http_rqfile), URLdecode(http_param), URLdecode(http_arg));
|
||||
}
|
||||
else { // An empty "GET"?
|
||||
if(WEBSRV_onInfo) WEBSRV_onInfo("Filename is: index.html");
|
||||
if(WEBSRV_onCommand) WEBSRV_onCommand("index.html", URLdecode(http_param), URLdecode(http_arg));
|
||||
}
|
||||
currentLine = "";
|
||||
http_cmd = "";
|
||||
http_param = "";
|
||||
http_arg = "";
|
||||
http_rqfile = "";
|
||||
method = HTTP_NONE;
|
||||
break;
|
||||
} else {
|
||||
// Newline seen
|
||||
inx0 = 0;
|
||||
|
||||
if (currentLine.startsWith("Content-Length:")) contentLength = currentLine.substring(15).toInt();
|
||||
|
||||
if (currentLine.startsWith("GET /")) {method = HTTP_GET; inx0 = 5;} // GET request?
|
||||
if (currentLine.startsWith("POST /")){method = HTTP_PUT; inx0 = 6;} // POST request?
|
||||
if (inx0 == 0) method = HTTP_NONE;
|
||||
|
||||
if(inx0>0){
|
||||
inx1 = currentLine.indexOf("?"); // Search for 1st parameter
|
||||
inx2 = currentLine.lastIndexOf("&"); // Search for 2nd parameter
|
||||
inx3 = currentLine.indexOf(" HTTP");// Search for 3th parameter
|
||||
|
||||
if(inx1 > inx0){ // it is a command
|
||||
http_cmd = currentLine.substring(inx0, inx1);//isolate the command
|
||||
http_rqfile = ""; // No file
|
||||
}
|
||||
if((inx1>0) && (inx1+1 < inx3)){ // it is a parameter
|
||||
http_param = currentLine.substring(inx1+1, inx3);//isolate the parameter
|
||||
|
||||
if(inx2>0){
|
||||
http_arg = currentLine.substring(inx2+1, inx3);//isolate the arguments
|
||||
http_param = currentLine.substring(inx1+1, inx2);//cut the parameter
|
||||
}
|
||||
http_rqfile = ""; // No file
|
||||
}
|
||||
if(inx1 < 0 && inx2 < 0){ // it is a filename
|
||||
http_rqfile = currentLine.substring(inx0, inx3);
|
||||
http_cmd = "";
|
||||
http_param = "";
|
||||
http_arg = "";
|
||||
}
|
||||
}
|
||||
currentLine = "";
|
||||
}
|
||||
} //end first while
|
||||
while(wswitch==false){ // second while
|
||||
if(cmdclient.available()) {
|
||||
//log_i("%i", cmdclient.available());
|
||||
currentLine = cmdclient.readStringUntil('\n');
|
||||
//log_i("currLine %s", currentLine.c_str());
|
||||
contentLength -= currentLine.length();
|
||||
}
|
||||
else{
|
||||
currentLine = "";
|
||||
}
|
||||
if(!currentLine.length()){
|
||||
return true;
|
||||
}
|
||||
if((currentLine.length() == 1 && count == 0) || count >= 2){
|
||||
wswitch=true; // use first while
|
||||
currentLine = "";
|
||||
count = 0;
|
||||
break;
|
||||
}
|
||||
else{ // its the requestbody
|
||||
if(currentLine.length() > 1){
|
||||
if(WEBSRV_onRequest) WEBSRV_onRequest(currentLine, 0);
|
||||
if(WEBSRV_onInfo) WEBSRV_onInfo(currentLine.c_str());
|
||||
}
|
||||
|
||||
if(currentLine.startsWith("------")) {
|
||||
count++; // WebKitFormBoundary header
|
||||
contentLength -= (currentLine.length() + 2); // WebKitFormBoundary footer ist 2 chars longer
|
||||
}
|
||||
if(currentLine.length() == 1 && count == 1){
|
||||
contentLength -= 6; // "\r\n\r\n..."
|
||||
if(WEBSRV_onRequest) WEBSRV_onRequest("fileUpload", contentLength);
|
||||
count++;
|
||||
}
|
||||
}
|
||||
|
||||
} // end second while
|
||||
cmdclient.stop();
|
||||
return true;
|
||||
}
|
||||
//--------------------------------------------------------------------------------------------------------------
|
||||
boolean WebSrv::handleWS() { // Websocketserver, receive messages
|
||||
String currentLine = ""; // Build up to complete line
|
||||
|
||||
if (!webSocketClient.connected()){
|
||||
log_e("webSocketClient schould be connected but is not!");
|
||||
hasclient_WS = false;
|
||||
return false;
|
||||
}
|
||||
|
||||
if(!hasclient_WS){
|
||||
while(true){
|
||||
currentLine = webSocketClient.readStringUntil('\n');
|
||||
|
||||
if (currentLine.length() == 1) { // contains '\n' only
|
||||
if(ws_conn_request_flag){
|
||||
ws_conn_request_flag = false;
|
||||
printWebSocketHeader(WS_resp_Key);
|
||||
hasclient_WS = true;
|
||||
}
|
||||
break;
|
||||
}
|
||||
|
||||
if (currentLine.startsWith("Sec-WebSocket-Key:")) { // Websocket connection request
|
||||
WS_sec_Key = currentLine.substring(18);
|
||||
WS_sec_Key.trim();
|
||||
WS_resp_Key = calculateWebSocketResponseKey(WS_sec_Key);
|
||||
ws_conn_request_flag = true;
|
||||
}
|
||||
}
|
||||
}
|
||||
int av = webSocketClient.available();
|
||||
|
||||
if(av){
|
||||
parseWsMessage(av);
|
||||
}
|
||||
return true;
|
||||
}
|
||||
//--------------------------------------------------------------------------------------------------------------
|
||||
void WebSrv::parseWsMessage(uint32_t len){
|
||||
uint8_t headerLen = 2;
|
||||
uint16_t paylodLen;
|
||||
uint8_t maskingKey[4];
|
||||
|
||||
if(len > UINT16_MAX){
|
||||
log_e("Websocketmessage too long");
|
||||
return;
|
||||
}
|
||||
|
||||
webSocketClient.readBytes(buff, 1);
|
||||
uint8_t fin = ((buff[0] >> 7) & 0x01); (void)fin;
|
||||
uint8_t rsv1 = ((buff[0] >> 6) & 0x01); (void)rsv1;
|
||||
uint8_t rsv2 = ((buff[0] >> 5) & 0x01); (void)rsv2;
|
||||
uint8_t rsv3 = ((buff[0] >> 4) & 0x01); (void)rsv3;
|
||||
uint8_t opcode = (buff[0] & 0x0F);
|
||||
|
||||
webSocketClient.readBytes(buff, 1);
|
||||
uint8_t mask = ((buff[0]>>7) & 0x01);
|
||||
paylodLen = (buff[0] & 0x7F);
|
||||
|
||||
if(paylodLen == 126){
|
||||
headerLen = 4;
|
||||
webSocketClient.readBytes(buff, 2);
|
||||
paylodLen = buff[0] << 8;
|
||||
paylodLen += buff[1];
|
||||
|
||||
}
|
||||
|
||||
(void)headerLen;
|
||||
|
||||
if(mask){
|
||||
maskingKey[0] = webSocketClient.read();
|
||||
maskingKey[1] = webSocketClient.read();
|
||||
maskingKey[2] = webSocketClient.read();
|
||||
maskingKey[3] = webSocketClient.read();
|
||||
}
|
||||
|
||||
if(opcode == 0x08) { // denotes a connection close
|
||||
hasclient_WS = false;
|
||||
webSocketClient.stop();
|
||||
return;
|
||||
}
|
||||
|
||||
if(opcode == 0x09) { // denotes a ping
|
||||
if(WEBSRV_onCommand) WEBSRV_onCommand("ping received, send pong", "", "");
|
||||
if(WEBSRV_onInfo) WEBSRV_onInfo("pong received, send pong");
|
||||
sendPong();
|
||||
}
|
||||
|
||||
if(opcode == 0x0A) { // denotes a pong
|
||||
if(WEBSRV_onCommand) WEBSRV_onCommand("pong received", "", "");
|
||||
if(WEBSRV_onInfo) WEBSRV_onInfo("pong received");
|
||||
return;
|
||||
}
|
||||
|
||||
if(opcode == 0x01) { // denotes a text frame
|
||||
int plen;
|
||||
while(paylodLen){
|
||||
if(paylodLen > 255){
|
||||
plen = 255;
|
||||
paylodLen -= webSocketClient.readBytes(buff, plen);
|
||||
}
|
||||
else{
|
||||
plen = paylodLen;
|
||||
paylodLen = 0;
|
||||
webSocketClient.readBytes(buff, plen);
|
||||
}
|
||||
if(mask){
|
||||
for(int i = 0; i < plen; i++){
|
||||
buff[i] = (buff[i] ^ maskingKey[i % 4]);
|
||||
}
|
||||
}
|
||||
buff[plen] = 0;
|
||||
if(WEBSRV_onInfo) WEBSRV_onInfo(buff);
|
||||
if(len < 256){ // can be a command like "mute=1"
|
||||
char *ret;
|
||||
ret = strchr((const char*)buff, '=');
|
||||
if(ret){
|
||||
*ret = 0;
|
||||
// log_i("cmd=%s, para=%s", buff, ret);
|
||||
if(WEBSRV_onCommand) WEBSRV_onCommand((const char*) buff, ret + 1, "");
|
||||
buff[0] = 0;
|
||||
return;
|
||||
}
|
||||
}
|
||||
if(WEBSRV_onCommand) WEBSRV_onCommand((const char*) buff, "", "");
|
||||
}
|
||||
buff[0] = 0;
|
||||
}
|
||||
}
|
||||
//--------------------------------------------------------------------------------------------------------------
|
||||
boolean WebSrv::loop() {
|
||||
|
||||
cmdclient = cmdserver.available();
|
||||
if (cmdclient.available()){ // Check Input from client?
|
||||
if(WEBSRV_onInfo) WEBSRV_onInfo("Command client available");
|
||||
return handlehttp();
|
||||
}
|
||||
|
||||
if(!webSocketClient.connected()){
|
||||
hasclient_WS = false;
|
||||
}
|
||||
if(!hasclient_WS) webSocketClient = webSocketServer.available();
|
||||
if (webSocketClient.available()){
|
||||
if(WEBSRV_onInfo) WEBSRV_onInfo("WebSocket client available");
|
||||
return handleWS();
|
||||
}
|
||||
|
||||
return false;
|
||||
}
|
||||
//--------------------------------------------------------------------------------------------------------------
|
||||
void WebSrv::reply(const String &response, bool header){
|
||||
if(header==true) {
|
||||
int l= response.length();
|
||||
// HTTP header
|
||||
String httpheader="";
|
||||
httpheader += "HTTP/1.1 200 OK\r\n";
|
||||
httpheader += "Connection: close\r\n";
|
||||
httpheader += "Content-type: text/html\r\n";
|
||||
httpheader += "Content-Length: " + String(l, 10) + "\r\n";
|
||||
httpheader += "Server: " + _Name+ "\r\n";
|
||||
httpheader += "Cache-Control: max-age=3600\r\n";
|
||||
httpheader += "Last-Modified: " + _Version + "\r\n\r\n";
|
||||
|
||||
cmdclient.print(httpheader) ; // header sent
|
||||
}
|
||||
cmdclient.print(response);
|
||||
}
|
||||
//--------------------------------------------------------------------------------------------------------------
|
||||
String WebSrv::UTF8toASCII(String str){
|
||||
uint16_t i=0;
|
||||
String res="";
|
||||
char tab[96]={
|
||||
96,173,155,156, 32,157, 32, 32, 32, 32,166,174,170, 32, 32, 32,248,241,253, 32,
|
||||
32,230, 32,250, 32, 32,167,175,172,171, 32,168, 32, 32, 32, 32,142,143,146,128,
|
||||
32,144, 32, 32, 32, 32, 32, 32, 32,165, 32, 32, 32, 32,153, 32, 32, 32, 32, 32,
|
||||
154, 32, 32,225,133,160,131, 32,132,134,145,135,138,130,136,137,141,161,140,139,
|
||||
32,164,149,162,147, 32,148,246, 32,151,163,150,129, 32, 32,152
|
||||
};
|
||||
while(str[i]!=0){
|
||||
if(str[i]==0xC2){ // compute unicode from utf8
|
||||
i++;
|
||||
if((str[i]>159)&&(str[i]<192)) res+=char(tab[str[i]-160]);
|
||||
else res+=char(32);
|
||||
}
|
||||
else if(str[i]==0xC3){
|
||||
i++;
|
||||
if((str[i]>127)&&(str[i]<192)) res+=char(tab[str[i]-96]);
|
||||
else res+=char(32);
|
||||
}
|
||||
else res+=str[i];
|
||||
i++;
|
||||
}
|
||||
return res;
|
||||
}
|
||||
//--------------------------------------------------------------------------------------------------------------
|
||||
String WebSrv::URLdecode(String str){
|
||||
String hex="0123456789ABCDEF";
|
||||
String res="";
|
||||
uint16_t i=0;
|
||||
while(str[i]!=0){
|
||||
if((str[i]=='%') && isHexadecimalDigit(str[i+1]) && isHexadecimalDigit(str[i+2])){
|
||||
res+=char((hex.indexOf(str[i+1])<<4) + hex.indexOf(str[i+2])); i+=3;}
|
||||
else{res+=str[i]; i++;}
|
||||
}
|
||||
return res;
|
||||
}
|
||||
//--------------------------------------------------------------------------------------------------------------
|
||||
String WebSrv::responseCodeToString(int code) {
|
||||
switch (code) {
|
||||
case 100: return F("Continue");
|
||||
case 101: return F("Switching Protocols");
|
||||
case 200: return F("OK");
|
||||
case 201: return F("Created");
|
||||
case 202: return F("Accepted");
|
||||
case 203: return F("Non-Authoritative Information");
|
||||
case 204: return F("No Content");
|
||||
case 205: return F("Reset Content");
|
||||
case 206: return F("Partial Content");
|
||||
case 300: return F("Multiple Choices");
|
||||
case 301: return F("Moved Permanently");
|
||||
case 302: return F("Found");
|
||||
case 303: return F("See Other");
|
||||
case 304: return F("Not Modified");
|
||||
case 305: return F("Use Proxy");
|
||||
case 307: return F("Temporary Redirect");
|
||||
case 400: return F("Bad Request");
|
||||
case 401: return F("Unauthorized");
|
||||
case 402: return F("Payment Required");
|
||||
case 403: return F("Forbidden");
|
||||
case 404: return F("Not Found");
|
||||
case 405: return F("Method Not Allowed");
|
||||
case 406: return F("Not Acceptable");
|
||||
case 407: return F("Proxy Authentication Required");
|
||||
case 408: return F("Request Time-out");
|
||||
case 409: return F("Conflict");
|
||||
case 410: return F("Gone");
|
||||
case 411: return F("Length Required");
|
||||
case 412: return F("Precondition Failed");
|
||||
case 413: return F("Request Entity Too Large");
|
||||
case 414: return F("Request-URI Too Large");
|
||||
case 415: return F("Unsupported Media Type");
|
||||
case 416: return F("Requested range not satisfiable");
|
||||
case 417: return F("Expectation Failed");
|
||||
case 500: return F("Internal Server Error");
|
||||
case 501: return F("Not Implemented");
|
||||
case 502: return F("Bad Gateway");
|
||||
case 503: return F("Service Unavailable");
|
||||
case 504: return F("Gateway Time-out");
|
||||
case 505: return F("HTTP Version not supported");
|
||||
default: return "";
|
||||
}
|
||||
}
|
||||
//--------------------------------------------------------------------------------------------------------------
|
96
lib/ESP32-audioI2S-3.0.0/examples/DLNA/lib/websrv/websrv.h
Normal file
@ -0,0 +1,96 @@
|
||||
/*
|
||||
* websrv.h
|
||||
*
|
||||
* Created on: 09.07.2017
|
||||
* updated on: 11.04.2022
|
||||
* Author: Wolle
|
||||
*/
|
||||
|
||||
#ifndef WEBSRV_H_
|
||||
#define WEBSRV_H_
|
||||
#include "Arduino.h"
|
||||
#include "WiFi.h"
|
||||
#include "SD.h"
|
||||
#include "FS.h"
|
||||
#include "mbedtls/sha1.h"
|
||||
#include "base64.h"
|
||||
|
||||
extern __attribute__((weak)) void WEBSRV_onInfo(const char*);
|
||||
extern __attribute__((weak)) void WEBSRV_onCommand(const String cmd, const String param, const String arg);
|
||||
extern __attribute__((weak)) void WEBSRV_onRequest(const String, uint32_t contentLength);
|
||||
|
||||
|
||||
|
||||
class WebSrv
|
||||
{
|
||||
protected:
|
||||
WiFiClient cmdclient; // An instance of the client for commands
|
||||
WiFiClient webSocketClient ;
|
||||
WiFiServer cmdserver;
|
||||
WiFiServer webSocketServer;
|
||||
|
||||
private:
|
||||
bool http_reponse_flag = false ; // Response required
|
||||
bool ws_conn_request_flag = false; // websocket connection attempt
|
||||
bool hasclient_WS = false;
|
||||
String http_rqfile ; // Requested file
|
||||
String http_cmd ; // Content of command
|
||||
String http_param; // Content of parameter
|
||||
String http_arg; // Content of argument
|
||||
String _Name;
|
||||
String _Version;
|
||||
String contenttype;
|
||||
char buff[256];
|
||||
uint8_t method;
|
||||
String WS_sec_Key;
|
||||
String WS_resp_Key;
|
||||
String WS_sec_conKey = "258EAFA5-E914-47DA-95CA-C5AB0DC85B11";
|
||||
|
||||
protected:
|
||||
String calculateWebSocketResponseKey(String sec_WS_key);
|
||||
void printWebSocketHeader(String wsRespKey);
|
||||
String getContentType(String filename);
|
||||
boolean handlehttp();
|
||||
boolean handleWS();
|
||||
void parseWsMessage(uint32_t len);
|
||||
uint8_t inbyte();
|
||||
String URLdecode(String str);
|
||||
String UTF8toASCII(String str);
|
||||
String responseCodeToString(int code);
|
||||
|
||||
|
||||
public:
|
||||
enum { HTTP_NONE = 0, HTTP_GET = 1, HTTP_PUT = 2 };
|
||||
enum { Continuation_Frame = 0x00, Text_Frame = 0x01, Binary_Frame = 0x02, Connection_Close_Frame = 0x08,
|
||||
Ping_Frame = 0x09, Pong_Frame = 0x0A };
|
||||
WebSrv(String Name="WebSrv library", String Version="1.0");
|
||||
void begin(uint16_t http_port = 80, uint16_t websocket_port = 81);
|
||||
void stop();
|
||||
boolean loop();
|
||||
void show(const char* pagename, int16_t len=-1);
|
||||
void show_not_found();
|
||||
boolean streamfile(fs::FS &fs,const char* path);
|
||||
boolean send(String msg, uint8_t opcode = Text_Frame);
|
||||
boolean send(const char* msg, uint8_t opcode = Text_Frame);
|
||||
void sendPing();
|
||||
void sendPong();
|
||||
boolean uploadfile(fs::FS &fs,const char* path, uint32_t contentLength);
|
||||
boolean uploadB64image(fs::FS &fs,const char* path, uint32_t contentLength);
|
||||
void reply(const String &response, boolean header=true);
|
||||
const char* ASCIItoUTF8(const char* str);
|
||||
|
||||
private:
|
||||
const int B64index[123] ={
|
||||
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
|
||||
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
|
||||
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 62, 63, 62, 62, 63,
|
||||
52, 53, 54, 55, 56, 57, 58, 59, 60, 61, 0, 0, 0, 0, 0, 0,
|
||||
0, 0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14,
|
||||
15, 16, 17, 18, 19, 20, 21, 22, 23, 24, 25, 0, 0, 0, 0, 63,
|
||||
0, 26, 27, 28, 29, 30, 31, 32, 33, 34, 35, 36, 37, 38, 39, 40,
|
||||
41, 42, 43, 44, 45, 46, 47, 48, 49, 50, 51
|
||||
};
|
||||
};
|
||||
|
||||
|
||||
#endif /* WEBSRV_H_ */
|
4
lib/ESP32-audioI2S-3.0.0/examples/DLNA/partitions.csv
Normal file
@ -0,0 +1,4 @@
|
||||
# Name, Type, SubType, Offset, Size
|
||||
nvs, data, nvs, 0x9000, 0x4000
|
||||
phy_init, data, phy, 0xd000, 0x1000
|
||||
factory, app, factory, 0x10000, 3M,
|
|
61
lib/ESP32-audioI2S-3.0.0/examples/DLNA/platformio.ini
Normal file
@ -0,0 +1,61 @@
|
||||
; PlatformIO Project Configuration File
|
||||
;
|
||||
; Build options: build flags, source filter
|
||||
; Upload options: custom upload port, speed and extra flags
|
||||
; Library options: dependencies, extra library storages
|
||||
; Advanced options: extra scripting
|
||||
;
|
||||
; Please visit documentation for the other options and examples
|
||||
; https://docs.platformio.org/en/latest/platforms/espressif32.html
|
||||
|
||||
[env:esp32dev]
|
||||
platform = https://github.com/platformio/platform-espressif32.git#v5.2.0 ; Arduino V2.0.5
|
||||
|
||||
board = esp32dev ;chipmodel ESP32, 4M FLASH, USBtoTTL
|
||||
|
||||
board_build.f_cpu = 240000000L
|
||||
board_build.flash_size=4MB
|
||||
board_build.flash_freq=80M
|
||||
board_build.spiram_mode=2
|
||||
framework = arduino
|
||||
monitor_speed = 115200
|
||||
monitor_filters = esp32_exception_decoder
|
||||
board_build.partitions = default.csv
|
||||
upload_speed = 460800 ; 921600, 512000, 460800, 256000, 115200
|
||||
|
||||
lib_deps =
|
||||
https://github.com/schreibfaul1/ESP32-audioI2S.git#2.0.6
|
||||
https://github.com/yellobyte/SoapESP32.git#1.1.4
|
||||
https://github.com/arduino-libraries/Arduino_JSON.git
|
||||
|
||||
platform_packages =
|
||||
|
||||
framework-arduinoespressif32 @ https://github.com/espressif/arduino-esp32.git#2.0.5
|
||||
|
||||
board_upload.maximum_size = 3145728
|
||||
board_upload.flash_size = 4MB
|
||||
board_build.flash_mode = qio
|
||||
board_build.bootloader = dio
|
||||
|
||||
;build_flags = -DCORE_DEBUG_LEVEL=0 ; None
|
||||
;build_flags = -DCORE_DEBUG_LEVEL=1 ; Error
|
||||
;build_flags = -DCORE_DEBUG_LEVEL=2 ; Warn
|
||||
;build_flags = -DCORE_DEBUG_LEVEL=3 ; Info
|
||||
;build_flags = -DCORE_DEBUG_LEVEL=4 ; Debug
|
||||
;build_flags = -DCORE_DEBUG_LEVEL=5 ; Verbose
|
||||
|
||||
build_flags =
|
||||
; -Wall
|
||||
; -Wextra
|
||||
-Wdouble-promotion ; double to float warnings
|
||||
-Wimplicit-fallthrough ; switch case without break
|
||||
-DCORE_DEBUG_LEVEL=3
|
||||
-DCONFIG_ARDUHAL_LOG_COLORS
|
||||
-DBOARD_HAS_PSRAM
|
||||
-DARDUINO_RUNNING_CORE=3 ; Arduino Runs On Core (setup, loop)
|
||||
-DARDUINO_EVENT_RUNNING_CORE=1 ; Events Run On Core
|
||||
; -DAUDIO_LOG
|
||||
|
||||
build_unflags =
|
||||
; -DARDUINO_USB_CDC_ON_BOOT=0 ; traditional log
|
||||
; -DBOARD_HAS_PSRAM
|
5
lib/ESP32-audioI2S-3.0.0/examples/DLNA/readme.md
Normal file
@ -0,0 +1,5 @@
|
||||
# DLNA
|
||||
DLNA servers are available in many home networks. Many Internet routers (e.g. Fritzbox) have an integrated DLNA service. It is also easy to set up a own DLNA server (e.g. miniDLNA on a Raspberry Pi). The SoapESP32 library https://github.com/yellobyte/SoapESP32 used here automatically recognizes the DLNA servers available in the home network. Thanks to yellobyte for this library. Since the SW is too extensive for a sketch, I have published a complete project here. Simply download the repository and unzip the DLNA folder. You can open the project with PlatformIO. Change the access data and, if necessary, the GPIOs in main.cpp. If a DLNA server was detected, its content will be displayed in the browser. If an audio file is selected, the playback process begins using the audioI2S library.
|
||||
<br>
|
||||
Webpage
|
||||

|
287
lib/ESP32-audioI2S-3.0.0/examples/DLNA/src/index.h
Normal file
@ -0,0 +1,287 @@
|
||||
/*
|
||||
* index.h
|
||||
*
|
||||
* Created on: 13.12.2022
|
||||
* Updated on: 20.12.2022
|
||||
* Author: Wolle
|
||||
*
|
||||
* ESP32 - DLNA
|
||||
*
|
||||
*/
|
||||
|
||||
#ifndef INDEX_H_
|
||||
#define INDEX_H_
|
||||
|
||||
#include "Arduino.h"
|
||||
|
||||
// file in raw data format for PROGMEM
|
||||
|
||||
const char index_html[] PROGMEM = R"=====(
|
||||
<!DOCTYPE HTML>
|
||||
<html>
|
||||
<head>
|
||||
<title>ESP32 - DLNA</title>
|
||||
<style type="text/css"> /* optimized with csstidy */
|
||||
html { /* This is the groundplane */
|
||||
font-family : serif;
|
||||
height : 100%;
|
||||
font-size: 16px;
|
||||
color : DarkSlateGray;
|
||||
background-color : navy;
|
||||
margin : 0;
|
||||
padding : 0;
|
||||
}
|
||||
#content {
|
||||
min-height : 540px;
|
||||
min-width : 725px;
|
||||
overflow : hidden;
|
||||
background-color : lightskyblue;
|
||||
margin : 0;
|
||||
padding : 5px;
|
||||
}
|
||||
#tab-content1 {
|
||||
margin : 20px;
|
||||
}
|
||||
.boxstyle {
|
||||
height : 36px;
|
||||
padding-top : 0;
|
||||
padding-left : 15px;
|
||||
padding-bottom : 0;
|
||||
background-color: white;
|
||||
font-size : 16px;
|
||||
line-height : normal;
|
||||
border-color: black;
|
||||
border-style: solid;
|
||||
border-width: thin;
|
||||
border-radius : 5px;
|
||||
}
|
||||
#BODY { display:block; }
|
||||
</style>
|
||||
</head>
|
||||
<script>
|
||||
// global variables and functions
|
||||
|
||||
// ---- websocket section------------------------
|
||||
|
||||
var socket = undefined
|
||||
var host = location.hostname
|
||||
var tm
|
||||
|
||||
function ping() {
|
||||
if (socket.readyState == 1) { // reayState 'open'
|
||||
socket.send("ping")
|
||||
console.log("send ping")
|
||||
tm = setTimeout(function () {
|
||||
console.log('The connection to the ESP32 is interrupted! Please reload the page!')
|
||||
}, 10000)
|
||||
}
|
||||
}
|
||||
|
||||
function connect() {
|
||||
socket = new WebSocket('ws://'+window.location.hostname+':81/');
|
||||
|
||||
socket.onopen = function () {
|
||||
console.log("Websocket connected")
|
||||
socket.send('DLNA_getServer')
|
||||
setInterval(ping, 20000)
|
||||
};
|
||||
|
||||
socket.onclose = function (e) {
|
||||
console.log(e)
|
||||
console.log('Socket is closed. Reconnect will be attempted in 1 second.', e)
|
||||
socket = null
|
||||
setTimeout(function () {
|
||||
connect()
|
||||
}, 1000)
|
||||
}
|
||||
|
||||
socket.onerror = function (err) {
|
||||
console.log(err)
|
||||
}
|
||||
|
||||
socket.onmessage = function(event) {
|
||||
var socketMsg = event.data
|
||||
var n = socketMsg.indexOf('=')
|
||||
var msg = ''
|
||||
var val = ''
|
||||
if (n >= 0) {
|
||||
var msg = socketMsg.substring(0, n)
|
||||
var val = socketMsg.substring(n + 1)
|
||||
// console.log("para ",msg, " val ",val)
|
||||
}
|
||||
else {
|
||||
msg = socketMsg
|
||||
}
|
||||
|
||||
switch(msg) {
|
||||
case "pong": clearTimeout(tm)
|
||||
break
|
||||
case "DLNA_Names": showServer(val)
|
||||
break
|
||||
case "Level1": show_DLNA_Content(val, 1)
|
||||
break
|
||||
case "Level2": show_DLNA_Content(val, 2)
|
||||
break
|
||||
case "Level3": show_DLNA_Content(val, 3)
|
||||
break
|
||||
case "Level4": show_DLNA_Content(val, 4)
|
||||
break
|
||||
case "Level5": show_DLNA_Content(val, 5)
|
||||
break
|
||||
default: console.log('unknown message', msg, val)
|
||||
}
|
||||
}
|
||||
}
|
||||
// ---- end websocket section------------------------
|
||||
|
||||
document.addEventListener('readystatechange', event => {
|
||||
if (event.target.readyState === 'interactive') { // same as: document.addEventListener('DOMContentLoaded'...
|
||||
// same as jQuery.ready
|
||||
console.log('All HTML DOM elements are accessible')
|
||||
// document.getElementById('dialog').style.display = 'none' // hide the div (its only a template)
|
||||
|
||||
}
|
||||
if (event.target.readyState === 'complete') {
|
||||
console.log('Now external resources are loaded too, like css,src etc... ')
|
||||
connect(); // establish websocket connection
|
||||
}
|
||||
})
|
||||
|
||||
function showServer(val){
|
||||
console.log(val)
|
||||
var select = document.getElementById('server')
|
||||
select.options.length = 0;
|
||||
var server = val.split(",")
|
||||
for (i = -1; i < (server.length); i++) {
|
||||
opt = document.createElement('OPTION')
|
||||
if(i == -1){
|
||||
opt.value = ""
|
||||
opt.text = "Select a DLNA Server here"
|
||||
}
|
||||
else{
|
||||
console.log(server[i])
|
||||
opt.value = server[i]
|
||||
opt.text = server[i]
|
||||
}
|
||||
select.add(opt)
|
||||
}
|
||||
}
|
||||
|
||||
function show_DLNA_Content(val, level){
|
||||
var select
|
||||
if(level == 1) select = document.getElementById('level1')
|
||||
if(level == 2) select = document.getElementById('level2')
|
||||
if(level == 3) select = document.getElementById('level3')
|
||||
if(level == 4) select = document.getElementById('level4')
|
||||
if(level == 5) select = document.getElementById('level5')
|
||||
content =JSON.parse(val)
|
||||
//console.log(ct[1].name)
|
||||
select.options.length = 0;
|
||||
for (i = -1; i < (content.length); i++) {
|
||||
opt = document.createElement('OPTION')
|
||||
if(i == -1){
|
||||
opt.value = ""
|
||||
opt.text = "Select level " + level.toString()
|
||||
}
|
||||
else{
|
||||
var n
|
||||
var c
|
||||
if(content[i].isDir == true){
|
||||
n = content[i].name.concat('\xa0\xa0', '<DIR>'); // more than one space
|
||||
c = 'D=' + content[i].id // is directory
|
||||
}
|
||||
else{
|
||||
n = content[i].name + '\xa0\xa0' + content[i].size;
|
||||
c = 'F=' + content[i].id // is file
|
||||
}
|
||||
opt.value = c
|
||||
opt.text = n
|
||||
}
|
||||
select.add(opt)
|
||||
}
|
||||
}
|
||||
|
||||
function selectserver (presctrl) { // preset, select a server, root, level0
|
||||
socket.send('DLNA_getContent0=' + presctrl.value)
|
||||
select = document.getElementById('level1'); select.options.length = 0; // clear next level
|
||||
select = document.getElementById('level2'); select.options.length = 0;
|
||||
select = document.getElementById('level3'); select.options.length = 0;
|
||||
select = document.getElementById('level4'); select.options.length = 0;
|
||||
select = document.getElementById('level5'); select.options.length = 0;
|
||||
console.log('DLNA_getContent0=' + presctrl.value)
|
||||
}
|
||||
|
||||
function select_l1 (presctrl) { // preset, select root
|
||||
socket.send('DLNA_getContent1=' + presctrl.value)
|
||||
select = document.getElementById('level2'); select.options.length = 0; // clear next level
|
||||
select = document.getElementById('level3'); select.options.length = 0;
|
||||
select = document.getElementById('level4'); select.options.length = 0;
|
||||
select = document.getElementById('level5'); select.options.length = 0;
|
||||
console.log('DLNA_getContent1=' + presctrl.value)
|
||||
}
|
||||
|
||||
function select_l2 (presctrl) { // preset, select level 1
|
||||
socket.send('DLNA_getContent2=' + presctrl.value)
|
||||
select = document.getElementById('level3'); select.options.length = 0;
|
||||
select = document.getElementById('level4'); select.options.length = 0;
|
||||
select = document.getElementById('level5'); select.options.length = 0;
|
||||
console.log('DLNA_getContent2=' + presctrl.value)
|
||||
}
|
||||
|
||||
function select_l3 (presctrl) { // preset, select level 2
|
||||
socket.send('DLNA_getContent3=' + presctrl.value)
|
||||
select = document.getElementById('level4'); select.options.length = 0;
|
||||
select = document.getElementById('level5'); select.options.length = 0;
|
||||
console.log('DLNA_getContent3=' + presctrl.value)
|
||||
}
|
||||
|
||||
function select_l4 (presctrl) { // preset, select level 3
|
||||
socket.send('DLNA_getContent4=' + presctrl.value)
|
||||
select = document.getElementById('level5'); select.options.length = 0;
|
||||
console.log('DLNA_getContent4=' + presctrl.value)
|
||||
}
|
||||
|
||||
function select_l5 (presctrl) { // preset, select level 4
|
||||
socket.send('DLNA_getContent5=' + presctrl.value)
|
||||
console.log('DLNA_getContent5=' + presctrl.value)
|
||||
}
|
||||
|
||||
</script>
|
||||
<body id="BODY">
|
||||
<!--==============================================================================================-->
|
||||
<div id="content">
|
||||
<div id="content1">
|
||||
<div style="font-size: 50px; text-align: center; flex: 1;">
|
||||
ESP32 - DLNA
|
||||
</div>
|
||||
<div style="display: flex;">
|
||||
<div style="flex: 0 0 calc(100% - 0px);">
|
||||
<select class="boxstyle" style="width: 100%;" onchange="selectserver(this)" id="server">
|
||||
<option value="-1">Select a DLNA Server here</option>
|
||||
</select>
|
||||
<select class="boxstyle" style="width: 100%; margin-top: 5px;" onchange="select_l1(this)" id="level1">
|
||||
<option value="-1"> </option>
|
||||
</select>
|
||||
<select class="boxstyle" style="width: 100%; margin-top: 5px;" onchange="select_l2(this)" id="level2">
|
||||
<option value="-1"> </option>
|
||||
</select>
|
||||
<select class="boxstyle" style="width: 100%; margin-top: 5px;" onchange="select_l3(this)" id="level3">
|
||||
<option value="-1"> </option>
|
||||
</select>
|
||||
<select class="boxstyle" style="width: 100%; margin-top: 5px;" onchange="select_l4(this)" id="level4">
|
||||
<option value="-1"> </option>
|
||||
</select>
|
||||
<select class="boxstyle" style="width: 100%; margin-top: 5px;" onchange="select_l5(this)" id="level5">
|
||||
<option value="-1"> </option>
|
||||
</select>
|
||||
</div>
|
||||
</div>
|
||||
<hr>
|
||||
</div>
|
||||
</div>
|
||||
<!--==============================================================================================-->
|
||||
</body>
|
||||
</html>
|
||||
|
||||
)=====";
|
||||
#endif /* INDEX_H_ */
|
188
lib/ESP32-audioI2S-3.0.0/examples/DLNA/src/main.cpp
Normal file
@ -0,0 +1,188 @@
|
||||
#include <Arduino.h>
|
||||
#include <WiFi.h>
|
||||
#include "websrv.h"
|
||||
#include "index.h"
|
||||
#include "Audio.h"
|
||||
#include "SoapESP32.h"
|
||||
#include "Arduino_JSON.h"
|
||||
#include <vector>
|
||||
|
||||
using namespace std;
|
||||
|
||||
#define I2S_DOUT 25
|
||||
#define I2S_BCLK 27
|
||||
#define I2S_LRC 26
|
||||
|
||||
|
||||
char SSID[] = "Wolles-FRITZBOX";
|
||||
char PASS[] = "40441061073895958449";
|
||||
|
||||
|
||||
|
||||
WebSrv webSrv;
|
||||
WiFiClient client;
|
||||
WiFiUDP udp;
|
||||
SoapESP32 soap(&client, &udp);
|
||||
Audio audio;
|
||||
|
||||
uint numServers = 0;
|
||||
int currentServer = -1;
|
||||
uint32_t media_downloadPort = 0;
|
||||
String media_downloadIP = "";
|
||||
vector<String> names{};
|
||||
//----------------------------------------------------------------------------------------------------------------------
|
||||
int DLNA_setCurrentServer(String serverName){
|
||||
int serverNum = -1;
|
||||
for(int i = 0; i < names.size(); i++){
|
||||
if(names[i] == serverName) serverNum = i;
|
||||
}
|
||||
currentServer = serverNum;
|
||||
return serverNum;
|
||||
}
|
||||
void DLNA_showServer(){ // Show connection details of all discovered, usable media servers
|
||||
String msg = "DLNA_Names=";
|
||||
soapServer_t srv;
|
||||
names.clear();
|
||||
for(int i = 0; i < numServers; i++){
|
||||
soap.getServerInfo(i, &srv);
|
||||
Serial.printf("Server[%d]: IP address: %s port: %d name: %s -> controlURL: %s\n",
|
||||
i, srv.ip.toString().c_str(), srv.port, srv.friendlyName.c_str(), srv.controlURL.c_str());
|
||||
msg += srv.friendlyName;
|
||||
if(i < numServers - 1) msg += ',';
|
||||
names.push_back(srv.friendlyName);
|
||||
}
|
||||
log_i("msg %s", msg.c_str());
|
||||
webSrv.send(msg);
|
||||
}
|
||||
void DLNA_browseServer(String objectId, uint8_t level){
|
||||
JSONVar myObject;
|
||||
soapObjectVect_t browseResult;
|
||||
soapObject_t object;
|
||||
|
||||
// Here the user selects the DLNA server whose content he wants to see, level 0 is root
|
||||
if(level == 0){
|
||||
if(DLNA_setCurrentServer(objectId) < 0) {log_e("DLNA Server not found"); return;}
|
||||
objectId = "0";
|
||||
}
|
||||
|
||||
soap.browseServer(currentServer, objectId.c_str(), &browseResult);
|
||||
if(browseResult.size() == 0){
|
||||
log_i("no content!"); // then the directory is empty
|
||||
return;
|
||||
}
|
||||
log_v("objectID: %s", objectId.c_str());
|
||||
for (int i = 0; i < browseResult.size(); i++){
|
||||
object = browseResult[i];
|
||||
myObject[i]["name"]= object.name;
|
||||
myObject[i]["isDir"] = object.isDirectory;
|
||||
if(object.isDirectory){
|
||||
myObject[i]["id"] = object.id;
|
||||
}
|
||||
else {
|
||||
myObject[i]["id"] = object.uri;
|
||||
media_downloadPort = object.downloadPort;
|
||||
media_downloadIP = object.downloadIp.toString();
|
||||
}
|
||||
myObject[i]["size"] = (uint32_t)object.size;
|
||||
myObject[i]["uri"] = object.id;
|
||||
log_v("objectName %s", browseResult[i].name.c_str());
|
||||
log_v("objectId %s", browseResult[i].artist.c_str());
|
||||
}
|
||||
level++;
|
||||
String msg = "Level" + String(level,10) + "=" + JSON.stringify(myObject);
|
||||
|
||||
log_v("msg = %s", msg.c_str());
|
||||
webSrv.send(msg);
|
||||
browseResult.clear();
|
||||
}
|
||||
|
||||
void DLNA_getFileItems(String uri){
|
||||
soapObjectVect_t browseResult;
|
||||
|
||||
log_v("uri: %s", uri.c_str());
|
||||
log_v("downloadIP: %s", media_downloadIP.c_str());
|
||||
log_v("downloadport: %d", media_downloadPort);
|
||||
String URL = "http://" + media_downloadIP + ":" + media_downloadPort + "/" + uri;
|
||||
log_i("URL=%s", URL.c_str());
|
||||
audio.connecttohost(URL.c_str());
|
||||
}
|
||||
void DLNA_showContent(String objectId, uint8_t level){
|
||||
log_v("obkId=%s", objectId.c_str());
|
||||
if(level == 0){
|
||||
DLNA_browseServer(objectId, level);
|
||||
}
|
||||
if(objectId.startsWith("D=")) {
|
||||
objectId = objectId.substring(2);
|
||||
DLNA_browseServer(objectId, level);
|
||||
}
|
||||
if(objectId.startsWith("F=")) {
|
||||
objectId = objectId.substring(2);
|
||||
DLNA_getFileItems(objectId);
|
||||
}
|
||||
}
|
||||
|
||||
//----------------------------------------------------------------------------------------------------------------------
|
||||
// S E T U P
|
||||
//----------------------------------------------------------------------------------------------------------------------
|
||||
void setup() {
|
||||
Serial.begin(115200);
|
||||
WiFi.mode(WIFI_STA);
|
||||
WiFi.begin(SSID, PASS);
|
||||
while (WiFi.status() != WL_CONNECTED) delay(1500);
|
||||
log_i("connected, IP=%s", WiFi.localIP().toString().c_str());
|
||||
webSrv.begin(80, 81); // HTTP port, WebSocket port
|
||||
|
||||
soap.seekServer();
|
||||
numServers = soap.getServerCount();
|
||||
audio.setPinout(I2S_BCLK, I2S_LRC, I2S_DOUT);
|
||||
audio.setVolume(21); // 0...21
|
||||
}
|
||||
|
||||
//----------------------------------------------------------------------------------------------------------------------
|
||||
// L O O P
|
||||
//----------------------------------------------------------------------------------------------------------------------
|
||||
void loop() {
|
||||
if(webSrv.loop()) return; // if true: ignore all other for faster response to web
|
||||
audio.loop();
|
||||
}
|
||||
//----------------------------------------------------------------------------------------------------------------------
|
||||
// E V E N T S
|
||||
//----------------------------------------------------------------------------------------------------------------------
|
||||
void WEBSRV_onCommand(const String cmd, const String param, const String arg){ // called from html
|
||||
log_d("WS_onCmd: cmd=\"%s\", params=\"%s\", arg=\"%s\"", cmd.c_str(),param.c_str(), arg.c_str());
|
||||
if(cmd == "index.html"){ webSrv.show(index_html); return;}
|
||||
if(cmd == "ping"){webSrv.send("pong"); return;}
|
||||
if(cmd == "favicon.ico") return;
|
||||
if(cmd == "DLNA_getServer") {DLNA_showServer(); return;}
|
||||
if(cmd == "DLNA_getContent0"){DLNA_showContent(param, 0); return;}
|
||||
if(cmd == "DLNA_getContent1"){DLNA_showContent(param, 1); return;} // search for level 1 content
|
||||
if(cmd == "DLNA_getContent2"){DLNA_showContent(param, 2); return;} // search for level 2 content
|
||||
if(cmd == "DLNA_getContent3"){DLNA_showContent(param, 3); return;} // search for level 3 content
|
||||
if(cmd == "DLNA_getContent4"){DLNA_showContent(param, 4); return;} // search for level 4 content
|
||||
if(cmd == "DLNA_getContent5"){DLNA_showContent(param, 5); return;} // search for level 5 content
|
||||
log_e("unknown HTMLcommand %s, param=%s", cmd.c_str(), param.c_str());
|
||||
}
|
||||
void WEBSRV_onRequest(const String request, uint32_t contentLength){
|
||||
log_d("WS_onReq: %s contentLength %d", request.c_str(), contentLength);
|
||||
if(request.startsWith("------")) return; // uninteresting WebKitFormBoundaryString
|
||||
if(request.indexOf("form-data") > 0) return; // uninteresting Info
|
||||
log_e("unknown request: %s",request.c_str());
|
||||
}
|
||||
void WEBSRV_onInfo(const char* info){
|
||||
log_v("HTML_info: %s", info); // infos for debug
|
||||
}
|
||||
void audio_info(const char *info){
|
||||
Serial.print("info "); Serial.println(info);
|
||||
}
|
||||
void audio_id3data(const char *info){ //id3 metadata
|
||||
Serial.print("id3data ");Serial.println(info);
|
||||
}
|
||||
void audio_eof_stream(const char* info){ // The webstream comes to an end
|
||||
Serial.print("end of stream: ");Serial.println(info);
|
||||
}
|
||||
void audio_bitrate(const char *info){
|
||||
Serial.print("bitrate ");Serial.println(info);
|
||||
}
|
||||
void audio_lasthost(const char *info){ //stream URL played
|
||||
Serial.print("lasthost ");Serial.println(info);
|
||||
}
|
11
lib/ESP32-audioI2S-3.0.0/examples/DLNA/test/README
Normal file
@ -0,0 +1,11 @@
|
||||
|
||||
This directory is intended for PlatformIO Test Runner and project tests.
|
||||
|
||||
Unit Testing is a software testing method by which individual units of
|
||||
source code, sets of one or more MCU program modules together with associated
|
||||
control data, usage procedures, and operating procedures, are tested to
|
||||
determine whether they are fit for use. Unit testing finds problems early
|
||||
in the development cycle.
|
||||
|
||||
More information about PlatformIO Unit Testing:
|
||||
- https://docs.platformio.org/en/latest/advanced/unit-testing/index.html
|
BIN
lib/ESP32-audioI2S-3.0.0/examples/ESP32_A1S/A1S.pdf
Normal file
BIN
lib/ESP32-audioI2S-3.0.0/examples/ESP32_A1S/A1S_Radio1.jpg
Normal file
After Width: | Height: | Size: 48 KiB |
BIN
lib/ESP32-audioI2S-3.0.0/examples/ESP32_A1S/A1S_Radio2.jpg
Normal file
After Width: | Height: | Size: 72 KiB |
After Width: | Height: | Size: 79 KiB |
156
lib/ESP32-audioI2S-3.0.0/examples/ESP32_A1S/ESP32_A1S.ino
Normal file
@ -0,0 +1,156 @@
|
||||
// found this sketch on https://www.mikrocontroller.net/topic/474383
|
||||
// thanks to Michael U. (amiga)
|
||||
|
||||
#include "Arduino.h"
|
||||
#include "WiFi.h"
|
||||
#include "SPI.h"
|
||||
#include "SD.h"
|
||||
#include "FS.h"
|
||||
#include "Wire.h"
|
||||
#include "AC101.h" //https://github.com/schreibfaul1/AC101
|
||||
// #include "ES8388.h" // https://github.com/maditnerd/es8388
|
||||
#include "Audio.h" //https://github.com/schreibfaul1/ESP32-audioI2S
|
||||
|
||||
|
||||
// SPI GPIOs
|
||||
#define SD_CS 13
|
||||
#define SPI_MOSI 15
|
||||
#define SPI_MISO 2
|
||||
#define SPI_SCK 14
|
||||
|
||||
// I2S GPIOs, the names refer on AC101, AS1 Audio Kit V2.2 2379
|
||||
#define I2S_DSIN 25
|
||||
#define I2S_BCLK 27
|
||||
#define I2S_LRC 26
|
||||
#define I2S_MCLK 0
|
||||
#define I2S_DOUT 35
|
||||
|
||||
// I2S GPIOs, the names refer on ES8388, AS1 Audio Kit V2.2 3378
|
||||
//#define I2S_DSIN 35
|
||||
//#define I2S_BCLK 27
|
||||
//#define I2S_LRC 25
|
||||
//#define I2S_MCLK 0
|
||||
//#define I2S_DOUT 26
|
||||
|
||||
// I2C GPIOs
|
||||
#define IIC_CLK 32
|
||||
#define IIC_DATA 33
|
||||
|
||||
// buttons
|
||||
// #define BUTTON_2_PIN 13 // shared mit SPI_CS
|
||||
#define BUTTON_3_PIN 19
|
||||
#define BUTTON_4_PIN 23
|
||||
#define BUTTON_5_PIN 18 // Stop
|
||||
#define BUTTON_6_PIN 5 // Play
|
||||
|
||||
// amplifier enable
|
||||
#define GPIO_PA_EN 21
|
||||
|
||||
//Switch S1: 1-OFF, 2-ON, 3-ON, 4-OFF, 5-OFF
|
||||
|
||||
String ssid = "xxxxxxxxx";
|
||||
String password = "xxxxxxxxx";
|
||||
|
||||
static AC101 dac; // AC101
|
||||
// ES8388 dac; // ES8388 (new board)
|
||||
int volume = 40; // 0...100
|
||||
|
||||
Audio audio;
|
||||
|
||||
//#####################################################################
|
||||
|
||||
void setup()
|
||||
{
|
||||
Serial.begin(115200);
|
||||
Serial.println("\r\nReset");
|
||||
Serial.printf_P(PSTR("Free mem=%d\n"), ESP.getFreeHeap());
|
||||
|
||||
pinMode(SD_CS, OUTPUT);
|
||||
digitalWrite(SD_CS, HIGH);
|
||||
SPI.begin(SPI_SCK, SPI_MISO, SPI_MOSI);
|
||||
SPI.setFrequency(1000000);
|
||||
|
||||
SD.begin(SD_CS);
|
||||
|
||||
WiFi.mode(WIFI_STA);
|
||||
WiFi.begin(ssid.c_str(), password.c_str());
|
||||
|
||||
while (WiFi.status() != WL_CONNECTED)
|
||||
{
|
||||
Serial.print(".");
|
||||
delay(100);
|
||||
}
|
||||
|
||||
Serial.printf_P(PSTR("Connected\r\nRSSI: "));
|
||||
Serial.print(WiFi.RSSI());
|
||||
Serial.print(" IP: ");
|
||||
Serial.println(WiFi.localIP());
|
||||
|
||||
Serial.printf("Connect to DAC codec... ");
|
||||
while (not dac.begin(IIC_DATA, IIC_CLK))
|
||||
{
|
||||
Serial.printf("Failed!\n");
|
||||
delay(1000);
|
||||
}
|
||||
Serial.printf("OK\n");
|
||||
|
||||
dac.SetVolumeSpeaker(volume);
|
||||
dac.SetVolumeHeadphone(volume);
|
||||
// ac.DumpRegisters();
|
||||
|
||||
// Enable amplifier
|
||||
pinMode(GPIO_PA_EN, OUTPUT);
|
||||
digitalWrite(GPIO_PA_EN, HIGH);
|
||||
|
||||
// set I2S_MasterClock
|
||||
audio.i2s_mclk_pin_select(I2S_MCLK);
|
||||
|
||||
audio.setPinout(I2S_BCLK, I2S_LRC, I2S_DSIN);
|
||||
audio.setVolume(10); // 0...21
|
||||
|
||||
audio.connecttoFS(SD, "/320k_test.mp3");
|
||||
// audio.connecttoSD("/Banana Boat Song - Harry Belafonte.mp3");
|
||||
// audio.connecttohost("http://mp3channels.webradio.antenne.de:80/oldies-but-goldies");
|
||||
// audio.connecttohost("http://dg-rbb-http-dus-dtag-cdn.cast.addradio.de/rbb/antennebrandenburg/live/mp3/128/stream.mp3");
|
||||
// audio.connecttospeech("Wenn die Hunde schlafen, kann der Wolf gut Schafe stehlen.", "de");
|
||||
|
||||
}
|
||||
|
||||
//-----------------------------------------------------------------------
|
||||
|
||||
void loop()
|
||||
{
|
||||
audio.loop();
|
||||
}
|
||||
|
||||
// optional
|
||||
void audio_info(const char *info){
|
||||
Serial.print("info "); Serial.println(info);
|
||||
}
|
||||
void audio_id3data(const char *info){ //id3 metadata
|
||||
Serial.print("id3data ");Serial.println(info);
|
||||
}
|
||||
void audio_eof_mp3(const char *info){ //end of file
|
||||
Serial.print("eof_mp3 ");Serial.println(info);
|
||||
}
|
||||
void audio_showstation(const char *info){
|
||||
Serial.print("station ");Serial.println(info);
|
||||
}
|
||||
void audio_showstreamtitle(const char *info){
|
||||
Serial.print("streamtitle ");Serial.println(info);
|
||||
}
|
||||
void audio_bitrate(const char *info){
|
||||
Serial.print("bitrate ");Serial.println(info);
|
||||
}
|
||||
void audio_commercial(const char *info){ //duration in sec
|
||||
Serial.print("commercial ");Serial.println(info);
|
||||
}
|
||||
void audio_icyurl(const char *info){ //homepage
|
||||
Serial.print("icyurl ");Serial.println(info);
|
||||
}
|
||||
void audio_lasthost(const char *info){ //stream URL played
|
||||
Serial.print("lasthost ");Serial.println(info);
|
||||
}
|
||||
void audio_eof_speech(const char *info){
|
||||
Serial.print("eof_speech ");Serial.println(info);
|
||||
}
|
79891
lib/ESP32-audioI2S-3.0.0/examples/ESP32_ES8388/ESP32-ADF_Rev_C.svg
Normal file
After Width: | Height: | Size: 1.5 MiB |
137
lib/ESP32-audioI2S-3.0.0/examples/ESP32_ES8388/ESP32_ES8388.ino
Normal file
@ -0,0 +1,137 @@
|
||||
// the pin assignment matches the Olimex ADF board
|
||||
|
||||
#include "Arduino.h"
|
||||
#include "WiFi.h"
|
||||
#include "SPI.h"
|
||||
#include "SD.h"
|
||||
#include "FS.h"
|
||||
#include "Wire.h"
|
||||
#include "ES8388.h" // https://github.com/maditnerd/es8388
|
||||
#include "Audio.h" // https://github.com/schreibfaul1/ESP32-audioI2S
|
||||
|
||||
|
||||
#define SD_CS 21
|
||||
|
||||
// GPIOs for SPI
|
||||
#define SPI_MOSI 13
|
||||
#define SPI_MISO 12
|
||||
#define SPI_SCK 14
|
||||
|
||||
// I2S GPIOs
|
||||
#define I2S_SDOUT 26
|
||||
#define I2S_BCLK 5
|
||||
#define I2S_LRCK 25
|
||||
#define I2S_MCLK 0
|
||||
|
||||
// I2C GPIOs
|
||||
#define IIC_CLK 23
|
||||
#define IIC_DATA 18
|
||||
|
||||
// Amplifier enable
|
||||
#define GPIO_PA_EN 19
|
||||
|
||||
char ssid[] = "xxxxxxxxx";
|
||||
char password[] = "xxxxxxxxx";
|
||||
|
||||
|
||||
int volume = 80; // 0...100
|
||||
|
||||
ES8388 es;
|
||||
Audio audio;
|
||||
|
||||
//----------------------------------------------------------------------------------------------------------------------
|
||||
|
||||
void setup()
|
||||
{
|
||||
Serial.begin(115200);
|
||||
Serial.println("\r\nReset");
|
||||
Serial.printf_P(PSTR("Free mem=%d\n"), ESP.getFreeHeap());
|
||||
|
||||
SPI.begin(SPI_SCK, SPI_MISO, SPI_MOSI);
|
||||
SPI.setFrequency(1000000);
|
||||
|
||||
SD.begin(SD_CS);
|
||||
|
||||
WiFi.mode(WIFI_STA);
|
||||
WiFi.begin(ssid, password);
|
||||
|
||||
while(WiFi.status() != WL_CONNECTED) {
|
||||
Serial.print(".");
|
||||
delay(100);
|
||||
}
|
||||
|
||||
Serial.printf_P(PSTR("Connected\r\nRSSI: "));
|
||||
Serial.print(WiFi.RSSI());
|
||||
Serial.print(" IP: ");
|
||||
Serial.println(WiFi.localIP());
|
||||
|
||||
Serial.printf("Connect to ES8388 codec... ");
|
||||
while (not es.begin(IIC_DATA, IIC_CLK))
|
||||
{
|
||||
Serial.printf("Failed!\n");
|
||||
delay(1000);
|
||||
}
|
||||
Serial.printf("OK\n");
|
||||
|
||||
es.volume(ES8388::ES_MAIN, volume);
|
||||
es.volume(ES8388::ES_OUT1, volume);
|
||||
es.volume(ES8388::ES_OUT2, volume);
|
||||
es.mute(ES8388::ES_OUT1, false);
|
||||
es.mute(ES8388::ES_OUT2, false);
|
||||
es.mute(ES8388::ES_MAIN, false);
|
||||
|
||||
// Enable amplifier
|
||||
pinMode(GPIO_PA_EN, OUTPUT);
|
||||
digitalWrite(GPIO_PA_EN, HIGH);
|
||||
|
||||
audio.setPinout(I2S_BCLK, I2S_LRCK, I2S_SDOUT);
|
||||
audio.i2s_mclk_pin_select(I2S_MCLK);
|
||||
audio.setVolume(21); // 0...21
|
||||
|
||||
audio.connecttohost("http://mp3channels.webradio.antenne.de:80/oldies-but-goldies");
|
||||
// audio.connecttoFS(SD, "320k_test.mp3");
|
||||
// audio.connecttospeech("Wenn die Hunde schlafen, kann der Wolf gut Schafe stehlen.", "de");
|
||||
}
|
||||
//----------------------------------------------------------------------------------------------------------------------
|
||||
void loop()
|
||||
{
|
||||
audio.loop();
|
||||
}
|
||||
//----------------------------------------------------------------------------------------------------------------------
|
||||
|
||||
// optional
|
||||
void audio_info(const char *info){
|
||||
Serial.print("info "); Serial.println(info);
|
||||
}
|
||||
void audio_id3data(const char *info){ //id3 metadata
|
||||
Serial.print("id3data ");Serial.println(info);
|
||||
}
|
||||
void audio_eof_mp3(const char *info){ //end of file
|
||||
Serial.print("eof_mp3 ");Serial.println(info);
|
||||
}
|
||||
void audio_showstation(const char *info){
|
||||
Serial.print("station ");Serial.println(info);
|
||||
}
|
||||
void audio_showstreaminfo(const char *info){
|
||||
Serial.print("streaminfo ");Serial.println(info);
|
||||
}
|
||||
void audio_showstreamtitle(const char *info){
|
||||
Serial.print("streamtitle ");Serial.println(info);
|
||||
}
|
||||
void audio_bitrate(const char *info){
|
||||
Serial.print("bitrate ");Serial.println(info);
|
||||
}
|
||||
void audio_commercial(const char *info){ //duration in sec
|
||||
Serial.print("commercial ");Serial.println(info);
|
||||
}
|
||||
void audio_icyurl(const char *info){ //homepage
|
||||
Serial.print("icyurl ");Serial.println(info);
|
||||
}
|
||||
void audio_lasthost(const char *info){ //stream URL played
|
||||
Serial.print("lasthost ");Serial.println(info);
|
||||
}
|
||||
void audio_eof_speech(const char *info){
|
||||
Serial.print("eof_speech ");Serial.println(info);
|
||||
}
|
||||
|
||||
|
@ -0,0 +1,86 @@
|
||||
// Copied from https://github.com/LilyGO/TTGO-TAudio/issues/12
|
||||
|
||||
|
||||
// Required Libraries (Download zips and add to the Arduino IDE library).
|
||||
#include "Arduino.h"
|
||||
#include <WM8978.h> // https://github.com/CelliesProjects/wm8978-esp32
|
||||
#include <Audio.h> // https://github.com/schreibfaul1/ESP32-audioI2S
|
||||
|
||||
// T-Audio 1.6 WM8978 I2C pins.
|
||||
#define I2C_SDA 19
|
||||
#define I2C_SCL 18
|
||||
|
||||
// T-Audio 1.6 WM8978 I2S pins.
|
||||
#define I2S_BCK 33
|
||||
#define I2S_WS 25
|
||||
#define I2S_DOUT 26
|
||||
|
||||
// T-Audio 1.6 WM8978 MCLK gpio number
|
||||
#define I2S_MCLKPIN 0
|
||||
|
||||
Audio audio;
|
||||
WM8978 dac;
|
||||
|
||||
void setup() {
|
||||
Serial.begin(115200);
|
||||
|
||||
// Setup wm8978 I2C interface.
|
||||
if (!dac.begin(I2C_SDA, I2C_SCL)) {
|
||||
ESP_LOGE(TAG, "Error setting up dac: System halted.");
|
||||
while (1) delay(100);
|
||||
}
|
||||
|
||||
// Select I2S pins
|
||||
audio.setPinout(I2S_BCK, I2S_WS, I2S_DOUT);
|
||||
audio.i2s_mclk_pin_select(I2S_MCLKPIN);
|
||||
|
||||
// WiFi Settings here.
|
||||
WiFi.begin("EnterSSIDHere", "EnterPasswordHere");
|
||||
while (!WiFi.isConnected()) {
|
||||
delay(10);
|
||||
}
|
||||
ESP_LOGI(TAG, "Connected. Starting MP3...");
|
||||
// Enter your Icecast station URL here.
|
||||
audio.setVolume(21);
|
||||
audio.connecttohost("http://hestia2.cdnstream.com/1458_128");
|
||||
// Volume control.
|
||||
dac.setSPKvol(63); // Change volume here for board speaker output (Max 63).
|
||||
dac.setHPvol(63, 63); // Change volume here for headphone jack left, right channel.
|
||||
}
|
||||
|
||||
void loop() {
|
||||
// Start the stream.
|
||||
audio.loop();
|
||||
}
|
||||
|
||||
// optional
|
||||
void audio_info(const char *info){
|
||||
Serial.print("info "); Serial.println(info);
|
||||
}
|
||||
void audio_id3data(const char *info){ //id3 metadata
|
||||
Serial.print("id3data ");Serial.println(info);
|
||||
}
|
||||
void audio_eof_mp3(const char *info){ //end of file
|
||||
Serial.print("eof_mp3 ");Serial.println(info);
|
||||
}
|
||||
void audio_showstation(const char *info){
|
||||
Serial.print("station ");Serial.println(info);
|
||||
}
|
||||
void audio_showstreamtitle(const char *info){
|
||||
Serial.print("streamtitle ");Serial.println(info);
|
||||
}
|
||||
void audio_bitrate(const char *info){
|
||||
Serial.print("bitrate ");Serial.println(info);
|
||||
}
|
||||
void audio_commercial(const char *info){ //duration in sec
|
||||
Serial.print("commercial ");Serial.println(info);
|
||||
}
|
||||
void audio_icyurl(const char *info){ //homepage
|
||||
Serial.print("icyurl ");Serial.println(info);
|
||||
}
|
||||
void audio_lasthost(const char *info){ //stream URL played
|
||||
Serial.print("lasthost ");Serial.println(info);
|
||||
}
|
||||
void audio_eof_speech(const char *info){
|
||||
Serial.print("eof_speech ");Serial.println(info);
|
||||
}
|
BIN
lib/ESP32-audioI2S-3.0.0/examples/ESP32_TTGO-TAudio/T9V1.5.jpg
Normal file
After Width: | Height: | Size: 271 KiB |
100
lib/ESP32-audioI2S-3.0.0/examples/I2Saudio/I2Saudio.ino
Normal file
@ -0,0 +1,100 @@
|
||||
//**********************************************************************************************************
|
||||
//* audioI2S-- I2S audiodecoder for ESP32, *
|
||||
//**********************************************************************************************************
|
||||
//
|
||||
// first release on 11/2018
|
||||
// Version 3 , Jul.02/2020
|
||||
//
|
||||
//
|
||||
// THE SOFTWARE IS PROVIDED "AS IS" FOR PRIVATE USE ONLY, IT IS NOT FOR COMMERCIAL USE IN WHOLE OR PART OR CONCEPT.
|
||||
// FOR PERSONAL USE IT IS SUPPLIED WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE
|
||||
// WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHOR
|
||||
// OR COPYRIGHT HOLDER BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
|
||||
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE
|
||||
//
|
||||
|
||||
#include "Arduino.h"
|
||||
#include "WiFiMulti.h"
|
||||
#include "Audio.h"
|
||||
#include "SPI.h"
|
||||
#include "SD.h"
|
||||
#include "FS.h"
|
||||
|
||||
// Digital I/O used
|
||||
#define SD_CS 5
|
||||
#define SPI_MOSI 23
|
||||
#define SPI_MISO 19
|
||||
#define SPI_SCK 18
|
||||
#define I2S_DOUT 25
|
||||
#define I2S_BCLK 27
|
||||
#define I2S_LRC 26
|
||||
|
||||
Audio audio;
|
||||
WiFiMulti wifiMulti;
|
||||
String ssid = "xxxxx";
|
||||
String password = "xxxxx";
|
||||
|
||||
|
||||
void setup() {
|
||||
pinMode(SD_CS, OUTPUT); digitalWrite(SD_CS, HIGH);
|
||||
SPI.begin(SPI_SCK, SPI_MISO, SPI_MOSI);
|
||||
SPI.setFrequency(1000000);
|
||||
Serial.begin(115200);
|
||||
SD.begin(SD_CS);
|
||||
WiFi.mode(WIFI_STA);
|
||||
wifiMulti.addAP(ssid.c_str(), password.c_str());
|
||||
wifiMulti.run();
|
||||
if(WiFi.status() != WL_CONNECTED){
|
||||
WiFi.disconnect(true);
|
||||
wifiMulti.run();
|
||||
}
|
||||
audio.setPinout(I2S_BCLK, I2S_LRC, I2S_DOUT);
|
||||
audio.setVolume(12); // 0...21
|
||||
|
||||
// audio.connecttoFS(SD, "/320k_test.mp3");
|
||||
// audio.connecttoFS(SD, "test.wav");
|
||||
// audio.connecttohost("http://www.wdr.de/wdrlive/media/einslive.m3u");
|
||||
// audio.connecttohost("http://somafm.com/wma128/missioncontrol.asx"); // asx
|
||||
// audio.connecttohost("http://mp3.ffh.de/radioffh/hqlivestream.aac"); // 128k aac
|
||||
audio.connecttohost("http://mp3.ffh.de/radioffh/hqlivestream.mp3"); // 128k mp3
|
||||
}
|
||||
|
||||
void loop()
|
||||
{
|
||||
audio.loop();
|
||||
if(Serial.available()){ // put streamURL in serial monitor
|
||||
audio.stopSong();
|
||||
String r=Serial.readString(); r.trim();
|
||||
if(r.length()>5) audio.connecttohost(r.c_str());
|
||||
log_i("free heap=%i", ESP.getFreeHeap());
|
||||
}
|
||||
}
|
||||
|
||||
// optional
|
||||
void audio_info(const char *info){
|
||||
Serial.print("info "); Serial.println(info);
|
||||
}
|
||||
void audio_id3data(const char *info){ //id3 metadata
|
||||
Serial.print("id3data ");Serial.println(info);
|
||||
}
|
||||
void audio_eof_mp3(const char *info){ //end of file
|
||||
Serial.print("eof_mp3 ");Serial.println(info);
|
||||
}
|
||||
void audio_showstation(const char *info){
|
||||
Serial.print("station ");Serial.println(info);
|
||||
}
|
||||
void audio_showstreamtitle(const char *info){
|
||||
Serial.print("streamtitle ");Serial.println(info);
|
||||
}
|
||||
void audio_bitrate(const char *info){
|
||||
Serial.print("bitrate ");Serial.println(info);
|
||||
}
|
||||
void audio_commercial(const char *info){ //duration in sec
|
||||
Serial.print("commercial ");Serial.println(info);
|
||||
}
|
||||
void audio_icyurl(const char *info){ //homepage
|
||||
Serial.print("icyurl ");Serial.println(info);
|
||||
}
|
||||
void audio_lasthost(const char *info){ //stream URL played
|
||||
Serial.print("lasthost ");Serial.println(info);
|
||||
}
|
@ -0,0 +1,44 @@
|
||||
//**********************************************************************************************************
|
||||
//* audioI2S-- I2S audiodecoder for ESP32, internal DAC example *
|
||||
//**********************************************************************************************************
|
||||
//
|
||||
// Sep.09/2022
|
||||
//
|
||||
// THE SOFTWARE IS PROVIDED "AS IS" FOR PRIVATE USE ONLY, IT IS NOT FOR COMMERCIAL USE IN WHOLE OR PART OR CONCEPT.
|
||||
// FOR PERSONAL USE IT IS SUPPLIED WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE
|
||||
// WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHOR
|
||||
// OR COPYRIGHT HOLDER BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
|
||||
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE
|
||||
//
|
||||
|
||||
#include "Arduino.h"
|
||||
#include "WiFiMulti.h"
|
||||
#include "Audio.h"
|
||||
|
||||
Audio audio(true, I2S_DAC_CHANNEL_BOTH_EN);
|
||||
WiFiMulti wifiMulti;
|
||||
String ssid = "xxxxx";
|
||||
String password = "xxxxx";
|
||||
|
||||
void setup() {
|
||||
Serial.begin(115200);
|
||||
WiFi.mode(WIFI_STA);
|
||||
wifiMulti.addAP(ssid.c_str(), password.c_str());
|
||||
wifiMulti.run();
|
||||
if(WiFi.status() != WL_CONNECTED){
|
||||
WiFi.disconnect(true);
|
||||
wifiMulti.run();
|
||||
}
|
||||
audio.setVolume(12); // 0...21
|
||||
|
||||
audio.connecttohost("http://mp3.ffh.de/radioffh/hqlivestream.mp3"); // 128k mp3
|
||||
}
|
||||
|
||||
void loop()
|
||||
{
|
||||
audio.loop();
|
||||
}
|
||||
|
||||
void audio_info(const char *info){
|
||||
Serial.print("info "); Serial.println(info);
|
||||
}
|
103
lib/ESP32-audioI2S-3.0.0/examples/M5Core2/M5Core2.ino
Normal file
@ -0,0 +1,103 @@
|
||||
//**********************************************************************************************************
|
||||
//* audioI2S-- I2S audiodecoder for M5Stack Core2 *
|
||||
//**********************************************************************************************************
|
||||
//
|
||||
// first release on May.12/2021
|
||||
//
|
||||
//
|
||||
// THE SOFTWARE IS PROVIDED "AS IS" FOR PRIVATE USE ONLY, IT IS NOT FOR COMMERCIAL USE IN WHOLE OR PART OR CONCEPT.
|
||||
// FOR PERSONAL USE IT IS SUPPLIED WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE
|
||||
// WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHOR
|
||||
// OR COPYRIGHT HOLDER BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
|
||||
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE
|
||||
//
|
||||
|
||||
#include <M5Core2.h>
|
||||
#include "Audio.h"
|
||||
|
||||
// Digital I/O used
|
||||
#define SD_CS 4
|
||||
#define SD_MOSI 23
|
||||
#define SD_MISO 38
|
||||
#define SD_SCK 18
|
||||
#define I2S_DOUT 2
|
||||
#define I2S_BCLK 12
|
||||
#define I2S_LRC 0
|
||||
|
||||
Audio audio;
|
||||
String ssid = "xxxxxx";
|
||||
String password = "xxxxxx";
|
||||
|
||||
|
||||
void setup() {
|
||||
M5.begin(true, true, true, true);
|
||||
M5.Axp.SetSpkEnable(true);
|
||||
M5.Lcd.fillScreen(BLACK);
|
||||
M5.Lcd.setTextColor(WHITE);
|
||||
M5.Lcd.setTextSize(2);
|
||||
|
||||
pinMode(SD_CS, OUTPUT);
|
||||
digitalWrite(SD_CS, HIGH);
|
||||
SPI.begin(SD_SCK, SD_MISO, SD_MOSI);
|
||||
SPI.setFrequency(1000000);
|
||||
SD.begin(SD_CS);
|
||||
|
||||
audio.setPinout(I2S_BCLK, I2S_LRC, I2S_DOUT);
|
||||
audio.setVolume(15); // 0...21
|
||||
|
||||
WiFi.mode(WIFI_STA);
|
||||
WiFi.begin(ssid.c_str(), password.c_str());
|
||||
while (!WiFi.isConnected()) {
|
||||
delay(10);
|
||||
}
|
||||
ESP_LOGI(TAG, "Connected");
|
||||
ESP_LOGI(TAG, "Starting MP3...\n");
|
||||
|
||||
// audio.connecttoFS(SD, "/320k_test.mp3");
|
||||
// audio.connecttoFS(SD, "test.wav");
|
||||
audio.connecttohost("http://air.ofr.fm:8008/jazz/mp3/128");
|
||||
// audio.connecttospeech("Миска вареників з картоплею та шкварками, змащених салом!", "uk-UA");
|
||||
}
|
||||
|
||||
void loop() {
|
||||
audio.loop();
|
||||
if(Serial.available()){ // put streamURL in serial monitor
|
||||
audio.stopSong();
|
||||
String r=Serial.readString();
|
||||
r.trim();
|
||||
if(r.length()>5) audio.connecttohost(r.c_str());
|
||||
log_i("free heap=%i", ESP.getFreeHeap());
|
||||
}
|
||||
}
|
||||
|
||||
// optional
|
||||
void audio_info(const char *info){
|
||||
Serial.print("info "); Serial.println(info);
|
||||
}
|
||||
void audio_id3data(const char *info){ //id3 metadata
|
||||
Serial.print("id3data ");Serial.println(info);
|
||||
}
|
||||
void audio_eof_mp3(const char *info){ //end of file
|
||||
Serial.print("eof_mp3 ");Serial.println(info);
|
||||
}
|
||||
void audio_showstation(const char *info){
|
||||
Serial.print("station ");Serial.println(info);
|
||||
}
|
||||
void audio_showstreamtitle(const char *info){
|
||||
Serial.print("streamtitle ");Serial.println(info);
|
||||
}
|
||||
void audio_bitrate(const char *info){
|
||||
Serial.print("bitrate ");Serial.println(info);
|
||||
}
|
||||
void audio_commercial(const char *info){ //duration in sec
|
||||
Serial.print("commercial ");Serial.println(info);
|
||||
}
|
||||
void audio_icyurl(const char *info){ //homepage
|
||||
Serial.print("icyurl ");Serial.println(info);
|
||||
}
|
||||
void audio_lasthost(const char *info){ //stream URL played
|
||||
Serial.print("lasthost ");Serial.println(info);
|
||||
}
|
||||
void audio_eof_speech(const char *info){
|
||||
Serial.print("eof_speech ");Serial.println(info);
|
||||
}
|
@ -0,0 +1,53 @@
|
||||
|
||||
// M5Stack Node support
|
||||
// thanks to Cellie - issue #35 25.Apr.2020
|
||||
// M5Stack board with Node base also need a MCLK signal on GPIO0.
|
||||
|
||||
#include <wm8978.h> /* https://github.com/CelliesProjects/wm8978-esp32 */
|
||||
#include <Audio.h> /* https://github.com/schreibfaul1/ESP32-audioI2S */
|
||||
|
||||
/* M5Stack Node WM8978 I2C pins */
|
||||
#define I2C_SDA 21
|
||||
#define I2C_SCL 22
|
||||
|
||||
/* M5Stack Node I2S pins */
|
||||
#define I2S_BCK 5
|
||||
#define I2S_WS 13
|
||||
#define I2S_DOUT 2
|
||||
#define I2S_DIN 34
|
||||
|
||||
/* M5Stack WM8978 MCLK gpio number */
|
||||
#define I2S_MCLKPIN 0
|
||||
|
||||
WM8978 dac;
|
||||
Audio audio;
|
||||
|
||||
void setup() {
|
||||
/* Setup wm8978 I2C interface */
|
||||
if (!dac.begin(I2C_SDA, I2C_SCL)) {
|
||||
ESP_LOGE(TAG, "Error setting up dac. System halted");
|
||||
while (1) delay(100);
|
||||
}
|
||||
|
||||
/* Setup wm8978 I2S interface */
|
||||
audio.setPinout(I2S_BCK, I2S_WS, I2S_DOUT, I2S_DIN);
|
||||
|
||||
/* Setup wm8978 MCLK - for example M5Stack Node needs MCLK on GPIO 0 */
|
||||
audio.i2s_mclk_pin_select(I2S_MCLKPIN);
|
||||
|
||||
WiFi.begin("xxx", "xxx");
|
||||
while (!WiFi.isConnected()) {
|
||||
delay(10);
|
||||
}
|
||||
ESP_LOGI(TAG, "Connected");
|
||||
|
||||
ESP_LOGI(TAG, "Starting MP3...\n");
|
||||
audio.connecttohost("http://icecast.omroep.nl/3fm-bb-mp3");
|
||||
|
||||
dac.setSPKvol(40); /* max 63 */
|
||||
dac.setHPvol(32, 32);
|
||||
}
|
||||
|
||||
void loop() {
|
||||
audio.loop();
|
||||
}
|
@ -0,0 +1,88 @@
|
||||
//**********************************************************************************************************
|
||||
//* audioI2S-- I2S audiodecoder for M5StickC Plus and SPK HAT *
|
||||
//**********************************************************************************************************
|
||||
//
|
||||
// first release on May.12/2021
|
||||
//
|
||||
//
|
||||
// THE SOFTWARE IS PROVIDED "AS IS" FOR PRIVATE USE ONLY, IT IS NOT FOR COMMERCIAL USE IN WHOLE OR PART OR CONCEPT.
|
||||
// FOR PERSONAL USE IT IS SUPPLIED WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE
|
||||
// WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHOR
|
||||
// OR COPYRIGHT HOLDER BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
|
||||
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE
|
||||
//
|
||||
|
||||
#include <M5StickCPlus.h>
|
||||
#include "Audio.h"
|
||||
|
||||
Audio audio = Audio(true);
|
||||
|
||||
String ssid = "xxxxxxxx";
|
||||
String password = "xxxxxxxx";
|
||||
|
||||
|
||||
void setup() {
|
||||
M5.begin(false); //Lcd disabled to reduce noise
|
||||
M5.Axp.ScreenBreath(1); //Lower Lcd backlight
|
||||
pinMode(36, INPUT);
|
||||
gpio_pulldown_dis(GPIO_NUM_25);
|
||||
gpio_pullup_dis(GPIO_NUM_25);
|
||||
M5.Beep.tone(44100); //Built-in buzzer tone
|
||||
M5.Beep.end(); //disabled
|
||||
|
||||
audio.setVolume(15); // 0...21
|
||||
|
||||
WiFi.mode(WIFI_STA);
|
||||
WiFi.begin(ssid.c_str(), password.c_str());
|
||||
while (!WiFi.isConnected()) {
|
||||
delay(10);
|
||||
}
|
||||
ESP_LOGI(TAG, "Connected");
|
||||
ESP_LOGI(TAG, "Starting MP3...\n");
|
||||
|
||||
audio.connecttohost("http://air.ofr.fm:8008/jazz/mp3/128");
|
||||
// audio.connecttospeech("Миска вареників з картоплею та шкварками, змащених салом!", "uk-UA");
|
||||
}
|
||||
|
||||
void loop() {
|
||||
audio.loop();
|
||||
if(Serial.available()){ // put streamURL in serial monitor
|
||||
audio.stopSong();
|
||||
String r=Serial.readString();
|
||||
r.trim();
|
||||
if(r.length()>5) audio.connecttohost(r.c_str());
|
||||
log_i("free heap=%i", ESP.getFreeHeap());
|
||||
}
|
||||
}
|
||||
|
||||
// optional
|
||||
void audio_info(const char *info){
|
||||
Serial.print("info "); Serial.println(info);
|
||||
}
|
||||
void audio_id3data(const char *info){ //id3 metadata
|
||||
Serial.print("id3data ");Serial.println(info);
|
||||
}
|
||||
void audio_eof_mp3(const char *info){ //end of file
|
||||
Serial.print("eof_mp3 ");Serial.println(info);
|
||||
}
|
||||
void audio_showstation(const char *info){
|
||||
Serial.print("station ");Serial.println(info);
|
||||
}
|
||||
void audio_showstreamtitle(const char *info){
|
||||
Serial.print("streamtitle ");Serial.println(info);
|
||||
}
|
||||
void audio_bitrate(const char *info){
|
||||
Serial.print("bitrate ");Serial.println(info);
|
||||
}
|
||||
void audio_commercial(const char *info){ //duration in sec
|
||||
Serial.print("commercial ");Serial.println(info);
|
||||
}
|
||||
void audio_icyurl(const char *info){ //homepage
|
||||
Serial.print("icyurl ");Serial.println(info);
|
||||
}
|
||||
void audio_lasthost(const char *info){ //stream URL played
|
||||
Serial.print("lasthost ");Serial.println(info);
|
||||
}
|
||||
void audio_eof_speech(const char *info){
|
||||
Serial.print("eof_speech ");Serial.println(info);
|
||||
}
|
@ -0,0 +1,58 @@
|
||||
//**********************************************************************************************************
|
||||
//* audioI2S-- I2S audiodecoder for ESP32, SdFat example *
|
||||
//**********************************************************************************************************
|
||||
//
|
||||
// first release on 05/2020
|
||||
// updated on Sep. 27, 2021
|
||||
/*
|
||||
|
||||
⒈ install SdFat V2 from https://github.com/greiman/SdFat
|
||||
⒉ activate "SDFATFS_USED" in Audio.h
|
||||
⒊ activate "#define USE_UTF8_LONG_NAMES 1" in SdFatConfig.h
|
||||
|
||||
*/
|
||||
|
||||
#include "Arduino.h"
|
||||
#include "Audio.h"
|
||||
#include "SPI.h"
|
||||
|
||||
// Digital I/O used
|
||||
#define SD_CS 5
|
||||
#define SPI_MOSI 23
|
||||
#define SPI_MISO 19
|
||||
#define SPI_SCK 18
|
||||
#define I2S_DOUT 25
|
||||
#define I2S_BCLK 27
|
||||
#define I2S_LRC 26
|
||||
|
||||
Audio audio;
|
||||
|
||||
void setup() {
|
||||
pinMode(SD_CS, OUTPUT); digitalWrite(SD_CS, HIGH);
|
||||
SPI.begin(SPI_SCK, SPI_MISO, SPI_MOSI);
|
||||
SPI.setFrequency(1000000);
|
||||
Serial.begin(115200);
|
||||
SD.begin(SD_CS);
|
||||
|
||||
audio.setPinout(I2S_BCLK, I2S_LRC, I2S_DOUT);
|
||||
audio.setVolume(12); // 0...21
|
||||
|
||||
// audio.connecttoFS(SD, "test.mp3");
|
||||
audio.connecttoFS(SD, "良い一日私の友達.mp3");
|
||||
}
|
||||
|
||||
void loop()
|
||||
{
|
||||
audio.loop();
|
||||
}
|
||||
|
||||
// optional
|
||||
void audio_info(const char *info){
|
||||
Serial.print("info "); Serial.println(info);
|
||||
}
|
||||
void audio_id3data(const char *info){ //id3 metadata
|
||||
Serial.print("id3data ");Serial.println(info);
|
||||
}
|
||||
void audio_eof_mp3(const char *info){ //end of file
|
||||
Serial.print("eof_mp3 ");Serial.println(info);
|
||||
}
|
@ -0,0 +1,224 @@
|
||||
#include <Arduino.h>
|
||||
#include <Preferences.h>
|
||||
#include <SPI.h>
|
||||
#include <WiFi.h>
|
||||
#include "tft.h" //see my repository at github "https://github.com/schreibfaul1/ESP32-TFT-Library-ILI9486"
|
||||
#include "Audio.h" //see my repository at github "https://github.com/schreibfaul1/ESP32-audioI2S"
|
||||
|
||||
#define TFT_CS 22
|
||||
#define TFT_DC 21
|
||||
#define TP_CS 14 //16
|
||||
#define TP_IRQ 39
|
||||
#define SPI_MOSI 23
|
||||
#define SPI_MISO 19
|
||||
#define SPI_SCK 18
|
||||
#define I2S_DOUT 25
|
||||
#define I2S_BCLK 27
|
||||
#define I2S_LRC 26
|
||||
|
||||
Preferences pref;
|
||||
TFT tft;
|
||||
TP tp(TP_CS, TP_IRQ);
|
||||
Audio audio;
|
||||
|
||||
String ssid = "*****";
|
||||
String password = "*****";
|
||||
|
||||
String stations[] ={
|
||||
"0n-80s.radionetz.de:8000/0n-70s.mp3",
|
||||
"mediaserv30.live-streams.nl:8000/stream",
|
||||
"www.surfmusic.de/m3u/100-5-das-hitradio,4529.m3u",
|
||||
"stream.1a-webradio.de/deutsch/mp3-128/vtuner-1a",
|
||||
"mp3.ffh.de/radioffh/hqlivestream.aac", // 128k aac
|
||||
"www.antenne.de/webradio/antenne.m3u",
|
||||
"listen.rusongs.ru/ru-mp3-128",
|
||||
"edge.audio.3qsdn.com/senderkw-mp3",
|
||||
"https://stream.srg-ssr.ch/rsp/aacp_48.asx", // SWISS POP
|
||||
};
|
||||
|
||||
//some global variables
|
||||
|
||||
uint8_t max_volume = 21;
|
||||
uint8_t max_stations = 0; //will be set later
|
||||
uint8_t cur_station = 0; //current station(nr), will be set later
|
||||
uint8_t cur_volume = 0; //will be set from stored preferences
|
||||
int8_t cur_btn =-1; //current button (, -1 means idle)
|
||||
|
||||
enum action{VOLUME_UP=0, VOLUME_DOWN=1, STATION_UP=2, STATION_DOWN=3};
|
||||
enum staus {RELEASED=0, PRESSED=1};
|
||||
|
||||
struct _btns{
|
||||
uint16_t x; //PosX
|
||||
uint16_t y; //PosY
|
||||
uint16_t w; //Width
|
||||
uint16_t h; //Hight
|
||||
uint8_t a; //Action
|
||||
uint8_t s; //Status
|
||||
};
|
||||
typedef _btns btns;
|
||||
|
||||
btns btn[4];
|
||||
|
||||
//**************************************************************************************************
|
||||
// G U I *
|
||||
//**************************************************************************************************
|
||||
void draw_button(btns b){
|
||||
uint16_t color=TFT_BLACK;
|
||||
uint8_t r=4, o=r*3;
|
||||
if(b.s==RELEASED) color=TFT_GOLD;
|
||||
if(b.s==PRESSED) color=TFT_DEEPSKYBLUE;
|
||||
tft.drawRoundRect(b.x, b.y, b.w, b.h, r, color);
|
||||
switch(b.a){
|
||||
case VOLUME_UP:
|
||||
tft.fillTriangle(b.x+b.w/2, b.y+o, b.x+o, b.y+b.h-o, b.x+b.w-o, b.y+b.h-o, color); break;
|
||||
case VOLUME_DOWN:
|
||||
tft.fillTriangle(b.x+o, b.y+o, b.x+b.w/2, b.y+b.h-o, b.x+b.w-o, b.y+o, color); break;
|
||||
case STATION_UP:
|
||||
tft.fillTriangle(b.x+o, b.y+o, b.x+o, b.y+b.h-o, b.x+b.w-o, b.y+b.h/2, color); break;
|
||||
case STATION_DOWN:
|
||||
tft.fillTriangle(b.x+b.w-o, b.y+o, b.x+o, b.y+b.h/2, b.x+b.w-o, b.y+b.h-o, color); break;
|
||||
}
|
||||
}
|
||||
void write_stationNr(uint8_t nr){
|
||||
tft.fillRect(80, 250, 80, 60, TFT_BLACK);
|
||||
String snr = String(nr);
|
||||
if(snr.length()<2) snr = "0"+snr;
|
||||
tft.setCursor(98, 255);
|
||||
tft.setFont(Times_New_Roman66x53);
|
||||
tft.setTextColor(TFT_YELLOW);
|
||||
tft.print(snr);
|
||||
}
|
||||
void write_volume(uint8_t vol){
|
||||
tft.fillRect(320, 250, 80, 60, TFT_BLACK);
|
||||
String svol = String(vol);
|
||||
if(svol.length()<2) svol = "0"+svol;
|
||||
tft.setCursor(338, 255);
|
||||
tft.setFont(Times_New_Roman66x53);
|
||||
tft.setTextColor(TFT_YELLOW);
|
||||
tft.print(svol);
|
||||
}
|
||||
void write_stationName(String sName){
|
||||
tft.fillRect(0, 0, 480, 100, TFT_BLACK);
|
||||
tft.setFont(Times_New_Roman43x35);
|
||||
tft.setTextColor(TFT_CORNSILK);
|
||||
tft.setCursor(20, 20);
|
||||
tft.print(sName);
|
||||
}
|
||||
void write_streamTitle(String sTitle){
|
||||
tft.fillRect(0, 100, 480, 150, TFT_BLACK);
|
||||
tft.setFont(Times_New_Roman43x35);
|
||||
tft.setTextColor(TFT_LIGHTBLUE);
|
||||
tft.setCursor(20, 100);
|
||||
int l = tft.writeText((const uint8_t*) sTitle.c_str(), 100 + 150); // do not write under y=250
|
||||
if(l < sTitle.length()){
|
||||
// sTitle has been shortened, is too long for the display
|
||||
}
|
||||
}
|
||||
//**************************************************************************************************
|
||||
// S E T U P *
|
||||
//**************************************************************************************************
|
||||
void setup() {
|
||||
btn[0].x= 20; btn[0].y=250; btn[0].w=60; btn[0].h=60; btn[0].a=STATION_DOWN; btn[0].s=RELEASED;
|
||||
btn[1].x=160; btn[1].y=250; btn[1].w=60; btn[1].h=60; btn[1].a=STATION_UP; btn[1].s=RELEASED;
|
||||
btn[2].x=260; btn[2].y=250; btn[2].w=60; btn[2].h=60; btn[2].a=VOLUME_UP; btn[2].s=RELEASED;
|
||||
btn[3].x=400; btn[3].y=250; btn[3].w=60; btn[3].h=60; btn[3].a=VOLUME_DOWN; btn[3].s=RELEASED;
|
||||
max_stations= sizeof(stations)/sizeof(stations[0]); log_i("max stations %i", max_stations);
|
||||
Serial.begin(115200);
|
||||
SPI.begin(SPI_SCK, SPI_MISO, SPI_MOSI);
|
||||
pref.begin("WebRadio", false); // instance of preferences for defaults (station, volume ...)
|
||||
if(pref.getShort("volume", 1000) == 1000){ // if that: pref was never been initialized
|
||||
pref.putShort("volume", 10);
|
||||
pref.putShort("station", 0);
|
||||
}
|
||||
else{ // get the stored values
|
||||
cur_station = pref.getShort("station");
|
||||
cur_volume = pref.getShort("volume");
|
||||
}
|
||||
|
||||
WiFi.begin(ssid.c_str(), password.c_str());
|
||||
while (WiFi.status() != WL_CONNECTED){
|
||||
delay(2000);
|
||||
Serial.print(".");
|
||||
}
|
||||
log_i("Connect to %s", WiFi.SSID().c_str());
|
||||
tft.begin(TFT_CS, TFT_DC, SPI_MOSI, SPI_MISO, SPI_SCK);
|
||||
tft.setRotation(3);
|
||||
tp.setRotation(3);
|
||||
tft.setFont(Times_New_Roman43x35);
|
||||
tft.fillScreen(TFT_BLACK);
|
||||
audio.setPinout(I2S_BCLK, I2S_LRC, I2S_DOUT);
|
||||
audio.setVolume(cur_volume); // 0...21
|
||||
audio.connecttohost(stations[cur_station].c_str());
|
||||
for(uint8_t i=0; i<(sizeof(btn)/sizeof(*btn)); i++) draw_button(btn[i]);
|
||||
write_volume(cur_volume);
|
||||
write_stationNr(cur_station);
|
||||
}
|
||||
//**************************************************************************************************
|
||||
// L O O P *
|
||||
//**************************************************************************************************
|
||||
void loop()
|
||||
{
|
||||
audio.loop();
|
||||
tp.loop();
|
||||
}
|
||||
//**************************************************************************************************
|
||||
// E V E N T S *
|
||||
//**************************************************************************************************
|
||||
void audio_info(const char *info){
|
||||
Serial.print("audio_info: "); Serial.println(info);
|
||||
}
|
||||
void audio_showstation(const char *info){
|
||||
write_stationName(String(info));
|
||||
}
|
||||
void audio_showstreamtitle(const char *info){
|
||||
String sinfo=String(info);
|
||||
sinfo.replace("|", "\n");
|
||||
write_streamTitle(sinfo);
|
||||
}
|
||||
|
||||
void tp_pressed(uint16_t x, uint16_t y){
|
||||
for(uint8_t i=0; i<(sizeof(btn)/sizeof(*btn)); i++){
|
||||
if(x>btn[i].x && (x<btn[i].x+btn[i].w)){
|
||||
if(y>btn[i].y && (y<btn[i].y+btn[i].h)){
|
||||
cur_btn=i;
|
||||
btn[cur_btn].s=PRESSED;
|
||||
draw_button(btn[cur_btn]);
|
||||
}
|
||||
}
|
||||
}
|
||||
}
|
||||
void tp_released(){
|
||||
if(cur_btn !=-1){
|
||||
btn[cur_btn].s=RELEASED;
|
||||
draw_button(btn[cur_btn]);
|
||||
switch(btn[cur_btn].a){
|
||||
case VOLUME_UP: if(cur_volume<max_volume){
|
||||
cur_volume++;
|
||||
write_volume(cur_volume);
|
||||
audio.setVolume(cur_volume);
|
||||
pref.putShort("volume", cur_volume);} // store the current volume in nvs
|
||||
break;
|
||||
case VOLUME_DOWN: if(cur_volume>0){
|
||||
cur_volume-- ;
|
||||
write_volume(cur_volume);
|
||||
audio.setVolume(cur_volume);
|
||||
pref.putShort("volume", cur_volume);} // store the current volume in nvs
|
||||
break;
|
||||
case STATION_UP: if(cur_station<max_stations-1){
|
||||
cur_station++;
|
||||
write_stationNr(cur_station);
|
||||
tft.fillRect(0, 0, 480, 250, TFT_BLACK);
|
||||
audio.connecttohost(stations[cur_station].c_str());
|
||||
pref.putShort("station", cur_station);} // store the current station in nvs
|
||||
break;
|
||||
case STATION_DOWN:if(cur_station>0){
|
||||
cur_station--;
|
||||
write_stationNr(cur_station);
|
||||
tft.fillRect(0, 0, 480, 250, TFT_BLACK);
|
||||
audio.connecttohost(stations[cur_station].c_str());
|
||||
pref.putShort("station", cur_station);} // store the current station in nvs
|
||||
break;
|
||||
}
|
||||
}
|
||||
cur_btn=-1;
|
||||
}
|
After Width: | Height: | Size: 86 KiB |
After Width: | Height: | Size: 162 KiB |
After Width: | Height: | Size: 215 KiB |
After Width: | Height: | Size: 158 KiB |
After Width: | Height: | Size: 162 KiB |
@ -0,0 +1,269 @@
|
||||
// this is the same example as Webradio_I2S.ino only with an IR remote control
|
||||
|
||||
#include <Arduino.h>
|
||||
#include <Preferences.h>
|
||||
#include <SPI.h>
|
||||
#include <WiFi.h>
|
||||
#include "tft.h" //see my repository at github "https://github.com/schreibfaul1/ESP32-TFT-Library-ILI9486"
|
||||
#include "Audio.h" //see my repository at github "https://github.com/schreibfaul1/ESP32-audioI2S"
|
||||
#include "IR.h" //see my repository at github "ESP32-IR-Remote-Control"
|
||||
|
||||
|
||||
#define TFT_CS 22
|
||||
#define TFT_DC 21
|
||||
#define TP_CS 16
|
||||
#define TP_IRQ 39
|
||||
#define SPI_MOSI 23
|
||||
#define SPI_MISO 19
|
||||
#define SPI_SCK 18
|
||||
#define I2S_DOUT 25
|
||||
#define I2S_BCLK 27
|
||||
#define I2S_LRC 26
|
||||
#define IR_PIN 34
|
||||
|
||||
Preferences pref;
|
||||
TFT tft; // @suppress("Abstract class cannot be instantiated")
|
||||
TP tp(TP_CS, TP_IRQ);
|
||||
Audio audio;
|
||||
IR ir(IR_PIN); // do not change the objectname, it must be "ir"
|
||||
|
||||
String ssid = "*********";
|
||||
String password = "*********";
|
||||
|
||||
String stations[] ={
|
||||
"0n-80s.radionetz.de:8000/0n-70s.mp3",
|
||||
"mediaserv30.live-streams.nl:8000/stream",
|
||||
"www.surfmusic.de/m3u/100-5-das-hitradio,4529.m3u",
|
||||
"stream.1a-webradio.de/deutsch/mp3-128/vtuner-1a",
|
||||
"mp3.ffh.de/radioffh/hqlivestream.aac", // 128k aac
|
||||
"www.antenne.de/webradio/antenne.m3u",
|
||||
"listen.rusongs.ru/ru-mp3-128",
|
||||
"edge.audio.3qsdn.com/senderkw-mp3",
|
||||
"macslons-irish-pub-radio.com/media.asx",
|
||||
};
|
||||
|
||||
//some global variables
|
||||
|
||||
uint8_t max_volume = 21;
|
||||
uint8_t max_stations = 0; //will be set later
|
||||
uint8_t cur_station = 0; //current station(nr), will be set later
|
||||
uint8_t cur_volume = 0; //will be set from stored preferences
|
||||
int8_t cur_btn =-1; //current button (, -1 means idle)
|
||||
|
||||
enum action{VOLUME_UP=0, VOLUME_DOWN=1, STATION_UP=2, STATION_DOWN=3};
|
||||
enum staus {RELEASED=0, PRESSED=1};
|
||||
|
||||
struct _btns{
|
||||
uint16_t x; //PosX
|
||||
uint16_t y; //PosY
|
||||
uint16_t w; //Width
|
||||
uint16_t h; //Hight
|
||||
uint8_t a; //Action
|
||||
uint8_t s; //Status
|
||||
};
|
||||
typedef _btns btns;
|
||||
|
||||
btns btn[4];
|
||||
|
||||
//**************************************************************************************************
|
||||
// G U I *
|
||||
//**************************************************************************************************
|
||||
void draw_button(btns b){
|
||||
uint16_t color=TFT_BLACK;
|
||||
uint8_t r=4, o=r*3;
|
||||
if(b.s==RELEASED) color=TFT_GOLD;
|
||||
if(b.s==PRESSED) color=TFT_DEEPSKYBLUE;
|
||||
tft.drawRoundRect(b.x, b.y, b.w, b.h, r, color);
|
||||
switch(b.a){
|
||||
case VOLUME_UP:
|
||||
tft.fillTriangle(b.x+b.w/2, b.y+o, b.x+o, b.y+b.h-o, b.x+b.w-o, b.y+b.h-o, color); break;
|
||||
case VOLUME_DOWN:
|
||||
tft.fillTriangle(b.x+o, b.y+o, b.x+b.w/2, b.y+b.h-o, b.x+b.w-o, b.y+o, color); break;
|
||||
case STATION_UP:
|
||||
tft.fillTriangle(b.x+o, b.y+o, b.x+o, b.y+b.h-o, b.x+b.w-o, b.y+b.h/2, color); break;
|
||||
case STATION_DOWN:
|
||||
tft.fillTriangle(b.x+b.w-o, b.y+o, b.x+o, b.y+b.h/2, b.x+b.w-o, b.y+b.h-o, color); break;
|
||||
}
|
||||
}
|
||||
void write_stationNr(uint8_t nr){
|
||||
tft.fillRect(80, 250, 80, 60, TFT_BLACK);
|
||||
String snr = String(nr);
|
||||
if(snr.length()<2) snr = "0"+snr;
|
||||
tft.setCursor(98, 255);
|
||||
tft.setFont(Times_New_Roman66x53);
|
||||
tft.setTextColor(TFT_YELLOW);
|
||||
tft.print(snr);
|
||||
}
|
||||
void write_volume(uint8_t vol){
|
||||
tft.fillRect(320, 250, 80, 60, TFT_BLACK);
|
||||
String svol = String(vol);
|
||||
if(svol.length()<2) svol = "0"+svol;
|
||||
tft.setCursor(338, 255);
|
||||
tft.setFont(Times_New_Roman66x53);
|
||||
tft.setTextColor(TFT_YELLOW);
|
||||
tft.print(svol);
|
||||
}
|
||||
void write_stationName(String sName){
|
||||
tft.fillRect(0, 0, 480, 100, TFT_BLACK);
|
||||
tft.setFont(Times_New_Roman43x35);
|
||||
tft.setTextColor(TFT_CORNSILK);
|
||||
tft.setCursor(20, 20);
|
||||
tft.print(sName);
|
||||
}
|
||||
void write_streamTitle(String sTitle){
|
||||
tft.fillRect(0, 100, 480, 150, TFT_BLACK);
|
||||
tft.setFont(Times_New_Roman43x35);
|
||||
tft.setTextColor(TFT_LIGHTBLUE);
|
||||
tft.setCursor(20, 100);
|
||||
tft.print(sTitle);
|
||||
}
|
||||
void volume_up(){
|
||||
if(cur_volume < max_volume){
|
||||
cur_volume++;
|
||||
write_volume(cur_volume);
|
||||
audio.setVolume(cur_volume);
|
||||
pref.putShort("volume", cur_volume);} // store the current volume in nvs
|
||||
}
|
||||
void volume_down(){
|
||||
if(cur_volume>0){
|
||||
cur_volume-- ;
|
||||
write_volume(cur_volume);
|
||||
audio.setVolume(cur_volume);
|
||||
pref.putShort("volume", cur_volume);} // store the current volume in nvs
|
||||
}
|
||||
void station_up(){
|
||||
if(cur_station < max_stations-1){
|
||||
cur_station++;
|
||||
write_stationNr(cur_station);
|
||||
tft.fillRect(0, 0, 480, 250, TFT_BLACK);
|
||||
audio.connecttohost(stations[cur_station].c_str());
|
||||
pref.putShort("station", cur_station);} // store the current station in nvs
|
||||
}
|
||||
void station_down(){
|
||||
if(cur_station > 0){
|
||||
cur_station--;
|
||||
write_stationNr(cur_station);
|
||||
tft.fillRect(0, 0, 480, 250, TFT_BLACK);
|
||||
audio.connecttohost(stations[cur_station].c_str());
|
||||
pref.putShort("station", cur_station);} // store the current station in nvs
|
||||
}
|
||||
|
||||
|
||||
//**************************************************************************************************
|
||||
// S E T U P *
|
||||
//**************************************************************************************************
|
||||
void setup() {
|
||||
btn[0].x= 20; btn[0].y=250; btn[0].w=60; btn[0].h=60; btn[0].a=STATION_DOWN; btn[0].s=RELEASED;
|
||||
btn[1].x=160; btn[1].y=250; btn[1].w=60; btn[1].h=60; btn[1].a=STATION_UP; btn[1].s=RELEASED;
|
||||
btn[2].x=260; btn[2].y=250; btn[2].w=60; btn[2].h=60; btn[2].a=VOLUME_UP; btn[2].s=RELEASED;
|
||||
btn[3].x=400; btn[3].y=250; btn[3].w=60; btn[3].h=60; btn[3].a=VOLUME_DOWN; btn[3].s=RELEASED;
|
||||
max_stations= sizeof(stations)/sizeof(stations[0]); log_i("max stations %i", max_stations);
|
||||
Serial.begin(115200);
|
||||
SPI.begin(SPI_SCK, SPI_MISO, SPI_MOSI);
|
||||
pref.begin("WebRadio", false); // instance of preferences for defaults (station, volume ...)
|
||||
if(pref.getShort("volume", 1000) == 1000){ // if that: pref was never been initialized
|
||||
pref.putShort("volume", 10);
|
||||
pref.putShort("station", 0);
|
||||
}
|
||||
else{ // get the stored values
|
||||
cur_station = pref.getShort("station");
|
||||
cur_volume = pref.getShort("volume");
|
||||
}
|
||||
WiFi.disconnect();
|
||||
WiFi.begin(ssid.c_str(), password.c_str());
|
||||
while (WiFi.status() != WL_CONNECTED) {delay(1500); Serial.print(".");}
|
||||
log_i("Connected to %s", WiFi.SSID().c_str());
|
||||
tft.begin(TFT_CS, TFT_DC, SPI_MOSI, SPI_MISO, SPI_SCK);
|
||||
tft.setFrequency(20000000);
|
||||
tft.setRotation(3);
|
||||
tp.setRotation(3);
|
||||
tft.setFont(Times_New_Roman43x35);
|
||||
tft.fillScreen(TFT_BLACK);
|
||||
ir.begin(); // Init InfraredDecoder
|
||||
audio.setPinout(I2S_BCLK, I2S_LRC, I2S_DOUT);
|
||||
audio.setVolume(cur_volume); // 0...21
|
||||
audio.connecttohost(stations[cur_station].c_str());
|
||||
for(uint8_t i=0; i<(sizeof(btn)/sizeof(*btn)); i++) draw_button(btn[i]);
|
||||
write_volume(cur_volume);
|
||||
write_stationNr(cur_station);
|
||||
}
|
||||
//**************************************************************************************************
|
||||
// L O O P *
|
||||
//**************************************************************************************************
|
||||
void loop()
|
||||
{
|
||||
audio.loop();
|
||||
tp.loop();
|
||||
ir.loop();
|
||||
}
|
||||
//**************************************************************************************************
|
||||
// E V E N T S *
|
||||
//**************************************************************************************************
|
||||
void audio_info(const char *info){
|
||||
Serial.print("audio_info: "); Serial.println(info);
|
||||
}
|
||||
void audio_showstation(const char *info){
|
||||
write_stationName(String(info));
|
||||
}
|
||||
void audio_showstreamtitle(const char *info){
|
||||
String sinfo=String(info);
|
||||
sinfo.replace("|", "\n");
|
||||
write_streamTitle(sinfo);
|
||||
}
|
||||
|
||||
void tp_pressed(uint16_t x, uint16_t y){
|
||||
for(uint8_t i=0; i<(sizeof(btn)/sizeof(*btn)); i++){
|
||||
if(x>btn[i].x && (x<btn[i].x+btn[i].w)){
|
||||
if(y>btn[i].y && (y<btn[i].y+btn[i].h)){
|
||||
cur_btn=i;
|
||||
btn[cur_btn].s=PRESSED;
|
||||
draw_button(btn[cur_btn]);
|
||||
}
|
||||
}
|
||||
}
|
||||
}
|
||||
void tp_released(){
|
||||
if(cur_btn !=-1){
|
||||
btn[cur_btn].s=RELEASED;
|
||||
draw_button(btn[cur_btn]);
|
||||
switch(btn[cur_btn].a){
|
||||
case VOLUME_UP: volume_up(); break;
|
||||
case VOLUME_DOWN: volume_down(); break;
|
||||
case STATION_UP: station_up(); break;
|
||||
case STATION_DOWN: station_down(); break;
|
||||
}
|
||||
}
|
||||
cur_btn=-1;
|
||||
}
|
||||
// Events from IR Library
|
||||
void ir_res(uint32_t res){
|
||||
if(res < max_stations){
|
||||
cur_station = res;
|
||||
write_stationNr(cur_station);
|
||||
tft.fillRect(0, 0, 480, 250, TFT_BLACK);
|
||||
audio.connecttohost(stations[cur_station].c_str());
|
||||
pref.putShort("station", cur_station);} // store the current station in nvs
|
||||
else{
|
||||
tft.fillRect(0, 0, 480, 250, TFT_BLACK);
|
||||
audio.connecttohost(stations[cur_station].c_str());
|
||||
}
|
||||
}
|
||||
void ir_number(const char* num){
|
||||
tft.fillRect(0, 0, 480, 250, TFT_BLACK);
|
||||
tft.setTextSize(7);
|
||||
tft.setTextColor(TFT_CORNSILK);
|
||||
tft.setCursor(50, 70);
|
||||
tft.print(num);
|
||||
}
|
||||
void ir_key(const char* key){
|
||||
switch(key[0]){
|
||||
case 'k': break; // OK
|
||||
case 'r': volume_up(); break; // right
|
||||
case 'l': volume_down(); break; // left
|
||||
case 'u': station_up(); break; // up
|
||||
case 'd': station_down(); break; // down
|
||||
case '#': break; // #
|
||||
case '*': break; // *
|
||||
default: break;
|
||||
}
|
||||
}
|
@ -0,0 +1,68 @@
|
||||
#include "Arduino.h"
|
||||
#include "Audio.h"
|
||||
#include "SD.h"
|
||||
#include "SPI.h"
|
||||
#include "FS.h"
|
||||
#include "Ticker.h"
|
||||
|
||||
// Digital I/O used
|
||||
#define SD_CS 5
|
||||
#define SPI_MOSI 23
|
||||
#define SPI_MISO 19
|
||||
#define SPI_SCK 18
|
||||
#define I2S_DOUT 25
|
||||
#define I2S_BCLK 27
|
||||
#define I2S_LRC 26
|
||||
|
||||
Audio audio;
|
||||
Ticker ticker;
|
||||
struct tm timeinfo;
|
||||
time_t now;
|
||||
|
||||
uint8_t hour = 6;
|
||||
uint8_t minute = 59;
|
||||
uint8_t sec = 45;
|
||||
|
||||
bool f_time = false;
|
||||
int8_t timefile = -1;
|
||||
char chbuf[100];
|
||||
|
||||
void tckr1s(){
|
||||
sec++;
|
||||
if(sec > 59) {sec = 0; minute++;}
|
||||
if(minute > 59){minute = 0; hour++;}
|
||||
if(hour > 23) {hour = 0;}
|
||||
if(minute == 59 && sec == 50) f_time = true; // flag will be set 10s before full hour
|
||||
Serial.printf("%02d:%02d:%02d\n", hour, minute, sec);
|
||||
}
|
||||
|
||||
void setup() {
|
||||
Serial.begin(115200);
|
||||
SPI.begin(SPI_SCK, SPI_MISO, SPI_MOSI);
|
||||
SD.begin(SD_CS);
|
||||
audio.setPinout(I2S_BCLK, I2S_LRC, I2S_DOUT);
|
||||
audio.setVolume(10); // 0...21
|
||||
ticker.attach(1, tckr1s);
|
||||
}
|
||||
|
||||
void loop(){
|
||||
audio.loop();
|
||||
if(f_time == true){
|
||||
f_time = false;
|
||||
timefile = 3;
|
||||
uint8_t next_hour = hour + 1;
|
||||
if(next_hour == 25) next_hour = 1;
|
||||
sprintf(chbuf, "/voice_time/%03d.mp3", next_hour);
|
||||
audio.connecttoFS(SD, chbuf);
|
||||
}
|
||||
}
|
||||
|
||||
void audio_eof_mp3(const char *info){ //end of file
|
||||
//Serial.printf("file :%s\n", info);
|
||||
if(timefile>0){
|
||||
if(timefile==1){audio.connecttoFS(SD, "/voice_time/080.mp3"); timefile--;} // stroke
|
||||
if(timefile==2){audio.connecttoFS(SD, "/voice_time/200.mp3"); timefile--;} // precisely
|
||||
if(timefile==3){audio.connecttoFS(SD, "/voice_time/O'clock.mp3"); timefile--;}
|
||||
}
|
||||
}
|
||||
|
BIN
lib/ESP32-audioI2S-3.0.0/examples/TalkingClock/voice_time.zip
Normal file
@ -0,0 +1,161 @@
|
||||
#include "Arduino.h"
|
||||
#include "WiFi.h"
|
||||
#include "Audio.h"
|
||||
|
||||
// Digital I/O used
|
||||
#define I2S_DOUT 25
|
||||
#define I2S_BCLK 27
|
||||
#define I2S_LRC 26
|
||||
|
||||
Audio audio;
|
||||
|
||||
String ssid = "******";
|
||||
String password = "******";
|
||||
|
||||
//****************************************************************************************
|
||||
// A U D I O _ T A S K *
|
||||
//****************************************************************************************
|
||||
|
||||
struct audioMessage{
|
||||
uint8_t cmd;
|
||||
const char* txt;
|
||||
uint32_t value;
|
||||
uint32_t ret;
|
||||
} audioTxMessage, audioRxMessage;
|
||||
|
||||
enum : uint8_t { SET_VOLUME, GET_VOLUME, CONNECTTOHOST, CONNECTTOSD };
|
||||
|
||||
QueueHandle_t audioSetQueue = NULL;
|
||||
QueueHandle_t audioGetQueue = NULL;
|
||||
|
||||
void CreateQueues(){
|
||||
audioSetQueue = xQueueCreate(10, sizeof(struct audioMessage));
|
||||
audioGetQueue = xQueueCreate(10, sizeof(struct audioMessage));
|
||||
}
|
||||
|
||||
void audioTask(void *parameter) {
|
||||
CreateQueues();
|
||||
if(!audioSetQueue || !audioGetQueue){
|
||||
log_e("queues are not initialized");
|
||||
while(true){;} // endless loop
|
||||
}
|
||||
|
||||
struct audioMessage audioRxTaskMessage;
|
||||
struct audioMessage audioTxTaskMessage;
|
||||
|
||||
audio.setPinout(I2S_BCLK, I2S_LRC, I2S_DOUT);
|
||||
audio.setVolume(15); // 0...21
|
||||
|
||||
while(true){
|
||||
if(xQueueReceive(audioSetQueue, &audioRxTaskMessage, 1) == pdPASS) {
|
||||
if(audioRxTaskMessage.cmd == SET_VOLUME){
|
||||
audioTxTaskMessage.cmd = SET_VOLUME;
|
||||
audio.setVolume(audioRxTaskMessage.value);
|
||||
audioTxTaskMessage.ret = 1;
|
||||
xQueueSend(audioGetQueue, &audioTxTaskMessage, portMAX_DELAY);
|
||||
}
|
||||
else if(audioRxTaskMessage.cmd == CONNECTTOHOST){
|
||||
audioTxTaskMessage.cmd = CONNECTTOHOST;
|
||||
audioTxTaskMessage.ret = audio.connecttohost(audioRxTaskMessage.txt);
|
||||
xQueueSend(audioGetQueue, &audioTxTaskMessage, portMAX_DELAY);
|
||||
}
|
||||
else if(audioRxTaskMessage.cmd == CONNECTTOSD){
|
||||
audioTxTaskMessage.cmd = CONNECTTOSD;
|
||||
audioTxTaskMessage.ret = audio.connecttoSD(audioRxTaskMessage.txt);
|
||||
xQueueSend(audioGetQueue, &audioTxTaskMessage, portMAX_DELAY);
|
||||
}
|
||||
else if(audioRxTaskMessage.cmd == GET_VOLUME){
|
||||
audioTxTaskMessage.cmd = GET_VOLUME;
|
||||
audioTxTaskMessage.ret = audio.getVolume();
|
||||
xQueueSend(audioGetQueue, &audioTxTaskMessage, portMAX_DELAY);
|
||||
}
|
||||
else{
|
||||
log_i("error");
|
||||
}
|
||||
}
|
||||
audio.loop();
|
||||
if (!audio.isRunning()) {
|
||||
sleep(1);
|
||||
}
|
||||
}
|
||||
}
|
||||
|
||||
void audioInit() {
|
||||
xTaskCreatePinnedToCore(
|
||||
audioTask, /* Function to implement the task */
|
||||
"audioplay", /* Name of the task */
|
||||
5000, /* Stack size in words */
|
||||
NULL, /* Task input parameter */
|
||||
2 | portPRIVILEGE_BIT, /* Priority of the task */
|
||||
NULL, /* Task handle. */
|
||||
1 /* Core where the task should run */
|
||||
);
|
||||
}
|
||||
|
||||
audioMessage transmitReceive(audioMessage msg){
|
||||
xQueueSend(audioSetQueue, &msg, portMAX_DELAY);
|
||||
if(xQueueReceive(audioGetQueue, &audioRxMessage, portMAX_DELAY) == pdPASS){
|
||||
if(msg.cmd != audioRxMessage.cmd){
|
||||
log_e("wrong reply from message queue");
|
||||
}
|
||||
}
|
||||
return audioRxMessage;
|
||||
}
|
||||
|
||||
void audioSetVolume(uint8_t vol){
|
||||
audioTxMessage.cmd = SET_VOLUME;
|
||||
audioTxMessage.value = vol;
|
||||
audioMessage RX = transmitReceive(audioTxMessage);
|
||||
}
|
||||
|
||||
uint8_t audioGetVolume(){
|
||||
audioTxMessage.cmd = GET_VOLUME;
|
||||
audioMessage RX = transmitReceive(audioTxMessage);
|
||||
return RX.ret;
|
||||
}
|
||||
|
||||
bool audioConnecttohost(const char* host){
|
||||
audioTxMessage.cmd = CONNECTTOHOST;
|
||||
audioTxMessage.txt = host;
|
||||
audioMessage RX = transmitReceive(audioTxMessage);
|
||||
return RX.ret;
|
||||
}
|
||||
|
||||
bool audioConnecttoSD(const char* filename){
|
||||
audioTxMessage.cmd = CONNECTTOSD;
|
||||
audioTxMessage.txt = filename;
|
||||
audioMessage RX = transmitReceive(audioTxMessage);
|
||||
return RX.ret;
|
||||
}
|
||||
|
||||
//****************************************************************************************
|
||||
// S E T U P *
|
||||
//****************************************************************************************
|
||||
|
||||
void setup() {
|
||||
Serial.begin(115200);
|
||||
WiFi.begin(ssid.c_str(), password.c_str());
|
||||
while (WiFi.status() != WL_CONNECTED) delay(1500);
|
||||
|
||||
audioInit();
|
||||
|
||||
audioConnecttohost("http://mp3.ffh.de/radioffh/hqlivestream.mp3");
|
||||
audioSetVolume(15);
|
||||
log_i("current volume is: %d", audioGetVolume());
|
||||
}
|
||||
|
||||
//****************************************************************************************
|
||||
// L O O P *
|
||||
//****************************************************************************************
|
||||
|
||||
void loop(){
|
||||
// your own code here
|
||||
}
|
||||
//*****************************************************************************************
|
||||
// E V E N T S *
|
||||
//*****************************************************************************************
|
||||
|
||||
void audio_info(const char *info){
|
||||
Serial.print("info "); Serial.println(info);
|
||||
}
|
||||
|
90
lib/ESP32-audioI2S-3.0.0/examples/time_announcement/main.cpp
Normal file
@ -0,0 +1,90 @@
|
||||
// This arduino sketch for ESP32 announces the time every hour.
|
||||
// A connection to the Internet is required
|
||||
// 1) to synchronize with the internal RTC on startup
|
||||
// 2) so that GoogleTTS can be reached
|
||||
|
||||
|
||||
|
||||
#include <Arduino.h>
|
||||
#include "WiFiMulti.h"
|
||||
#include "Audio.h"
|
||||
#include "time.h"
|
||||
#include "esp_sntp.h"
|
||||
|
||||
//------------------------USER SETTINGS / GPIOs-------------------------------------------------------------------------
|
||||
String ssid = "xxxx";
|
||||
String password = "xxxx";
|
||||
uint8_t I2S_BCLK = 27;
|
||||
uint8_t I2S_LRC = 26;
|
||||
uint8_t I2S_DOUT = 25;
|
||||
//------------------------OBJECTS /GLOBAL VARS--------------------------------------------------------------------------
|
||||
Audio audio(false, 3, 0);
|
||||
WiFiMulti wifiMulti;
|
||||
uint32_t sec1 = millis();
|
||||
String time_s = "";
|
||||
char chbuf[200];
|
||||
int timeIdx = 0;
|
||||
//------------------------TIME / SNTP STUFF-----------------------------------------------------------------------------
|
||||
#define TZName "CET-1CEST,M3.5.0,M10.5.0/3" // https://remotemonitoringsystems.ca/time-zone-abbreviations.php
|
||||
char strftime_buf[64];
|
||||
struct tm timeinfo;
|
||||
time_t now;
|
||||
boolean obtain_time(){
|
||||
time_t now = 0;
|
||||
int retry = 0;
|
||||
Serial.println("Initializing SNTP");
|
||||
sntp_setoperatingmode(SNTP_OPMODE_POLL);
|
||||
sntp_setservername(0, "pool.ntp.org");
|
||||
sntp_init();
|
||||
|
||||
const int retry_count = 10;
|
||||
while(timeinfo.tm_year < (2016 - 1900) && ++retry < retry_count) {
|
||||
Serial.printf("Waiting for system time to be set... (%d/%d)\n", retry, retry_count);
|
||||
vTaskDelay(uint16_t(2000 / portTICK_PERIOD_MS));
|
||||
time(&now);
|
||||
localtime_r(&now, &timeinfo);
|
||||
}
|
||||
setenv("TZ", TZName, 1);
|
||||
tzset();
|
||||
localtime_r(&now, &timeinfo);
|
||||
strftime(strftime_buf, sizeof(strftime_buf), "%c", &timeinfo);
|
||||
if(retry < retry_count) return true;
|
||||
else return false;
|
||||
}
|
||||
|
||||
const char* gettime_s(){ // hh:mm:ss
|
||||
time(&now);
|
||||
localtime_r(&now, &timeinfo);
|
||||
sprintf(strftime_buf,"%02d:%02d:%02d", timeinfo.tm_hour, timeinfo.tm_min, timeinfo.tm_sec);
|
||||
return strftime_buf;
|
||||
}
|
||||
//-----------------------SETUP------------------------------------------------------------------------------------------
|
||||
void setup() {
|
||||
Serial.begin(115200);
|
||||
wifiMulti.addAP(ssid.c_str(), password.c_str());
|
||||
wifiMulti.run();
|
||||
obtain_time();
|
||||
audio.setPinout(I2S_BCLK, I2S_LRC, I2S_DOUT);
|
||||
audio.setVolume(15);
|
||||
}
|
||||
//-----------------------LOOP-------------------------------------------------------------------------------------------
|
||||
void loop() {
|
||||
audio.loop();
|
||||
if(sec1 < millis()){ // every second
|
||||
sec1 = millis() + 1000;
|
||||
time_s = gettime_s();
|
||||
Serial.println(time_s);
|
||||
if(time_s.endsWith("00:00")){ // time announcement every full hour
|
||||
char am_pm[5] = "am.";
|
||||
int h = time_s.substring(0,2).toInt();
|
||||
if(h > 12){h -= 12; strcpy(am_pm,"pm.");}
|
||||
sprintf(chbuf, "It is now %i%s and %i minutes", h, am_pm, time_s.substring(3,5).toInt());
|
||||
Serial.println(chbuf);
|
||||
audio.connecttospeech(chbuf, "en");
|
||||
}
|
||||
}
|
||||
}
|
||||
//------------------EVENTS----------------------------------------------------------------------------------------------
|
||||
void audio_info(const char *info){
|
||||
Serial.printf("info: %s\n", info);
|
||||
}
|
60
lib/ESP32-audioI2S-3.0.0/keywords.txt
Normal file
@ -0,0 +1,60 @@
|
||||
#######################################
|
||||
# Syntax Coloring Map For Ultrasound
|
||||
#######################################
|
||||
|
||||
#######################################
|
||||
# Datatypes (KEYWORD1)
|
||||
#######################################
|
||||
|
||||
Audio KEYWORD1
|
||||
|
||||
#######################################
|
||||
# Methods and Functions (KEYWORD2)
|
||||
#######################################
|
||||
|
||||
audio_bitrate KEYWORD2
|
||||
audio_commercial KEYWORD2
|
||||
audio_eof_mp3 KEYWORD2
|
||||
audio_eof_speech KEYWORD2
|
||||
audio_icyurl KEYWORD2
|
||||
audio_info KEYWORD2
|
||||
audio_id3data KEYWORD2
|
||||
audio_lasthost KEYWORD2
|
||||
audio_showstreamtitle KEYWORD2
|
||||
audio_showstation KEYWORD2
|
||||
audio_eof_stream KEYWORD2
|
||||
audio_id3image KEYWORD2
|
||||
connecttoSD KEYWORD2
|
||||
connecttoFS KEYWORD2
|
||||
connecttohost KEYWORD2
|
||||
getAudioCurrentTime KEYWORD2
|
||||
getAudioFileDuration KEYWORD2
|
||||
getDatamode KEYWORD2
|
||||
getFilePos KEYWORD2
|
||||
getFileSize KEYWORD2
|
||||
getVolume KEYWORD2
|
||||
loop KEYWORD2
|
||||
pauseResume KEYWORD2
|
||||
setDatamode KEYWORD2
|
||||
setFilePos KEYWORD2
|
||||
setPinout KEYWORD2
|
||||
setVolume KEYWORD2
|
||||
stopSong KEYWORD2
|
||||
streamavail KEYWORD2
|
||||
isRunning KEYWORD2
|
||||
inBufferFilled KEYWORD2
|
||||
inBufferFree KEYWORD2
|
||||
i2s_mclk_pin_select KEYWORD2
|
||||
setFileLoop KEYWORD2
|
||||
setTone KEYWORD2
|
||||
setBalance KEYWORD2
|
||||
forceMono KEYWORD2
|
||||
setInternalDAC KEYWORD2
|
||||
setI2SCommFMT_LSB KEYWORD2
|
||||
setTimeOffset KEYWORD2
|
||||
|
||||
|
||||
|
||||
#######################################
|
||||
# Constants (LITERAL1)
|
||||
#######################################
|
9
lib/ESP32-audioI2S-3.0.0/library.properties
Normal file
@ -0,0 +1,9 @@
|
||||
name=ESP32-audioI2S-master
|
||||
version=2.0.0
|
||||
author=schreibfaul1
|
||||
maintainer=schreibfaul1
|
||||
sentence=With this library You can easily build a WebRadio with a ESP32 board and a I2S-module.
|
||||
paragraph=Plays webradio, playlists can be m3u, pls or asx. Data format can be only mp3, aac, flac or m4a. It can also play files from a SD Card.
|
||||
category=Device Control
|
||||
url=https://github.com/schreibfaul1/ESP32-audioI2S
|
||||
architectures=esp32
|
5319
lib/ESP32-audioI2S-3.0.0/src/Audio.cpp
Normal file
575
lib/ESP32-audioI2S-3.0.0/src/Audio.h
Normal file
@ -0,0 +1,575 @@
|
||||
/*
|
||||
* Audio.h
|
||||
*
|
||||
* Created on: Oct 28,2018
|
||||
*
|
||||
* Version 2.0.8c
|
||||
* Updated on: Jan 13,2023
|
||||
* Author: Wolle (schreibfaul1)
|
||||
*/
|
||||
|
||||
//#define SDFATFS_USED // activate for SdFat
|
||||
|
||||
|
||||
#pragma once
|
||||
#pragma GCC optimize ("Ofast")
|
||||
#include <vector>
|
||||
#include <Arduino.h>
|
||||
#include <libb64/cencode.h>
|
||||
#include <esp32-hal-log.h>
|
||||
|
||||
#include <SPI.h>
|
||||
#include <WiFi.h>
|
||||
#include <WiFiClientSecure.h>
|
||||
#include <vector>
|
||||
#include <driver/i2s.h>
|
||||
|
||||
#ifdef SDFATFS_USED
|
||||
#include <SdFat.h> // https://github.com/greiman/SdFat
|
||||
#else
|
||||
#include <SD.h>
|
||||
#include <SD_MMC.h>
|
||||
#include <SPIFFS.h>
|
||||
#include <FS.h>
|
||||
#include <FFat.h>
|
||||
#endif // SDFATFS_USED
|
||||
|
||||
|
||||
#ifdef SDFATFS_USED
|
||||
//typedef File32 File;
|
||||
typedef FsFile File;
|
||||
|
||||
namespace fs {
|
||||
class FS : public SdFat {
|
||||
public:
|
||||
bool begin(SdCsPin_t csPin = SS, uint32_t maxSck = SD_SCK_MHZ(25)) { return SdFat::begin(csPin, maxSck); }
|
||||
};
|
||||
|
||||
class SDFATFS : public fs::FS {
|
||||
public:
|
||||
// sdcard_type_t cardType();
|
||||
uint64_t cardSize() {
|
||||
return totalBytes();
|
||||
}
|
||||
uint64_t usedBytes() {
|
||||
// set SdFatConfig MAINTAIN_FREE_CLUSTER_COUNT non-zero. Then only the first call will take time.
|
||||
return (uint64_t)(clusterCount() - freeClusterCount()) * (uint64_t)bytesPerCluster();
|
||||
}
|
||||
uint64_t totalBytes() {
|
||||
return (uint64_t)clusterCount() * (uint64_t)bytesPerCluster();
|
||||
}
|
||||
};
|
||||
}
|
||||
|
||||
extern fs::SDFATFS SD_SDFAT;
|
||||
|
||||
using namespace fs;
|
||||
#define SD SD_SDFAT
|
||||
#endif //SDFATFS_USED
|
||||
|
||||
using namespace std;
|
||||
|
||||
|
||||
extern __attribute__((weak)) void audio_info(const char*);
|
||||
extern __attribute__((weak)) void audio_id3data(const char*); //ID3 metadata
|
||||
extern __attribute__((weak)) void audio_id3image(File& file, const size_t pos, const size_t size); //ID3 metadata image
|
||||
extern __attribute__((weak)) void audio_eof_mp3(const char*); //end of mp3 file
|
||||
extern __attribute__((weak)) void audio_showstreamtitle(const char*);
|
||||
extern __attribute__((weak)) void audio_showstation(const char*);
|
||||
extern __attribute__((weak)) void audio_bitrate(const char*);
|
||||
extern __attribute__((weak)) void audio_commercial(const char*);
|
||||
extern __attribute__((weak)) void audio_icyurl(const char*);
|
||||
extern __attribute__((weak)) void audio_icydescription(const char*);
|
||||
extern __attribute__((weak)) void audio_lasthost(const char*);
|
||||
extern __attribute__((weak)) void audio_eof_speech(const char*);
|
||||
extern __attribute__((weak)) void audio_eof_stream(const char*); // The webstream comes to an end
|
||||
extern __attribute__((weak)) void audio_process_extern(int16_t* buff, uint16_t len, bool *continueI2S); // record audiodata or send via BT
|
||||
extern __attribute__((weak)) void audio_process_i2s(uint32_t* sample, bool *continueI2S); // record audiodata or send via BT
|
||||
|
||||
#define AUDIO_INFO(...) {char buff[512 + 64]; sprintf(buff,__VA_ARGS__); if(audio_info) audio_info(buff);}
|
||||
|
||||
//----------------------------------------------------------------------------------------------------------------------
|
||||
|
||||
class AudioBuffer {
|
||||
// AudioBuffer will be allocated in PSRAM, If PSRAM not available or has not enough space AudioBuffer will be
|
||||
// allocated in FlashRAM with reduced size
|
||||
//
|
||||
// m_buffer m_readPtr m_writePtr m_endPtr
|
||||
// | |<------dataLength------->|<------ writeSpace ----->|
|
||||
// ▼ ▼ ▼ ▼
|
||||
// ---------------------------------------------------------------------------------------------------------------
|
||||
// | <--m_buffSize--> | <--m_resBuffSize --> |
|
||||
// ---------------------------------------------------------------------------------------------------------------
|
||||
// |<-----freeSpace------->| |<------freeSpace-------->|
|
||||
//
|
||||
//
|
||||
//
|
||||
// if the space between m_readPtr and buffend < m_resBuffSize copy data from the beginning to resBuff
|
||||
// so that the mp3/aac/flac frame is always completed
|
||||
//
|
||||
// m_buffer m_writePtr m_readPtr m_endPtr
|
||||
// | |<-------writeSpace------>|<--dataLength-->|
|
||||
// ▼ ▼ ▼ ▼
|
||||
// ---------------------------------------------------------------------------------------------------------------
|
||||
// | <--m_buffSize--> | <--m_resBuffSize --> |
|
||||
// ---------------------------------------------------------------------------------------------------------------
|
||||
// |<--- ------dataLength-- ------>|<-------freeSpace------->|
|
||||
//
|
||||
//
|
||||
|
||||
public:
|
||||
AudioBuffer(size_t maxBlockSize = 0); // constructor
|
||||
~AudioBuffer(); // frees the buffer
|
||||
size_t init(); // set default values
|
||||
bool isInitialized() { return m_f_init; };
|
||||
void setBufsize(int ram, int psram);
|
||||
void changeMaxBlockSize(uint16_t mbs); // is default 1600 for mp3 and aac, set 16384 for FLAC
|
||||
uint16_t getMaxBlockSize(); // returns maxBlockSize
|
||||
size_t freeSpace(); // number of free bytes to overwrite
|
||||
size_t writeSpace(); // space fom writepointer to bufferend
|
||||
size_t bufferFilled(); // returns the number of filled bytes
|
||||
void bytesWritten(size_t bw); // update writepointer
|
||||
void bytesWasRead(size_t br); // update readpointer
|
||||
uint8_t* getWritePtr(); // returns the current writepointer
|
||||
uint8_t* getReadPtr(); // returns the current readpointer
|
||||
uint32_t getWritePos(); // write position relative to the beginning
|
||||
uint32_t getReadPos(); // read position relative to the beginning
|
||||
void resetBuffer(); // restore defaults
|
||||
bool havePSRAM() { return m_f_psram; };
|
||||
|
||||
protected:
|
||||
size_t m_buffSizePSRAM = 300000; // most webstreams limit the advance to 100...300Kbytes
|
||||
size_t m_buffSizeRAM = 1600 * 5;
|
||||
size_t m_buffSize = 0;
|
||||
size_t m_freeSpace = 0;
|
||||
size_t m_writeSpace = 0;
|
||||
size_t m_dataLength = 0;
|
||||
size_t m_resBuffSizeRAM = 1600; // reserved buffspace, >= one mp3 frame
|
||||
size_t m_resBuffSizePSRAM = 4096 * 4; // reserved buffspace, >= one flac frame
|
||||
size_t m_maxBlockSize = 1600;
|
||||
uint8_t* m_buffer = NULL;
|
||||
uint8_t* m_writePtr = NULL;
|
||||
uint8_t* m_readPtr = NULL;
|
||||
uint8_t* m_endPtr = NULL;
|
||||
bool m_f_start = true;
|
||||
bool m_f_init = false;
|
||||
bool m_f_psram = false; // PSRAM is available (and used...)
|
||||
};
|
||||
//----------------------------------------------------------------------------------------------------------------------
|
||||
|
||||
class Audio : private AudioBuffer{
|
||||
|
||||
AudioBuffer InBuff; // instance of input buffer
|
||||
|
||||
public:
|
||||
Audio(bool internalDAC = false, uint8_t channelEnabled = 3, uint8_t i2sPort = I2S_NUM_0); // #99
|
||||
~Audio();
|
||||
void setBufsize(int rambuf_sz, int psrambuf_sz);
|
||||
bool connecttohost(const char* host, const char* user = "", const char* pwd = "");
|
||||
bool connecttospeech(const char* speech, const char* lang);
|
||||
bool connecttomarytts(const char* speech, const char* lang, const char* voice);
|
||||
bool connecttoFS(fs::FS &fs, const char* path, uint32_t resumeFilePos = 0);
|
||||
bool connecttoSD(const char* path, uint32_t resumeFilePos = 0);
|
||||
bool setFileLoop(bool input);//TEST loop
|
||||
void setConnectionTimeout(uint16_t timeout_ms, uint16_t timeout_ms_ssl);
|
||||
bool setAudioPlayPosition(uint16_t sec);
|
||||
bool setFilePos(uint32_t pos);
|
||||
bool audioFileSeek(const float speed);
|
||||
bool setTimeOffset(int sec);
|
||||
bool setPinout(uint8_t BCLK, uint8_t LRC, uint8_t DOUT, int8_t DIN = I2S_PIN_NO_CHANGE, int8_t MCK = I2S_PIN_NO_CHANGE);
|
||||
bool pauseResume();
|
||||
bool isRunning() {return m_f_running;}
|
||||
void loop();
|
||||
uint32_t stopSong();
|
||||
void forceMono(bool m);
|
||||
void setBalance(int8_t bal = 0);
|
||||
void setVolumeSteps(uint8_t steps);
|
||||
void setVolume(uint8_t vol);
|
||||
uint8_t getVolume();
|
||||
uint8_t maxVolume();
|
||||
uint8_t getI2sPort();
|
||||
|
||||
uint32_t getAudioDataStartPos();
|
||||
uint32_t getFileSize();
|
||||
uint32_t getFilePos();
|
||||
uint32_t getSampleRate();
|
||||
uint8_t getBitsPerSample();
|
||||
uint8_t getChannels();
|
||||
uint32_t getBitRate(bool avg = false);
|
||||
uint32_t getAudioFileDuration();
|
||||
uint32_t getAudioCurrentTime();
|
||||
uint32_t getTotalPlayingTime();
|
||||
|
||||
esp_err_t i2s_mclk_pin_select(const uint8_t pin);
|
||||
uint32_t inBufferFilled(); // returns the number of stored bytes in the inputbuffer
|
||||
uint32_t inBufferFree(); // returns the number of free bytes in the inputbuffer
|
||||
void setTone(int8_t gainLowPass, int8_t gainBandPass, int8_t gainHighPass);
|
||||
void setI2SCommFMT_LSB(bool commFMT);
|
||||
int getCodec() {return m_codec;}
|
||||
const char *getCodecname() {return codecname[m_codec];}
|
||||
|
||||
private:
|
||||
|
||||
#ifndef ESP_ARDUINO_VERSION_VAL
|
||||
#define ESP_ARDUINO_VERSION_MAJOR 0
|
||||
#define ESP_ARDUINO_VERSION_MINOR 0
|
||||
#define ESP_ARDUINO_VERSION_PATCH 0
|
||||
#endif
|
||||
|
||||
void UTF8toASCII(char* str);
|
||||
bool latinToUTF8(char* buff, size_t bufflen);
|
||||
void setDefaults(); // free buffers and set defaults
|
||||
void initInBuff();
|
||||
bool httpPrint(const char* host);
|
||||
void processLocalFile();
|
||||
void processWebStream();
|
||||
void processWebFile();
|
||||
void processWebStreamTS();
|
||||
void processWebStreamHLS();
|
||||
void playAudioData();
|
||||
bool readPlayListData();
|
||||
const char* parsePlaylist_M3U();
|
||||
const char* parsePlaylist_PLS();
|
||||
const char* parsePlaylist_ASX();
|
||||
const char* parsePlaylist_M3U8();
|
||||
bool STfromEXTINF(char* str);
|
||||
void showCodecParams();
|
||||
int findNextSync(uint8_t* data, size_t len);
|
||||
int sendBytes(uint8_t* data, size_t len);
|
||||
void setDecoderItems();
|
||||
void compute_audioCurrentTime(int bd);
|
||||
void printDecodeError(int r);
|
||||
void showID3Tag(const char* tag, const char* val);
|
||||
void unicode2utf8(char* buff, uint32_t len);
|
||||
size_t readAudioHeader(uint32_t bytes);
|
||||
int read_WAV_Header(uint8_t* data, size_t len);
|
||||
int read_FLAC_Header(uint8_t *data, size_t len);
|
||||
int read_ID3_Header(uint8_t* data, size_t len);
|
||||
int read_M4A_Header(uint8_t* data, size_t len);
|
||||
size_t process_m3u8_ID3_Header(uint8_t* packet);
|
||||
bool setSampleRate(uint32_t hz);
|
||||
bool setBitsPerSample(int bits);
|
||||
bool setChannels(int channels);
|
||||
bool setBitrate(int br);
|
||||
bool playChunk();
|
||||
bool playSample(int16_t sample[2]) ;
|
||||
int32_t Gain(int16_t s[2]);
|
||||
void showstreamtitle(const char* ml);
|
||||
bool parseContentType(char* ct);
|
||||
bool parseHttpResponseHeader();
|
||||
bool initializeDecoder();
|
||||
esp_err_t I2Sstart(uint8_t i2s_num);
|
||||
esp_err_t I2Sstop(uint8_t i2s_num);
|
||||
void urlencode(char* buff, uint16_t buffLen, bool spacesOnly = false);
|
||||
int16_t* IIR_filterChain0(int16_t iir_in[2], bool clear = false);
|
||||
int16_t* IIR_filterChain1(int16_t* iir_in, bool clear = false);
|
||||
int16_t* IIR_filterChain2(int16_t* iir_in, bool clear = false);
|
||||
inline void setDatamode(uint8_t dm){m_datamode=dm;}
|
||||
inline uint8_t getDatamode(){return m_datamode;}
|
||||
inline uint32_t streamavail(){ return _client ? _client->available() : 0;}
|
||||
void IIR_calculateCoefficients(int8_t G1, int8_t G2, int8_t G3);
|
||||
bool ts_parsePacket(uint8_t* packet, uint8_t* packetStart, uint8_t* packetLength);
|
||||
|
||||
//+++ W E B S T R E A M - H E L P F U N C T I O N S +++
|
||||
uint16_t readMetadata(uint16_t b, bool first = false);
|
||||
size_t chunkedDataTransfer(uint8_t* bytes);
|
||||
bool readID3V1Tag();
|
||||
boolean streamDetection(uint32_t bytesAvail);
|
||||
void seek_m4a_stsz();
|
||||
void seek_m4a_ilst();
|
||||
uint32_t m4a_correctResumeFilePos(uint32_t resumeFilePos);
|
||||
uint32_t flac_correctResumeFilePos(uint32_t resumeFilePos);
|
||||
uint32_t mp3_correctResumeFilePos(uint32_t resumeFilePos);
|
||||
uint8_t determineOggCodec(uint8_t* data, uint16_t len);
|
||||
|
||||
|
||||
//++++ implement several function with respect to the index of string ++++
|
||||
void trim(char *s) {
|
||||
//fb trim in place
|
||||
char *pe;
|
||||
char *p = s;
|
||||
while ( isspace(*p) ) p++; //left
|
||||
pe = p; //right
|
||||
while ( *pe != '\0' ) pe++;
|
||||
do {
|
||||
pe--;
|
||||
} while ( (pe > p) && isspace(*pe) );
|
||||
if (p == s) {
|
||||
*++pe = '\0';
|
||||
} else { //move
|
||||
while ( p <= pe ) *s++ = *p++;
|
||||
*s = '\0';
|
||||
}
|
||||
}
|
||||
|
||||
bool startsWith (const char* base, const char* str) {
|
||||
//fb
|
||||
char c;
|
||||
while ( (c = *str++) != '\0' )
|
||||
if (c != *base++) return false;
|
||||
return true;
|
||||
}
|
||||
|
||||
bool endsWith (const char* base, const char* str) {
|
||||
//fb
|
||||
int slen = strlen(str) - 1;
|
||||
const char *p = base + strlen(base) - 1;
|
||||
while(p > base && isspace(*p)) p--; // rtrim
|
||||
p -= slen;
|
||||
if (p < base) return false;
|
||||
return (strncmp(p, str, slen) == 0);
|
||||
}
|
||||
|
||||
int indexOf (const char* base, const char* str, int startIndex = 0) {
|
||||
//fb
|
||||
const char *p = base;
|
||||
for (; startIndex > 0; startIndex--)
|
||||
if (*p++ == '\0') return -1;
|
||||
char* pos = strstr(p, str);
|
||||
if (pos == nullptr) return -1;
|
||||
return pos - base;
|
||||
}
|
||||
|
||||
int indexOf (const char* base, char ch, int startIndex = 0) {
|
||||
//fb
|
||||
const char *p = base;
|
||||
for (; startIndex > 0; startIndex--)
|
||||
if (*p++ == '\0') return -1;
|
||||
char *pos = strchr(p, ch);
|
||||
if (pos == nullptr) return -1;
|
||||
return pos - base;
|
||||
}
|
||||
|
||||
int lastIndexOf(const char* haystack, const char* needle) {
|
||||
//fb
|
||||
int nlen = strlen(needle);
|
||||
if (nlen == 0) return -1;
|
||||
const char *p = haystack - nlen + strlen(haystack);
|
||||
while (p >= haystack) {
|
||||
int i = 0;
|
||||
while (needle[i] == p[i])
|
||||
if (++i == nlen) return p - haystack;
|
||||
p--;
|
||||
}
|
||||
return -1;
|
||||
}
|
||||
|
||||
int lastIndexOf(const char* haystack, const char needle) {
|
||||
//fb
|
||||
const char *p = strrchr(haystack, needle);
|
||||
return (p ? p - haystack : -1);
|
||||
}
|
||||
|
||||
int specialIndexOf (uint8_t* base, const char* str, int baselen, bool exact = false){
|
||||
int result; // seek for str in buffer or in header up to baselen, not nullterninated
|
||||
if (strlen(str) > baselen) return -1; // if exact == true seekstr in buffer must have "\0" at the end
|
||||
for (int i = 0; i < baselen - strlen(str); i++){
|
||||
result = i;
|
||||
for (int j = 0; j < strlen(str) + exact; j++){
|
||||
if (*(base + i + j) != *(str + j)){
|
||||
result = -1;
|
||||
break;
|
||||
}
|
||||
}
|
||||
if (result >= 0) break;
|
||||
}
|
||||
return result;
|
||||
}
|
||||
|
||||
// some other functions
|
||||
size_t bigEndian(uint8_t* base, uint8_t numBytes, uint8_t shiftLeft = 8){
|
||||
uint64_t result = 0;
|
||||
if(numBytes < 1 || numBytes > 8) return 0;
|
||||
for (int i = 0; i < numBytes; i++) {
|
||||
result += *(base + i) << (numBytes -i - 1) * shiftLeft;
|
||||
}
|
||||
if(result > SIZE_MAX) {log_e("range overflow"); result = 0;} // overflow
|
||||
return (size_t)result;
|
||||
}
|
||||
|
||||
bool b64encode(const char* source, uint16_t sourceLength, char* dest){
|
||||
size_t size = base64_encode_expected_len(sourceLength) + 1;
|
||||
char * buffer = (char *) malloc(size);
|
||||
if(buffer) {
|
||||
base64_encodestate _state;
|
||||
base64_init_encodestate(&_state);
|
||||
int len = base64_encode_block(&source[0], sourceLength, &buffer[0], &_state);
|
||||
len = base64_encode_blockend((buffer + len), &_state);
|
||||
memcpy(dest, buffer, strlen(buffer));
|
||||
dest[strlen(buffer)] = '\0';
|
||||
free(buffer);
|
||||
return true;
|
||||
}
|
||||
return false;
|
||||
}
|
||||
size_t urlencode_expected_len(const char* source){
|
||||
size_t expectedLen = strlen(source);
|
||||
for(int i = 0; i < strlen(source); i++) {
|
||||
if(isalnum(source[i])){;}
|
||||
else expectedLen += 2;
|
||||
}
|
||||
return expectedLen;
|
||||
}
|
||||
void vector_clear_and_shrink(vector<char*>&vec){
|
||||
uint size = vec.size();
|
||||
for (int i = 0; i < size; i++) {
|
||||
if(vec[i]){
|
||||
free(vec[i]);
|
||||
vec[i] = NULL;
|
||||
}
|
||||
}
|
||||
vec.clear();
|
||||
vec.shrink_to_fit();
|
||||
}
|
||||
uint32_t simpleHash(const char* str){
|
||||
if(str == NULL) return 0;
|
||||
uint32_t hash = 0;
|
||||
for(int i=0; i<strlen(str); i++){
|
||||
if(str[i] < 32) continue; // ignore control sign
|
||||
hash += (str[i] - 31) * i * 32;
|
||||
}
|
||||
return hash;
|
||||
}
|
||||
|
||||
private:
|
||||
const char *codecname[9] = {"unknown", "WAV", "MP3", "AAC", "M4A", "FLAC"};
|
||||
enum : int { APLL_AUTO = -1, APLL_ENABLE = 1, APLL_DISABLE = 0 };
|
||||
enum : int { EXTERNAL_I2S = 0, INTERNAL_DAC = 1, INTERNAL_PDM = 2 };
|
||||
enum : int { FORMAT_NONE = 0, FORMAT_M3U = 1, FORMAT_PLS = 2, FORMAT_ASX = 3, FORMAT_M3U8 = 4};
|
||||
enum : int { AUDIO_NONE, HTTP_RESPONSE_HEADER, AUDIO_DATA, AUDIO_LOCALFILE,
|
||||
AUDIO_PLAYLISTINIT, AUDIO_PLAYLISTHEADER, AUDIO_PLAYLISTDATA};
|
||||
enum : int { FLAC_BEGIN = 0, FLAC_MAGIC = 1, FLAC_MBH =2, FLAC_SINFO = 3, FLAC_PADDING = 4, FLAC_APP = 5,
|
||||
FLAC_SEEK = 6, FLAC_VORBIS = 7, FLAC_CUESHEET = 8, FLAC_PICTURE = 9, FLAC_OKAY = 100};
|
||||
enum : int { M4A_BEGIN = 0, M4A_FTYP = 1, M4A_CHK = 2, M4A_MOOV = 3, M4A_FREE = 4, M4A_TRAK = 5, M4A_MDAT = 6,
|
||||
M4A_ILST = 7, M4A_MP4A = 8, M4A_AMRDY = 99, M4A_OKAY = 100};
|
||||
enum : int { CODEC_NONE = 0, CODEC_WAV = 1, CODEC_MP3 = 2, CODEC_AAC = 3, CODEC_M4A = 4, CODEC_FLAC = 5,
|
||||
CODEC_AACP = 6, CODEC_OPUS = 7, CODEC_OGG = 8};
|
||||
enum : int { ST_NONE = 0, ST_WEBFILE = 1, ST_WEBSTREAM = 2};
|
||||
typedef enum { LEFTCHANNEL=0, RIGHTCHANNEL=1 } SampleIndex;
|
||||
typedef enum { LOWSHELF = 0, PEAKEQ = 1, HIFGSHELF =2 } FilterType;
|
||||
|
||||
const uint8_t volumetable[22]={ 0, 1, 2, 3, 4 , 6 , 8, 10, 12, 14, 17,
|
||||
20, 23, 27, 30 ,34, 38, 43 ,48, 52, 58, 64}; //22 elements
|
||||
|
||||
typedef struct _filter{
|
||||
float a0;
|
||||
float a1;
|
||||
float a2;
|
||||
float b1;
|
||||
float b2;
|
||||
} filter_t;
|
||||
|
||||
typedef struct _pis_array{
|
||||
int number;
|
||||
int pids[4];
|
||||
} pid_array;
|
||||
|
||||
File audiofile; // @suppress("Abstract class cannot be instantiated")
|
||||
WiFiClient client; // @suppress("Abstract class cannot be instantiated")
|
||||
WiFiClientSecure clientsecure; // @suppress("Abstract class cannot be instantiated")
|
||||
WiFiClient* _client = nullptr;
|
||||
SemaphoreHandle_t mutex_audio;
|
||||
i2s_config_t m_i2s_config = {}; // stores values for I2S driver
|
||||
i2s_pin_config_t m_pin_config = {};
|
||||
std::vector<char*> m_playlistContent; // m3u8 playlist buffer
|
||||
std::vector<char*> m_playlistURL; // m3u8 streamURLs buffer
|
||||
std::vector<uint32_t> m_hashQueue;
|
||||
|
||||
const size_t m_frameSizeWav = 1024;
|
||||
const size_t m_frameSizeMP3 = 1600;
|
||||
const size_t m_frameSizeAAC = 1600;
|
||||
const size_t m_frameSizeFLAC = 4096 * 4;
|
||||
const size_t m_frameSizeOPUS = 1024;
|
||||
|
||||
static const uint8_t m_tsPacketSize = 188;
|
||||
static const uint8_t m_tsHeaderSize = 4;
|
||||
|
||||
char* m_chbuf = NULL;
|
||||
uint16_t m_chbufSize = 0; // will set in constructor (depending on PSRAM)
|
||||
char m_lastHost[512]; // Store the last URL to a webstream
|
||||
char* m_playlistBuff = NULL; // stores playlistdata
|
||||
const uint16_t m_plsBuffEntryLen = 256; // length of each entry in playlistBuff
|
||||
filter_t m_filter[3]; // digital filters
|
||||
int m_LFcount = 0; // Detection of end of header
|
||||
uint32_t m_sampleRate=16000;
|
||||
uint32_t m_bitRate=0; // current bitrate given fom decoder
|
||||
uint32_t m_avr_bitrate = 0; // average bitrate, median computed by VBR
|
||||
int m_readbytes = 0; // bytes read
|
||||
uint32_t m_metacount = 0; // counts down bytes between metadata
|
||||
int m_controlCounter = 0; // Status within readID3data() and readWaveHeader()
|
||||
int8_t m_balance = 0; // -16 (mute left) ... +16 (mute right)
|
||||
uint16_t m_vol=64; // volume
|
||||
uint8_t m_vol_steps = 0; // 0 == legacy 21 steps, > 0: number of volume steps
|
||||
int32_t m_vol_step_div = 64; // max of volumetable[]
|
||||
uint8_t m_bitsPerSample = 16; // bitsPerSample
|
||||
uint8_t m_channels = 2;
|
||||
uint8_t m_i2s_num = I2S_NUM_0; // I2S_NUM_0 or I2S_NUM_1
|
||||
uint8_t m_playlistFormat = 0; // M3U, PLS, ASX
|
||||
uint8_t m_codec = CODEC_NONE; //
|
||||
uint8_t m_expectedCodec = CODEC_NONE; // set in connecttohost (e.g. http://url.mp3 -> CODEC_MP3)
|
||||
uint8_t m_expectedPlsFmt = FORMAT_NONE; // set in connecttohost (e.g. streaming01.m3u) -> FORMAT_M3U)
|
||||
uint8_t m_filterType[2]; // lowpass, highpass
|
||||
uint8_t m_streamType = ST_NONE;
|
||||
uint8_t m_ID3Size = 0; // lengt of ID3frame - ID3header
|
||||
int16_t m_outBuff[2048*2]; // Interleaved L/R
|
||||
int16_t m_validSamples = 0;
|
||||
int16_t m_curSample = 0;
|
||||
uint16_t m_datamode = 0; // Statemaschine
|
||||
uint16_t m_streamTitleHash = 0; // remember streamtitle, ignore multiple occurence in metadata
|
||||
uint16_t m_timeout_ms = 250;
|
||||
uint16_t m_timeout_ms_ssl = 2700;
|
||||
uint8_t m_flacBitsPerSample = 0; // bps should be 16
|
||||
uint8_t m_flacNumChannels = 0; // can be read out in the FLAC file header
|
||||
uint32_t m_flacSampleRate = 0; // can be read out in the FLAC file header
|
||||
uint16_t m_flacMaxFrameSize = 0; // can be read out in the FLAC file header
|
||||
uint16_t m_flacMaxBlockSize = 0; // can be read out in the FLAC file header
|
||||
uint32_t m_flacTotalSamplesInStream = 0; // can be read out in the FLAC file header
|
||||
uint32_t m_metaint = 0; // Number of databytes between metadata
|
||||
uint32_t m_chunkcount = 0 ; // Counter for chunked transfer
|
||||
uint32_t m_t0 = 0; // store millis(), is needed for a small delay
|
||||
uint32_t m_contentlength = 0; // Stores the length if the stream comes from fileserver
|
||||
uint32_t m_bytesNotDecoded = 0; // pictures or something else that comes with the stream
|
||||
uint32_t m_PlayingStartTime = 0; // Stores the milliseconds after the start of the audio
|
||||
uint32_t m_resumeFilePos = 0; // the return value from stopSong() can be entered here
|
||||
uint16_t m_m3u8_targetDuration = 10; //
|
||||
uint32_t m_stsz_numEntries = 0; // num of entries inside stsz atom (uint32_t)
|
||||
uint32_t m_stsz_position = 0; // pos of stsz atom within file
|
||||
bool m_f_metadata = false; // assume stream without metadata
|
||||
bool m_f_unsync = false; // set within ID3 tag but not used
|
||||
bool m_f_exthdr = false; // ID3 extended header
|
||||
bool m_f_ssl = false;
|
||||
bool m_f_running = false;
|
||||
bool m_f_firstCall = false; // InitSequence for processWebstream and processLokalFile
|
||||
bool m_f_chunked = false ; // Station provides chunked transfer
|
||||
bool m_f_firstmetabyte = false; // True if first metabyte (counter)
|
||||
bool m_f_playing = false; // valid mp3 stream recognized
|
||||
bool m_f_tts = false; // text to speech
|
||||
bool m_f_loop = false; // Set if audio file should loop
|
||||
bool m_f_forceMono = false; // if true stereo -> mono
|
||||
bool m_f_internalDAC = false; // false: output vis I2S, true output via internal DAC
|
||||
bool m_f_rtsp = false; // set if RTSP is used (m3u8 stream)
|
||||
bool m_f_m3u8data = false; // used in processM3U8entries
|
||||
bool m_f_Log = false; // set in platformio.ini -DAUDIO_LOG and -DCORE_DEBUG_LEVEL=3 or 4
|
||||
bool m_f_continue = false; // next m3u8 chunk is available
|
||||
bool m_f_ts = true; // transport stream
|
||||
bool m_f_m4aID3dataAreRead = false; // has the m4a-ID3data already been read?
|
||||
uint8_t m_f_channelEnabled = 3; // internal DAC, both channels
|
||||
uint32_t m_audioFileDuration = 0;
|
||||
float m_audioCurrentTime = 0;
|
||||
uint32_t m_audioDataStart = 0; // in bytes
|
||||
size_t m_audioDataSize = 0; //
|
||||
float m_filterBuff[3][2][2][2]; // IIR filters memory for Audio DSP
|
||||
size_t m_i2s_bytesWritten = 0; // set in i2s_write() but not used
|
||||
size_t m_file_size = 0; // size of the file
|
||||
uint16_t m_filterFrequency[2];
|
||||
int8_t m_gain0 = 0; // cut or boost filters (EQ)
|
||||
int8_t m_gain1 = 0;
|
||||
int8_t m_gain2 = 0;
|
||||
|
||||
pid_array m_pidsOfPMT;
|
||||
int16_t m_pidOfAAC;
|
||||
uint8_t m_packetBuff[m_tsPacketSize];
|
||||
int16_t m_pesDataLength = 0;
|
||||
};
|
||||
|
||||
//----------------------------------------------------------------------------------------------------------------------
|
10220
lib/ESP32-audioI2S-3.0.0/src/aac_decoder/aac_decoder.cpp
Normal file
586
lib/ESP32-audioI2S-3.0.0/src/aac_decoder/aac_decoder.h
Normal file
@ -0,0 +1,586 @@
|
||||
// based on helix aac decoder
|
||||
#pragma once
|
||||
//#pragma GCC optimize ("O3")
|
||||
//#pragma GCC diagnostic ignored "-Wnarrowing"
|
||||
|
||||
#include "Arduino.h"
|
||||
|
||||
#define AAC_ENABLE_MPEG4
|
||||
|
||||
#if (defined CONFIG_IDF_TARGET_ESP32S3 && defined BOARD_HAS_PSRAM)
|
||||
#define AAC_ENABLE_SBR // needs additional 60KB DRAM,
|
||||
#endif
|
||||
|
||||
#define ASSERT(x) /* do nothing */
|
||||
|
||||
#ifndef MAX
|
||||
#define MAX(a,b) std::max(a,b)
|
||||
#endif
|
||||
|
||||
#ifndef MIN
|
||||
#define MIN(a,b) std::min(a,b)
|
||||
#endif
|
||||
|
||||
|
||||
/* AAC file format */
|
||||
enum {
|
||||
AAC_FF_Unknown = 0, /* should be 0 on init */
|
||||
AAC_FF_ADTS = 1,
|
||||
AAC_FF_ADIF = 2,
|
||||
AAC_FF_RAW = 3
|
||||
};
|
||||
|
||||
/* syntactic element type */
|
||||
enum {
|
||||
AAC_ID_INVALID = -1,
|
||||
AAC_ID_SCE = 0,
|
||||
AAC_ID_CPE = 1,
|
||||
AAC_ID_CCE = 2,
|
||||
AAC_ID_LFE = 3,
|
||||
AAC_ID_DSE = 4,
|
||||
AAC_ID_PCE = 5,
|
||||
AAC_ID_FIL = 6,
|
||||
AAC_ID_END = 7
|
||||
};
|
||||
|
||||
enum {
|
||||
ERR_AAC_NONE = 0,
|
||||
ERR_AAC_INDATA_UNDERFLOW = -1,
|
||||
ERR_AAC_NULL_POINTER = -2,
|
||||
ERR_AAC_INVALID_ADTS_HEADER = -3,
|
||||
ERR_AAC_INVALID_ADIF_HEADER = -4,
|
||||
ERR_AAC_INVALID_FRAME = -5,
|
||||
ERR_AAC_MPEG4_UNSUPPORTED = -6,
|
||||
ERR_AAC_CHANNEL_MAP = -7,
|
||||
ERR_AAC_SYNTAX_ELEMENT = -8,
|
||||
ERR_AAC_DEQUANT = -9,
|
||||
ERR_AAC_STEREO_PROCESS = -10,
|
||||
ERR_AAC_PNS = -11,
|
||||
ERR_AAC_SHORT_BLOCK_DEINT = -12,
|
||||
ERR_AAC_TNS = -13,
|
||||
ERR_AAC_IMDCT = -14,
|
||||
ERR_AAC_NCHANS_TOO_HIGH = -15,
|
||||
ERR_AAC_SBR_INIT = -16,
|
||||
ERR_AAC_SBR_BITSTREAM = -17,
|
||||
ERR_AAC_SBR_DATA = -18,
|
||||
ERR_AAC_SBR_PCM_FORMAT = -19,
|
||||
ERR_AAC_SBR_NCHANS_TOO_HIGH = -20,
|
||||
ERR_AAC_SBR_SINGLERATE_UNSUPPORTED = -21,
|
||||
ERR_AAC_RAWBLOCK_PARAMS = -22,
|
||||
ERR_AAC_UNKNOWN = -9999
|
||||
};
|
||||
|
||||
enum {
|
||||
SBR_GRID_FIXFIX = 0,
|
||||
SBR_GRID_FIXVAR = 1,
|
||||
SBR_GRID_VARFIX = 2,
|
||||
SBR_GRID_VARVAR = 3
|
||||
};
|
||||
|
||||
enum {
|
||||
HuffTabSBR_tEnv15 = 0,
|
||||
HuffTabSBR_fEnv15 = 1,
|
||||
HuffTabSBR_tEnv15b = 2,
|
||||
HuffTabSBR_fEnv15b = 3,
|
||||
HuffTabSBR_tEnv30 = 4,
|
||||
HuffTabSBR_fEnv30 = 5,
|
||||
HuffTabSBR_tEnv30b = 6,
|
||||
HuffTabSBR_fEnv30b = 7,
|
||||
HuffTabSBR_tNoise30 = 8,
|
||||
HuffTabSBR_fNoise30 = 5,
|
||||
HuffTabSBR_tNoise30b = 9,
|
||||
HuffTabSBR_fNoise30b = 7
|
||||
};
|
||||
|
||||
typedef struct _AACDecInfo_t {
|
||||
/* raw decoded data, before rounding to 16-bit PCM (for postprocessing such as SBR) */
|
||||
void *rawSampleBuf[2];
|
||||
int rawSampleBytes;
|
||||
int rawSampleFBits;
|
||||
/* fill data (can be used for processing SBR or other extensions) */
|
||||
uint8_t *fillBuf;
|
||||
int fillCount;
|
||||
int fillExtType;
|
||||
int prevBlockID; /* block information */
|
||||
int currBlockID;
|
||||
int currInstTag;
|
||||
int sbDeinterleaveReqd[2]; // [MAX_NCHANS_ELEM]
|
||||
int adtsBlocksLeft;
|
||||
int bitRate; /* user-accessible info */
|
||||
int nChans;
|
||||
int sampRate;
|
||||
float compressionRatio;
|
||||
int id; /* 0: MPEG-4, 1: MPEG2 */
|
||||
int profile; /* 0: Main profile, 1: LowComplexity (LC), 2: ScalableSamplingRate (SSR), 3: reserved */
|
||||
int format;
|
||||
int sbrEnabled;
|
||||
int tnsUsed;
|
||||
int pnsUsed;
|
||||
int frameCount;
|
||||
} AACDecInfo_t;
|
||||
|
||||
|
||||
typedef struct _aac_BitStreamInfo_t {
|
||||
uint8_t *bytePtr;
|
||||
uint32_t iCache;
|
||||
int cachedBits;
|
||||
int nBytes;
|
||||
} aac_BitStreamInfo_t;
|
||||
|
||||
typedef union _U64 {
|
||||
int64_t w64;
|
||||
struct {
|
||||
uint32_t lo32;
|
||||
signed int hi32;
|
||||
} r;
|
||||
} U64;
|
||||
|
||||
typedef struct _AACFrameInfo_t {
|
||||
int bitRate;
|
||||
int nChans;
|
||||
int sampRateCore;
|
||||
int sampRateOut;
|
||||
int bitsPerSample;
|
||||
int outputSamps;
|
||||
int profile;
|
||||
int tnsUsed;
|
||||
int pnsUsed;
|
||||
} AACFrameInfo_t;
|
||||
|
||||
typedef struct _HuffInfo_t {
|
||||
int maxBits; /* number of bits in longest codeword */
|
||||
uint8_t count[20]; /* count[MAX_HUFF_BITS] = number of codes with length i+1 bits */
|
||||
int offset; /* offset into symbol table */
|
||||
} HuffInfo_t;
|
||||
|
||||
typedef struct _PulseInfo_t {
|
||||
uint8_t pulseDataPresent;
|
||||
uint8_t numPulse;
|
||||
uint8_t startSFB;
|
||||
uint8_t offset[4]; // [MAX_PULSES]
|
||||
uint8_t amp[4]; // [MAX_PULSES]
|
||||
} PulseInfo_t;
|
||||
|
||||
typedef struct _TNSInfo_t {
|
||||
uint8_t tnsDataPresent;
|
||||
uint8_t numFilt[8]; // [MAX_TNS_FILTERS] max 1 filter each for 8 short windows, or 3 filters for 1 long window
|
||||
uint8_t coefRes[8]; // [MAX_TNS_FILTERS]
|
||||
uint8_t length[8]; // [MAX_TNS_FILTERS]
|
||||
uint8_t order[8]; // [MAX_TNS_FILTERS]
|
||||
uint8_t dir[8]; // [MAX_TNS_FILTERS]
|
||||
int8_t coef[60]; // [MAX_TNS_COEFS] max 3 filters * 20 coefs for 1 long window,
|
||||
// or 1 filter * 7 coefs for each of 8 short windows
|
||||
} TNSInfo_t;
|
||||
|
||||
typedef struct _GainControlInfo_t {
|
||||
uint8_t gainControlDataPresent;
|
||||
uint8_t maxBand;
|
||||
uint8_t adjNum[3][8]; // [MAX_GAIN_BANDS][MAX_GAIN_WIN]
|
||||
uint8_t alevCode[3][8][7]; // [MAX_GAIN_BANDS][MAX_GAIN_WIN][MAX_GAIN_ADJUST]
|
||||
uint8_t alocCode[3][8][7]; // [MAX_GAIN_BANDS][MAX_GAIN_WIN][MAX_GAIN_ADJUST]
|
||||
} GainControlInfo_t;
|
||||
|
||||
typedef struct _ICSInfo_t {
|
||||
uint8_t icsResBit;
|
||||
uint8_t winSequence;
|
||||
uint8_t winShape;
|
||||
uint8_t maxSFB;
|
||||
uint8_t sfGroup;
|
||||
uint8_t predictorDataPresent;
|
||||
uint8_t predictorReset;
|
||||
uint8_t predictorResetGroupNum;
|
||||
uint8_t predictionUsed[41]; // [MAX_PRED_SFB]
|
||||
uint8_t numWinGroup;
|
||||
uint8_t winGroupLen[8]; // [MAX_WIN_GROUPS]
|
||||
} ICSInfo_t;
|
||||
|
||||
typedef struct _ADTSHeader_t {
|
||||
/* fixed */
|
||||
uint8_t id; /* MPEG bit - should be 1 */
|
||||
uint8_t layer; /* MPEG layer - should be 0 */
|
||||
uint8_t protectBit; /* 0 = CRC word follows, 1 = no CRC word */
|
||||
uint8_t profile; /* 0 = main, 1 = LC, 2 = SSR, 3 = reserved */
|
||||
uint8_t sampRateIdx; /* sample rate index range = [0, 11] */
|
||||
uint8_t privateBit; /* ignore */
|
||||
uint8_t channelConfig; /* 0 = implicit, >0 = use default table */
|
||||
uint8_t origCopy; /* 0 = copy, 1 = original */
|
||||
uint8_t home; /* ignore */
|
||||
/* variable */
|
||||
uint8_t copyBit; /* 1 bit of the 72-bit copyright ID (transmitted as 1 bit per frame) */
|
||||
uint8_t copyStart; /* 1 = this bit starts the 72-bit ID, 0 = it does not */
|
||||
int frameLength; /* length of frame */
|
||||
int bufferFull; /* number of 32-bit words left in enc buffer, 0x7FF = VBR */
|
||||
uint8_t numRawDataBlocks; /* number of raw data blocks in frame */
|
||||
/* CRC */
|
||||
int crcCheckWord; /* 16-bit CRC check word (present if protectBit == 0) */
|
||||
} ADTSHeader_t;
|
||||
|
||||
typedef struct _ADIFHeader_t {
|
||||
uint8_t copyBit; /* 0 = no copyright ID, 1 = 72-bit copyright ID follows immediately */
|
||||
uint8_t origCopy; /* 0 = copy, 1 = original */
|
||||
uint8_t home; /* ignore */
|
||||
uint8_t bsType; /* bitstream type: 0 = CBR, 1 = VBR */
|
||||
int bitRate; /* bitRate: CBR = bits/sec, VBR = peak bits/frame, 0 = unknown */
|
||||
uint8_t numPCE; /* number of program config elements (max = 16) */
|
||||
int bufferFull; /* bits left in bit reservoir */
|
||||
uint8_t copyID[9]; /* [ADIF_COPYID_SIZE] optional 72-bit copyright ID */
|
||||
} ADIFHeader_t;
|
||||
|
||||
/* sizeof(ProgConfigElement_t) = 82 bytes (if KEEP_PCE_COMMENTS not defined) */
|
||||
typedef struct _ProgConfigElement_t {
|
||||
uint8_t elemInstTag; /* element instance tag */
|
||||
uint8_t profile; /* 0 = main, 1 = LC, 2 = SSR, 3 = reserved */
|
||||
uint8_t sampRateIdx; /* sample rate index range = [0, 11] */
|
||||
uint8_t numFCE; /* number of front channel elements (max = 15) */
|
||||
uint8_t numSCE; /* number of side channel elements (max = 15) */
|
||||
uint8_t numBCE; /* number of back channel elements (max = 15) */
|
||||
uint8_t numLCE; /* number of LFE channel elements (max = 3) */
|
||||
uint8_t numADE; /* number of associated data elements (max = 7) */
|
||||
uint8_t numCCE; /* number of valid channel coupling elements (max = 15) */
|
||||
uint8_t monoMixdown; /* mono mixdown: bit 4 = present flag, bits 3-0 = element number */
|
||||
uint8_t stereoMixdown; /* stereo mixdown: bit 4 = present flag, bits 3-0 = element number */
|
||||
uint8_t matrixMixdown; /* bit 4 = present flag, bit 3 = unused,bits 2-1 = index, bit 0 = pseudo-surround enable */
|
||||
uint8_t fce[15]; /* [MAX_NUM_FCE] front element channel pair: bit 4 = SCE/CPE flag, bits 3-0 = inst tag */
|
||||
uint8_t sce[15]; /* [MAX_NUM_SCE] side element channel pair: bit 4 = SCE/CPE flag, bits 3-0 = inst tag */
|
||||
uint8_t bce[15]; /* [MAX_NUM_BCE] back element channel pair: bit 4 = SCE/CPE flag, bits 3-0 = inst tag */
|
||||
uint8_t lce[3]; /* [MAX_NUM_LCE] instance tag for LFE elements */
|
||||
uint8_t ade[7]; /* [MAX_NUM_ADE] instance tag for ADE elements */
|
||||
uint8_t cce[15]; /* [MAX_NUM_BCE] channel coupling elements: bit 4 = switching flag, bits 3-0 = inst tag */
|
||||
} ProgConfigElement_t;
|
||||
|
||||
typedef struct _SBRHeader {
|
||||
int count;
|
||||
|
||||
uint8_t ampRes;
|
||||
uint8_t startFreq;
|
||||
uint8_t stopFreq;
|
||||
uint8_t crossOverBand;
|
||||
uint8_t resBitsHdr;
|
||||
uint8_t hdrExtra1;
|
||||
uint8_t hdrExtra2;
|
||||
|
||||
uint8_t freqScale;
|
||||
uint8_t alterScale;
|
||||
uint8_t noiseBands;
|
||||
|
||||
uint8_t limiterBands;
|
||||
uint8_t limiterGains;
|
||||
uint8_t interpFreq;
|
||||
uint8_t smoothMode;
|
||||
} SBRHeader;
|
||||
|
||||
/* need one SBRGrid per channel, updated every frame */
|
||||
typedef struct _SBRGrid {
|
||||
uint8_t frameClass;
|
||||
uint8_t ampResFrame;
|
||||
uint8_t pointer;
|
||||
|
||||
uint8_t numEnv; /* L_E */
|
||||
uint8_t envTimeBorder[5 + 1]; // [MAX_NUM_ENV+1] /* t_E */
|
||||
uint8_t freqRes[5]; // [MAX_NUM_ENV]/* r */
|
||||
uint8_t numNoiseFloors; /* L_Q */
|
||||
uint8_t noiseTimeBorder[2 + 1]; // [MAX_NUM_NOISE_FLOORS+1] /* t_Q */
|
||||
|
||||
uint8_t numEnvPrev;
|
||||
uint8_t numNoiseFloorsPrev;
|
||||
uint8_t freqResPrev;
|
||||
} SBRGrid;
|
||||
|
||||
/* need one SBRFreq per element (SCE/CPE/LFE), updated only on header reset */
|
||||
typedef struct _SBRFreq {
|
||||
int kStart; /* k_x */
|
||||
int nMaster;
|
||||
int nHigh;
|
||||
int nLow;
|
||||
int nLimiter; /* N_l */
|
||||
int numQMFBands; /* M */
|
||||
int numNoiseFloorBands; /* Nq */
|
||||
int kStartPrev;
|
||||
int numQMFBandsPrev;
|
||||
uint8_t freqMaster[48 + 1]; // [MAX_QMF_BANDS + 1] /* not necessary to save this after derived tables are generated */
|
||||
uint8_t freqHigh[48 + 1]; // [MAX_QMF_BANDS + 1]
|
||||
uint8_t freqLow[48 / 2 + 1]; // [MAX_QMF_BANDS / 2 + 1] /* nLow = nHigh - (nHigh >> 1) */
|
||||
uint8_t freqNoise[5 + 1]; // [MAX_NUM_NOISE_FLOOR_BANDS+1]
|
||||
uint8_t freqLimiter[48 / 2 + 5];// [MAX_QMF_BANDS / 2 + MAX_NUM_PATCHES] /* max (intermediate) size = nLow + numPatches - 1 */
|
||||
|
||||
uint8_t numPatches;
|
||||
uint8_t patchNumSubbands[5 + 1]; // [MAX_NUM_PATCHES + 1]
|
||||
uint8_t patchStartSubband[5 + 1]; // [MAX_NUM_PATCHES + 1]
|
||||
} SBRFreq;
|
||||
|
||||
typedef struct _SBRChan {
|
||||
int reset;
|
||||
uint8_t deltaFlagEnv[5]; // [MAX_NUM_ENV]
|
||||
uint8_t deltaFlagNoise[2]; // [MAX_NUM_NOISE_FLOORS]
|
||||
int8_t envDataQuant[5][48]; // [MAX_NUM_ENV][MAX_QMF_BANDS] /* range = [0, 127] */
|
||||
int8_t noiseDataQuant[2][5]; // [MAX_NUM_NOISE_FLOORS][MAX_NUM_NOISE_FLOOR_BANDS]
|
||||
|
||||
uint8_t invfMode[2][5]; // [2][MAX_NUM_NOISE_FLOOR_BANDS] /* invfMode[0/1][band] = prev/curr */
|
||||
int chirpFact[5]; // [MAX_NUM_NOISE_FLOOR_BANDS] /* bwArray */
|
||||
uint8_t addHarmonicFlag[2]; /* addHarmonicFlag[0/1] = prev/curr */
|
||||
uint8_t addHarmonic[2][64]; /* addHarmonic[0/1][band] = prev/curr */
|
||||
|
||||
int gbMask[2]; /* gbMask[0/1] = XBuf[0-31]/XBuf[32-39] */
|
||||
int8_t laPrev;
|
||||
|
||||
int noiseTabIndex;
|
||||
int sinIndex;
|
||||
int gainNoiseIndex;
|
||||
int gTemp[5][48]; // [MAX_NUM_SMOOTH_COEFS][MAX_QMF_BANDS]
|
||||
int qTemp[5][48]; // [MAX_NUM_SMOOTH_COEFS][MAX_QMF_BANDS]
|
||||
|
||||
} SBRChan;
|
||||
|
||||
|
||||
/* state info struct for baseline (MPEG-4 LC) decoding */
|
||||
typedef struct _PSInfoBase_t {
|
||||
int dataCount;
|
||||
uint8_t dataBuf[510]; // [DATA_BUF_SIZE]
|
||||
int fillCount;
|
||||
uint8_t fillBuf[269]; //[FILL_BUF_SIZE]
|
||||
/* state information which is the same throughout whole frame */
|
||||
int nChans;
|
||||
int useImpChanMap;
|
||||
int sampRateIdx;
|
||||
/* state information which can be overwritten by subsequent elements within frame */
|
||||
ICSInfo_t icsInfo[2]; // [MAX_NCHANS_ELEM]
|
||||
int commonWin;
|
||||
short scaleFactors[2][15*8]; // [MAX_NCHANS_ELEM][MAX_SF_BANDS]
|
||||
uint8_t sfbCodeBook[2][15*8]; // [MAX_NCHANS_ELEM][MAX_SF_BANDS]
|
||||
int msMaskPresent;
|
||||
uint8_t msMaskBits[(15 * 8 + 7) >> 3]; // [MAX_MS_MASK_BYTES]
|
||||
int pnsUsed[2]; // [MAX_NCHANS_ELEM]
|
||||
int pnsLastVal;
|
||||
int intensityUsed[2]; // [MAX_NCHANS_ELEM]
|
||||
// PulseInfo_t pulseInfo[2]; // [MAX_NCHANS_ELEM]
|
||||
TNSInfo_t tnsInfo[2]; // [MAX_NCHANS_ELEM]
|
||||
int tnsLPCBuf[20]; // [MAX_TNS_ORDER]
|
||||
int tnsWorkBuf[20]; //[MAX_TNS_ORDER]
|
||||
GainControlInfo_t gainControlInfo[2]; // [MAX_NCHANS_ELEM]
|
||||
int gbCurrent[2]; // [MAX_NCHANS_ELEM]
|
||||
int coef[2][1024]; // [MAX_NCHANS_ELEM][AAC_MAX_NSAMPS]
|
||||
#ifdef AAC_ENABLE_SBR
|
||||
int sbrWorkBuf[2][1024]; // [MAX_NCHANS_ELEM][AAC_MAX_NSAMPS];
|
||||
#endif
|
||||
/* state information which must be saved for each element and used in next frame */
|
||||
int overlap[2][1024]; // [AAC_MAX_NCHANS][AAC_MAX_NSAMPS]
|
||||
int prevWinShape[2]; // [AAC_MAX_NCHANS]
|
||||
} PSInfoBase_t;
|
||||
|
||||
typedef struct _PSInfoSBR {
|
||||
/* save for entire file */
|
||||
int frameCount;
|
||||
int sampRateIdx;
|
||||
|
||||
/* state info that must be saved for each channel */
|
||||
SBRHeader sbrHdr[2];
|
||||
SBRGrid sbrGrid[2];
|
||||
SBRFreq sbrFreq[2];
|
||||
SBRChan sbrChan[2];
|
||||
|
||||
/* temp variables, no need to save between blocks */
|
||||
uint8_t dataExtra;
|
||||
uint8_t resBitsData;
|
||||
uint8_t extendedDataPresent;
|
||||
int extendedDataSize;
|
||||
|
||||
int8_t envDataDequantScale[2][5]; // [MAX_NCHANS_ELEM][MAX_NUM_ENV
|
||||
int envDataDequant[2][5][48]; // [MAX_NCHANS_ELEM][MAX_NUM_ENV][MAX_QMF_BANDS
|
||||
int noiseDataDequant[2][2][5]; // [MAX_NCHANS_ELEM][MAX_NUM_NOISE_FLOORS][MAX_NUM_NOISE_FLOOR_BANDS]
|
||||
|
||||
int eCurr[48]; // [MAX_QMF_BANDS]
|
||||
uint8_t eCurrExp[48]; // [MAX_QMF_BANDS]
|
||||
uint8_t eCurrExpMax;
|
||||
int8_t la;
|
||||
|
||||
int crcCheckWord;
|
||||
int couplingFlag;
|
||||
int envBand;
|
||||
int eOMGainMax;
|
||||
int gainMax;
|
||||
int gainMaxFBits;
|
||||
int noiseFloorBand;
|
||||
int qp1Inv;
|
||||
int qqp1Inv;
|
||||
int sMapped;
|
||||
int sBand;
|
||||
int highBand;
|
||||
|
||||
int sumEOrigMapped;
|
||||
int sumECurrGLim;
|
||||
int sumSM;
|
||||
int sumQM;
|
||||
int gLimBoost[48];
|
||||
int qmLimBoost[48];
|
||||
int smBoost[48];
|
||||
|
||||
int smBuf[48];
|
||||
int qmLimBuf[48];
|
||||
int gLimBuf[48];
|
||||
int gLimFbits[48];
|
||||
|
||||
int gFiltLast[48];
|
||||
int qFiltLast[48];
|
||||
|
||||
/* large buffers */
|
||||
int delayIdxQMFA[2]; // [AAC_MAX_NCHANS]
|
||||
int delayQMFA[2][10 * 32]; // [AAC_MAX_NCHANS][DELAY_SAMPS_QMFA]
|
||||
int delayIdxQMFS[2]; // [AAC_MAX_NCHANS]
|
||||
int delayQMFS[2][10 * 128]; // [AAC_MAX_NCHANS][DELAY_SAMPS_QMFS]
|
||||
int XBufDelay[2][8][64][2]; // [AAC_MAX_NCHANS][HF_GEN][64][2]
|
||||
int XBuf[32+8][64][2];
|
||||
} PSInfoSBR_t;
|
||||
|
||||
bool AACDecoder_AllocateBuffers(void);
|
||||
int AACFlushCodec();
|
||||
void AACDecoder_FreeBuffers(void);
|
||||
bool AACDecoder_IsInit(void);
|
||||
int AACFindSyncWord(uint8_t *buf, int nBytes);
|
||||
int AACSetRawBlockParams(int copyLast, int nChans, int sampRateCore, int profile);
|
||||
int AACDecode(uint8_t *inbuf, int *bytesLeft, short *outbuf);
|
||||
int AACGetSampRate();
|
||||
int AACGetChannels();
|
||||
int AACGetID(); // 0-MPEG4, 1-MPEG2
|
||||
uint8_t AACGetProfile(); // 0-Main, 1-LC, 2-SSR, 3-reserved
|
||||
uint8_t AACGetFormat(); // 0-unknown 1-ADTS 2-ADIF, 3-RAW
|
||||
int AACGetBitsPerSample();
|
||||
int AACGetBitrate();
|
||||
int AACGetOutputSamps();
|
||||
int AACGetBitrate();
|
||||
void DecodeLPCCoefs(int order, int res, int8_t *filtCoef, int *a, int *b);
|
||||
int FilterRegion(int size, int dir, int order, int *audioCoef, int *a, int *hist);
|
||||
int TNSFilter(int ch);
|
||||
int DecodeSingleChannelElement();
|
||||
int DecodeChannelPairElement();
|
||||
int DecodeLFEChannelElement();
|
||||
int DecodeDataStreamElement();
|
||||
int DecodeProgramConfigElement(uint8_t idx);
|
||||
int DecodeFillElement();
|
||||
int DecodeNextElement(uint8_t **buf, int *bitOffset, int *bitsAvail);
|
||||
void PreMultiply(int tabidx, int *zbuf1);
|
||||
void PostMultiply(int tabidx, int *fft1);
|
||||
void PreMultiplyRescale(int tabidx, int *zbuf1, int es);
|
||||
void PostMultiplyRescale(int tabidx, int *fft1, int es);
|
||||
void DCT4(int tabidx, int *coef, int gb);
|
||||
void BitReverse(int *inout, int tabidx);
|
||||
void R4FirstPass(int *x, int bg);
|
||||
void R8FirstPass(int *x, int bg);
|
||||
void R4Core(int *x, int bg, int gp, int *wtab);
|
||||
void R4FFT(int tabidx, int *x);
|
||||
void UnpackZeros(int nVals, int *coef);
|
||||
void UnpackQuads(int cb, int nVals, int *coef);
|
||||
void UnpackPairsNoEsc(int cb, int nVals, int *coef);
|
||||
void UnpackPairsEsc(int cb, int nVals, int *coef);
|
||||
void DecodeSpectrumLong(int ch);
|
||||
void DecodeSpectrumShort(int ch);
|
||||
void DecWindowOverlap(int *buf0, int *over0, short *pcm0, int nChans, int winTypeCurr, int winTypePrev);
|
||||
void DecWindowOverlapLongStart(int *buf0, int *over0, short *pcm0, int nChans, int winTypeCurr, int winTypePrev);
|
||||
void DecWindowOverlapLongStop(int *buf0, int *over0, short *pcm0, int nChans, int winTypeCurr, int winTypePrev);
|
||||
void DecWindowOverlapShort(int *buf0, int *over0, short *pcm0, int nChans, int winTypeCurr, int winTypePrev);
|
||||
int IMDCT(int ch, int chOut, short *outbuf);
|
||||
void DecodeICSInfo(ICSInfo_t *icsInfo, int sampRateIdx);
|
||||
void DecodeSectionData(int winSequence, int numWinGrp, int maxSFB, uint8_t *sfbCodeBook);
|
||||
int DecodeOneScaleFactor();
|
||||
void DecodeScaleFactors(int numWinGrp, int maxSFB, int globalGain, uint8_t *sfbCodeBook, short *scaleFactors);
|
||||
void DecodePulseInfo(uint8_t ch);
|
||||
void DecodeTNSInfo(int winSequence, TNSInfo_t *ti, int8_t *tnsCoef);
|
||||
void DecodeGainControlInfo(int winSequence, GainControlInfo_t *gi);
|
||||
void DecodeICS(int ch);
|
||||
int DecodeNoiselessData(uint8_t **buf, int *bitOffset, int *bitsAvail, int ch);
|
||||
int DecodeHuffmanScalar(const signed short *huffTab, const HuffInfo_t *huffTabInfo, uint32_t bitBuf, int32_t *val);
|
||||
int UnpackADTSHeader(uint8_t **buf, int *bitOffset, int *bitsAvail);
|
||||
int GetADTSChannelMapping(uint8_t *buf, int bitOffset, int bitsAvail);
|
||||
int GetNumChannelsADIF(int nPCE);
|
||||
int GetSampleRateIdxADIF(int nPCE);
|
||||
int UnpackADIFHeader(uint8_t **buf, int *bitOffset, int *bitsAvail);
|
||||
int SetRawBlockParams(int copyLast, int nChans, int sampRate, int profile);
|
||||
int PrepareRawBlock();
|
||||
int DequantBlock(int *inbuf, int nSamps, int scale);
|
||||
int AACDequantize(int ch);
|
||||
int DeinterleaveShortBlocks(int ch);
|
||||
uint32_t Get32BitVal(uint32_t *last);
|
||||
int InvRootR(int r);
|
||||
int ScaleNoiseVector(int *coef, int nVals, int sf);
|
||||
void GenerateNoiseVector(int *coef, int *last, int nVals);
|
||||
void CopyNoiseVector(int *coefL, int *coefR, int nVals);
|
||||
int PNS(int ch);
|
||||
int GetSampRateIdx(int sampRate);
|
||||
void StereoProcessGroup(int *coefL, int *coefR, const uint16_t *sfbTab, int msMaskPres, uint8_t *msMaskPtr,
|
||||
int msMaskOffset, int maxSFB, uint8_t *cbRight, short *sfRight, int *gbCurrent);
|
||||
int StereoProcess();
|
||||
int RatioPowInv(int a, int b, int c);
|
||||
int SqrtFix(int q, int fBitsIn, int *fBitsOut);
|
||||
int InvRNormalized(int r);
|
||||
void BitReverse32(int *inout);
|
||||
void R8FirstPass32(int *r0);
|
||||
void R4Core32(int *r0);
|
||||
void FFT32C(int *x);
|
||||
void CVKernel1(int *XBuf, int *accBuf);
|
||||
void CVKernel2(int *XBuf, int *accBuf);
|
||||
void SetBitstreamPointer(int nBytes, uint8_t *buf);
|
||||
inline void RefillBitstreamCache();
|
||||
uint32_t GetBits(int nBits);
|
||||
uint32_t GetBitsNoAdvance(int nBits);
|
||||
void AdvanceBitstream(int nBits);
|
||||
int CalcBitsUsed(uint8_t *startBuf, int startOffset);
|
||||
void ByteAlignBitstream();
|
||||
// SBR
|
||||
void InitSBRState();
|
||||
int DecodeSBRBitstream(int chBase);
|
||||
int DecodeSBRData(int chBase, short *outbuf);
|
||||
int FlushCodecSBR();
|
||||
void BubbleSort(uint8_t *v, int nItems);
|
||||
uint8_t VMin(uint8_t *v, int nItems);
|
||||
uint8_t VMax(uint8_t *v, int nItems);
|
||||
int CalcFreqMasterScaleZero(uint8_t *freqMaster, int alterScale, int k0, int k2);
|
||||
int CalcFreqMaster(uint8_t *freqMaster, int freqScale, int alterScale, int k0, int k2);
|
||||
int CalcFreqHigh(uint8_t *freqHigh, uint8_t *freqMaster, int nMaster, int crossOverBand);
|
||||
int CalcFreqLow(uint8_t *freqLow, uint8_t *freqHigh, int nHigh);
|
||||
int CalcFreqNoise(uint8_t *freqNoise, uint8_t *freqLow, int nLow, int kStart, int k2, int noiseBands);
|
||||
int BuildPatches(uint8_t *patchNumSubbands, uint8_t *patchStartSubband, uint8_t *freqMaster, int nMaster, int k0,
|
||||
int kStart, int numQMFBands, int sampRateIdx);
|
||||
int FindFreq(uint8_t *freq, int nFreq, uint8_t val);
|
||||
void RemoveFreq(uint8_t *freq, int nFreq, int removeIdx);
|
||||
int CalcFreqLimiter(uint8_t *freqLimiter, uint8_t *patchNumSubbands, uint8_t *freqLow, int nLow, int kStart,
|
||||
int limiterBands, int numPatches);
|
||||
int CalcFreqTables(SBRHeader *sbrHdr, SBRFreq *sbrFreq, int sampRateIdx);
|
||||
void EstimateEnvelope(SBRHeader *sbrHdr, SBRGrid *sbrGrid, SBRFreq *sbrFreq, int env);
|
||||
int GetSMapped(SBRGrid *sbrGrid, SBRFreq *sbrFreq, SBRChan *sbrChan, int env, int band, int la);
|
||||
void CalcMaxGain(SBRHeader *sbrHdr, SBRGrid *sbrGrid, SBRFreq *sbrFreq, int ch, int env, int lim, int fbitsDQ);
|
||||
void CalcNoiseDivFactors(int q, int *qp1Inv, int *qqp1Inv);
|
||||
void CalcComponentGains(SBRGrid *sbrGrid, SBRFreq *sbrFreq, SBRChan *sbrChan, int ch, int env, int lim, int fbitsDQ);
|
||||
void ApplyBoost(SBRFreq *sbrFreq, int lim, int fbitsDQ);
|
||||
void CalcGain(SBRHeader *sbrHdr, SBRGrid *sbrGrid, SBRFreq *sbrFreq, SBRChan *sbrChan, int ch, int env);
|
||||
void MapHF(SBRHeader *sbrHdr, SBRGrid *sbrGrid, SBRFreq *sbrFreq, SBRChan *sbrChan, int env, int hfReset);
|
||||
void AdjustHighFreq(SBRHeader *sbrHdr, SBRGrid *sbrGrid, SBRFreq *sbrFreq, SBRChan *sbrChan, int ch);
|
||||
int CalcCovariance1(int *XBuf, int *p01reN, int *p01imN, int *p12reN, int *p12imN, int *p11reN, int *p22reN);
|
||||
int CalcCovariance2(int *XBuf, int *p02reN, int *p02imN);
|
||||
void CalcLPCoefs(int *XBuf, int *a0re, int *a0im, int *a1re, int *a1im, int gb);
|
||||
void GenerateHighFreq(SBRGrid *sbrGrid, SBRFreq *sbrFreq, SBRChan *sbrChan, int ch);
|
||||
int DecodeHuffmanScalar(const signed int *huffTab, const HuffInfo_t *huffTabInfo, uint32_t bitBuf, signed int *val);
|
||||
int DecodeOneSymbol(int huffTabIndex);
|
||||
int DequantizeEnvelope(int nBands, int ampRes, int8_t *envQuant, int *envDequant);
|
||||
void DequantizeNoise(int nBands, int8_t *noiseQuant, int *noiseDequant);
|
||||
void DecodeSBREnvelope(SBRGrid *sbrGrid, SBRFreq *sbrFreq, SBRChan *sbrChan, int ch);
|
||||
void DecodeSBRNoise(SBRGrid *sbrGrid, SBRFreq *sbrFreq, SBRChan *sbrChan, int ch);
|
||||
void UncoupleSBREnvelope(SBRGrid *sbrGrid, SBRFreq *sbrFreq, SBRChan *sbrChanR);
|
||||
void UncoupleSBRNoise(SBRGrid *sbrGrid, SBRFreq *sbrFreq, SBRChan *sbrChanR);
|
||||
void DecWindowOverlapNoClip(int *buf0, int *over0, int *out0, int winTypeCurr, int winTypePrev);
|
||||
void DecWindowOverlapLongStartNoClip(int *buf0, int *over0, int *out0, int winTypeCurr, int winTypePrev);
|
||||
void DecWindowOverlapLongStopNoClip(int *buf0, int *over0, int *out0, int winTypeCurr, int winTypePrev);
|
||||
void DecWindowOverlapShortNoClip(int *buf0, int *over0, int *out0, int winTypeCurr, int winTypePrev);
|
||||
void PreMultiply64(int *zbuf1);
|
||||
void PostMultiply64(int *fft1, int nSampsOut);
|
||||
void QMFAnalysisConv(int *cTab, int *delay, int dIdx, int *uBuf);
|
||||
int QMFAnalysis(int *inbuf, int *delay, int *XBuf, int fBitsIn, int *delayIdx, int qmfaBands);
|
||||
void QMFSynthesisConv(int *cPtr, int *delay, int dIdx, short *outbuf, int nChans);
|
||||
void QMFSynthesis(int *inbuf, int *delay, int *delayIdx, int qmfsBands, short *outbuf, int nChans);
|
||||
int UnpackSBRHeader(SBRHeader *sbrHdr);
|
||||
void UnpackSBRGrid(SBRHeader *sbrHdr, SBRGrid *sbrGrid);
|
||||
void UnpackDeltaTimeFreq(int numEnv, uint8_t *deltaFlagEnv, int numNoiseFloors, uint8_t *deltaFlagNoise);
|
||||
void UnpackInverseFilterMode(int numNoiseFloorBands, uint8_t *mode);
|
||||
void UnpackSinusoids(int nHigh, int addHarmonicFlag, uint8_t *addHarmonic);
|
||||
void CopyCouplingGrid(SBRGrid *sbrGridLeft, SBRGrid *sbrGridRight);
|
||||
void CopyCouplingInverseFilterMode(int numNoiseFloorBands, uint8_t *modeLeft, uint8_t *modeRight);
|
||||
void UnpackSBRSingleChannel(int chBase);
|
||||
void UnpackSBRChannelPair(int chBase);
|
613
lib/ESP32-audioI2S-3.0.0/src/flac_decoder/flac_decoder.cpp
Normal file
@ -0,0 +1,613 @@
|
||||
/*
|
||||
* flac_decoder.cpp
|
||||
* Java source code from https://www.nayuki.io/page/simple-flac-implementation
|
||||
* adapted to ESP32
|
||||
*
|
||||
* Created on: Jul 03,2020
|
||||
* Updated on: Feb 10,2023
|
||||
*
|
||||
* Author: Wolle
|
||||
*
|
||||
*
|
||||
*/
|
||||
#include "flac_decoder.h"
|
||||
#include "vector"
|
||||
using namespace std;
|
||||
|
||||
|
||||
FLACFrameHeader_t *FLACFrameHeader;
|
||||
FLACMetadataBlock_t *FLACMetadataBlock;
|
||||
FLACsubFramesBuff_t *FLACsubFramesBuff;
|
||||
|
||||
vector<int32_t> coefs;
|
||||
const uint16_t outBuffSize = 2048;
|
||||
uint16_t m_blockSize = 0;
|
||||
uint16_t m_blockSizeLeft = 0;
|
||||
uint16_t m_validSamples = 0;
|
||||
uint8_t m_status = 0;
|
||||
uint8_t *m_inptr;
|
||||
uint16_t *s_flacSegmentTable = NULL;
|
||||
float m_compressionRatio = 0;
|
||||
uint16_t m_rIndex = 0;
|
||||
uint64_t m_bitBuffer = 0;
|
||||
uint8_t m_bitBufferLen = 0;
|
||||
bool s_f_flacParseOgg = false;
|
||||
uint8_t m_flacPageSegments = 0;
|
||||
uint8_t m_page0_len = 0;
|
||||
char *m_streamTitle = NULL;
|
||||
boolean s_f_newSt = false;
|
||||
|
||||
//----------------------------------------------------------------------------------------------------------------------
|
||||
// FLAC INI SECTION
|
||||
//----------------------------------------------------------------------------------------------------------------------
|
||||
bool FLACDecoder_AllocateBuffers(void){
|
||||
if(psramFound()) {
|
||||
// PSRAM found, Buffer will be allocated in PSRAM
|
||||
if(!FLACFrameHeader) {FLACFrameHeader = (FLACFrameHeader_t*) ps_malloc(sizeof(FLACFrameHeader_t));}
|
||||
if(!FLACMetadataBlock) {FLACMetadataBlock = (FLACMetadataBlock_t*) ps_malloc(sizeof(FLACMetadataBlock_t));}
|
||||
if(!FLACsubFramesBuff) {FLACsubFramesBuff = (FLACsubFramesBuff_t*) ps_malloc(sizeof(FLACsubFramesBuff_t));}
|
||||
if(!m_streamTitle) {m_streamTitle = (char*) ps_malloc(256);}
|
||||
if(!s_flacSegmentTable) {s_flacSegmentTable = (uint16_t*) ps_malloc(256 * sizeof(uint16_t));}
|
||||
}
|
||||
else {
|
||||
if(!FLACFrameHeader) {FLACFrameHeader = (FLACFrameHeader_t*) malloc(sizeof(FLACFrameHeader_t));}
|
||||
if(!FLACMetadataBlock) {FLACMetadataBlock = (FLACMetadataBlock_t*) malloc(sizeof(FLACMetadataBlock_t));}
|
||||
if(!FLACsubFramesBuff) {FLACsubFramesBuff = (FLACsubFramesBuff_t*) malloc(sizeof(FLACsubFramesBuff_t));}
|
||||
if(!m_streamTitle) {m_streamTitle = (char*) malloc(256);}
|
||||
if(!s_flacSegmentTable) {s_flacSegmentTable = (uint16_t*) malloc(256 * sizeof(uint16_t));}
|
||||
|
||||
}
|
||||
if(!FLACFrameHeader || !FLACMetadataBlock || !FLACsubFramesBuff || !m_streamTitle || !s_flacSegmentTable){
|
||||
log_e("not enough memory to allocate flacdecoder buffers");
|
||||
return false;
|
||||
}
|
||||
FLACDecoder_ClearBuffer();
|
||||
return true;
|
||||
}
|
||||
//----------------------------------------------------------------------------------------------------------------------
|
||||
void FLACDecoder_ClearBuffer(){
|
||||
memset(FLACFrameHeader, 0, sizeof(FLACFrameHeader_t));
|
||||
memset(FLACMetadataBlock, 0, sizeof(FLACMetadataBlock_t));
|
||||
memset(FLACsubFramesBuff, 0, sizeof(FLACsubFramesBuff_t));
|
||||
m_status = DECODE_FRAME;
|
||||
return;
|
||||
}
|
||||
//----------------------------------------------------------------------------------------------------------------------
|
||||
void FLACDecoder_FreeBuffers(){
|
||||
if(FLACFrameHeader) {free(FLACFrameHeader); FLACFrameHeader = NULL;}
|
||||
if(FLACMetadataBlock) {free(FLACMetadataBlock); FLACMetadataBlock = NULL;}
|
||||
if(FLACsubFramesBuff) {free(FLACsubFramesBuff); FLACsubFramesBuff = NULL;}
|
||||
if(m_streamTitle) {free(m_streamTitle); m_streamTitle = NULL;}
|
||||
if(s_flacSegmentTable) {free(s_flacSegmentTable); s_flacSegmentTable = NULL;}
|
||||
}
|
||||
//----------------------------------------------------------------------------------------------------------------------
|
||||
// B I T R E A D E R
|
||||
//----------------------------------------------------------------------------------------------------------------------
|
||||
uint32_t readUint(uint8_t nBits, int *bytesLeft){
|
||||
while (m_bitBufferLen < nBits){
|
||||
uint8_t temp = *(m_inptr + m_rIndex);
|
||||
m_rIndex++;
|
||||
(*bytesLeft)--;
|
||||
if(*bytesLeft < 0) { log_i("error in bitreader"); }
|
||||
m_bitBuffer = (m_bitBuffer << 8) | temp;
|
||||
m_bitBufferLen += 8;
|
||||
}
|
||||
m_bitBufferLen -= nBits;
|
||||
uint32_t result = m_bitBuffer >> m_bitBufferLen;
|
||||
if (nBits < 32)
|
||||
result &= (1 << nBits) - 1;
|
||||
return result;
|
||||
}
|
||||
|
||||
int32_t readSignedInt(int nBits, int* bytesLeft){
|
||||
int32_t temp = readUint(nBits, bytesLeft) << (32 - nBits);
|
||||
temp = temp >> (32 - nBits); // The C++ compiler uses the sign bit to fill vacated bit positions
|
||||
return temp;
|
||||
}
|
||||
|
||||
int64_t readRiceSignedInt(uint8_t param, int* bytesLeft){
|
||||
long val = 0;
|
||||
while (readUint(1, bytesLeft) == 0)
|
||||
val++;
|
||||
val = (val << param) | readUint(param, bytesLeft);
|
||||
return (val >> 1) ^ -(val & 1);
|
||||
}
|
||||
|
||||
void alignToByte() {
|
||||
m_bitBufferLen -= m_bitBufferLen % 8;
|
||||
}
|
||||
//----------------------------------------------------------------------------------------------------------------------
|
||||
// F L A C - D E C O D E R
|
||||
//----------------------------------------------------------------------------------------------------------------------
|
||||
void FLACSetRawBlockParams(uint8_t Chans, uint32_t SampRate, uint8_t BPS, uint32_t tsis, uint32_t AuDaLength){
|
||||
FLACMetadataBlock->numChannels = Chans;
|
||||
FLACMetadataBlock->sampleRate = SampRate;
|
||||
FLACMetadataBlock->bitsPerSample = BPS;
|
||||
FLACMetadataBlock->totalSamples = tsis; // total samples in stream
|
||||
FLACMetadataBlock->audioDataLength = AuDaLength;
|
||||
}
|
||||
//----------------------------------------------------------------------------------------------------------------------
|
||||
void FLACDecoderReset(){ // set var to default
|
||||
m_status = DECODE_FRAME;
|
||||
m_bitBuffer = 0;
|
||||
m_bitBufferLen = 0;
|
||||
}
|
||||
//----------------------------------------------------------------------------------------------------------------------
|
||||
int FLACFindSyncWord(unsigned char *buf, int nBytes) {
|
||||
int i;
|
||||
i = FLAC_specialIndexOf(buf, "OggS", nBytes);
|
||||
if(i == 0){
|
||||
// flag has ogg wrapper
|
||||
return 0;
|
||||
}
|
||||
/* find byte-aligned sync code - need 14 matching bits */
|
||||
for (i = 0; i < nBytes - 1; i++) {
|
||||
if ((buf[i + 0] & 0xFF) == 0xFF && (buf[i + 1] & 0xFC) == 0xF8) { // <14> Sync code '11111111111110xx'
|
||||
FLACDecoderReset();
|
||||
return i;
|
||||
}
|
||||
}
|
||||
return -1;
|
||||
}
|
||||
//----------------------------------------------------------------------------------------------------------------------
|
||||
boolean FLACFindMagicWord(unsigned char* buf, int nBytes){
|
||||
int idx = FLAC_specialIndexOf(buf, "fLaC", nBytes);
|
||||
if(idx >0){ // Metadatablock follows
|
||||
idx += 4;
|
||||
boolean lmdbf = ((buf[idx + 1] & 0x80) == 0x80); // Last-metadata-block flag
|
||||
uint8_t bt = (buf[idx + 1] & 0x7F); // block type
|
||||
uint32_t lomd = (buf[idx + 2] << 16) + (buf[idx + 3] << 8) + buf[idx + 4]; // Length of metadata to follow
|
||||
|
||||
// TODO - parse metadata block data
|
||||
(void)lmdbf; (void)bt; (void)lomd;
|
||||
// log_i("Last-metadata-block flag: %d", lmdbf);
|
||||
// log_i("block type: %d", bt);
|
||||
// log_i("Length (in bytes) of metadata to follow: %d", lomd);
|
||||
return true;
|
||||
}
|
||||
return false;
|
||||
}
|
||||
//----------------------------------------------------------------------------------------------------------------------
|
||||
char* FLACgetStreamTitle(){
|
||||
if(s_f_newSt){
|
||||
s_f_newSt = false;
|
||||
return m_streamTitle;
|
||||
}
|
||||
return NULL;
|
||||
}
|
||||
//----------------------------------------------------------------------------------------------------------------------
|
||||
int FLACparseOGG(uint8_t *inbuf, int *bytesLeft){ // reference https://www.xiph.org/ogg/doc/rfc3533.txt
|
||||
|
||||
s_f_flacParseOgg = false;
|
||||
int idx = FLAC_specialIndexOf(inbuf, "OggS", 6);
|
||||
if(idx != 0) return ERR_FLAC_DECODER_ASYNC;
|
||||
|
||||
uint8_t version = *(inbuf + 4); (void) version;
|
||||
uint8_t headerType = *(inbuf + 5); (void) headerType;
|
||||
uint64_t granulePosition = (uint64_t)*(inbuf + 13) << 56; // granule_position: an 8 Byte field containing -
|
||||
granulePosition += (uint64_t)*(inbuf + 12) << 48; // position information. For an audio stream, it MAY
|
||||
granulePosition += (uint64_t)*(inbuf + 11) << 40; // contain the total number of PCM samples encoded
|
||||
granulePosition += (uint64_t)*(inbuf + 10) << 32; // after including all frames finished on this page.
|
||||
granulePosition += *(inbuf + 9) << 24; // This is a hint for the decoder and gives it some timing
|
||||
granulePosition += *(inbuf + 8) << 16; // and position information. A special value of -1 (in two's
|
||||
granulePosition += *(inbuf + 7) << 8; // complement) indicates that no packets finish on this page.
|
||||
granulePosition += *(inbuf + 6); (void) granulePosition;
|
||||
uint32_t bitstreamSerialNr = *(inbuf + 17) << 24; // bitstream_serial_number: a 4 Byte field containing the
|
||||
bitstreamSerialNr += *(inbuf + 16) << 16; // unique serial number by which the logical bitstream
|
||||
bitstreamSerialNr += *(inbuf + 15) << 8; // is identified.
|
||||
bitstreamSerialNr += *(inbuf + 14); (void) bitstreamSerialNr;
|
||||
uint32_t pageSequenceNr = *(inbuf + 21) << 24; // page_sequence_number: a 4 Byte field containing the sequence
|
||||
pageSequenceNr += *(inbuf + 20) << 16; // number of the page so the decoder can identify page loss
|
||||
pageSequenceNr += *(inbuf + 19) << 8; // This sequence number is increasing on each logical bitstream
|
||||
pageSequenceNr += *(inbuf + 18); (void) pageSequenceNr;
|
||||
uint32_t CRCchecksum = *(inbuf + 25) << 24;
|
||||
CRCchecksum += *(inbuf + 24) << 16;
|
||||
CRCchecksum += *(inbuf + 23) << 8;
|
||||
CRCchecksum += *(inbuf + 22); (void) CRCchecksum;
|
||||
uint8_t pageSegments = *(inbuf + 26); // giving the number of segment entries
|
||||
|
||||
// read the segment table (contains pageSegments bytes), 1...251: Length of the frame in bytes,
|
||||
// 255: A second byte is needed. The total length is first_byte + second byte
|
||||
|
||||
int16_t segmentTableWrPtr = 0;
|
||||
|
||||
for(int i = 0; i < pageSegments; i++){
|
||||
int n = *(inbuf + 27 + i);
|
||||
while(*(inbuf + 27 + i) == 255){
|
||||
i++;
|
||||
n+= *(inbuf + 27 + i);
|
||||
}
|
||||
s_flacSegmentTable[segmentTableWrPtr] = n;
|
||||
segmentTableWrPtr++;
|
||||
// s_flacSegmentLength += n;
|
||||
}
|
||||
m_page0_len = s_flacSegmentTable[0];
|
||||
|
||||
// for(int i = 0; i<pageSegments; i++){
|
||||
// log_i("%i %i", i, s_flacSegmentTable[i]);
|
||||
// }
|
||||
|
||||
|
||||
bool continuedPage = headerType & 0x01; // set: page contains data of a packet continued from the previous page
|
||||
bool firstPage = headerType & 0x02; // set: this is the first page of a logical bitstream (bos)
|
||||
bool lastPage = headerType & 0x04; // set: this is the last page of a logical bitstream (eos)
|
||||
static uint8_t secondPage = 0; (void)continuedPage; (void)lastPage;
|
||||
|
||||
if(firstPage) secondPage = 3;
|
||||
if(secondPage) secondPage--;
|
||||
|
||||
uint16_t headerSize = 0;
|
||||
uint8_t aLen = 0, tLen = 0;
|
||||
uint8_t *aPos = NULL, *tPos = NULL;
|
||||
if(firstPage || secondPage == 1){
|
||||
// log_i("s_flacSegmentTable[0] %i", s_flacSegmentTable[0]);
|
||||
headerSize = pageSegments + s_flacSegmentTable[0] +27;
|
||||
idx = FLAC_specialIndexOf(inbuf + 28, "ARTIST", s_flacSegmentTable[0]);
|
||||
if(idx > 0){
|
||||
aPos = inbuf + 28 + idx + 7;
|
||||
aLen = *(inbuf + 28 +idx -4) - 7;
|
||||
}
|
||||
idx = FLAC_specialIndexOf(inbuf + 28, "TITLE", s_flacSegmentTable[0]);
|
||||
if(idx > 0){
|
||||
tPos = inbuf + 28 + idx + 6;
|
||||
tLen = *(inbuf + 28 + idx -4) - 6;
|
||||
}
|
||||
int pos = 0;
|
||||
if(aLen) {memcpy(m_streamTitle, aPos, aLen); m_streamTitle[aLen] = '\0'; pos = aLen;}
|
||||
if(aLen && tLen) {strcat(m_streamTitle, " - "); pos += 3;}
|
||||
if(tLen) {memcpy(m_streamTitle + pos, tPos, tLen); m_streamTitle[pos + tLen] = '\0';}
|
||||
if(tLen || aLen) s_f_newSt = true;
|
||||
}
|
||||
else{
|
||||
headerSize = pageSegments + 27;
|
||||
}
|
||||
*bytesLeft -= headerSize;
|
||||
return ERR_FLAC_NONE; // no error
|
||||
}
|
||||
//----------------------------------------------------------------------------------------------------------------------
|
||||
int8_t FLACDecode(uint8_t *inbuf, int *bytesLeft, short *outbuf){
|
||||
|
||||
if(s_f_flacParseOgg == true){
|
||||
int ret = FLACparseOGG(inbuf, bytesLeft);
|
||||
if(ret == ERR_FLAC_NONE) return FLAC_PARSE_OGG_DONE; // ok
|
||||
else return ret; // error
|
||||
}
|
||||
|
||||
if(m_status != OUT_SAMPLES){
|
||||
m_rIndex = 0;
|
||||
m_inptr = inbuf;
|
||||
}
|
||||
|
||||
if(m_status == DECODE_FRAME){ // Read a ton of header fields, and ignore most of them
|
||||
|
||||
if ((inbuf[0] == 'O') && (inbuf[1] == 'g') && (inbuf[2] == 'g') && (inbuf[3] == 'S')){
|
||||
s_f_flacParseOgg = true;
|
||||
return FLAC_PARSE_OGG_DONE;
|
||||
}
|
||||
return flacDecodeFrame (inbuf, bytesLeft);
|
||||
}
|
||||
|
||||
if(m_status == DECODE_SUBFRAMES){
|
||||
|
||||
// Decode each channel's subframe, then skip footer
|
||||
int ret = decodeSubframes(bytesLeft);
|
||||
if(ret != 0) return ret;
|
||||
m_status = OUT_SAMPLES;
|
||||
}
|
||||
|
||||
if(m_status == OUT_SAMPLES){ // Write the decoded samples
|
||||
// blocksize can be much greater than outbuff, so we can't stuff all in once
|
||||
// therefore we need often more than one loop (split outputblock into pieces)
|
||||
uint16_t blockSize;
|
||||
static uint16_t offset = 0;
|
||||
if(m_blockSize < outBuffSize + offset) blockSize = m_blockSize - offset;
|
||||
else blockSize = outBuffSize;
|
||||
|
||||
|
||||
for (int i = 0; i < blockSize; i++) {
|
||||
for (int j = 0; j < FLACMetadataBlock->numChannels; j++) {
|
||||
int val = FLACsubFramesBuff->samplesBuffer[j][i + offset];
|
||||
if (FLACMetadataBlock->bitsPerSample == 8) val += 128;
|
||||
outbuf[2*i+j] = val;
|
||||
}
|
||||
}
|
||||
|
||||
m_validSamples = blockSize * FLACMetadataBlock->numChannels;
|
||||
offset += blockSize;
|
||||
|
||||
if(offset != m_blockSize) return GIVE_NEXT_LOOP;
|
||||
offset = 0;
|
||||
if(offset > m_blockSize) { log_e("offset has a wrong value"); }
|
||||
}
|
||||
|
||||
alignToByte();
|
||||
readUint(16, bytesLeft);
|
||||
// log_i("m_bytesDecoded %i", m_bytesDecoded);
|
||||
// m_compressionRatio = (float)m_bytesDecoded / (float)m_blockSize * FLACMetadataBlock->numChannels * (16/8);
|
||||
// log_i("m_compressionRatio % f", m_compressionRatio);
|
||||
m_status = DECODE_FRAME;
|
||||
return ERR_FLAC_NONE;
|
||||
}
|
||||
//----------------------------------------------------------------------------------------------------------------------
|
||||
int8_t flacDecodeFrame(uint8_t *inbuf, int *bytesLeft){
|
||||
readUint(14 + 1, bytesLeft); // synccode + reserved bit
|
||||
FLACFrameHeader->blockingStrategy = readUint(1, bytesLeft);
|
||||
FLACFrameHeader->blockSizeCode = readUint(4, bytesLeft);
|
||||
FLACFrameHeader->sampleRateCode = readUint(4, bytesLeft);
|
||||
FLACFrameHeader->chanAsgn = readUint(4, bytesLeft);
|
||||
FLACFrameHeader->sampleSizeCode = readUint(3, bytesLeft);
|
||||
if(!FLACMetadataBlock->numChannels){
|
||||
if(FLACFrameHeader->chanAsgn == 0) FLACMetadataBlock->numChannels = 1;
|
||||
if(FLACFrameHeader->chanAsgn == 1) FLACMetadataBlock->numChannels = 2;
|
||||
if(FLACFrameHeader->chanAsgn > 7) FLACMetadataBlock->numChannels = 2;
|
||||
}
|
||||
if(FLACMetadataBlock->numChannels < 1) return ERR_FLAC_UNKNOWN_CHANNEL_ASSIGNMENT;
|
||||
if(!FLACMetadataBlock->bitsPerSample){
|
||||
if(FLACFrameHeader->sampleSizeCode == 1) FLACMetadataBlock->bitsPerSample = 8;
|
||||
if(FLACFrameHeader->sampleSizeCode == 2) FLACMetadataBlock->bitsPerSample = 12;
|
||||
if(FLACFrameHeader->sampleSizeCode == 4) FLACMetadataBlock->bitsPerSample = 16;
|
||||
if(FLACFrameHeader->sampleSizeCode == 5) FLACMetadataBlock->bitsPerSample = 20;
|
||||
if(FLACFrameHeader->sampleSizeCode == 6) FLACMetadataBlock->bitsPerSample = 24;
|
||||
}
|
||||
if(FLACMetadataBlock->bitsPerSample > 16) return ERR_FLAC_BITS_PER_SAMPLE_TOO_BIG;
|
||||
if(FLACMetadataBlock->bitsPerSample < 8 ) return ERR_FLAG_BITS_PER_SAMPLE_UNKNOWN;
|
||||
if(!FLACMetadataBlock->sampleRate){
|
||||
if(FLACFrameHeader->sampleRateCode == 1) FLACMetadataBlock->sampleRate = 88200;
|
||||
if(FLACFrameHeader->sampleRateCode == 2) FLACMetadataBlock->sampleRate = 176400;
|
||||
if(FLACFrameHeader->sampleRateCode == 3) FLACMetadataBlock->sampleRate = 192000;
|
||||
if(FLACFrameHeader->sampleRateCode == 4) FLACMetadataBlock->sampleRate = 8000;
|
||||
if(FLACFrameHeader->sampleRateCode == 5) FLACMetadataBlock->sampleRate = 16000;
|
||||
if(FLACFrameHeader->sampleRateCode == 6) FLACMetadataBlock->sampleRate = 22050;
|
||||
if(FLACFrameHeader->sampleRateCode == 7) FLACMetadataBlock->sampleRate = 24000;
|
||||
if(FLACFrameHeader->sampleRateCode == 8) FLACMetadataBlock->sampleRate = 32000;
|
||||
if(FLACFrameHeader->sampleRateCode == 9) FLACMetadataBlock->sampleRate = 44100;
|
||||
if(FLACFrameHeader->sampleRateCode == 10) FLACMetadataBlock->sampleRate = 48000;
|
||||
if(FLACFrameHeader->sampleRateCode == 11) FLACMetadataBlock->sampleRate = 96000;
|
||||
}
|
||||
readUint(1, bytesLeft);
|
||||
uint32_t temp = (readUint(8, bytesLeft) << 24);
|
||||
temp = ~temp;
|
||||
uint32_t shift = 0x80000000; // Number of leading zeros
|
||||
int8_t count = 0;
|
||||
for(int i=0; i<32; i++){
|
||||
if((temp & shift) == 0) {count++; shift >>= 1;}
|
||||
else break;
|
||||
}
|
||||
count--;
|
||||
for (int i = 0; i < count; i++) readUint(8, bytesLeft);
|
||||
m_blockSize = 0;
|
||||
if (FLACFrameHeader->blockSizeCode == 1)
|
||||
m_blockSize = 192;
|
||||
else if (2 <= FLACFrameHeader->blockSizeCode && FLACFrameHeader->blockSizeCode <= 5)
|
||||
m_blockSize = 576 << (FLACFrameHeader->blockSizeCode - 2);
|
||||
else if (FLACFrameHeader->blockSizeCode == 6)
|
||||
m_blockSize = readUint(8, bytesLeft) + 1;
|
||||
else if (FLACFrameHeader->blockSizeCode == 7)
|
||||
m_blockSize = readUint(16, bytesLeft) + 1;
|
||||
else if (8 <= FLACFrameHeader->blockSizeCode && FLACFrameHeader->blockSizeCode <= 15)
|
||||
m_blockSize = 256 << (FLACFrameHeader->blockSizeCode - 8);
|
||||
else{
|
||||
return ERR_FLAC_RESERVED_BLOCKSIZE_UNSUPPORTED;
|
||||
}
|
||||
if(m_blockSize > 8192){
|
||||
log_e("Error: blockSize too big ,%i bytes", m_blockSize);
|
||||
return ERR_FLAC_BLOCKSIZE_TOO_BIG;
|
||||
}
|
||||
if(FLACFrameHeader->sampleRateCode == 12)
|
||||
readUint(8, bytesLeft);
|
||||
else if (FLACFrameHeader->sampleRateCode == 13 || FLACFrameHeader->sampleRateCode == 14){
|
||||
readUint(16, bytesLeft);
|
||||
}
|
||||
readUint(8, bytesLeft);
|
||||
m_status = DECODE_SUBFRAMES;
|
||||
m_blockSizeLeft = m_blockSize;
|
||||
return ERR_FLAC_NONE;
|
||||
}
|
||||
//----------------------------------------------------------------------------------------------------------------------
|
||||
uint16_t FLACGetOutputSamps(){
|
||||
int vs = m_validSamples;
|
||||
m_validSamples=0;
|
||||
return vs;
|
||||
}
|
||||
//----------------------------------------------------------------------------------------------------------------------
|
||||
uint64_t FLACGetTotoalSamplesInStream(){
|
||||
return FLACMetadataBlock->totalSamples;
|
||||
}
|
||||
//----------------------------------------------------------------------------------------------------------------------
|
||||
uint8_t FLACGetBitsPerSample(){
|
||||
return FLACMetadataBlock->bitsPerSample;
|
||||
}
|
||||
//----------------------------------------------------------------------------------------------------------------------
|
||||
uint8_t FLACGetChannels(){
|
||||
return FLACMetadataBlock->numChannels;
|
||||
}
|
||||
//----------------------------------------------------------------------------------------------------------------------
|
||||
uint32_t FLACGetSampRate(){
|
||||
return FLACMetadataBlock->sampleRate;
|
||||
}
|
||||
//----------------------------------------------------------------------------------------------------------------------
|
||||
uint32_t FLACGetBitRate(){
|
||||
if(FLACMetadataBlock->totalSamples){
|
||||
float BitsPerSamp = (float)FLACMetadataBlock->audioDataLength / (float)FLACMetadataBlock->totalSamples * 8;
|
||||
return ((uint32_t)BitsPerSamp * FLACMetadataBlock->sampleRate);
|
||||
}
|
||||
return 0;
|
||||
}
|
||||
//----------------------------------------------------------------------------------------------------------------------
|
||||
uint32_t FLACGetAudioFileDuration() {
|
||||
if(FLACGetSampRate()){
|
||||
uint32_t afd = FLACGetTotoalSamplesInStream()/ FLACGetSampRate(); // AudioFileDuration
|
||||
return afd;
|
||||
}
|
||||
return 0;
|
||||
}
|
||||
//----------------------------------------------------------------------------------------------------------------------
|
||||
int8_t decodeSubframes(int* bytesLeft){
|
||||
if(FLACFrameHeader->chanAsgn <= 7) {
|
||||
for (int ch = 0; ch < FLACMetadataBlock->numChannels; ch++)
|
||||
decodeSubframe(FLACMetadataBlock->bitsPerSample, ch, bytesLeft);
|
||||
}
|
||||
else if (8 <= FLACFrameHeader->chanAsgn && FLACFrameHeader->chanAsgn <= 10) {
|
||||
decodeSubframe(FLACMetadataBlock->bitsPerSample + (FLACFrameHeader->chanAsgn == 9 ? 1 : 0), 0, bytesLeft);
|
||||
decodeSubframe(FLACMetadataBlock->bitsPerSample + (FLACFrameHeader->chanAsgn == 9 ? 0 : 1), 1, bytesLeft);
|
||||
if(FLACFrameHeader->chanAsgn == 8) {
|
||||
for (int i = 0; i < m_blockSize; i++)
|
||||
FLACsubFramesBuff->samplesBuffer[1][i] = (
|
||||
FLACsubFramesBuff->samplesBuffer[0][i] -
|
||||
FLACsubFramesBuff->samplesBuffer[1][i]);
|
||||
}
|
||||
else if (FLACFrameHeader->chanAsgn == 9) {
|
||||
for (int i = 0; i < m_blockSize; i++)
|
||||
FLACsubFramesBuff->samplesBuffer[0][i] += FLACsubFramesBuff->samplesBuffer[1][i];
|
||||
}
|
||||
else if (FLACFrameHeader->chanAsgn == 10) {
|
||||
for (int i = 0; i < m_blockSize; i++) {
|
||||
long side = FLACsubFramesBuff->samplesBuffer[1][i];
|
||||
long right = FLACsubFramesBuff->samplesBuffer[0][i] - (side >> 1);
|
||||
FLACsubFramesBuff->samplesBuffer[1][i] = right;
|
||||
FLACsubFramesBuff->samplesBuffer[0][i] = right + side;
|
||||
}
|
||||
}
|
||||
else {
|
||||
log_e("unknown channel assignment, %i", FLACFrameHeader->chanAsgn);
|
||||
return ERR_FLAC_UNKNOWN_CHANNEL_ASSIGNMENT;
|
||||
}
|
||||
}
|
||||
else{
|
||||
log_e("Reserved channel assignment, %i", FLACFrameHeader->chanAsgn);
|
||||
return ERR_FLAC_RESERVED_CHANNEL_ASSIGNMENT;
|
||||
}
|
||||
return ERR_FLAC_NONE;
|
||||
}
|
||||
//----------------------------------------------------------------------------------------------------------------------
|
||||
int8_t decodeSubframe(uint8_t sampleDepth, uint8_t ch, int* bytesLeft) {
|
||||
int8_t ret = 0;
|
||||
readUint(1, bytesLeft);
|
||||
uint8_t type = readUint(6, bytesLeft);
|
||||
int shift = readUint(1, bytesLeft);
|
||||
if (shift == 1) {
|
||||
while (readUint(1, bytesLeft) == 0)
|
||||
shift++;
|
||||
}
|
||||
sampleDepth -= shift;
|
||||
|
||||
if(type == 0){ // Constant coding
|
||||
int16_t s= readSignedInt(sampleDepth, bytesLeft);
|
||||
for(int i=0; i < m_blockSize; i++){
|
||||
FLACsubFramesBuff->samplesBuffer[ch][i] = s;
|
||||
}
|
||||
}
|
||||
else if (type == 1) { // Verbatim coding
|
||||
for (int i = 0; i < m_blockSize; i++)
|
||||
FLACsubFramesBuff->samplesBuffer[ch][i] = readSignedInt(sampleDepth, bytesLeft);
|
||||
}
|
||||
else if (8 <= type && type <= 12){
|
||||
ret = decodeFixedPredictionSubframe(type - 8, sampleDepth, ch, bytesLeft);
|
||||
if(ret) return ret;
|
||||
}
|
||||
else if (32 <= type && type <= 63){
|
||||
ret = decodeLinearPredictiveCodingSubframe(type - 31, sampleDepth, ch, bytesLeft);
|
||||
if(ret) return ret;
|
||||
}
|
||||
else{
|
||||
return ERR_FLAC_RESERVED_SUB_TYPE;
|
||||
}
|
||||
if(shift>0){
|
||||
for (int i = 0; i < m_blockSize; i++){
|
||||
FLACsubFramesBuff->samplesBuffer[ch][i] <<= shift;
|
||||
}
|
||||
}
|
||||
return ERR_FLAC_NONE;
|
||||
}
|
||||
//----------------------------------------------------------------------------------------------------------------------
|
||||
int8_t decodeFixedPredictionSubframe(uint8_t predOrder, uint8_t sampleDepth, uint8_t ch, int* bytesLeft) {
|
||||
uint8_t ret = 0;
|
||||
for(uint8_t i = 0; i < predOrder; i++)
|
||||
FLACsubFramesBuff->samplesBuffer[ch][i] = readSignedInt(sampleDepth, bytesLeft);
|
||||
ret = decodeResiduals(predOrder, ch, bytesLeft);
|
||||
if(ret) return ret;
|
||||
coefs.clear();
|
||||
if(predOrder == 0) coefs.resize(0);
|
||||
if(predOrder == 1) coefs.push_back(1); // FIXED_PREDICTION_COEFFICIENTS
|
||||
if(predOrder == 2){coefs.push_back(2); coefs.push_back(-1);}
|
||||
if(predOrder == 3){coefs.push_back(3); coefs.push_back(-3); coefs.push_back(1);}
|
||||
if(predOrder == 4){coefs.push_back(4); coefs.push_back(-6); coefs.push_back(4); coefs.push_back(-1);}
|
||||
if(predOrder > 4) return ERR_FLAC_PREORDER_TOO_BIG; // Error: preorder > 4"
|
||||
restoreLinearPrediction(ch, 0);
|
||||
return ERR_FLAC_NONE;
|
||||
}
|
||||
//----------------------------------------------------------------------------------------------------------------------
|
||||
int8_t decodeLinearPredictiveCodingSubframe(int lpcOrder, int sampleDepth, uint8_t ch, int* bytesLeft){
|
||||
int8_t ret = 0;
|
||||
for (int i = 0; i < lpcOrder; i++)
|
||||
FLACsubFramesBuff->samplesBuffer[ch][i] = readSignedInt(sampleDepth, bytesLeft);
|
||||
int precision = readUint(4, bytesLeft) + 1;
|
||||
int shift = readSignedInt(5, bytesLeft);
|
||||
coefs.resize(0);
|
||||
for (uint8_t i = 0; i < lpcOrder; i++)
|
||||
coefs.push_back(readSignedInt(precision, bytesLeft));
|
||||
ret = decodeResiduals(lpcOrder, ch, bytesLeft);
|
||||
if(ret) return ret;
|
||||
restoreLinearPrediction(ch, shift);
|
||||
return ERR_FLAC_NONE;
|
||||
}
|
||||
//----------------------------------------------------------------------------------------------------------------------
|
||||
int8_t decodeResiduals(uint8_t warmup, uint8_t ch, int* bytesLeft) {
|
||||
|
||||
int method = readUint(2, bytesLeft);
|
||||
if (method >= 2)
|
||||
return ERR_FLAC_RESERVED_RESIDUAL_CODING; // Reserved residual coding method
|
||||
uint8_t paramBits = method == 0 ? 4 : 5;
|
||||
int escapeParam = (method == 0 ? 0xF : 0x1F);
|
||||
int partitionOrder = readUint(4, bytesLeft);
|
||||
|
||||
int numPartitions = 1 << partitionOrder;
|
||||
if (m_blockSize % numPartitions != 0)
|
||||
return ERR_FLAC_WRONG_RICE_PARTITION_NR; //Error: Block size not divisible by number of Rice partitions
|
||||
int partitionSize = m_blockSize/ numPartitions;
|
||||
|
||||
for (int i = 0; i < numPartitions; i++) {
|
||||
int start = i * partitionSize + (i == 0 ? warmup : 0);
|
||||
int end = (i + 1) * partitionSize;
|
||||
|
||||
int param = readUint(paramBits, bytesLeft);
|
||||
if (param < escapeParam) {
|
||||
for (int j = start; j < end; j++){
|
||||
FLACsubFramesBuff->samplesBuffer[ch][j] = readRiceSignedInt(param, bytesLeft);
|
||||
}
|
||||
} else {
|
||||
int numBits = readUint(5, bytesLeft);
|
||||
for (int j = start; j < end; j++){
|
||||
FLACsubFramesBuff->samplesBuffer[ch][j] = readSignedInt(numBits, bytesLeft);
|
||||
}
|
||||
}
|
||||
}
|
||||
return ERR_FLAC_NONE;
|
||||
}
|
||||
//----------------------------------------------------------------------------------------------------------------------
|
||||
void restoreLinearPrediction(uint8_t ch, uint8_t shift) {
|
||||
|
||||
for (int i = coefs.size(); i < m_blockSize; i++) {
|
||||
int32_t sum = 0;
|
||||
for (int j = 0; j < coefs.size(); j++){
|
||||
sum += FLACsubFramesBuff->samplesBuffer[ch][i - 1 - j] * coefs[j];
|
||||
}
|
||||
FLACsubFramesBuff->samplesBuffer[ch][i] += (sum >> shift);
|
||||
}
|
||||
}
|
||||
//----------------------------------------------------------------------------------------------------------------------
|
||||
int FLAC_specialIndexOf(uint8_t* base, const char* str, int baselen, bool exact){
|
||||
int result; // seek for str in buffer or in header up to baselen, not nullterninated
|
||||
if (strlen(str) > baselen) return -1; // if exact == true seekstr in buffer must have "\0" at the end
|
||||
for (int i = 0; i < baselen - strlen(str); i++){
|
||||
result = i;
|
||||
for (int j = 0; j < strlen(str) + exact; j++){
|
||||
if (*(base + i + j) != *(str + j)){
|
||||
result = -1;
|
||||
break;
|
||||
}
|
||||
}
|
||||
if (result >= 0) break;
|
||||
}
|
||||
return result;
|
||||
}
|
176
lib/ESP32-audioI2S-3.0.0/src/flac_decoder/flac_decoder.h
Normal file
@ -0,0 +1,176 @@
|
||||
/*
|
||||
* flac_decoder.h
|
||||
*
|
||||
* Created on: Jul 03,2020
|
||||
* Updated on: Jan 08,2023
|
||||
*
|
||||
* Author: wolle
|
||||
*
|
||||
* Restrictions:
|
||||
* blocksize must not exceed 8192
|
||||
* bits per sample must be 8 or 16
|
||||
* num Channels must be 1 or 2
|
||||
*
|
||||
*
|
||||
*/
|
||||
#pragma once
|
||||
#pragma GCC optimize ("Ofast")
|
||||
|
||||
#include "Arduino.h"
|
||||
|
||||
#define MAX_CHANNELS 2
|
||||
#define MAX_BLOCKSIZE 8192
|
||||
|
||||
typedef struct FLACsubFramesBuff_t{
|
||||
int32_t samplesBuffer[MAX_CHANNELS][MAX_BLOCKSIZE];
|
||||
}FLACsubframesBuffer_t;
|
||||
|
||||
enum : uint8_t {FLACDECODER_INIT, FLACDECODER_READ_IN, FLACDECODER_WRITE_OUT};
|
||||
enum : uint8_t {DECODE_FRAME, DECODE_SUBFRAMES, OUT_SAMPLES};
|
||||
enum : int8_t {FLAC_PARSE_OGG_DONE = 100,
|
||||
GIVE_NEXT_LOOP = +1,
|
||||
ERR_FLAC_NONE = 0,
|
||||
ERR_FLAC_BLOCKSIZE_TOO_BIG = -1,
|
||||
ERR_FLAC_RESERVED_BLOCKSIZE_UNSUPPORTED = -2,
|
||||
ERR_FLAC_SYNC_CODE_NOT_FOUND = -3,
|
||||
ERR_FLAC_UNKNOWN_CHANNEL_ASSIGNMENT = -4,
|
||||
ERR_FLAC_RESERVED_CHANNEL_ASSIGNMENT = -5,
|
||||
ERR_FLAC_RESERVED_SUB_TYPE = -6,
|
||||
ERR_FLAC_PREORDER_TOO_BIG = -7,
|
||||
ERR_FLAC_RESERVED_RESIDUAL_CODING = -8,
|
||||
ERR_FLAC_WRONG_RICE_PARTITION_NR = -9,
|
||||
ERR_FLAC_BITS_PER_SAMPLE_TOO_BIG = -10,
|
||||
ERR_FLAG_BITS_PER_SAMPLE_UNKNOWN = -11,
|
||||
ERR_FLAC_DECODER_ASYNC = -12};
|
||||
|
||||
typedef struct FLACMetadataBlock_t{
|
||||
// METADATA_BLOCK_STREAMINFO
|
||||
uint16_t minblocksize; // The minimum block size (in samples) used in the stream.
|
||||
//----------------------------------------------------------------------------------------
|
||||
// The maximum block size (in samples) used in the stream.
|
||||
uint16_t maxblocksize; // (Minimum blocksize == maximum blocksize) implies a fixed-blocksize stream.
|
||||
//----------------------------------------------------------------------------------------
|
||||
// The minimum frame size (in bytes) used in the stream.
|
||||
uint32_t minframesize; // May be 0 to imply the value is not known.
|
||||
//----------------------------------------------------------------------------------------
|
||||
// The maximum frame size (in bytes) used in the stream.
|
||||
uint32_t maxframesize; // May be 0 to imply the value is not known.
|
||||
//----------------------------------------------------------------------------------------
|
||||
// Sample rate in Hz. Though 20 bits are available,
|
||||
// the maximum sample rate is limited by the structure of frame headers to 655350Hz.
|
||||
uint32_t sampleRate; // Also, a value of 0 is invalid.
|
||||
//----------------------------------------------------------------------------------------
|
||||
// Number of channels FLAC supports from 1 to 8 channels
|
||||
uint8_t numChannels; // 000 : 1 channel .... 111 : 8 channels
|
||||
//----------------------------------------------------------------------------------------
|
||||
// Sample size in bits:
|
||||
// 000 : get from STREAMINFO metadata block
|
||||
// 001 : 8 bits per sample
|
||||
// 010 : 12 bits per sample
|
||||
// 011 : reserved
|
||||
// 100 : 16 bits per sample
|
||||
// 101 : 20 bits per sample
|
||||
// 110 : 24 bits per sample
|
||||
uint8_t bitsPerSample; // 111 : reserved
|
||||
//----------------------------------------------------------------------------------------
|
||||
// Total samples in stream. 'Samples' means inter-channel sample,
|
||||
// i.e. one second of 44.1Khz audio will have 44100 samples regardless of the number
|
||||
uint64_t totalSamples; // of channels. A value of zero here means the number of total samples is unknown.
|
||||
//----------------------------------------------------------------------------------------
|
||||
uint32_t audioDataLength;// is not the filelength, is only the length of the audio datablock in bytes
|
||||
|
||||
|
||||
|
||||
}FLACMetadataBlock_t;
|
||||
|
||||
|
||||
typedef struct FLACFrameHeader_t {
|
||||
// 0 : fixed-blocksize stream; frame header encodes the frame number
|
||||
uint8_t blockingStrategy; // 1 : variable-blocksize stream; frame header encodes the sample number
|
||||
//----------------------------------------------------------------------------------------
|
||||
// Block size in inter-channel samples:
|
||||
// 0000 : reserved
|
||||
// 0001 : 192 samples
|
||||
// 0010-0101 : 576 * (2^(n-2)) samples, i.e. 576/1152/2304/4608
|
||||
// 0110 : get 8 bit (blocksize-1) from end of header
|
||||
// 0111 : get 16 bit (blocksize-1) from end of header
|
||||
uint8_t blockSizeCode; // 1000-1111 : 256 * (2^(n-8)) samples, i.e. 256/512/1024/2048/4096/8192/16384/32768
|
||||
//----------------------------------------------------------------------------------------
|
||||
// 0000 : get from STREAMINFO metadata block
|
||||
// 0001 : 88.2kHz
|
||||
// 0010 : 176.4kHz
|
||||
// 0011 : 192kHz
|
||||
// 0100 : 8kHz
|
||||
// 0101 : 16kHz
|
||||
// 0110 : 22.05kHz
|
||||
// 0111 : 24kHz
|
||||
// 1000 : 32kHz
|
||||
// 1001 : 44.1kHz
|
||||
// 1010 : 48kHz
|
||||
// 1011 : 96kHz
|
||||
// 1100 : get 8 bit sample rate (in kHz) from end of header
|
||||
// 1101 : get 16 bit sample rate (in Hz) from end of header
|
||||
// 1110 : get 16 bit sample rate (in tens of Hz) from end of header
|
||||
uint8_t sampleRateCode; // 1111 : invalid, to prevent sync-fooling string of 1s
|
||||
//----------------------------------------------------------------------------------------
|
||||
// Channel assignment
|
||||
// 0000 1 channel: mono
|
||||
// 0001 2 channels: left, right
|
||||
// 0010 3 channels
|
||||
// 0011 4 channels
|
||||
// 0100 5 channels
|
||||
// 0101 6 channels
|
||||
// 0110 7 channels
|
||||
// 0111 8 channels
|
||||
// 1000 : left/side stereo: channel 0 is the left channel, channel 1 is the side(difference) channel
|
||||
// 1001 : right/side stereo: channel 0 is the side(difference) channel, channel 1 is the right channel
|
||||
// 1010 : mid/side stereo: channel 0 is the mid(average) channel, channel 1 is the side(difference) channel
|
||||
uint8_t chanAsgn; // 1011-1111 : reserved
|
||||
//----------------------------------------------------------------------------------------
|
||||
// Sample size in bits:
|
||||
// 000 : get from STREAMINFO metadata block
|
||||
// 001 : 8 bits per sample
|
||||
// 010 : 12 bits per sample
|
||||
// 011 : reserved
|
||||
// 100 : 16 bits per sample
|
||||
// 101 : 20 bits per sample
|
||||
// 110 : 24 bits per sample
|
||||
uint8_t sampleSizeCode; // 111 : reserved
|
||||
//----------------------------------------------------------------------------------------
|
||||
uint32_t totalSamples; // totalSamplesInStream
|
||||
//----------------------------------------------------------------------------------------
|
||||
uint32_t bitrate; // bitrate
|
||||
|
||||
|
||||
}FLACFrameHeader_t;
|
||||
|
||||
int FLACFindSyncWord(unsigned char *buf, int nBytes);
|
||||
boolean FLACFindMagicWord(unsigned char* buf, int nBytes);
|
||||
char* FLACgetStreamTitle();
|
||||
int FLACparseOGG(uint8_t *inbuf, int *bytesLeft);
|
||||
bool FLACDecoder_AllocateBuffers(void);
|
||||
void FLACDecoder_ClearBuffer();
|
||||
void FLACDecoder_FreeBuffers();
|
||||
void FLACSetRawBlockParams(uint8_t Chans, uint32_t SampRate, uint8_t BPS, uint32_t tsis, uint32_t AuDaLength);
|
||||
void FLACDecoderReset();
|
||||
int8_t FLACDecode(uint8_t *inbuf, int *bytesLeft, short *outbuf);
|
||||
int8_t flacDecodeFrame(uint8_t *inbuf, int *bytesLeft);
|
||||
uint16_t FLACGetOutputSamps();
|
||||
uint64_t FLACGetTotoalSamplesInStream();
|
||||
uint8_t FLACGetBitsPerSample();
|
||||
uint8_t FLACGetChannels();
|
||||
uint32_t FLACGetSampRate();
|
||||
uint32_t FLACGetBitRate();
|
||||
uint32_t FLACGetAudioFileDuration();
|
||||
uint32_t readUint(uint8_t nBits, int *bytesLeft);
|
||||
int32_t readSignedInt(int nBits, int* bytesLeft);
|
||||
int64_t readRiceSignedInt(uint8_t param, int* bytesLeft);
|
||||
void alignToByte();
|
||||
int8_t decodeSubframes(int* bytesLeft);
|
||||
int8_t decodeSubframe(uint8_t sampleDepth, uint8_t ch, int* bytesLeft);
|
||||
int8_t decodeFixedPredictionSubframe(uint8_t predOrder, uint8_t sampleDepth, uint8_t ch, int* bytesLeft);
|
||||
int8_t decodeLinearPredictiveCodingSubframe(int lpcOrder, int sampleDepth, uint8_t ch, int* bytesLeft);
|
||||
int8_t decodeResiduals(uint8_t warmup, uint8_t ch, int* bytesLeft);
|
||||
void restoreLinearPrediction(uint8_t ch, uint8_t shift);
|
||||
int FLAC_specialIndexOf(uint8_t* base, const char* str, int baselen, bool exact = false);
|
||||
|
3804
lib/ESP32-audioI2S-3.0.0/src/mp3_decoder/mp3_decoder.cpp
Normal file
513
lib/ESP32-audioI2S-3.0.0/src/mp3_decoder/mp3_decoder.h
Normal file
@ -0,0 +1,513 @@
|
||||
// based om helix mp3 decoder
|
||||
#pragma once
|
||||
|
||||
#include "Arduino.h"
|
||||
#include "assert.h"
|
||||
|
||||
static const uint8_t m_HUFF_PAIRTABS =32;
|
||||
static const uint8_t m_BLOCK_SIZE =18;
|
||||
static const uint8_t m_NBANDS =32;
|
||||
static const uint8_t m_MAX_REORDER_SAMPS =(192-126)*3; // largest critical band for short blocks (see sfBandTable)
|
||||
static const uint16_t m_VBUF_LENGTH =17*2* m_NBANDS; // for double-sized vbuf FIFO
|
||||
static const uint8_t m_MAX_SCFBD =4; // max scalefactor bands per channel
|
||||
static const uint16_t m_MAINBUF_SIZE =1940;
|
||||
static const uint8_t m_MAX_NGRAN =2; // max granules
|
||||
static const uint8_t m_MAX_NCHAN =2; // max channels
|
||||
static const uint16_t m_MAX_NSAMP =576; // max samples per channel, per granule
|
||||
|
||||
enum {
|
||||
ERR_MP3_NONE = 0,
|
||||
ERR_MP3_INDATA_UNDERFLOW = -1,
|
||||
ERR_MP3_MAINDATA_UNDERFLOW = -2,
|
||||
ERR_MP3_FREE_BITRATE_SYNC = -3,
|
||||
ERR_MP3_OUT_OF_MEMORY = -4,
|
||||
ERR_MP3_NULL_POINTER = -5,
|
||||
ERR_MP3_INVALID_FRAMEHEADER = -6,
|
||||
ERR_MP3_INVALID_SIDEINFO = -7,
|
||||
ERR_MP3_INVALID_SCALEFACT = -8,
|
||||
ERR_MP3_INVALID_HUFFCODES = -9,
|
||||
ERR_MP3_INVALID_DEQUANTIZE = -10,
|
||||
ERR_MP3_INVALID_IMDCT = -11,
|
||||
ERR_MP3_INVALID_SUBBAND = -12,
|
||||
|
||||
ERR_UNKNOWN = -9999
|
||||
};
|
||||
|
||||
typedef struct MP3FrameInfo {
|
||||
int bitrate;
|
||||
int nChans;
|
||||
int samprate;
|
||||
int bitsPerSample;
|
||||
int outputSamps;
|
||||
int layer;
|
||||
int version;
|
||||
} MP3FrameInfo_t;
|
||||
|
||||
typedef struct SFBandTable {
|
||||
int/*short*/ l[23];
|
||||
int/*short*/ s[14];
|
||||
} SFBandTable_t;
|
||||
|
||||
typedef struct BitStreamInfo {
|
||||
unsigned char *bytePtr;
|
||||
unsigned int iCache;
|
||||
int cachedBits;
|
||||
int nBytes;
|
||||
} BitStreamInfo_t;
|
||||
|
||||
typedef enum { /* map these to the corresponding 2-bit values in the frame header */
|
||||
Stereo = 0x00, /* two independent channels, but L and R frames might have different # of bits */
|
||||
Joint = 0x01, /* coupled channels - layer III: mix of M-S and intensity, Layers I/II: intensity and direct coding only */
|
||||
Dual = 0x02, /* two independent channels, L and R always have exactly 1/2 the total bitrate */
|
||||
Mono = 0x03 /* one channel */
|
||||
} StereoMode_t;
|
||||
|
||||
typedef enum { /* map to 0,1,2 to make table indexing easier */
|
||||
MPEG1 = 0,
|
||||
MPEG2 = 1,
|
||||
MPEG25 = 2
|
||||
} MPEGVersion_t;
|
||||
|
||||
typedef struct FrameHeader {
|
||||
int layer; /* layer index (1, 2, or 3) */
|
||||
int crc; /* CRC flag: 0 = disabled, 1 = enabled */
|
||||
int brIdx; /* bitrate index (0 - 15) */
|
||||
int srIdx; /* sample rate index (0 - 2) */
|
||||
int paddingBit; /* padding flag: 0 = no padding, 1 = single pad byte */
|
||||
int privateBit; /* unused */
|
||||
int modeExt; /* used to decipher joint stereo mode */
|
||||
int copyFlag; /* copyright flag: 0 = no, 1 = yes */
|
||||
int origFlag; /* original flag: 0 = copy, 1 = original */
|
||||
int emphasis; /* deemphasis mode */
|
||||
int CRCWord; /* CRC word (16 bits, 0 if crc not enabled) */
|
||||
} FrameHeader_t;
|
||||
|
||||
typedef struct SideInfoSub {
|
||||
int part23Length; /* number of bits in main data */
|
||||
int nBigvals; /* 2x this = first set of Huffman cw's (maximum amplitude can be > 1) */
|
||||
int globalGain; /* overall gain for dequantizer */
|
||||
int sfCompress; /* unpacked to figure out number of bits in scale factors */
|
||||
int winSwitchFlag; /* window switching flag */
|
||||
int blockType; /* block type */
|
||||
int mixedBlock; /* 0 = regular block (all short or long), 1 = mixed block */
|
||||
int tableSelect[3]; /* index of Huffman tables for the big values regions */
|
||||
int subBlockGain[3]; /* subblock gain offset, relative to global gain */
|
||||
int region0Count; /* 1+region0Count = num scale factor bands in first region of bigvals */
|
||||
int region1Count; /* 1+region1Count = num scale factor bands in second region of bigvals */
|
||||
int preFlag; /* for optional high frequency boost */
|
||||
int sfactScale; /* scaling of the scalefactors */
|
||||
int count1TableSelect; /* index of Huffman table for quad codewords */
|
||||
} SideInfoSub_t;
|
||||
|
||||
typedef struct SideInfo {
|
||||
int mainDataBegin;
|
||||
int privateBits;
|
||||
int scfsi[m_MAX_NCHAN][m_MAX_SCFBD]; /* 4 scalefactor bands per channel */
|
||||
} SideInfo_t;
|
||||
|
||||
typedef struct {
|
||||
int cbType; /* pure long = 0, pure short = 1, mixed = 2 */
|
||||
int cbEndS[3]; /* number nonzero short cb's, per subbblock */
|
||||
int cbEndSMax; /* max of cbEndS[] */
|
||||
int cbEndL; /* number nonzero long cb's */
|
||||
} CriticalBandInfo_t;
|
||||
|
||||
typedef struct DequantInfo {
|
||||
int workBuf[m_MAX_REORDER_SAMPS]; /* workbuf for reordering short blocks */
|
||||
} DequantInfo_t;
|
||||
|
||||
typedef struct HuffmanInfo {
|
||||
int huffDecBuf[m_MAX_NCHAN][m_MAX_NSAMP]; /* used both for decoded Huffman values and dequantized coefficients */
|
||||
int nonZeroBound[m_MAX_NCHAN]; /* number of coeffs in huffDecBuf[ch] which can be > 0 */
|
||||
int gb[m_MAX_NCHAN]; /* minimum number of guard bits in huffDecBuf[ch] */
|
||||
} HuffmanInfo_t;
|
||||
|
||||
typedef enum HuffTabType {
|
||||
noBits,
|
||||
oneShot,
|
||||
loopNoLinbits,
|
||||
loopLinbits,
|
||||
quadA,
|
||||
quadB,
|
||||
invalidTab
|
||||
} HuffTabType_t;
|
||||
|
||||
typedef struct HuffTabLookup {
|
||||
int linBits;
|
||||
int tabType; /*HuffTabType*/
|
||||
} HuffTabLookup_t;
|
||||
|
||||
typedef struct IMDCTInfo {
|
||||
int outBuf[m_MAX_NCHAN][m_BLOCK_SIZE][m_NBANDS]; /* output of IMDCT */
|
||||
int overBuf[m_MAX_NCHAN][m_MAX_NSAMP / 2]; /* overlap-add buffer (by symmetry, only need 1/2 size) */
|
||||
int numPrevIMDCT[m_MAX_NCHAN]; /* how many IMDCT's calculated in this channel on prev. granule */
|
||||
int prevType[m_MAX_NCHAN];
|
||||
int prevWinSwitch[m_MAX_NCHAN];
|
||||
int gb[m_MAX_NCHAN];
|
||||
} IMDCTInfo_t;
|
||||
|
||||
typedef struct BlockCount {
|
||||
int nBlocksLong;
|
||||
int nBlocksTotal;
|
||||
int nBlocksPrev;
|
||||
int prevType;
|
||||
int prevWinSwitch;
|
||||
int currWinSwitch;
|
||||
int gbIn;
|
||||
int gbOut;
|
||||
} BlockCount_t;
|
||||
|
||||
typedef struct ScaleFactorInfoSub { /* max bits in scalefactors = 5, so use char's to save space */
|
||||
char l[23]; /* [band] */
|
||||
char s[13][3]; /* [band][window] */
|
||||
} ScaleFactorInfoSub_t;
|
||||
|
||||
typedef struct ScaleFactorJS { /* used in MPEG 2, 2.5 intensity (joint) stereo only */
|
||||
int intensityScale;
|
||||
int slen[4];
|
||||
int nr[4];
|
||||
} ScaleFactorJS_t;
|
||||
|
||||
/* NOTE - could get by with smaller vbuf if memory is more important than speed
|
||||
* (in Subband, instead of replicating each block in FDCT32 you would do a memmove on the
|
||||
* last 15 blocks to shift them down one, a hardware style FIFO)
|
||||
*/
|
||||
typedef struct SubbandInfo {
|
||||
int vbuf[m_MAX_NCHAN * m_VBUF_LENGTH]; /* vbuf for fast DCT-based synthesis PQMF - double size for speed (no modulo indexing) */
|
||||
int vindex; /* internal index for tracking position in vbuf */
|
||||
} SubbandInfo_t;
|
||||
|
||||
typedef struct MP3DecInfo {
|
||||
/* buffer which must be large enough to hold largest possible main_data section */
|
||||
unsigned char mainBuf[m_MAINBUF_SIZE];
|
||||
/* special info for "free" bitrate files */
|
||||
int freeBitrateFlag;
|
||||
int freeBitrateSlots;
|
||||
/* user-accessible info */
|
||||
int bitrate;
|
||||
int nChans;
|
||||
int samprate;
|
||||
int nGrans; /* granules per frame */
|
||||
int nGranSamps; /* samples per granule */
|
||||
int nSlots;
|
||||
int layer;
|
||||
|
||||
int mainDataBegin;
|
||||
int mainDataBytes;
|
||||
int part23Length[m_MAX_NGRAN][m_MAX_NCHAN];
|
||||
} MP3DecInfo_t;
|
||||
|
||||
|
||||
|
||||
|
||||
/* format = Q31
|
||||
* #define M_PI 3.14159265358979323846
|
||||
* double u = 2.0 * M_PI / 9.0;
|
||||
* float c0 = sqrt(3.0) / 2.0;
|
||||
* float c1 = cos(u);
|
||||
* float c2 = cos(2*u);
|
||||
* float c3 = sin(u);
|
||||
* float c4 = sin(2*u);
|
||||
*/
|
||||
|
||||
const int c9_0 = 0x6ed9eba1;
|
||||
const int c9_1 = 0x620dbe8b;
|
||||
const int c9_2 = 0x163a1a7e;
|
||||
const int c9_3 = 0x5246dd49;
|
||||
const int c9_4 = 0x7e0e2e32;
|
||||
|
||||
|
||||
|
||||
const int c3_0 = 0x6ed9eba1; /* format = Q31, cos(pi/6) */
|
||||
const int c6[3] = { 0x7ba3751d, 0x5a82799a, 0x2120fb83 }; /* format = Q31, cos(((0:2) + 0.5) * (pi/6)) */
|
||||
|
||||
/* format = Q31
|
||||
* cos(((0:8) + 0.5) * (pi/18))
|
||||
*/
|
||||
const uint32_t c18[9] = { 0x7f834ed0, 0x7ba3751d, 0x7401e4c1, 0x68d9f964, 0x5a82799a, 0x496af3e2, 0x36185aee, 0x2120fb83, 0x0b27eb5c};
|
||||
|
||||
/* scale factor lengths (num bits) */
|
||||
const char m_SFLenTab[16][2] = { {0, 0}, {0, 1}, {0, 2}, {0, 3}, {3, 0}, {1, 1}, {1, 2}, {1, 3},
|
||||
{2, 1}, {2, 2}, {2, 3}, {3, 1}, {3, 2}, {3, 3}, {4, 2}, {4, 3}};
|
||||
|
||||
/* NRTab[size + 3*is_right][block type][partition]
|
||||
* block type index: 0 = (bt0,bt1,bt3), 1 = bt2 non-mixed, 2 = bt2 mixed
|
||||
* partition: scale factor groups (sfb1 through sfb4)
|
||||
* for block type = 2 (mixed or non-mixed) / by 3 is rolled into this table
|
||||
* (for 3 short blocks per long block)
|
||||
* see 2.4.3.2 in MPEG 2 (low sample rate) spec
|
||||
* stuff rolled into this table:
|
||||
* NRTab[x][1][y] --> (NRTab[x][1][y]) / 3
|
||||
* NRTab[x][2][>=1] --> (NRTab[x][2][>=1]) / 3 (first partition is long block)
|
||||
*/
|
||||
const char NRTab[6][3][4] = {
|
||||
{{ 6, 5, 5, 5}, {3, 3, 3, 3}, {6, 3, 3, 3}},
|
||||
{{ 6, 5, 7, 3}, {3, 3, 4, 2}, {6, 3, 4, 2}},
|
||||
{{11, 10, 0, 0}, {6, 6, 0, 0}, {6, 3, 6, 0}},
|
||||
{{ 7, 7, 7, 0}, {4, 4, 4, 0}, {6, 5, 4, 0}},
|
||||
{{ 6, 6, 6, 3}, {4, 3, 3, 2}, {6, 4, 3, 2}},
|
||||
{{ 8, 8, 5, 0}, {5, 4, 3, 0}, {6, 6, 3, 0}}
|
||||
};
|
||||
|
||||
|
||||
|
||||
/* optional pre-emphasis for high-frequency scale factor bands */
|
||||
const char preTab[22] = { 0,0,0,0,0,0,0,0,0,0,0,1,1,1,1,2,2,3,3,3,2,0 };
|
||||
|
||||
/* pow(2,-i/4) for i=0..3, Q31 format */
|
||||
const int pow14[4] PROGMEM = {
|
||||
0x7fffffff, 0x6ba27e65, 0x5a82799a, 0x4c1bf829
|
||||
};
|
||||
|
||||
|
||||
/*
|
||||
* Minimax polynomial approximation to pow(x, 4/3), over the range
|
||||
* poly43lo: x = [0.5, 0.7071]
|
||||
* poly43hi: x = [0.7071, 1.0]
|
||||
*
|
||||
* Relative error < 1E-7
|
||||
* Coefs are scaled by 4, 2, 1, 0.5, 0.25
|
||||
*/
|
||||
const unsigned int poly43lo[5] PROGMEM = { 0x29a0bda9, 0xb02e4828, 0x5957aa1b, 0x236c498d, 0xff581859 };
|
||||
const unsigned int poly43hi[5] PROGMEM = { 0x10852163, 0xd333f6a4, 0x46e9408b, 0x27c2cef0, 0xfef577b4 };
|
||||
|
||||
/* pow(2, i*4/3) as exp and frac */
|
||||
const int pow2exp[8] PROGMEM = { 14, 13, 11, 10, 9, 7, 6, 5 };
|
||||
|
||||
const int pow2frac[8] PROGMEM = {
|
||||
0x6597fa94, 0x50a28be6, 0x7fffffff, 0x6597fa94,
|
||||
0x50a28be6, 0x7fffffff, 0x6597fa94, 0x50a28be6
|
||||
};
|
||||
|
||||
const uint16_t m_HUFF_OFFSET_01= 0;
|
||||
const uint16_t m_HUFF_OFFSET_02= 9 + m_HUFF_OFFSET_01;
|
||||
const uint16_t m_HUFF_OFFSET_03= 65 + m_HUFF_OFFSET_02;
|
||||
const uint16_t m_HUFF_OFFSET_05= 65 + m_HUFF_OFFSET_03;
|
||||
const uint16_t m_HUFF_OFFSET_06=257 + m_HUFF_OFFSET_05;
|
||||
const uint16_t m_HUFF_OFFSET_07=129 + m_HUFF_OFFSET_06;
|
||||
const uint16_t m_HUFF_OFFSET_08=110 + m_HUFF_OFFSET_07;
|
||||
const uint16_t m_HUFF_OFFSET_09=280 + m_HUFF_OFFSET_08;
|
||||
const uint16_t m_HUFF_OFFSET_10= 93 + m_HUFF_OFFSET_09;
|
||||
const uint16_t m_HUFF_OFFSET_11=320 + m_HUFF_OFFSET_10;
|
||||
const uint16_t m_HUFF_OFFSET_12=296 + m_HUFF_OFFSET_11;
|
||||
const uint16_t m_HUFF_OFFSET_13=185 + m_HUFF_OFFSET_12;
|
||||
const uint16_t m_HUFF_OFFSET_15=497 + m_HUFF_OFFSET_13;
|
||||
const uint16_t m_HUFF_OFFSET_16=580 + m_HUFF_OFFSET_15;
|
||||
const uint16_t m_HUFF_OFFSET_24=651 + m_HUFF_OFFSET_16;
|
||||
|
||||
const int huffTabOffset[m_HUFF_PAIRTABS] PROGMEM = {
|
||||
0, m_HUFF_OFFSET_01, m_HUFF_OFFSET_02, m_HUFF_OFFSET_03,
|
||||
0, m_HUFF_OFFSET_05, m_HUFF_OFFSET_06, m_HUFF_OFFSET_07,
|
||||
m_HUFF_OFFSET_08, m_HUFF_OFFSET_09, m_HUFF_OFFSET_10, m_HUFF_OFFSET_11,
|
||||
m_HUFF_OFFSET_12, m_HUFF_OFFSET_13, 0, m_HUFF_OFFSET_15,
|
||||
m_HUFF_OFFSET_16, m_HUFF_OFFSET_16, m_HUFF_OFFSET_16, m_HUFF_OFFSET_16,
|
||||
m_HUFF_OFFSET_16, m_HUFF_OFFSET_16, m_HUFF_OFFSET_16, m_HUFF_OFFSET_16,
|
||||
m_HUFF_OFFSET_24, m_HUFF_OFFSET_24, m_HUFF_OFFSET_24, m_HUFF_OFFSET_24,
|
||||
m_HUFF_OFFSET_24, m_HUFF_OFFSET_24, m_HUFF_OFFSET_24, m_HUFF_OFFSET_24,};
|
||||
|
||||
const HuffTabLookup_t huffTabLookup[m_HUFF_PAIRTABS] PROGMEM = {
|
||||
{ 0, noBits },
|
||||
{ 0, oneShot },
|
||||
{ 0, oneShot },
|
||||
{ 0, oneShot },
|
||||
{ 0, invalidTab },
|
||||
{ 0, oneShot },
|
||||
{ 0, oneShot },
|
||||
{ 0, loopNoLinbits },
|
||||
{ 0, loopNoLinbits },
|
||||
{ 0, loopNoLinbits },
|
||||
{ 0, loopNoLinbits },
|
||||
{ 0, loopNoLinbits },
|
||||
{ 0, loopNoLinbits },
|
||||
{ 0, loopNoLinbits },
|
||||
{ 0, invalidTab },
|
||||
{ 0, loopNoLinbits },
|
||||
{ 1, loopLinbits },
|
||||
{ 2, loopLinbits },
|
||||
{ 3, loopLinbits },
|
||||
{ 4, loopLinbits },
|
||||
{ 6, loopLinbits },
|
||||
{ 8, loopLinbits },
|
||||
{ 10, loopLinbits },
|
||||
{ 13, loopLinbits },
|
||||
{ 4, loopLinbits },
|
||||
{ 5, loopLinbits },
|
||||
{ 6, loopLinbits },
|
||||
{ 7, loopLinbits },
|
||||
{ 8, loopLinbits },
|
||||
{ 9, loopLinbits },
|
||||
{ 11, loopLinbits },
|
||||
{ 13, loopLinbits },
|
||||
};
|
||||
|
||||
|
||||
const int quadTabOffset[2] PROGMEM = {0, 64};
|
||||
const int quadTabMaxBits[2] PROGMEM = {6, 4};
|
||||
|
||||
/* indexing = [version][samplerate index]
|
||||
* sample rate of frame (Hz)
|
||||
*/
|
||||
const int samplerateTab[3][3] PROGMEM = {
|
||||
{ 44100, 48000, 32000 }, /* MPEG-1 */
|
||||
{ 22050, 24000, 16000 }, /* MPEG-2 */
|
||||
{ 11025, 12000, 8000 }, /* MPEG-2.5 */
|
||||
};
|
||||
|
||||
|
||||
|
||||
/* indexing = [version][layer]
|
||||
* number of samples in one frame (per channel)
|
||||
*/
|
||||
const int/*short*/samplesPerFrameTab[3][3] PROGMEM = { { 384, 1152, 1152 }, /* MPEG1 */
|
||||
{ 384, 1152, 576 }, /* MPEG2 */
|
||||
{ 384, 1152, 576 }, /* MPEG2.5 */
|
||||
};
|
||||
|
||||
/* layers 1, 2, 3 */
|
||||
const short bitsPerSlotTab[3] = { 32, 8, 8 };
|
||||
|
||||
/* indexing = [version][mono/stereo]
|
||||
* number of bytes in side info section of bitstream
|
||||
*/
|
||||
const int/*short*/sideBytesTab[3][2] PROGMEM = { { 17, 32 }, /* MPEG-1: mono, stereo */
|
||||
{ 9, 17 }, /* MPEG-2: mono, stereo */
|
||||
{ 9, 17 }, /* MPEG-2.5: mono, stereo */
|
||||
};
|
||||
|
||||
/* indexing = [version][sampleRate][long (.l) or short (.s) block]
|
||||
* sfBandTable[v][s].l[cb] = index of first bin in critical band cb (long blocks)
|
||||
* sfBandTable[v][s].s[cb] = index of first bin in critical band cb (short blocks)
|
||||
*/
|
||||
const SFBandTable_t sfBandTable[3][3] PROGMEM = {
|
||||
{ /* MPEG-1 (44, 48, 32 kHz) */
|
||||
{ {0, 4, 8, 12, 16, 20, 24, 30, 36, 44, 52, 62, 74, 90, 110, 134, 162, 196, 238, 288, 342, 418, 576 },
|
||||
{0, 4, 8, 12, 16, 22, 30, 40, 52, 66, 84, 106, 136, 192} },
|
||||
{ {0, 4, 8, 12, 16, 20, 24, 30, 36, 42, 50, 60, 72, 88, 106, 128, 156, 190, 230, 276, 330, 384, 576 },
|
||||
{0, 4, 8, 12, 16, 22, 28, 38, 50, 64, 80, 100, 126, 192} },
|
||||
{ {0, 4, 8, 12, 16, 20, 24, 30, 36, 44, 54, 66, 82, 102, 126, 156, 194, 240, 296, 364, 448, 550, 576 },
|
||||
{0, 4, 8, 12, 16, 22, 30, 42, 58, 78, 104, 138, 180, 192} } },
|
||||
{ /* MPEG-2 (22, 24, 16 kHz) */
|
||||
{ {0, 6, 12, 18, 24, 30, 36, 44, 54, 66, 80, 96, 116, 140, 168, 200, 238, 284, 336, 396, 464, 522, 576 },
|
||||
{0, 4, 8, 12, 18, 24, 32, 42, 56, 74, 100, 132, 174, 192} },
|
||||
{ {0, 6, 12, 18, 24, 30, 36, 44, 54, 66, 80, 96, 114, 136, 162, 194, 232, 278, 332, 394, 464, 540, 576 },
|
||||
{0, 4, 8, 12, 18, 26, 36, 48, 62, 80, 104, 136, 180, 192} },
|
||||
{ {0, 6, 12, 18, 24, 30, 36, 44, 54, 66, 80, 96, 116, 140, 168, 200, 238, 284, 336, 396, 464, 522, 576 },
|
||||
{0, 4, 8, 12, 18, 26, 36, 48, 62, 80, 104, 134, 174, 192} }, },
|
||||
{ /* MPEG-2.5 (11, 12, 8 kHz) */
|
||||
{ {0, 6, 12, 18, 24, 30, 36, 44, 54, 66, 80, 96, 116, 140, 168, 200, 238, 284, 336, 396, 464, 522, 576 },
|
||||
{0, 4, 8, 12, 18, 26, 36, 48, 62, 80, 104, 134, 174, 192 } },
|
||||
{ {0, 6, 12, 18, 24, 30, 36, 44, 54, 66, 80, 96, 116, 140, 168, 200, 238, 284, 336, 396, 464, 522, 576 },
|
||||
{0, 4, 8, 12, 18, 26, 36, 48, 62, 80, 104, 134, 174, 192 } },
|
||||
{ {0, 12, 24, 36, 48, 60, 72, 88, 108, 132, 160, 192, 232, 280, 336, 400, 476, 566, 568, 570, 572, 574, 576 },
|
||||
{0, 8, 16, 24, 36, 52, 72, 96, 124, 160, 162, 164, 166, 192 } }, },
|
||||
};
|
||||
|
||||
|
||||
/* indexing = [intensity scale on/off][left/right]
|
||||
* format = Q30, range = [0.0, 1.414]
|
||||
*
|
||||
* illegal intensity position scalefactors (see comments on ISFMpeg1)
|
||||
*/
|
||||
const int ISFIIP[2][2] PROGMEM = {
|
||||
{0x40000000, 0x00000000}, /* mid-side off */
|
||||
{0x40000000, 0x40000000}, /* mid-side on */
|
||||
};
|
||||
|
||||
const unsigned char uniqueIDTab[8] = {0x5f, 0x4b, 0x43, 0x5f, 0x5f, 0x4a, 0x52, 0x5f};
|
||||
|
||||
/* anti-alias coefficients - see spec Annex B, table 3-B.9
|
||||
* csa[0][i] = CSi, csa[1][i] = CAi
|
||||
* format = Q31
|
||||
*/
|
||||
const uint32_t csa[8][2] PROGMEM = {
|
||||
{0x6dc253f0, 0xbe2500aa},
|
||||
{0x70dcebe4, 0xc39e4949},
|
||||
{0x798d6e73, 0xd7e33f4a},
|
||||
{0x7ddd40a7, 0xe8b71176},
|
||||
{0x7f6d20b7, 0xf3e4fe2f},
|
||||
{0x7fe47e40, 0xfac1a3c7},
|
||||
{0x7ffcb263, 0xfe2ebdc6},
|
||||
{0x7fffc694, 0xff86c25d},
|
||||
};
|
||||
|
||||
/* format = Q30, right shifted by 12 (sign bits only in top 12 - undo this when rounding to short)
|
||||
* this is to enable early-terminating multiplies on ARM
|
||||
* range = [-1.144287109, 1.144989014]
|
||||
* max gain of filter (per output sample) ~= 2.731
|
||||
*
|
||||
* new (properly sign-flipped) values
|
||||
* - these actually are correct to 32 bits, (floating-pt coefficients in spec
|
||||
* chosen such that only ~20 bits are required)
|
||||
*
|
||||
* Reordering - see table 3-B.3 in spec (appendix B)
|
||||
*
|
||||
* polyCoef[i] =
|
||||
* D[ 0, 32, 64, ... 480], i = [ 0, 15]
|
||||
* D[ 1, 33, 65, ... 481], i = [ 16, 31]
|
||||
* D[ 2, 34, 66, ... 482], i = [ 32, 47]
|
||||
* ...
|
||||
* D[15, 47, 79, ... 495], i = [240,255]
|
||||
*
|
||||
* also exploits symmetry: D[i] = -D[512 - i], for i = [1, 255]
|
||||
*
|
||||
* polyCoef[256, 257, ... 263] are for special case of sample 16 (out of 0)
|
||||
* see PolyphaseStereo() and PolyphaseMono()
|
||||
*/
|
||||
|
||||
// prototypes
|
||||
bool MP3Decoder_AllocateBuffers(void);
|
||||
void MP3Decoder_FreeBuffers();
|
||||
int MP3Decode( unsigned char *inbuf, int *bytesLeft, short *outbuf, int useSize);
|
||||
void MP3GetLastFrameInfo();
|
||||
int MP3GetNextFrameInfo(unsigned char *buf);
|
||||
int MP3FindSyncWord(unsigned char *buf, int nBytes);
|
||||
int MP3GetSampRate();
|
||||
int MP3GetChannels();
|
||||
int MP3GetBitsPerSample();
|
||||
int MP3GetBitrate();
|
||||
int MP3GetOutputSamps();
|
||||
|
||||
//internally used
|
||||
void MP3Decoder_ClearBuffer(void);
|
||||
void PolyphaseMono(short *pcm, int *vbuf, const uint32_t *coefBase);
|
||||
void PolyphaseStereo(short *pcm, int *vbuf, const uint32_t *coefBase);
|
||||
void SetBitstreamPointer(BitStreamInfo_t *bsi, int nBytes, unsigned char *buf);
|
||||
unsigned int GetBits(BitStreamInfo_t *bsi, int nBits);
|
||||
int CalcBitsUsed(BitStreamInfo_t *bsi, unsigned char *startBuf, int startOffset);
|
||||
int DequantChannel(int *sampleBuf, int *workBuf, int *nonZeroBound, SideInfoSub_t *sis, ScaleFactorInfoSub_t *sfis, CriticalBandInfo_t *cbi);
|
||||
void MidSideProc(int x[m_MAX_NCHAN][m_MAX_NSAMP], int nSamps, int mOut[2]);
|
||||
void IntensityProcMPEG1(int x[m_MAX_NCHAN][m_MAX_NSAMP], int nSamps, ScaleFactorInfoSub_t *sfis, CriticalBandInfo_t *cbi, int midSideFlag, int mixFlag, int mOut[2]);
|
||||
void IntensityProcMPEG2(int x[m_MAX_NCHAN][m_MAX_NSAMP], int nSamps, ScaleFactorInfoSub_t *sfis, CriticalBandInfo_t *cbi, ScaleFactorJS_t *sfjs, int midSideFlag, int mixFlag, int mOut[2]);
|
||||
void FDCT32(int *x, int *d, int offset, int oddBlock, int gb);// __attribute__ ((section (".data")));
|
||||
void FreeBuffers();
|
||||
int CheckPadBit();
|
||||
int UnpackFrameHeader(unsigned char *buf);
|
||||
int UnpackSideInfo(unsigned char *buf);
|
||||
int DecodeHuffman( unsigned char *buf, int *bitOffset, int huffBlockBits, int gr, int ch);
|
||||
int MP3Dequantize( int gr);
|
||||
int IMDCT( int gr, int ch);
|
||||
int UnpackScaleFactors( unsigned char *buf, int *bitOffset, int bitsAvail, int gr, int ch);
|
||||
int Subband(short *pcmBuf);
|
||||
short ClipToShort(int x, int fracBits);
|
||||
void RefillBitstreamCache(BitStreamInfo_t *bsi);
|
||||
void UnpackSFMPEG1(BitStreamInfo_t *bsi, SideInfoSub_t *sis, ScaleFactorInfoSub_t *sfis, int *scfsi, int gr, ScaleFactorInfoSub_t *sfisGr0);
|
||||
void UnpackSFMPEG2(BitStreamInfo_t *bsi, SideInfoSub_t *sis, ScaleFactorInfoSub_t *sfis, int gr, int ch, int modeExt, ScaleFactorJS_t *sfjs);
|
||||
int MP3FindFreeSync(unsigned char *buf, unsigned char firstFH[4], int nBytes);
|
||||
void MP3ClearBadFrame( short *outbuf);
|
||||
int DecodeHuffmanPairs(int *xy, int nVals, int tabIdx, int bitsLeft, unsigned char *buf, int bitOffset);
|
||||
int DecodeHuffmanQuads(int *vwxy, int nVals, int tabIdx, int bitsLeft, unsigned char *buf, int bitOffset);
|
||||
int DequantBlock(int *inbuf, int *outbuf, int num, int scale);
|
||||
void AntiAlias(int *x, int nBfly);
|
||||
void WinPrevious(int *xPrev, int *xPrevWin, int btPrev);
|
||||
int FreqInvertRescale(int *y, int *xPrev, int blockIdx, int es);
|
||||
void idct9(int *x);
|
||||
int IMDCT36(int *xCurr, int *xPrev, int *y, int btCurr, int btPrev, int blockIdx, int gb);
|
||||
void imdct12(int *x, int *out);
|
||||
int IMDCT12x3(int *xCurr, int *xPrev, int *y, int btPrev, int blockIdx, int gb);
|
||||
int HybridTransform(int *xCurr, int *xPrev, int y[m_BLOCK_SIZE][m_NBANDS], SideInfoSub_t *sis, BlockCount_t *bc);
|
||||
inline uint64_t SAR64(uint64_t x, int n) {return x >> n;}
|
||||
inline int MULSHIFT32(int x, int y) { int z; z = (uint64_t) x * (uint64_t) y >> 32; return z;}
|
||||
inline uint64_t MADD64(uint64_t sum64, int x, int y) {sum64 += (uint64_t) x * (uint64_t) y; return sum64;}/* returns 64-bit value in [edx:eax] */
|
||||
inline uint64_t xSAR64(uint64_t x, int n){return x >> n;}
|
||||
inline int FASTABS(int x){ return __builtin_abs(x);} //xtensa has a fast abs instruction //fb
|
||||
#define CLZ(x) __builtin_clz(x) //fb
|
3742
lib/ESP32-audioI2S-3.0.0/src/opus_decoder/celt.cpp
Normal file
587
lib/ESP32-audioI2S-3.0.0/src/opus_decoder/celt.h
Normal file
@ -0,0 +1,587 @@
|
||||
/* Copyright (c) 2007-2008 CSIRO
|
||||
Copyright (c) 2007-2009 Xiph.Org Foundation
|
||||
Copyright (c) 2008 Gregory Maxwell
|
||||
Written by Jean-Marc Valin and Gregory Maxwell */
|
||||
/**
|
||||
@file celt.h
|
||||
@brief Contains all the functions for encoding and decoding audio
|
||||
*/
|
||||
|
||||
/*
|
||||
Redistribution and use in source and binary forms, with or without
|
||||
modification, are permitted provided that the following conditions
|
||||
are met:
|
||||
|
||||
- Redistributions of source code must retain the above copyright
|
||||
notice, this list of conditions and the following disclaimer.
|
||||
|
||||
- Redistributions in binary form must reproduce the above copyright
|
||||
notice, this list of conditions and the following disclaimer in the
|
||||
documentation and/or other materials provided with the distribution.
|
||||
|
||||
THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS
|
||||
``AS IS'' AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT
|
||||
LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR
|
||||
A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER
|
||||
OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL,
|
||||
EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO,
|
||||
PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR
|
||||
PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF
|
||||
LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING
|
||||
NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS
|
||||
SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
|
||||
*/
|
||||
|
||||
#pragma once
|
||||
#pragma GCC optimize ("Os")
|
||||
|
||||
#include "Arduino.h"
|
||||
|
||||
#define OPUS_RESET_STATE 4028
|
||||
#define OPUS_GET_SAMPLE_RATE_REQUEST 4029
|
||||
|
||||
#define LEAK_BANDS 19
|
||||
|
||||
typedef struct {
|
||||
int32_t valid;
|
||||
float tonality;
|
||||
float tonality_slope;
|
||||
float noisiness;
|
||||
float activity;
|
||||
float music_prob;
|
||||
float music_prob_min;
|
||||
float music_prob_max;
|
||||
int32_t bandwidth;
|
||||
float activity_probability;
|
||||
float max_pitch_ratio;
|
||||
/* Store as Q6 char to save space. */
|
||||
uint8_t leak_boost[LEAK_BANDS];
|
||||
} AnalysisInfo;
|
||||
|
||||
/*OPT: ec_window must be at least 32 bits, but if you have fast arithmetic on a larger type, you can speed up the
|
||||
decoder by using it here.*/
|
||||
|
||||
typedef struct CELTMode CELTMode;
|
||||
typedef struct CELTDecoder CELTDecoder;
|
||||
|
||||
typedef struct _ec_ctx {
|
||||
uint8_t *buf; /*Buffered input/output.*/
|
||||
uint32_t storage; /*The size of the buffer.*/
|
||||
uint32_t end_offs; /*The offset at which the last byte containing raw bits was read/written.*/
|
||||
uint32_t end_window; /*Bits that will be read from/written at the end.*/
|
||||
int32_t nend_bits; /*Number of valid bits in end_window.*/
|
||||
int32_t nbits_total;
|
||||
uint32_t offs; /*The offset at which the next range coder byte will be read/written.*/
|
||||
uint32_t rng; /*The number of values in the current range.*/
|
||||
uint32_t val;
|
||||
uint32_t ext;
|
||||
int32_t rem; /*A buffered input/output symbol, awaiting carry propagation.*/
|
||||
int32_t error; /*Nonzero if an error occurred.*/
|
||||
} ec_ctx_t;
|
||||
|
||||
extern ec_ctx_t s_ec;
|
||||
extern const uint8_t cache_bits50[392];
|
||||
extern const int16_t cache_index50[105];
|
||||
|
||||
typedef struct _band_ctx{
|
||||
int32_t encode;
|
||||
int32_t resynth;
|
||||
int32_t i;
|
||||
int32_t intensity;
|
||||
int32_t spread;
|
||||
int32_t tf_change;
|
||||
int32_t remaining_bits;
|
||||
uint32_t seed;
|
||||
int32_t theta_round;
|
||||
int32_t disable_inv;
|
||||
int32_t avoid_split_noise;
|
||||
} band_ctx_t;
|
||||
|
||||
struct split_ctx{
|
||||
int32_t inv;
|
||||
int32_t imid;
|
||||
int32_t iside;
|
||||
int32_t delta;
|
||||
int32_t itheta;
|
||||
int32_t qalloc;
|
||||
};
|
||||
|
||||
struct CELTDecoder {
|
||||
const CELTMode *mode;
|
||||
int32_t overlap;
|
||||
int32_t channels;
|
||||
int32_t stream_channels;
|
||||
|
||||
int32_t start, end;
|
||||
int32_t signalling;
|
||||
int32_t disable_inv;
|
||||
|
||||
uint32_t rng;
|
||||
int32_t error;
|
||||
int32_t postfilter_period;
|
||||
int32_t postfilter_period_old;
|
||||
int16_t postfilter_gain;
|
||||
int16_t postfilter_gain_old;
|
||||
int32_t postfilter_tapset;
|
||||
int32_t postfilter_tapset_old;
|
||||
|
||||
int32_t preemph_memD[2];
|
||||
|
||||
int32_t _decode_mem[1]; /* Size = channels*(DECODE_BUFFER_SIZE+mode->overlap) */
|
||||
/* int16_t lpc[], Size = channels*LPC_ORDER */
|
||||
/* int16_t oldEBands[], Size = 2*mode->nbEBands */
|
||||
/* int16_t oldLogE[], Size = 2*mode->nbEBands */
|
||||
/* int16_t oldLogE2[], Size = 2*mode->nbEBands */
|
||||
/* int16_t backgroundLogE[], Size = 2*mode->nbEBands */
|
||||
};
|
||||
|
||||
typedef struct {
|
||||
int32_t r;
|
||||
int32_t i;
|
||||
}kiss_fft_cpx;
|
||||
|
||||
typedef struct {
|
||||
int16_t r;
|
||||
int16_t i;
|
||||
}kiss_twiddle_cpx;
|
||||
|
||||
#define MAXFACTORS 8
|
||||
|
||||
typedef struct kiss_fft_state{
|
||||
int32_t nfft;
|
||||
int16_t scale;
|
||||
int32_t scale_shift;
|
||||
int32_t shift;
|
||||
int16_t factors[2*MAXFACTORS];
|
||||
const int16_t *bitrev;
|
||||
const kiss_twiddle_cpx *twiddles;
|
||||
} kiss_fft_state;
|
||||
|
||||
typedef struct {
|
||||
int32_t n;
|
||||
int32_t maxshift;
|
||||
const kiss_fft_state *kfft[4];
|
||||
const int16_t * trig;
|
||||
} mdct_lookup_t;
|
||||
|
||||
|
||||
|
||||
/** Mode definition (opaque)
|
||||
@brief Mode definition
|
||||
*/
|
||||
struct CELTMode {
|
||||
int32_t Fs;
|
||||
int32_t overlap;
|
||||
int32_t nbEBands;
|
||||
int32_t effEBands;
|
||||
int16_t preemph[4];
|
||||
int32_t maxLM;
|
||||
int32_t nbShortMdcts;
|
||||
int32_t shortMdctSize;
|
||||
int32_t nbAllocVectors; /**< Number of lines in the matrix below */
|
||||
};
|
||||
|
||||
extern const CELTMode m_CELTMode;
|
||||
|
||||
#define min(a,b) ((a)<(b)?(a):(b))
|
||||
#define max(a,b) ((a)>(b)?(a):(b))
|
||||
|
||||
inline int32_t S_MUL(int32_t a, int16_t b){return (int64_t)b * a >> 15;}
|
||||
#define C_MUL(m,a,b) do{ (m).r = SUB32_ovflw(S_MUL((a).r,(b).r) , S_MUL((a).i,(b).i)); \
|
||||
(m).i = ADD32_ovflw(S_MUL((a).r,(b).i) , S_MUL((a).i,(b).r)); }while(0)
|
||||
|
||||
#define C_MULBYSCALAR( c, s ) do{ (c).r = S_MUL( (c).r , s ) ; (c).i = S_MUL( (c).i , s ) ; }while(0)
|
||||
|
||||
#define DIVSCALAR(x,k) (x) = S_MUL( x, (32767-((k)>>1))/(k)+1 )
|
||||
|
||||
#define C_FIXDIV(c,div) do { DIVSCALAR( (c).r , div); DIVSCALAR( (c).i , div); }while (0)
|
||||
|
||||
#define C_ADD( res, a,b) do {(res).r=ADD32_ovflw((a).r,(b).r); (res).i=ADD32_ovflw((a).i,(b).i); }while(0)
|
||||
|
||||
#define C_SUB( res, a,b) do {(res).r=SUB32_ovflw((a).r,(b).r); (res).i=SUB32_ovflw((a).i,(b).i); }while(0)
|
||||
#define C_ADDTO( res , a) do {(res).r = ADD32_ovflw((res).r, (a).r); (res).i = ADD32_ovflw((res).i,(a).i); }while(0)
|
||||
|
||||
#define VERY_LARGE16 ((int16_t)32767)
|
||||
#define Q15_ONE ((int16_t)32767)
|
||||
|
||||
|
||||
#define EC_MINI(_a,_b) ((_a)+(((_b)-(_a))&-((_b)<(_a))))
|
||||
#define EC_CLZ0 ((int32_t)sizeof(uint32_t)*CHAR_BIT)
|
||||
#define EC_CLZ(_x) (__builtin_clz(_x))
|
||||
#define EC_ILOG(_x) (EC_CLZ0-EC_CLZ(_x))
|
||||
|
||||
/** Multiply a 16-bit signed value by a 16-bit uint32_t value. The result is a 32-bit signed value */
|
||||
#define MULT16_16SU(a,b) ((int32_t)(int16_t)(a)*(int32_t)(uint16_t)(b))
|
||||
|
||||
/** 16x32 multiplication, followed by a 16-bit shift right. Results fits in 32 bits */
|
||||
inline int32_t MULT16_32_Q16(int64_t a, int64_t b){return (int32_t) (a * b) >> 16;}
|
||||
|
||||
/** 16x32 multiplication, followed by a 16-bit shift right (round-to-nearest). Results fits in 32 bits */
|
||||
#define MULT16_32_P16(a,b) ((int32_t)PSHR((int64_t)((int16_t)(a))*(b),16))
|
||||
|
||||
/** 16x32 multiplication, followed by a 15-bit shift right. Results fits in 32 bits */
|
||||
inline int32_t MULT16_32_Q15(int16_t a, int32_t b){return (int64_t)a * b >> 15;}
|
||||
|
||||
/** 32x32 multiplication, followed by a 31-bit shift right. Results fits in 32 bits */
|
||||
#define MULT32_32_Q31(a,b) ((int32_t)((int64_t)(a)*(int64_t)(b) >> 31))
|
||||
|
||||
/** Compile-time conversion of float constant to 16-bit value */
|
||||
#define QCONST16(x,bits) ((int16_t)(0.5L+(x)*(((int32_t)1)<<(bits))))
|
||||
|
||||
/** Compile-time conversion of float constant to 32-bit value */
|
||||
#define QCONST32(x,bits) ((int32_t)(0.5L+(x)*(((int32_t)1)<<(bits))))
|
||||
|
||||
/** Change a 16-bit value into a 32-bit value */
|
||||
#define EXTEND32(x) ((int32_t)(x))
|
||||
|
||||
/** Arithmetic shift-right of a 16-bit value */
|
||||
#define SHR16(a,shift) ((a) >> (shift))
|
||||
/** Arithmetic shift-left of a 16-bit value */
|
||||
#define SHL16(a,shift) ((int16_t)((uint16_t)(a)<<(shift)))
|
||||
/** Arithmetic shift-right of a 32-bit value */
|
||||
#define SHR32(a,shift) ((a) >> (shift))
|
||||
/** Arithmetic shift-left of a 32-bit value */
|
||||
#define SHL32(a,shift) ((int32_t)((uint32_t)(a)<<(shift)))
|
||||
|
||||
/** 32-bit arithmetic shift right with rounding-to-nearest instead of rounding down */
|
||||
inline int32_t PSHR(int32_t a, uint32_t shift){return (a + ((int32_t)1 << (shift >> 1))) >> shift;}
|
||||
|
||||
/** 32-bit arithmetic shift right where the argument can be negative */
|
||||
#define VSHR32(a, shift) (((shift)>0) ? SHR32(a, shift) : SHL32(a, -(shift)))
|
||||
|
||||
#define SATURATE(x,a) (((x)>(a) ? (a) : (x)<-(a) ? -(a) : (x)))
|
||||
|
||||
#define SATURATE16(x) ((int16_t)((x)>32767 ? 32767 : (x)<-32768 ? -32768 : (x)))
|
||||
|
||||
/** Shift by a and round-to-neareast 32-bit value. Result is a 16-bit value */
|
||||
#define ROUND16(x,a) ((int16_t)(PSHR((x),(a))))
|
||||
/** Shift by a and round-to-neareast 32-bit value. Result is a saturated 16-bit value */
|
||||
#define SROUND16(x,a) (int16_t)(SATURATE(PSHR(x,a), 32767));
|
||||
|
||||
/** Divide by two */
|
||||
#define HALF16(x) (SHR16(x,1))
|
||||
#define HALF32(x) (SHR32(x,1))
|
||||
|
||||
/** Add two 16-bit values */
|
||||
#define ADD16(a,b) ((int16_t)((int16_t)(a)+(int16_t)(b)))
|
||||
/** Subtract two 16-bit values */
|
||||
#define SUB16(a,b) ((int16_t)(a)-(int16_t)(b))
|
||||
/** Add two 32-bit values */
|
||||
#define ADD32(a,b) ((int32_t)(a)+(int32_t)(b))
|
||||
/** Subtract two 32-bit values */
|
||||
#define SUB32(a,b) ((int32_t)(a)-(int32_t)(b))
|
||||
|
||||
/** Add two 32-bit values, ignore any overflows */
|
||||
#define ADD32_ovflw(a,b) ((int32_t)((uint32_t)(a)+(uint32_t)(b)))
|
||||
/** Subtract two 32-bit values, ignore any overflows */
|
||||
#define SUB32_ovflw(a,b) ((int32_t)((uint32_t)(a)-(uint32_t)(b)))
|
||||
/* Avoid MSVC warning C4146: unary minus operator applied to uint32_t type */
|
||||
/** Negate 32-bit value, ignore any overflows */
|
||||
#define NEG32_ovflw(a) ((int32_t)(0-(uint32_t)(a)))
|
||||
|
||||
/** 16x16 multiplication where the result fits in 16 bits */
|
||||
#define MULT16_16_16(a,b) ((((int16_t)(a))*((int16_t)(b))))
|
||||
|
||||
/* (int32_t)(int16_t) gives TI compiler a hint that it's 16x16->32 multiply */
|
||||
/** 16x16 multiplication where the result fits in 32 bits */
|
||||
#define MULT16_16(a,b) (((int32_t)(int16_t)(a))*((int32_t)(int16_t)(b)))
|
||||
|
||||
/** 16x16 multiply-add where the result fits in 32 bits */
|
||||
#define MAC16_16(c,a,b) (ADD32((c),MULT16_16((a),(b))))
|
||||
/** 16x32 multiply, followed by a 15-bit shift right and 32-bit add.
|
||||
b must fit in 31 bits.
|
||||
Result fits in 32 bits. */
|
||||
#define MAC16_32_Q15(c,a,b) ADD32((c),ADD32(MULT16_16((a),(b) >> 15), MULT16_16((a),((b)&0x00007fff)) >> 15))
|
||||
|
||||
/** 16x32 multiplication, followed by a 16-bit shift right and 32-bit add.
|
||||
Results fits in 32 bits */
|
||||
#define MAC16_32_Q16(c,a,b) ADD32((c),ADD32(MULT16_16((a),(b) >> 16), MULT16_16SU((a),((b)&0x0000ffff)) >> 16))
|
||||
|
||||
#define MULT16_16_Q11_32(a,b) (MULT16_16((a),(b)) >> 11)
|
||||
#define MULT16_16_Q11(a,b) (MULT16_16((a),(b)) >> 11)
|
||||
#define MULT16_16_Q13(a,b) (MULT16_16((a),(b)) >> 13)
|
||||
#define MULT16_16_Q14(a,b) (MULT16_16((a),(b)) >> 14)
|
||||
#define MULT16_16_Q15(a,b) (MULT16_16((a),(b)) >> 15)
|
||||
|
||||
#define MULT16_16_P13(a,b) (ADD32(4096, MULT16_16((a),(b))) >> 13)
|
||||
#define MULT16_16_P14(a,b) (ADD32(8192, MULT16_16((a),(b))) >> 14)
|
||||
#define MULT16_16_P15(a,b) (ADD32(16384,MULT16_16((a),(b))) >> 15)
|
||||
|
||||
/** Divide a 32-bit value by a 16-bit value. Result fits in 16 bits */
|
||||
#define DIV32_16(a,b) ((int16_t)(((int32_t)(a))/((int16_t)(b))))
|
||||
|
||||
/** Divide a 32-bit value by a 32-bit value. Result fits in 32 bits */
|
||||
#define DIV32(a,b) (((int32_t)(a))/((int32_t)(b)))
|
||||
int32_t celt_rcp(int32_t x);
|
||||
#define celt_div(a,b) MULT32_32_Q31((int32_t)(a),celt_rcp(b))
|
||||
#define MAX_PERIOD 1024
|
||||
#define OPUS_MOVE(dst, src, n) (memmove((dst), (src), (n)*sizeof(*(dst)) + 0*((dst)-(src)) ))
|
||||
#define ALLOC_STEPS 6
|
||||
|
||||
/* Multiplies two 16-bit fractional values. Bit-exactness of this macro is important */
|
||||
#define FRAC_MUL16(a,b) ((16384+((int32_t)(int16_t)(a)*(int16_t)(b)))>>15)
|
||||
|
||||
#define QTHETA_OFFSET 4
|
||||
#define QTHETA_OFFSET_TWOPHASE 16
|
||||
#define MAX_FINE_BITS 8
|
||||
#define MAX_PSEUDO 40
|
||||
#define LOG_MAX_PSEUDO 6
|
||||
#define ALLOC_NONE 1
|
||||
|
||||
/* Prototypes and inlines*/
|
||||
|
||||
inline int16_t SAT16(int32_t x) {
|
||||
if(x > INT16_MAX) return INT16_MAX;
|
||||
if(x < INT16_MIN) return INT16_MIN;
|
||||
return (int16_t)x;
|
||||
}
|
||||
|
||||
inline int32_t celt_sudiv(int32_t n, int32_t d) {
|
||||
assert(d>0); return n/d;
|
||||
}
|
||||
|
||||
inline int16_t sig2word16(int32_t x){
|
||||
x = PSHR(x, 12);
|
||||
x = max(x, -32768);
|
||||
x = min(x, 32767);
|
||||
return (int16_t)(x);
|
||||
}
|
||||
|
||||
inline int32_t ec_tell(){
|
||||
return s_ec.nbits_total-EC_ILOG(s_ec.rng);
|
||||
}
|
||||
|
||||
/* Atan approximation using a 4th order polynomial. Input is in Q15 format and normalized by pi/4. Output is in
|
||||
Q15 format */
|
||||
inline int16_t celt_atan01(int16_t x) {
|
||||
return MULT16_16_P15(
|
||||
x, ADD32(32767, MULT16_16_P15(x, ADD32(-21, MULT16_16_P15(x, ADD32(-11943, MULT16_16_P15(4936, x)))))));
|
||||
}
|
||||
|
||||
/* atan2() approximation valid for positive input values */
|
||||
inline int16_t celt_atan2p(int16_t y, int16_t x) {
|
||||
if(y < x) {
|
||||
int32_t arg;
|
||||
arg = celt_div(SHL32(EXTEND32(y), 15), x);
|
||||
if(arg >= 32767) arg = 32767;
|
||||
return SHR16(celt_atan01((int16_t)(arg)), 1);
|
||||
} else {
|
||||
int32_t arg;
|
||||
arg = celt_div(SHL32(EXTEND32(x), 15), y);
|
||||
if(arg >= 32767) arg = 32767;
|
||||
return 25736 - SHR16(celt_atan01((int16_t)(arg)), 1);
|
||||
}
|
||||
}
|
||||
|
||||
inline int32_t celt_maxabs16(const int16_t *x, int32_t len) {
|
||||
int32_t i;
|
||||
int16_t maxval = 0;
|
||||
int16_t minval = 0;
|
||||
for(i = 0; i < len; i++) {
|
||||
maxval = max(maxval, x[i]);
|
||||
minval = min(minval, x[i]);
|
||||
}
|
||||
return max(EXTEND32(maxval), -EXTEND32(minval));
|
||||
}
|
||||
|
||||
inline int32_t celt_maxabs32(const int32_t *x, int32_t len) {
|
||||
int32_t i;
|
||||
int32_t maxval = 0;
|
||||
int32_t minval = 0;
|
||||
for(i = 0; i < len; i++) {
|
||||
maxval = max(maxval, x[i]);
|
||||
minval = min(minval, x[i]);
|
||||
}
|
||||
return max(maxval, -minval);
|
||||
}
|
||||
|
||||
/** Integer log in base2. Undefined for zero and negative numbers */
|
||||
inline int16_t celt_ilog2(uint32_t x) {
|
||||
assert(x > 0);
|
||||
return EC_ILOG(x) - 1;
|
||||
}
|
||||
|
||||
/** Integer log in base2. Defined for zero, but not for negative numbers */
|
||||
inline int16_t celt_zlog2(uint32_t x) { return x <= 0 ? 0 : celt_ilog2(x); }
|
||||
|
||||
/** Base-2 logarithm approximation (log2(x)). (Q14 input, Q10 output) */
|
||||
inline int16_t celt_log2(int32_t x) {
|
||||
int32_t i;
|
||||
int16_t n, frac, var1;
|
||||
/* -0.41509302963303146, 0.9609890551383969, -0.31836011537636605, 0.15530808010959576, -0.08556153059057618 */
|
||||
static const int16_t C[5] = {-6801 + (1 << 3), 15746, -5217, 2545, -1401};
|
||||
if(x == 0) return -32767;
|
||||
i = celt_ilog2(x);
|
||||
n = VSHR32(x, i - 15) - 32768 - 16384;
|
||||
var1 = MULT16_16_Q15(n, ADD16(C[3], MULT16_16_Q15(n, C[4])));
|
||||
frac = ADD16(C[0], MULT16_16_Q15(n, ADD16(C[1], MULT16_16_Q15(n, ADD16(C[2], var1)))));
|
||||
return SHL16(i - 13, 10) + SHR16(frac, 14 - 10);
|
||||
}
|
||||
|
||||
inline int32_t celt_exp2_frac(int16_t x) {
|
||||
int16_t frac = SHL16(x, 4);
|
||||
int16_t var1 = ADD16(14819, MULT16_16_Q15(10204, frac));
|
||||
return ADD16(16383, MULT16_16_Q15(frac, ADD16(22804, MULT16_16_Q15(frac, var1))));
|
||||
}
|
||||
/** Base-2 exponential approximation (2^x). (Q10 input, Q16 output) */
|
||||
inline int32_t celt_exp2(int16_t x) {
|
||||
int32_t integer;
|
||||
int16_t frac;
|
||||
integer = SHR16(x, 10);
|
||||
if (integer > 14)
|
||||
return 0x7f000000;
|
||||
else if (integer < -15)
|
||||
return 0;
|
||||
frac = celt_exp2_frac(x - SHL16(integer, 10));
|
||||
return VSHR32(EXTEND32(frac), -integer - 2);
|
||||
}
|
||||
|
||||
inline void dual_inner_prod(const int16_t *x, const int16_t *y01, const int16_t *y02, int32_t N, int32_t *xy1,
|
||||
int32_t *xy2) {
|
||||
int32_t i;
|
||||
int32_t xy01 = 0;
|
||||
int32_t xy02 = 0;
|
||||
for(i = 0; i < N; i++) {
|
||||
xy01 = MAC16_16(xy01, x[i], y01[i]);
|
||||
xy02 = MAC16_16(xy02, x[i], y02[i]);
|
||||
}
|
||||
*xy1 = xy01;
|
||||
*xy2 = xy02;
|
||||
}
|
||||
|
||||
inline uint32_t celt_inner_prod(const int16_t *x, const int16_t *y, int32_t N) {
|
||||
int i;
|
||||
uint32_t xy = 0;
|
||||
for (i = 0; i < N; i++) xy = (int32_t)x[i] * (int32_t)y[i] + xy;
|
||||
return xy;
|
||||
}
|
||||
|
||||
inline int32_t get_pulses(int32_t i){
|
||||
return i<8 ? i : (8 + (i&7)) << ((i>>3)-1);
|
||||
}
|
||||
|
||||
inline int32_t bits2pulses(int32_t band, int32_t LM, int32_t bits){
|
||||
int32_t i;
|
||||
int32_t lo, hi;
|
||||
const uint8_t *cache;
|
||||
|
||||
LM++;
|
||||
cache = cache_bits50 + cache_index50[LM * m_CELTMode.nbEBands + band];
|
||||
|
||||
lo = 0;
|
||||
hi = cache[0];
|
||||
bits--;
|
||||
for (i=0;i<LOG_MAX_PSEUDO;i++)
|
||||
{
|
||||
int32_t mid = (lo+hi+1)>>1;
|
||||
/* OPT: Make sure this is implemented with a conditional move */
|
||||
if ((int32_t)cache[mid] >= bits)
|
||||
hi = mid;
|
||||
else
|
||||
lo = mid;
|
||||
}
|
||||
if (bits- (lo == 0 ? -1 : (int32_t)cache[lo]) <= (int32_t)cache[hi]-bits)
|
||||
return lo;
|
||||
else
|
||||
return hi;
|
||||
}
|
||||
|
||||
inline int32_t pulses2bits(int32_t band, int32_t LM, int32_t pulses){
|
||||
const uint8_t *cache;
|
||||
|
||||
LM++;
|
||||
cache = cache_bits50 + cache_index50[LM * m_CELTMode.nbEBands + band];
|
||||
return pulses == 0 ? 0 : cache[pulses]+1;
|
||||
}
|
||||
|
||||
void comb_filter_const(int32_t *y, int32_t *x, int32_t T, int32_t N, int16_t g10, int16_t g11, int16_t g12);
|
||||
void comb_filter(int32_t *y, int32_t *x, int32_t T0, int32_t T1, int32_t N, int16_t g0, int16_t g1, int32_t tapset0,
|
||||
int32_t tapset1);
|
||||
void init_caps(int32_t *cap, int32_t LM, int32_t C);
|
||||
uint32_t celt_lcg_rand(uint32_t seed);
|
||||
int16_t bitexact_cos(int16_t x);
|
||||
int32_t bitexact_log2tan(int32_t isin, int32_t icos);
|
||||
void denormalise_bands(const int16_t *X, int32_t *freq, const int16_t *bandLogE, int32_t end, int32_t M,
|
||||
int32_t silence);
|
||||
void anti_collapse(int16_t *X_, uint8_t *collapse_masks, int32_t LM, int32_t C, int32_t size, const int16_t *logE,
|
||||
const int16_t *prev1logE, const int16_t *prev2logE, const int32_t *pulses, uint32_t seed);
|
||||
void compute_channel_weights(int32_t Ex, int32_t Ey, int16_t w[2]);
|
||||
void stereo_split(int16_t *X, int16_t *Y, int32_t N);
|
||||
void stereo_merge(int16_t *X, int16_t *Y, int16_t mid, int32_t N);
|
||||
void deinterleave_hadamard(int16_t *X, int32_t N0, int32_t stride, int32_t hadamard);
|
||||
void interleave_hadamard(int16_t *X, int32_t N0, int32_t stride, int32_t hadamard);
|
||||
void haar1(int16_t *X, int32_t N0, int32_t stride);
|
||||
int32_t compute_qn(int32_t N, int32_t b, int32_t offset, int32_t pulse_cap, int32_t stereo);
|
||||
void compute_theta(struct split_ctx *sctx, int16_t *X, int16_t *Y, int32_t N, int32_t *b, int32_t B, int32_t __B0,
|
||||
int32_t LM, int32_t stereo, int32_t *fill);
|
||||
uint32_t quant_band_n1(int16_t *X, int16_t *Y, int32_t b, int16_t *lowband_out);
|
||||
uint32_t quant_partition(int16_t *X, int32_t N, int32_t b, int32_t B, int16_t *lowband, int32_t LM, int16_t gain,
|
||||
int32_t fill);
|
||||
uint32_t quant_band(int16_t *X, int32_t N, int32_t b, int32_t B, int16_t *lowband, int32_t LM, int16_t *lowband_out,
|
||||
int16_t gain, int16_t *lowband_scratch, int32_t fill);
|
||||
uint32_t quant_band_stereo(int16_t *X, int16_t *Y, int32_t N, int32_t b, int32_t B, int16_t *lowband, int32_t LM,
|
||||
int16_t *lowband_out, int16_t *lowband_scratch, int32_t fill);
|
||||
void special_hybrid_folding(int16_t *norm, int16_t *norm2, int32_t M, int32_t dual_stereo);
|
||||
void quant_all_bands(int16_t *X_, int16_t *Y_, uint8_t *collapse_masks, int32_t *pulses, int32_t shortBlocks,
|
||||
int32_t spread, int32_t dual_stereo, int32_t intensity, int32_t *tf_res, int32_t total_bits,
|
||||
int32_t balance, int32_t LM, int32_t codedBands);
|
||||
int32_t celt_decoder_get_size(int32_t channels);
|
||||
int32_t celt_decoder_init(int32_t channels);
|
||||
void deemphasis_stereo_simple(int32_t *in[], int16_t *pcm, int32_t N, const int16_t coef0, int32_t *mem);
|
||||
void deemphasis(int32_t *in[], int16_t *pcm, int32_t N);
|
||||
void celt_synthesis(int16_t *X, int32_t *out_syn[], int16_t *oldBandE, int32_t C, int32_t isTransient, int32_t LM,
|
||||
int32_t silence);
|
||||
void tf_decode(int32_t isTransient, int32_t *tf_res, int32_t LM);
|
||||
int32_t celt_decode_with_ec(const uint8_t *inbuf, int32_t len, int16_t *outbuf, int32_t frame_size);
|
||||
int32_t celt_decoder_ctl(int32_t request, ...);
|
||||
int32_t cwrsi(int32_t _n, int32_t _k, uint32_t _i, int32_t *_y);
|
||||
int32_t decode_pulses(int32_t *_y, int32_t _n, int32_t _k);
|
||||
uint32_t ec_tell_frac();
|
||||
int32_t ec_read_byte();
|
||||
int32_t ec_read_byte_from_end();
|
||||
void ec_dec_normalize();
|
||||
void ec_dec_init(uint8_t *_buf, uint32_t _storage);
|
||||
uint32_t ec_decode(uint32_t _ft);
|
||||
uint32_t ec_decode_bin(uint32_t _bits);
|
||||
void ec_dec_update(uint32_t _fl, uint32_t _fh, uint32_t _ft);
|
||||
int32_t ec_dec_bit_logp(uint32_t _logp);
|
||||
int32_t ec_dec_icdf(const uint8_t *_icdf, uint32_t _ftb);
|
||||
uint32_t ec_dec_uint(uint32_t _ft);
|
||||
uint32_t ec_dec_bits(uint32_t _bits);
|
||||
void kf_bfly2(kiss_fft_cpx *Fout, int32_t m, int32_t N);
|
||||
void kf_bfly4(kiss_fft_cpx *Fout, const size_t fstride, const kiss_fft_state *st, int32_t m, int32_t N, int32_t mm);
|
||||
void kf_bfly3(kiss_fft_cpx *Fout, const size_t fstride, const kiss_fft_state *st, int32_t m, int32_t N, int32_t mm);
|
||||
void kf_bfly5(kiss_fft_cpx *Fout, const size_t fstride, const kiss_fft_state *st, int32_t m, int32_t N, int32_t mm);
|
||||
void opus_fft_impl(const kiss_fft_state *st, kiss_fft_cpx *fout);
|
||||
uint32_t ec_laplace_get_freq1(uint32_t fs0, int32_t decay);
|
||||
int32_t ec_laplace_decode(uint32_t fs, int32_t decay);
|
||||
uint32_t isqrt32(uint32_t _val);
|
||||
int16_t celt_rsqrt_norm(int32_t x);
|
||||
int32_t celt_sqrt(int32_t x);
|
||||
int16_t celt_cos_norm(int32_t x);
|
||||
int32_t celt_rcp(int32_t x);
|
||||
void clt_mdct_backward(int32_t *in, int32_t *out, int32_t overlap, int32_t shift, int32_t stride);
|
||||
void exp_rotation1(int16_t *X, int32_t len, int32_t stride, int16_t c, int16_t s);
|
||||
void exp_rotation(int16_t *X, int32_t len, int32_t dir, int32_t stride, int32_t K, int32_t spread);
|
||||
void normalise_residual(int32_t *iy, int16_t *X, int32_t N, int32_t Ryy, int16_t gain);
|
||||
uint32_t extract_collapse_mask(int32_t *iy, int32_t N, int32_t B);
|
||||
uint32_t alg_unquant(int16_t *X, int32_t N, int32_t K, int32_t spread, int32_t B, int16_t gain);
|
||||
void renormalise_vector(int16_t *X, int32_t N, int16_t gain);
|
||||
int32_t interp_bits2pulses(int32_t end, int32_t skip_start, const int32_t *bits1, const int32_t *bits2,
|
||||
const int32_t *thresh, const int32_t *cap, int32_t total, int32_t *_balance,
|
||||
int32_t skip_rsv, int32_t *intensity, int32_t intensity_rsv, int32_t *dual_stereo,
|
||||
int32_t dual_stereo_rsv, int32_t *bits, int32_t *ebits, int32_t *fine_priority, int32_t C,
|
||||
int32_t LM);
|
||||
int32_t clt_compute_allocation(const int32_t *offsets, const int32_t *cap, int32_t alloc_trim, int32_t *intensity,
|
||||
int32_t *dual_stereo, int32_t total, int32_t *balance, int32_t *pulses, int32_t *ebits,
|
||||
int32_t *fine_priority, int32_t C, int32_t LM);
|
||||
void unquant_coarse_energy(int16_t *oldEBands, int32_t intra, int32_t C, int32_t LM);
|
||||
void unquant_fine_energy(int16_t *oldEBands, int32_t *fine_quant, int32_t C);
|
||||
void unquant_energy_finalise(int16_t *oldEBands, int32_t *fine_quant, int32_t *fine_priority, int32_t bits_left,
|
||||
int32_t C);
|
||||
uint32_t celt_pvq_u_row(uint32_t row, uint32_t data);
|
||||
|
||||
bool CELTDecoder_AllocateBuffers(void);
|
||||
void CELTDecoder_FreeBuffers();
|
||||
void CELTDecoder_ClearBuffer(void);
|
||||
|
379
lib/ESP32-audioI2S-3.0.0/src/opus_decoder/opus_decoder.cpp
Normal file
@ -0,0 +1,379 @@
|
||||
/*
|
||||
* opus_decoder.cpp
|
||||
* based on Xiph.Org Foundation celt decoder
|
||||
*
|
||||
* Created on: 26.01.2023
|
||||
* Updated on: 06.02.2023
|
||||
*/
|
||||
//----------------------------------------------------------------------------------------------------------------------
|
||||
// O G G / O P U S I M P L.
|
||||
//----------------------------------------------------------------------------------------------------------------------
|
||||
#include "opus_decoder.h"
|
||||
#include "celt.h"
|
||||
|
||||
// global vars
|
||||
bool s_f_opusSubsequentPage = false;
|
||||
bool s_f_opusParseOgg = false;
|
||||
bool s_f_newSteamTitle = false; // streamTitle
|
||||
bool s_f_opusFramePacket = false;
|
||||
uint8_t s_opusChannels = 0;
|
||||
uint16_t s_opusSamplerate = 0;
|
||||
uint32_t s_opusSegmentLength = 0;
|
||||
char *s_opusChbuf = NULL;
|
||||
int32_t s_opusValidSamples = 0;
|
||||
uint8_t s_opusOldMode = 0;
|
||||
|
||||
uint16_t *s_opusSegmentTable;
|
||||
uint8_t s_opusSegmentTableSize = 0;
|
||||
int16_t s_opusSegmentTableRdPtr = -1;
|
||||
int8_t s_opusError = 0;
|
||||
float s_opusCompressionRatio = 0;
|
||||
|
||||
bool OPUSDecoder_AllocateBuffers(){
|
||||
const uint32_t CELT_SET_END_BAND_REQUEST = 10012;
|
||||
const uint32_t CELT_SET_SIGNALLING_REQUEST = 10016;
|
||||
s_opusChbuf = (char*)malloc(512);
|
||||
if(!CELTDecoder_AllocateBuffers()) {log_e("CELT not init"); return false;}
|
||||
s_opusSegmentTable = (uint16_t*)malloc(256 * sizeof(uint16_t));
|
||||
if(!s_opusSegmentTable) {log_e("CELT not init"); return false;}
|
||||
CELTDecoder_ClearBuffer();
|
||||
OPUSDecoder_ClearBuffers();
|
||||
s_opusError = celt_decoder_init(2); if(s_opusError < 0) {log_e("CELT not init"); return false;}
|
||||
s_opusError = celt_decoder_ctl(CELT_SET_SIGNALLING_REQUEST, 0); if(s_opusError < 0) {log_e("CELT not init"); return false;}
|
||||
s_opusError = celt_decoder_ctl(CELT_SET_END_BAND_REQUEST, 21); if(s_opusError < 0) {log_e("CELT not init"); return false;}
|
||||
OPUSsetDefaults();
|
||||
return true;
|
||||
}
|
||||
void OPUSDecoder_FreeBuffers(){
|
||||
if(s_opusChbuf) {free(s_opusChbuf); s_opusChbuf = NULL;}
|
||||
if(s_opusSegmentTable) {free(s_opusSegmentTable); s_opusSegmentTable = NULL;}
|
||||
CELTDecoder_FreeBuffers();
|
||||
}
|
||||
void OPUSDecoder_ClearBuffers(){
|
||||
if(s_opusChbuf) memset(s_opusChbuf, 0, 512);
|
||||
if(s_opusSegmentTable) memset(s_opusSegmentTable, 0, 256 * sizeof(int16_t));
|
||||
}
|
||||
void OPUSsetDefaults(){
|
||||
s_f_opusSubsequentPage = false;
|
||||
s_f_opusParseOgg = false;
|
||||
s_f_newSteamTitle = false; // streamTitle
|
||||
s_f_opusFramePacket = false;
|
||||
s_opusChannels = 0;
|
||||
s_opusSamplerate = 0;
|
||||
s_opusSegmentLength = 0;
|
||||
s_opusValidSamples = 0;
|
||||
s_opusSegmentTableSize = 0;
|
||||
s_opusOldMode = 0xFF;
|
||||
s_opusSegmentTableRdPtr = -1;
|
||||
|
||||
s_opusError = 0;
|
||||
}
|
||||
|
||||
//----------------------------------------------------------------------------------------------------------------------
|
||||
|
||||
int OPUSDecode(uint8_t *inbuf, int *bytesLeft, short *outbuf){
|
||||
|
||||
if(s_f_opusParseOgg){
|
||||
int ret = OPUSparseOGG(inbuf, bytesLeft);
|
||||
if(ret == ERR_OPUS_NONE) return OPUS_PARSE_OGG_DONE; // ok
|
||||
else return ret; // error
|
||||
}
|
||||
|
||||
if(s_f_opusFramePacket){
|
||||
if(s_opusSegmentTableSize > 0){
|
||||
s_opusSegmentTableRdPtr++;
|
||||
s_opusSegmentTableSize--;
|
||||
int len = s_opusSegmentTable[s_opusSegmentTableRdPtr];
|
||||
*bytesLeft -= len;
|
||||
int32_t ret = parseOpusTOC(inbuf[0]);
|
||||
if(ret < 0) return ret;
|
||||
int frame_size = opus_packet_get_samples_per_frame(inbuf, 48000);
|
||||
len--;
|
||||
inbuf++;
|
||||
|
||||
ec_dec_init((uint8_t *)inbuf, len);
|
||||
ret = celt_decode_with_ec(inbuf, len, (int16_t*)outbuf, frame_size);
|
||||
if(ret < 0) return ret; // celt error
|
||||
s_opusValidSamples = ret;
|
||||
|
||||
if(s_opusSegmentTableSize== 0){
|
||||
s_opusSegmentTableRdPtr = -1; // back to the parking position
|
||||
s_f_opusFramePacket = false;
|
||||
s_f_opusParseOgg = true;
|
||||
}
|
||||
}
|
||||
}
|
||||
return ERR_OPUS_NONE;
|
||||
}
|
||||
//----------------------------------------------------------------------------------------------------------------------
|
||||
|
||||
int32_t opus_packet_get_samples_per_frame(const uint8_t *data, int32_t Fs) {
|
||||
int32_t audiosize;
|
||||
if (data[0] & 0x80) {
|
||||
audiosize = ((data[0] >> 3) & 0x3);
|
||||
audiosize = (Fs << audiosize) / 400;
|
||||
} else if ((data[0] & 0x60) == 0x60) {
|
||||
audiosize = (data[0] & 0x08) ? Fs / 50 : Fs / 100;
|
||||
} else {
|
||||
audiosize = ((data[0] >> 3) & 0x3);
|
||||
if (audiosize == 3)
|
||||
audiosize = Fs * 60 / 1000;
|
||||
else
|
||||
audiosize = (Fs << audiosize) / 100;
|
||||
}
|
||||
return audiosize;
|
||||
}
|
||||
//----------------------------------------------------------------------------------------------------------------------
|
||||
|
||||
uint8_t OPUSGetChannels(){
|
||||
return s_opusChannels;
|
||||
}
|
||||
uint32_t OPUSGetSampRate(){
|
||||
return s_opusSamplerate;
|
||||
}
|
||||
uint8_t OPUSGetBitsPerSample(){
|
||||
return 16;
|
||||
}
|
||||
uint32_t OPUSGetBitRate(){
|
||||
if(s_opusCompressionRatio != 0){
|
||||
return (16 * 2 * 48000) / s_opusCompressionRatio; //bitsPerSample * channel* SampleRate/CompressionRatio
|
||||
}
|
||||
else return 0;
|
||||
}
|
||||
uint16_t OPUSGetOutputSamps(){
|
||||
return s_opusValidSamples; // 1024
|
||||
}
|
||||
char* OPUSgetStreamTitle(){
|
||||
if(s_f_newSteamTitle){
|
||||
s_f_newSteamTitle = false;
|
||||
return s_opusChbuf;
|
||||
}
|
||||
return NULL;
|
||||
}
|
||||
//----------------------------------------------------------------------------------------------------------------------
|
||||
int parseOpusTOC(uint8_t TOC_Byte){ // https://www.rfc-editor.org/rfc/rfc6716 page 16 ff
|
||||
|
||||
uint8_t mode = 0;
|
||||
uint8_t configNr = 0;
|
||||
uint8_t s = 0;
|
||||
uint8_t c = 0; (void)c;
|
||||
|
||||
configNr = (TOC_Byte & 0b11111000) >> 3;
|
||||
s = (TOC_Byte & 0b00000100) >> 2;
|
||||
c = (TOC_Byte & 0b00000011);
|
||||
if(TOC_Byte & 0x80) mode = 2; else mode = 1;
|
||||
|
||||
if(s_opusOldMode != mode) {
|
||||
s_opusOldMode = mode;
|
||||
// if(mode == 2) log_i("opus mode is MODE_CELT_ONLY");
|
||||
}
|
||||
|
||||
/* Configuration Mode Bandwidth FrameSizes Audio Bandwidth Sample Rate (Effective)
|
||||
configNr 16 ... 19 CELT NB (narrowband) 2.5, 5, 10, 20ms 4 kHz 8 kHz
|
||||
configNr 20 ... 23 CELT WB (wideband) 2.5, 5, 10, 20ms 8 kHz 16 kHz
|
||||
configNr 24 ... 27 CELT SWB(super wideband) 2.5, 5, 10, 20ms 12 kHz 24 kHz
|
||||
configNr 28 ... 31 CELT FB (fullband) 2.5, 5, 10, 20ms 20 kHz (*) 48 kHz <-------
|
||||
|
||||
(*) Although the sampling theorem allows a bandwidth as large as half the sampling rate, Opus never codes
|
||||
audio above 20 kHz, as that is the generally accepted upper limit of human hearing.
|
||||
|
||||
s = 0: mono 1: stereo
|
||||
|
||||
c = 0: 1 frame in the packet
|
||||
c = 1: 2 frames in the packet, each with equal compressed size
|
||||
c = 2: 2 frames in the packet, with different compressed sizes
|
||||
c = 3: an arbitrary number of frames in the packet
|
||||
*/
|
||||
|
||||
// log_i("configNr %i, s %i, c %i", configNr, s, c);
|
||||
|
||||
if(configNr < 12) return ERR_OPUS_SILK_MODE_UNSUPPORTED;
|
||||
if(configNr < 16) return ERR_OPUS_HYBRID_MODE_UNSUPPORTED;
|
||||
|
||||
return s;
|
||||
}
|
||||
//----------------------------------------------------------------------------------------------------------------------
|
||||
int parseOpusComment(uint8_t *inbuf, int nBytes){ // reference https://exiftool.org/TagNames/Vorbis.html#Comments
|
||||
// reference https://www.rfc-editor.org/rfc/rfc7845#section-5
|
||||
int idx = OPUS_specialIndexOf(inbuf, "OpusTags", 10);
|
||||
if(idx != 0) return 0; // is not OpusTags
|
||||
char* artist = NULL;
|
||||
char* title = NULL;
|
||||
|
||||
uint16_t pos = 8;
|
||||
uint32_t vendorLength = *(inbuf + 11) << 24; // lengt of vendor string, e.g. Lavf58.65.101
|
||||
vendorLength += *(inbuf + 10) << 16;
|
||||
vendorLength += *(inbuf + 9) << 8;
|
||||
vendorLength += *(inbuf + 8);
|
||||
pos += vendorLength + 4;
|
||||
uint32_t commentListLength = *(inbuf + 3 + pos) << 24; // nr. of comment entries
|
||||
commentListLength += *(inbuf + 2 + pos) << 16;
|
||||
commentListLength += *(inbuf + 1 + pos) << 8;
|
||||
commentListLength += *(inbuf + 0 + pos);
|
||||
pos += 4;
|
||||
for(int i = 0; i < commentListLength; i++){
|
||||
uint32_t commentStringLen = *(inbuf + 3 + pos) << 24;
|
||||
commentStringLen += *(inbuf + 2 + pos) << 16;
|
||||
commentStringLen += *(inbuf + 1 + pos) << 8;
|
||||
commentStringLen += *(inbuf + 0 + pos);
|
||||
pos += 4;
|
||||
idx = OPUS_specialIndexOf(inbuf + pos, "artist=", 10);
|
||||
if(idx == 0){ artist = strndup((const char*)(inbuf + pos + 7), commentStringLen - 7);
|
||||
}
|
||||
idx = OPUS_specialIndexOf(inbuf + pos, "title=", 10);
|
||||
if(idx == 0){ title = strndup((const char*)(inbuf + pos + 6), commentStringLen - 6);
|
||||
}
|
||||
pos += commentStringLen;
|
||||
}
|
||||
if(artist && title){
|
||||
strcpy(s_opusChbuf, artist);
|
||||
strcat(s_opusChbuf, " - ");
|
||||
strcat(s_opusChbuf, title);
|
||||
s_f_newSteamTitle = true;
|
||||
}
|
||||
else if(artist){
|
||||
strcpy(s_opusChbuf, artist);
|
||||
s_f_newSteamTitle = true;
|
||||
}
|
||||
else if(title){
|
||||
strcpy(s_opusChbuf, title);
|
||||
s_f_newSteamTitle = true;
|
||||
}
|
||||
if(artist){free(artist); artist = NULL;}
|
||||
if(title) {free(title); title = NULL;}
|
||||
|
||||
return 1;
|
||||
}
|
||||
//----------------------------------------------------------------------------------------------------------------------
|
||||
int parseOpusHead(uint8_t *inbuf, int nBytes){ // reference https://wiki.xiph.org/OggOpus
|
||||
|
||||
int idx = OPUS_specialIndexOf(inbuf, "OpusHead", 10);
|
||||
if(idx != 0) return 0; //is not OpusHead
|
||||
uint8_t version = *(inbuf + 8); (void) version;
|
||||
uint8_t channelCount = *(inbuf + 9); // nr of channels
|
||||
uint16_t preSkip = *(inbuf + 11) << 8;
|
||||
preSkip += *(inbuf + 10);
|
||||
uint32_t sampleRate = *(inbuf + 15) << 24; // informational only
|
||||
sampleRate += *(inbuf + 14) << 16;
|
||||
sampleRate += *(inbuf + 13) << 8;
|
||||
sampleRate += *(inbuf + 12);
|
||||
uint16_t outputGain = *(inbuf + 17) << 8; // Q7.8 in dB
|
||||
outputGain += *(inbuf + 16);
|
||||
uint8_t channelMap = *(inbuf + 18);
|
||||
|
||||
if(channelCount == 0 or channelCount >2) return ERR_OPUS_CHANNELS_OUT_OF_RANGE;
|
||||
s_opusChannels = channelCount;
|
||||
if(sampleRate != 48000) return ERR_OPUS_INVALID_SAMPLERATE;
|
||||
s_opusSamplerate = sampleRate;
|
||||
if(channelMap > 1) return ERR_OPUS_EXTRA_CHANNELS_UNSUPPORTED;
|
||||
|
||||
(void)outputGain;
|
||||
|
||||
return 1;
|
||||
}
|
||||
|
||||
//----------------------------------------------------------------------------------------------------------------------
|
||||
int OPUSparseOGG(uint8_t *inbuf, int *bytesLeft){ // reference https://www.xiph.org/ogg/doc/rfc3533.txt
|
||||
|
||||
s_f_opusParseOgg = false;
|
||||
int ret = 0;
|
||||
int idx = OPUS_specialIndexOf(inbuf, "OggS", 6);
|
||||
if(idx != 0) return ERR_OPUS_DECODER_ASYNC;
|
||||
|
||||
int16_t segmentTableWrPtr = -1;
|
||||
|
||||
uint8_t version = *(inbuf + 4); (void) version;
|
||||
uint8_t headerType = *(inbuf + 5); (void) headerType;
|
||||
uint64_t granulePosition = (uint64_t)*(inbuf + 13) << 56; // granule_position: an 8 Byte field containing -
|
||||
granulePosition += (uint64_t)*(inbuf + 12) << 48; // position information. For an audio stream, it MAY
|
||||
granulePosition += (uint64_t)*(inbuf + 11) << 40; // contain the total number of PCM samples encoded
|
||||
granulePosition += (uint64_t)*(inbuf + 10) << 32; // after including all frames finished on this page.
|
||||
granulePosition += *(inbuf + 9) << 24; // This is a hint for the decoder and gives it some timing
|
||||
granulePosition += *(inbuf + 8) << 16; // and position information. A special value of -1 (in two's
|
||||
granulePosition += *(inbuf + 7) << 8; // complement) indicates that no packets finish on this page.
|
||||
granulePosition += *(inbuf + 6); (void) granulePosition;
|
||||
uint32_t bitstreamSerialNr = *(inbuf + 17) << 24; // bitstream_serial_number: a 4 Byte field containing the
|
||||
bitstreamSerialNr += *(inbuf + 16) << 16; // unique serial number by which the logical bitstream
|
||||
bitstreamSerialNr += *(inbuf + 15) << 8; // is identified.
|
||||
bitstreamSerialNr += *(inbuf + 14); (void) bitstreamSerialNr;
|
||||
uint32_t pageSequenceNr = *(inbuf + 21) << 24; // page_sequence_number: a 4 Byte field containing the sequence
|
||||
pageSequenceNr += *(inbuf + 20) << 16; // number of the page so the decoder can identify page loss
|
||||
pageSequenceNr += *(inbuf + 19) << 8; // This sequence number is increasing on each logical bitstream
|
||||
pageSequenceNr += *(inbuf + 18); (void) pageSequenceNr;
|
||||
uint32_t CRCchecksum = *(inbuf + 25) << 24;
|
||||
CRCchecksum += *(inbuf + 24) << 16;
|
||||
CRCchecksum += *(inbuf + 23) << 8;
|
||||
CRCchecksum += *(inbuf + 22); (void) CRCchecksum;
|
||||
uint8_t pageSegments = *(inbuf + 26); // giving the number of segment entries
|
||||
|
||||
// read the segment table (contains pageSegments bytes), 1...251: Length of the frame in bytes,
|
||||
// 255: A second byte is needed. The total length is first_byte + second byte
|
||||
s_opusSegmentLength = 0;
|
||||
segmentTableWrPtr = -1;
|
||||
|
||||
for(int i = 0; i < pageSegments; i++){
|
||||
int n = *(inbuf + 27 + i);
|
||||
while(*(inbuf + 27 + i) == 255){
|
||||
i++;
|
||||
n+= *(inbuf + 27 + i);
|
||||
}
|
||||
segmentTableWrPtr++;
|
||||
s_opusSegmentTable[segmentTableWrPtr] = n;
|
||||
s_opusSegmentLength += n;
|
||||
}
|
||||
s_opusSegmentTableSize = segmentTableWrPtr + 1;
|
||||
s_opusCompressionRatio = (float)(960 * 2 * pageSegments)/s_opusSegmentLength; // const 960 validBytes out
|
||||
|
||||
bool continuedPage = headerType & 0x01; // set: page contains data of a packet continued from the previous page
|
||||
bool firstPage = headerType & 0x02; // set: this is the first page of a logical bitstream (bos)
|
||||
bool lastPage = headerType & 0x04; // set: this is the last page of a logical bitstream (eos)
|
||||
|
||||
uint16_t headerSize = pageSegments + 27;
|
||||
(void)continuedPage; (void)lastPage;
|
||||
*bytesLeft -= headerSize;
|
||||
|
||||
if(firstPage || s_f_opusSubsequentPage){ // OpusHead or OggComment may follows
|
||||
ret = parseOpusHead(inbuf + headerSize, s_opusSegmentTable[0]);
|
||||
if(ret == 1) *bytesLeft -= s_opusSegmentTable[0];
|
||||
if(ret < 0){ *bytesLeft -= s_opusSegmentTable[0]; return ret;}
|
||||
ret = parseOpusComment(inbuf + headerSize, s_opusSegmentTable[0]);
|
||||
if(ret == 1) *bytesLeft -= s_opusSegmentTable[0];
|
||||
if(ret < 0){ *bytesLeft -= s_opusSegmentTable[0]; return ret;}
|
||||
s_f_opusParseOgg = true;// goto next page
|
||||
}
|
||||
|
||||
s_f_opusFramePacket = true;
|
||||
if(firstPage) s_f_opusSubsequentPage = true; else s_f_opusSubsequentPage = false;
|
||||
|
||||
return ERR_OPUS_NONE; // no error
|
||||
}
|
||||
|
||||
//----------------------------------------------------------------------------------------------------------------------
|
||||
int OPUSFindSyncWord(unsigned char *buf, int nBytes){
|
||||
// assume we have a ogg wrapper
|
||||
int idx = OPUS_specialIndexOf(buf, "OggS", nBytes);
|
||||
if(idx >= 0){ // Magic Word found
|
||||
// log_i("OggS found at %i", idx);
|
||||
s_f_opusParseOgg = true;
|
||||
return idx;
|
||||
}
|
||||
log_i("find sync");
|
||||
s_f_opusParseOgg = false;
|
||||
return ERR_OPUS_OGG_SYNC_NOT_FOUND;
|
||||
}
|
||||
//----------------------------------------------------------------------------------------------------------------------
|
||||
int OPUS_specialIndexOf(uint8_t* base, const char* str, int baselen, bool exact){
|
||||
int result; // seek for str in buffer or in header up to baselen, not nullterninated
|
||||
if (strlen(str) > baselen) return -1; // if exact == true seekstr in buffer must have "\0" at the end
|
||||
for (int i = 0; i < baselen - strlen(str); i++){
|
||||
result = i;
|
||||
for (int j = 0; j < strlen(str) + exact; j++){
|
||||
if (*(base + i + j) != *(str + j)){
|
||||
result = -1;
|
||||
break;
|
||||
}
|
||||
}
|
||||
if (result >= 0) break;
|
||||
}
|
||||
return result;
|
||||
}
|
47
lib/ESP32-audioI2S-3.0.0/src/opus_decoder/opus_decoder.h
Normal file
@ -0,0 +1,47 @@
|
||||
// based on Xiph.Org Foundation celt decoder
|
||||
#pragma once
|
||||
//#pragma GCC optimize ("O3")
|
||||
//#pragma GCC diagnostic ignored "-Wnarrowing"
|
||||
|
||||
#include "Arduino.h"
|
||||
|
||||
enum : int8_t {OPUS_PARSE_OGG_DONE = 100,
|
||||
ERR_OPUS_NONE = 0,
|
||||
ERR_OPUS_CHANNELS_OUT_OF_RANGE = -1,
|
||||
ERR_OPUS_INVALID_SAMPLERATE = -2,
|
||||
ERR_OPUS_EXTRA_CHANNELS_UNSUPPORTED = -3,
|
||||
ERR_OPUS_DECODER_ASYNC = -4,
|
||||
ERR_OPUS_SILK_MODE_UNSUPPORTED = -5,
|
||||
ERR_OPUS_HYBRID_MODE_UNSUPPORTED = -6,
|
||||
ERR_OPUS_OGG_SYNC_NOT_FOUND = - 7,
|
||||
ERR_OPUS_CELT_BAD_ARG = -18,
|
||||
ERR_OPUS_CELT_INTERNAL_ERROR = -19,
|
||||
ERR_OPUS_CELT_UNIMPLEMENTED = -20,
|
||||
ERR_OPUS_CELT_ALLOC_FAIL = -21,
|
||||
ERR_OPUS_CELT_UNKNOWN_REQUEST = -22,
|
||||
ERR_OPUS_CELT_GET_MODE_REQUEST = - 23,
|
||||
ERR_OPUS_CELT_CLEAR_REQUEST = -24,
|
||||
ERR_OPUS_CELT_SET_CHANNELS = -25,
|
||||
ERR_OPUS_CELT_END_BAND = -26,
|
||||
ERR_CELT_OPUS_INTERNAL_ERROR = -27};
|
||||
|
||||
bool OPUSDecoder_AllocateBuffers();
|
||||
void OPUSDecoder_FreeBuffers();
|
||||
void OPUSDecoder_ClearBuffers();
|
||||
void OPUSsetDefaults();
|
||||
int OPUSDecode(uint8_t *inbuf, int *bytesLeft, short *outbuf);
|
||||
uint8_t OPUSGetChannels();
|
||||
uint32_t OPUSGetSampRate();
|
||||
uint8_t OPUSGetBitsPerSample();
|
||||
uint32_t OPUSGetBitRate();
|
||||
uint16_t OPUSGetOutputSamps();
|
||||
char *OPUSgetStreamTitle();
|
||||
int OPUSFindSyncWord(unsigned char *buf, int nBytes);
|
||||
int OPUSparseOGG(uint8_t *inbuf, int *bytesLeft);
|
||||
int parseOpusHead(uint8_t *inbuf, int nBytes);
|
||||
int parseOpusComment(uint8_t *inbuf, int nBytes);
|
||||
int parseOpusTOC(uint8_t TOC_Byte);
|
||||
int32_t opus_packet_get_samples_per_frame(const uint8_t *data, int32_t Fs);
|
||||
|
||||
// some helper functions
|
||||
int OPUS_specialIndexOf(uint8_t* base, const char* str, int baselen, bool exact = false);
|
1
lib/PCF8575 library/.gitattributes
vendored
Normal file
@ -0,0 +1 @@
|
||||
resources export-ignore
|
1
lib/PCF8575 library/.piopm
Normal file
@ -0,0 +1 @@
|
||||
{"type": "library", "name": "PCF8575 library", "version": "1.1.0", "spec": {"owner": "xreef", "id": 11372, "name": "PCF8575 library", "requirements": null, "uri": null}}
|
11
lib/PCF8575 library/.project
Normal file
@ -0,0 +1,11 @@
|
||||
<?xml version="1.0" encoding="UTF-8"?>
|
||||
<projectDescription>
|
||||
<name>PCF8575_library</name>
|
||||
<comment></comment>
|
||||
<projects>
|
||||
</projects>
|
||||
<buildSpec>
|
||||
</buildSpec>
|
||||
<natures>
|
||||
</natures>
|
||||
</projectDescription>
|
21
lib/PCF8575 library/LICENSE
Normal file
@ -0,0 +1,21 @@
|
||||
MIT License
|
||||
|
||||
Copyright (c) 2017 Renzo Mischianti
|
||||
|
||||
Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
of this software and associated documentation files (the "Software"), to deal
|
||||
in the Software without restriction, including without limitation the rights
|
||||
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
copies of the Software, and to permit persons to whom the Software is
|
||||
furnished to do so, subject to the following conditions:
|
||||
|
||||
The above copyright notice and this permission notice shall be included in all
|
||||
copies or substantial portions of the Software.
|
||||
|
||||
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
SOFTWARE.
|
24
lib/PCF8575 library/LICENSE.md
Normal file
@ -0,0 +1,24 @@
|
||||
The MIT License (MIT)
|
||||
|
||||
Copyright (c) 2017 Renzo Mischianti www.mischianti.org All right reserved.
|
||||
|
||||
You may copy, alter and reuse this code in any way you like, but please leave
|
||||
reference to www.mischianti.org in your comments if you redistribute this code.
|
||||
|
||||
Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
of this software and associated documentation files (the "Software"), to deal
|
||||
in the Software without restriction, including without limitation the rights
|
||||
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
copies of the Software, and to permit persons to whom the Software is
|
||||
furnished to do so, subject to the following conditions:
|
||||
|
||||
The above copyright notice and this permission notice shall be included in
|
||||
all copies or substantial portions of the Software.
|
||||
|
||||
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
|
||||
THE SOFTWARE.
|
486
lib/PCF8575 library/PCF8575.cpp
Normal file
@ -0,0 +1,486 @@
|
||||
/*
|
||||
* PCF8575 GPIO Port Expand
|
||||
* https://www.mischianti.org/
|
||||
*
|
||||
* The MIT License (MIT)
|
||||
*
|
||||
* Copyright (c) 2019 Renzo Mischianti www.mischianti.org All right reserved.
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
* of this software and associated documentation files (the "Software"), to deal
|
||||
* in the Software without restriction, including without limitation the rights
|
||||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
* copies of the Software, and to permit persons to whom the Software is
|
||||
* furnished to do so, subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in
|
||||
* all copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
|
||||
* THE SOFTWARE.
|
||||
*/
|
||||
|
||||
#include "PCF8575.h"
|
||||
#include "Wire.h"
|
||||
|
||||
/**
|
||||
* Constructor
|
||||
* @param address: i2c address
|
||||
*/
|
||||
PCF8575::PCF8575(uint8_t address){
|
||||
_wire = &Wire;
|
||||
|
||||
_address = address;
|
||||
};
|
||||
|
||||
/**
|
||||
* Construcor
|
||||
* @param address: i2c address
|
||||
* @param interruptPin: pin to set interrupt
|
||||
* @param interruptFunction: function to call when interrupt raised
|
||||
*/
|
||||
PCF8575::PCF8575(uint8_t address, uint8_t interruptPin, void (*interruptFunction)() ){
|
||||
_wire = &Wire;
|
||||
|
||||
_address = address;
|
||||
_interruptPin = interruptPin;
|
||||
_interruptFunction = interruptFunction;
|
||||
_usingInterrupt = true;
|
||||
};
|
||||
|
||||
#if !defined(__AVR) && !defined(ARDUINO_ARCH_SAMD) && !defined(TEENSYDUINO)
|
||||
/**
|
||||
* Constructor
|
||||
* @param address: i2c address
|
||||
* @param sda: sda pin
|
||||
* @param scl: scl pin
|
||||
*/
|
||||
PCF8575::PCF8575(uint8_t address, int sda, int scl){
|
||||
_wire = &Wire;
|
||||
|
||||
_address = address;
|
||||
_sda = sda;
|
||||
_scl = scl;
|
||||
};
|
||||
|
||||
/**
|
||||
* Constructor
|
||||
* @param address: i2c address
|
||||
* @param sda: sda pin
|
||||
* @param scl: scl pin
|
||||
* @param interruptPin: pin to set interrupt
|
||||
* @param interruptFunction: function to call when interrupt raised
|
||||
*/
|
||||
PCF8575::PCF8575(uint8_t address, int sda, int scl, uint8_t interruptPin, void (*interruptFunction)() ){
|
||||
_wire = &Wire;
|
||||
|
||||
_address = address;
|
||||
_sda = sda;
|
||||
_scl = scl;
|
||||
|
||||
_interruptPin = interruptPin;
|
||||
_interruptFunction = interruptFunction;
|
||||
|
||||
_usingInterrupt = true;
|
||||
};
|
||||
#endif
|
||||
|
||||
#if defined(ESP32) || defined(ARDUINO_ARCH_SAMD) || defined(ARDUINO_ARCH_RP2040) || defined(ARDUINO_ARCH_STM32)
|
||||
/**
|
||||
* Constructor
|
||||
* @param address: i2c address
|
||||
*/
|
||||
PCF8575::PCF8575(TwoWire *pWire, uint8_t address){
|
||||
_wire = pWire;
|
||||
|
||||
_address = address;
|
||||
};
|
||||
|
||||
/**
|
||||
* Construcor
|
||||
* @param address: i2c address
|
||||
* @param interruptPin: pin to set interrupt
|
||||
* @param interruptFunction: function to call when interrupt raised
|
||||
*/
|
||||
PCF8575::PCF8575(TwoWire *pWire, uint8_t address, uint8_t interruptPin, void (*interruptFunction)() ){
|
||||
_wire = pWire;
|
||||
|
||||
_address = address;
|
||||
_interruptPin = interruptPin;
|
||||
_interruptFunction = interruptFunction;
|
||||
_usingInterrupt = true;
|
||||
};
|
||||
#endif
|
||||
#if defined(ESP32)
|
||||
|
||||
/**
|
||||
* Constructor
|
||||
* @param address: i2c address
|
||||
* @param sda: sda pin
|
||||
* @param scl: scl pin
|
||||
*/
|
||||
PCF8575::PCF8575(TwoWire *pWire, uint8_t address, int sda, int scl){
|
||||
_wire = pWire;
|
||||
|
||||
_address = address;
|
||||
_sda = sda;
|
||||
_scl = scl;
|
||||
};
|
||||
|
||||
/**
|
||||
* Constructor
|
||||
* @param address: i2c address
|
||||
* @param sda: sda pin
|
||||
* @param scl: scl pin
|
||||
* @param interruptPin: pin to set interrupt
|
||||
* @param interruptFunction: function to call when interrupt raised
|
||||
*/
|
||||
PCF8575::PCF8575(TwoWire *pWire, uint8_t address, int sda, int scl, uint8_t interruptPin, void (*interruptFunction)() ){
|
||||
_wire = pWire;
|
||||
|
||||
_address = address;
|
||||
_sda = sda;
|
||||
_scl = scl;
|
||||
|
||||
_interruptPin = interruptPin;
|
||||
_interruptFunction = interruptFunction;
|
||||
|
||||
_usingInterrupt = true;
|
||||
};
|
||||
#endif
|
||||
|
||||
/**
|
||||
* wake up i2c controller
|
||||
*/
|
||||
void PCF8575::begin(){
|
||||
#if !defined(__AVR) && !defined(ARDUINO_ARCH_SAMD) && !defined(TEENSYDUINO)
|
||||
DEBUG_PRINT(F("begin(sda, scl) -> "));DEBUG_PRINT(_sda);DEBUG_PRINT(F(" "));DEBUG_PRINTLN(_scl);
|
||||
// _wire->begin(_sda, _scl);
|
||||
#ifdef ARDUINO_ARCH_STM32
|
||||
_wire->begin((uint32_t)_sda, (uint32_t)_scl);
|
||||
#elif defined(ARDUINO_ARCH_RP2040)
|
||||
_wire->setSCL(_scl);
|
||||
_wire->setSDA(_sda);
|
||||
_wire->begin();
|
||||
#else
|
||||
_wire->begin((int)_sda, (int)_scl);
|
||||
#endif
|
||||
#else
|
||||
// Default pin for AVR some problem on software emulation
|
||||
// #define SCL_PIN _scl
|
||||
// #define SDA_PIN _sda
|
||||
_wire->begin();
|
||||
#endif
|
||||
|
||||
// Serial.println( writeMode, BIN);
|
||||
// Serial.println( readMode, BIN);
|
||||
|
||||
// Check if there are pins to set low
|
||||
if (writeMode>0 || readMode>0){
|
||||
DEBUG_PRINTLN("Set write mode");
|
||||
_wire->beginTransmission(_address);
|
||||
DEBUG_PRINT(" ");
|
||||
DEBUG_PRINT("usedPin pin ");
|
||||
|
||||
|
||||
uint16_t usedPin = writeMode | readMode;
|
||||
DEBUG_PRINTLN( ~usedPin, BIN);
|
||||
// Serial.println( ~usedPin, BIN);
|
||||
|
||||
_wire->write((uint8_t) ~usedPin);
|
||||
_wire->write((uint8_t) (~(usedPin >> 8)));
|
||||
|
||||
DEBUG_PRINTLN("Start end trasmission if stop here check pullup resistor.");
|
||||
_wire->endTransmission();
|
||||
}
|
||||
|
||||
// If using interrupt set interrupt value to pin
|
||||
if (_usingInterrupt){
|
||||
DEBUG_PRINTLN("Using interrupt pin (not all pin is interrupted)");
|
||||
::pinMode(_interruptPin, INPUT_PULLUP);
|
||||
attachInterrupt(digitalPinToInterrupt(_interruptPin), (*_interruptFunction), FALLING );
|
||||
}
|
||||
|
||||
// inizialize last read
|
||||
lastReadMillis = millis();
|
||||
}
|
||||
|
||||
/**
|
||||
* Set if fin is OUTPUT or INPUT
|
||||
* @param pin: pin to set
|
||||
* @param mode: mode, supported only INPUT or OUTPUT (to semplify)
|
||||
*/
|
||||
void PCF8575::pinMode(uint8_t pin, uint8_t mode){
|
||||
DEBUG_PRINT("Set pin ");
|
||||
DEBUG_PRINT(pin);
|
||||
DEBUG_PRINT(" as ");
|
||||
DEBUG_PRINTLN(mode);
|
||||
|
||||
if (mode == OUTPUT){
|
||||
writeMode = writeMode | bit(pin);
|
||||
readMode = readMode & ~bit(pin);
|
||||
// DEBUG_PRINT("writeMode: ");
|
||||
// DEBUG_PRINT(writeMode, BIN);
|
||||
// DEBUG_PRINT("readMode: ");
|
||||
// DEBUG_PRINTLN(readMode, BIN);
|
||||
|
||||
}else if (mode == INPUT){
|
||||
writeMode = writeMode & ~bit(pin);
|
||||
readMode = readMode | bit(pin);
|
||||
// DEBUG_PRINT("writeMode: ");
|
||||
// DEBUG_PRINT(writeMode, BIN);
|
||||
// DEBUG_PRINT("readMode: ");
|
||||
// DEBUG_PRINTLN(readMode, BIN);
|
||||
}
|
||||
else{
|
||||
DEBUG_PRINTLN("Mode non supported by PCF8575")
|
||||
}
|
||||
DEBUG_PRINT("Write mode: ");
|
||||
DEBUG_PRINTLN(writeMode, BIN);
|
||||
|
||||
};
|
||||
|
||||
/**
|
||||
* Read value from i2c and bufferize it
|
||||
* @param force
|
||||
*/
|
||||
void PCF8575::readBuffer(bool force){
|
||||
if (millis() > PCF8575::lastReadMillis+READ_ELAPSED_TIME || _usingInterrupt || force){
|
||||
_wire->requestFrom(_address,(uint8_t)2);// Begin transmission to PCF8575 with the buttons
|
||||
lastReadMillis = millis();
|
||||
if(_wire->available()) // If uint16_ts are available to be recieved
|
||||
{
|
||||
uint16_t iInput = _wire->read();// Read a uint16_t
|
||||
iInput |= _wire->read() << 8;// Read a uint16_t
|
||||
if ((iInput & readMode)>0){
|
||||
byteBuffered = byteBuffered | (uint16_t)iInput;
|
||||
}
|
||||
}
|
||||
}
|
||||
}
|
||||
|
||||
#ifndef PCF8575_LOW_MEMORY
|
||||
/**
|
||||
* Read value of all INPUT pin
|
||||
* Debounce read more fast than 10millis, non managed for interrupt mode
|
||||
* @return
|
||||
*/
|
||||
PCF8575::DigitalInput PCF8575::digitalReadAll(void){
|
||||
DEBUG_PRINTLN("Read from buffer");
|
||||
_wire->requestFrom(_address,(uint8_t)2);// Begin transmission to PCF8575 with the buttons
|
||||
lastReadMillis = millis();
|
||||
if(_wire->available()) // If uint16_ts are available to be recieved
|
||||
{
|
||||
DEBUG_PRINTLN("Data ready");
|
||||
uint16_t iInput = _wire->read();// Read a uint16_t
|
||||
iInput |= _wire->read() << 8;// Read a uint16_t
|
||||
|
||||
if ((iInput & readMode)>0){
|
||||
DEBUG_PRINT("Input ");
|
||||
DEBUG_PRINTLN((uint16_t)iInput, BIN);
|
||||
|
||||
byteBuffered = byteBuffered | (uint16_t)iInput;
|
||||
DEBUG_PRINT("byteBuffered ");
|
||||
DEBUG_PRINTLN(byteBuffered, BIN);
|
||||
}
|
||||
}
|
||||
|
||||
DEBUG_PRINT("Buffer value ");
|
||||
DEBUG_PRINTLN(byteBuffered, BIN);
|
||||
#ifdef NOT_SEQUENTIAL_PINOUT
|
||||
if ((bit(0) & readMode)>0) digitalInput.p00 = ((byteBuffered & bit(0))>0)?HIGH:LOW;
|
||||
if ((bit(1) & readMode)>0) digitalInput.p01 = ((byteBuffered & bit(1))>0)?HIGH:LOW;
|
||||
if ((bit(2) & readMode)>0) digitalInput.p02 = ((byteBuffered & bit(2))>0)?HIGH:LOW;
|
||||
if ((bit(3) & readMode)>0) digitalInput.p03 = ((byteBuffered & bit(3))>0)?HIGH:LOW;
|
||||
if ((bit(4) & readMode)>0) digitalInput.p04 = ((byteBuffered & bit(4))>0)?HIGH:LOW;
|
||||
if ((bit(5) & readMode)>0) digitalInput.p05 = ((byteBuffered & bit(5))>0)?HIGH:LOW;
|
||||
if ((bit(6) & readMode)>0) digitalInput.p06 = ((byteBuffered & bit(6))>0)?HIGH:LOW;
|
||||
if ((bit(7) & readMode)>0) digitalInput.p07 = ((byteBuffered & bit(7))>0)?HIGH:LOW;
|
||||
if ((bit(8) & readMode)>0) digitalInput.p10 = ((byteBuffered & bit(8))>0)?HIGH:LOW;
|
||||
if ((bit(9) & readMode)>0) digitalInput.p11 = ((byteBuffered & bit(9))>0)?HIGH:LOW;
|
||||
if ((bit(10) & readMode)>0) digitalInput.p12 = ((byteBuffered & bit(10))>0)?HIGH:LOW;
|
||||
if ((bit(11) & readMode)>0) digitalInput.p13 = ((byteBuffered & bit(11))>0)?HIGH:LOW;
|
||||
if ((bit(12) & readMode)>0) digitalInput.p14 = ((byteBuffered & bit(12))>0)?HIGH:LOW;
|
||||
if ((bit(13) & readMode)>0) digitalInput.p15 = ((byteBuffered & bit(13))>0)?HIGH:LOW;
|
||||
if ((bit(14) & readMode)>0) digitalInput.p16 = ((byteBuffered & bit(14))>0)?HIGH:LOW;
|
||||
if ((bit(15) & readMode)>0) digitalInput.p17 = ((byteBuffered & bit(15))>0)?HIGH:LOW;
|
||||
#else
|
||||
if ((bit(0) & readMode)>0) digitalInput.p0 = ((byteBuffered & bit(0))>0)?HIGH:LOW;
|
||||
if ((bit(1) & readMode)>0) digitalInput.p1 = ((byteBuffered & bit(1))>0)?HIGH:LOW;
|
||||
if ((bit(2) & readMode)>0) digitalInput.p2 = ((byteBuffered & bit(2))>0)?HIGH:LOW;
|
||||
if ((bit(3) & readMode)>0) digitalInput.p3 = ((byteBuffered & bit(3))>0)?HIGH:LOW;
|
||||
if ((bit(4) & readMode)>0) digitalInput.p4 = ((byteBuffered & bit(4))>0)?HIGH:LOW;
|
||||
if ((bit(5) & readMode)>0) digitalInput.p5 = ((byteBuffered & bit(5))>0)?HIGH:LOW;
|
||||
if ((bit(6) & readMode)>0) digitalInput.p6 = ((byteBuffered & bit(6))>0)?HIGH:LOW;
|
||||
if ((bit(7) & readMode)>0) digitalInput.p7 = ((byteBuffered & bit(7))>0)?HIGH:LOW;
|
||||
if ((bit(8) & readMode)>0) digitalInput.p8 = ((byteBuffered & bit(8))>0)?HIGH:LOW;
|
||||
if ((bit(9) & readMode)>0) digitalInput.p9 = ((byteBuffered & bit(9))>0)?HIGH:LOW;
|
||||
if ((bit(10) & readMode)>0) digitalInput.p10 = ((byteBuffered & bit(10))>0)?HIGH:LOW;
|
||||
if ((bit(11) & readMode)>0) digitalInput.p11 = ((byteBuffered & bit(11))>0)?HIGH:LOW;
|
||||
if ((bit(12) & readMode)>0) digitalInput.p12 = ((byteBuffered & bit(12))>0)?HIGH:LOW;
|
||||
if ((bit(13) & readMode)>0) digitalInput.p13 = ((byteBuffered & bit(13))>0)?HIGH:LOW;
|
||||
if ((bit(14) & readMode)>0) digitalInput.p14 = ((byteBuffered & bit(14))>0)?HIGH:LOW;
|
||||
if ((bit(15) & readMode)>0) digitalInput.p15 = ((byteBuffered & bit(15))>0)?HIGH:LOW;
|
||||
#endif
|
||||
if ((readMode & byteBuffered)>0){
|
||||
byteBuffered = ~readMode & byteBuffered;
|
||||
DEBUG_PRINT("Buffer hight value readed set readed ");
|
||||
DEBUG_PRINTLN(byteBuffered, BIN);
|
||||
}
|
||||
DEBUG_PRINT("Return value ");
|
||||
return digitalInput;
|
||||
};
|
||||
#else
|
||||
/**
|
||||
* Read value of all INPUT pin in byte format for low memory usage
|
||||
* Debounce read more fast than 10millis, non managed for interrupt mode
|
||||
* @return
|
||||
*/
|
||||
uint16_t PCF8575::digitalReadAll(void){
|
||||
DEBUG_PRINTLN("Read from buffer");
|
||||
_wire->requestFrom(_address,(uint8_t)2);// Begin transmission to PCF8575 with the buttons
|
||||
lastReadMillis = millis();
|
||||
if(_wire->available()) // If uint16_ts are available to be recieved
|
||||
{
|
||||
DEBUG_PRINTLN("Data ready");
|
||||
uint16_t iInput = _wire->read();// Read a uint16_t
|
||||
iInput |= _wire->read() << 8;// Read a uint16_t
|
||||
|
||||
if ((iInput & readMode)>0){
|
||||
DEBUG_PRINT("Input ");
|
||||
DEBUG_PRINTLN((uint16_t)iInput, BIN);
|
||||
|
||||
byteBuffered = byteBuffered | (uint16_t)iInput;
|
||||
DEBUG_PRINT("byteBuffered ");
|
||||
DEBUG_PRINTLN(byteBuffered, BIN);
|
||||
}
|
||||
}
|
||||
|
||||
DEBUG_PRINT("Buffer value ");
|
||||
DEBUG_PRINTLN(byteBuffered, BIN);
|
||||
|
||||
uint16_t byteRead = byteBuffered;
|
||||
|
||||
if ((readMode & byteBuffered)>0){
|
||||
byteBuffered = ~readMode & byteBuffered;
|
||||
DEBUG_PRINT("Buffer hight value readed set readed ");
|
||||
DEBUG_PRINTLN(byteBuffered, BIN);
|
||||
}
|
||||
DEBUG_PRINT("Return value ");
|
||||
return byteRead;
|
||||
};
|
||||
#endif
|
||||
|
||||
/**
|
||||
* Read value of specified pin
|
||||
* Debounce read more fast than 10millis, non managed for interrupt mode
|
||||
* @param pin
|
||||
* @return
|
||||
*/
|
||||
uint8_t PCF8575::digitalRead(uint8_t pin){
|
||||
uint8_t value = LOW;
|
||||
if ((bit(pin) & writeMode)>0){
|
||||
DEBUG_PRINTLN("Pin in write mode, return value");
|
||||
DEBUG_PRINT("Write data ");
|
||||
DEBUG_PRINT(writeByteBuffered, BIN);
|
||||
DEBUG_PRINT(" for pin ");
|
||||
DEBUG_PRINT(pin);
|
||||
DEBUG_PRINT(" bin value ");
|
||||
DEBUG_PRINT(bit(pin), BIN);
|
||||
DEBUG_PRINT(" value ");
|
||||
DEBUG_PRINTLN(value);
|
||||
|
||||
if ((bit(pin) & writeByteBuffered)>0){
|
||||
value = HIGH;
|
||||
}else{
|
||||
value = LOW;
|
||||
}
|
||||
return value;
|
||||
}
|
||||
|
||||
DEBUG_PRINT("Read pin ");
|
||||
DEBUG_PRINTLN(pin);
|
||||
// Check if pin already HIGH than read and prevent reread of i2c
|
||||
if ((bit(pin) & byteBuffered)>0){
|
||||
DEBUG_PRINTLN("Pin already up");
|
||||
value = HIGH;
|
||||
}else if ((/*(bit(pin) & byteBuffered)<=0 && */millis() > PCF8575::lastReadMillis+READ_ELAPSED_TIME) /*|| _usingInterrupt*/){
|
||||
DEBUG_PRINTLN("Read from buffer");
|
||||
_wire->requestFrom(_address,(uint8_t)2);// Begin transmission to PCF8575 with the buttons
|
||||
lastReadMillis = millis();
|
||||
if(_wire->available()) // If bytes are available to be recieved
|
||||
{
|
||||
DEBUG_PRINTLN("Data ready");
|
||||
uint16_t iInput = _wire->read();// Read a uint16_t
|
||||
iInput |= _wire->read() << 8;// Read a uint16_t
|
||||
|
||||
// Serial.println(iInput, BIN);
|
||||
|
||||
if ((iInput & readMode)>0){
|
||||
DEBUG_PRINT("Input ");
|
||||
DEBUG_PRINTLN((uint16_t)iInput, BIN);
|
||||
|
||||
byteBuffered = byteBuffered | (uint16_t)iInput;
|
||||
DEBUG_PRINT("byteBuffered ");
|
||||
DEBUG_PRINTLN(byteBuffered, BIN);
|
||||
|
||||
if ((bit(pin) & byteBuffered)>0){
|
||||
value = HIGH;
|
||||
}
|
||||
}
|
||||
}
|
||||
}
|
||||
DEBUG_PRINT("Buffer value ");
|
||||
DEBUG_PRINTLN(byteBuffered, BIN);
|
||||
// If HIGH set to low to read buffer only one time
|
||||
if (value==HIGH){
|
||||
byteBuffered = ~bit(pin) & byteBuffered;
|
||||
DEBUG_PRINT("Buffer hight value readed set readed ");
|
||||
DEBUG_PRINTLN(byteBuffered, BIN);
|
||||
}
|
||||
DEBUG_PRINT("Return value ");
|
||||
DEBUG_PRINTLN(value);
|
||||
return value;
|
||||
};
|
||||
|
||||
/**
|
||||
* Write on pin
|
||||
* @param pin
|
||||
* @param value
|
||||
*/
|
||||
void PCF8575::digitalWrite(uint8_t pin, uint8_t value){
|
||||
DEBUG_PRINTLN("Begin trasmission");
|
||||
_wire->beginTransmission(_address); //Begin the transmission to PCF8575
|
||||
if (value==HIGH){
|
||||
writeByteBuffered = writeByteBuffered | bit(pin);
|
||||
}else{
|
||||
writeByteBuffered = writeByteBuffered & ~bit(pin);
|
||||
}
|
||||
// DEBUG_PRINT("Write data ");
|
||||
// DEBUG_PRINT(writeByteBuffered, BIN);
|
||||
// DEBUG_PRINT(" for pin ");
|
||||
// DEBUG_PRINT(pin);
|
||||
// DEBUG_PRINT(" bin value ");
|
||||
// DEBUG_PRINT(bit(pin), BIN);
|
||||
// DEBUG_PRINT(" value ");
|
||||
// DEBUG_PRINTLN(value);
|
||||
|
||||
// Serial.print(" --> ");
|
||||
// Serial.println(writeByteBuffered);
|
||||
// Serial.println((uint8_t) writeByteBuffered);
|
||||
// Serial.println((uint8_t) (writeByteBuffered >> 8));
|
||||
|
||||
writeByteBuffered = writeByteBuffered & writeMode;
|
||||
_wire->write((uint8_t) writeByteBuffered);
|
||||
_wire->write((uint8_t) (writeByteBuffered >> 8));
|
||||
DEBUG_PRINTLN("Start end trasmission if stop here check pullup resistor.");
|
||||
|
||||
_wire->endTransmission();
|
||||
};
|
||||
|
||||
|
230
lib/PCF8575 library/PCF8575.h
Normal file
@ -0,0 +1,230 @@
|
||||
/*
|
||||
* PCF8575 GPIO Port Expand
|
||||
* https://www.mischianti.org/2019/07/22/pcf8575-i2c-16-bit-digital-i-o-expander/
|
||||
*
|
||||
* The MIT License (MIT)
|
||||
*
|
||||
* Copyright (c) 2019 Renzo Mischianti www.mischianti.org All right reserved.
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
* of this software and associated documentation files (the "Software"), to deal
|
||||
* in the Software without restriction, including without limitation the rights
|
||||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
* copies of the Software, and to permit persons to whom the Software is
|
||||
* furnished to do so, subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in
|
||||
* all copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
|
||||
* THE SOFTWARE.
|
||||
*/
|
||||
|
||||
#ifndef PCF8575_h
|
||||
#define PCF8575_h
|
||||
|
||||
#include "Wire.h"
|
||||
|
||||
#if ARDUINO >= 100
|
||||
#include "Arduino.h"
|
||||
#else
|
||||
#include "WProgram.h"
|
||||
#endif
|
||||
|
||||
#define DEFAULT_SDA SDA;
|
||||
#define DEFAULT_SCL SCL;
|
||||
|
||||
// Uncomment to enable printing out nice debug messages.
|
||||
// #define PCF8575_DEBUG
|
||||
|
||||
// Uncomment for low memory usage this prevent use of complex DigitalInput structure and free 7byte of memory
|
||||
// #define PCF8575_LOW_MEMORY
|
||||
|
||||
// Define where debug output will be printed.
|
||||
#define DEBUG_PRINTER Serial
|
||||
|
||||
// Define to manage original pinout of pcf8575
|
||||
// like datasheet but not sequential
|
||||
//#define NOT_SEQUENTIAL_PINOUT
|
||||
|
||||
// Setup debug printing macros.
|
||||
#ifdef PCF8575_DEBUG
|
||||
#define DEBUG_PRINT(...) { DEBUG_PRINTER.print(__VA_ARGS__); }
|
||||
#define DEBUG_PRINTLN(...) { DEBUG_PRINTER.println(__VA_ARGS__); }
|
||||
#else
|
||||
#define DEBUG_PRINT(...) {}
|
||||
#define DEBUG_PRINTLN(...) {}
|
||||
#endif
|
||||
|
||||
#define READ_ELAPSED_TIME 10
|
||||
|
||||
//#define P0 B00000001
|
||||
//#define P1 B00000010
|
||||
//#define P2 B00000100
|
||||
//#define P3 B00001000
|
||||
//#define P4 B00010000
|
||||
//#define P5 B00100000
|
||||
//#define P6 B01000000
|
||||
//#define P7 B10000000
|
||||
//
|
||||
#ifdef NOT_SEQUENTIAL_PINOUT
|
||||
#define P00 0
|
||||
#define P01 1
|
||||
#define P02 2
|
||||
#define P03 3
|
||||
#define P04 4
|
||||
#define P05 5
|
||||
#define P06 6
|
||||
#define P07 7
|
||||
#define P10 8
|
||||
#define P11 9
|
||||
#define P12 10
|
||||
#define P13 11
|
||||
#define P14 12
|
||||
#define P15 13
|
||||
#define P16 14
|
||||
#define P17 15
|
||||
#else
|
||||
#define P0 0
|
||||
#define P1 1
|
||||
#define P2 2
|
||||
#define P3 3
|
||||
#define P4 4
|
||||
#define P5 5
|
||||
#define P6 6
|
||||
#define P7 7
|
||||
#define P8 8
|
||||
#define P9 9
|
||||
#define P10 10
|
||||
#define P11 11
|
||||
#define P12 12
|
||||
#define P13 13
|
||||
#define P14 14
|
||||
#define P15 15
|
||||
#endif
|
||||
|
||||
#include <math.h>
|
||||
|
||||
|
||||
class PCF8575 {
|
||||
public:
|
||||
|
||||
PCF8575(uint8_t address);
|
||||
PCF8575(uint8_t address, uint8_t interruptPin, void (*interruptFunction)() );
|
||||
|
||||
#if !defined(__AVR) && !defined(ARDUINO_ARCH_SAMD) && !defined(TEENSYDUINO)
|
||||
PCF8575(uint8_t address, int sda, int scl);
|
||||
PCF8575(uint8_t address, int sda, int scl, uint8_t interruptPin, void (*interruptFunction)());
|
||||
#endif
|
||||
|
||||
#if defined(ESP32) || defined(ARDUINO_ARCH_SAMD) || defined(ARDUINO_ARCH_RP2040) || defined(ARDUINO_ARCH_STM32)
|
||||
///// changes for second i2c bus
|
||||
PCF8575(TwoWire *pWire, uint8_t address);
|
||||
PCF8575(TwoWire *pWire, uint8_t address, uint8_t interruptPin, void (*interruptFunction)() );
|
||||
#endif
|
||||
#if defined(ESP32)
|
||||
PCF8575(TwoWire *pWire, uint8_t address, int sda, int scl);
|
||||
PCF8575(TwoWire *pWire, uint8_t address, int sda, int scl, uint8_t interruptPin, void (*interruptFunction)());
|
||||
#endif
|
||||
|
||||
void begin();
|
||||
void pinMode(uint8_t pin, uint8_t mode);
|
||||
|
||||
void readBuffer(bool force = true);
|
||||
uint8_t digitalRead(uint8_t pin);
|
||||
#ifndef PCF8575_LOW_MEMORY
|
||||
struct DigitalInput {
|
||||
#ifdef NOT_SEQUENTIAL_PINOUT
|
||||
uint8_t p00;
|
||||
uint8_t p01;
|
||||
uint8_t p02;
|
||||
uint8_t p03;
|
||||
uint8_t p04;
|
||||
uint8_t p05;
|
||||
uint8_t p06;
|
||||
uint8_t p07;
|
||||
uint8_t p10;
|
||||
uint8_t p11;
|
||||
uint8_t p12;
|
||||
uint8_t p13;
|
||||
uint8_t p14;
|
||||
uint8_t p15;
|
||||
uint8_t p16;
|
||||
uint8_t p17;
|
||||
#else
|
||||
uint8_t p0;
|
||||
uint8_t p1;
|
||||
uint8_t p2;
|
||||
uint8_t p3;
|
||||
uint8_t p4;
|
||||
uint8_t p5;
|
||||
uint8_t p6;
|
||||
uint8_t p7;
|
||||
uint8_t p8;
|
||||
uint8_t p9;
|
||||
uint8_t p10;
|
||||
uint8_t p11;
|
||||
uint8_t p12;
|
||||
uint8_t p13;
|
||||
uint8_t p14;
|
||||
uint8_t p15;
|
||||
#endif
|
||||
} digitalInput;
|
||||
|
||||
|
||||
DigitalInput digitalReadAll(void);
|
||||
#else
|
||||
uint16_t digitalReadAll(void);
|
||||
#endif
|
||||
void digitalWrite(uint8_t pin, uint8_t value);
|
||||
|
||||
private:
|
||||
uint8_t _address;
|
||||
|
||||
#if !defined(DEFAULT_SDA)
|
||||
# if defined(ARDUINO_ARCH_STM32)
|
||||
# define DEFAULT_SDA PB7
|
||||
# elif defined(ESP8266)
|
||||
# define DEFAULT_SDA 4
|
||||
# elif defined(SDA)
|
||||
# define DEFAULT_SDA SDA
|
||||
# else
|
||||
# error "Error define DEFAULT_SDA, SDA not declared, if you have this error contact the mantainer"
|
||||
# endif
|
||||
#endif
|
||||
#if !defined(DEFAULT_SCL)
|
||||
# if defined(ARDUINO_ARCH_STM32)
|
||||
# define DEFAULT_SCL PB6
|
||||
# elif defined(ESP8266)
|
||||
# define DEFAULT_SCL 5
|
||||
# elif defined(SDA)
|
||||
# define DEFAULT_SCL SCL
|
||||
# else
|
||||
# error "Error define DEFAULT_SCL, SCL not declared, if you have this error contact the mantainer"
|
||||
# endif
|
||||
#endif
|
||||
|
||||
int _sda = DEFAULT_SDA;
|
||||
int _scl = DEFAULT_SCL;
|
||||
|
||||
TwoWire *_wire;
|
||||
|
||||
bool _usingInterrupt = false;
|
||||
uint8_t _interruptPin = 2;
|
||||
void (*_interruptFunction)(){};
|
||||
|
||||
uint16_t writeMode = 0;
|
||||
uint16_t readMode = 0;
|
||||
uint16_t byteBuffered = 0;
|
||||
unsigned long lastReadMillis = 0;
|
||||
|
||||
uint16_t writeByteBuffered = 0;
|
||||
|
||||
};
|
||||
|
||||
#endif
|
35
lib/PCF8575 library/PCF8575_library.h
Normal file
@ -0,0 +1,35 @@
|
||||
/*
|
||||
* PCF8575 GPIO Port Expand
|
||||
* https://www.mischianti.org/2019/07/22/pcf8575-i2c-16-bit-digital-i-o-expander/
|
||||
*
|
||||
* The MIT License (MIT)
|
||||
*
|
||||
* Copyright (c) 2019 Renzo Mischianti www.mischianti.org All right reserved.
|
||||
*
|
||||
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
* of this software and associated documentation files (the "Software"), to deal
|
||||
* in the Software without restriction, including without limitation the rights
|
||||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
* copies of the Software, and to permit persons to whom the Software is
|
||||
* furnished to do so, subject to the following conditions:
|
||||
*
|
||||
* The above copyright notice and this permission notice shall be included in
|
||||
* all copies or substantial portions of the Software.
|
||||
*
|
||||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
|
||||
* THE SOFTWARE.
|
||||
*/
|
||||
|
||||
#ifndef PCF8575_LIBRARY_H
|
||||
#define PCF8575_LIBRARY_H
|
||||
|
||||
#include "PCF8575.h"
|
||||
|
||||
#endif
|
||||
|
||||
#pragma once
|
132
lib/PCF8575 library/README.md
Normal file
@ -0,0 +1,132 @@
|
||||
<div>
|
||||
<a href="https://www.mischianti.org/forums/forum/mischiantis-libraries/pcf8575-16bits-i2c-digital-i-o-expander/"><img
|
||||
src="https://github.com/xreef/LoRa_E32_Series_Library/raw/master/resources/buttonSupportForumEnglish.png" alt="Support forum pcf8575 English"
|
||||
align="right"></a>
|
||||
</div>
|
||||
<div>
|
||||
<a href="https://www.mischianti.org/it/forums/forum/le-librerie-di-mischianti/pcf8575-expander-digitale-i-o-i2c-a-16bits/"><img
|
||||
src="https://github.com/xreef/LoRa_E32_Series_Library/raw/master/resources/buttonSupportForumItaliano.png" alt="Forum supporto pcf8575 italiano"
|
||||
align="right"></a>
|
||||
</div>
|
||||
|
||||
###Additional information and document update here on my site: [pcf8575 Article](https://www.mischianti.org/2019/07/22/pcf8575-i2c-16-bit-digital-i-o-expander/).
|
||||
|
||||
###If you need less pins [here](https://www.mischianti.org/2019/01/02/pcf8574-i2c-digital-i-o-expander-fast-easy-usage/) you can find pcf8574 discrete 8bit version of the IC.
|
||||
|
||||
Library to use i2c analog IC with arduino and esp8266. Can read and write digital value with only 2 wire (perfect for ESP-01).
|
||||
|
||||
- 16/02/2023: v1.1.0
|
||||
- Fix STM32 support and add support for Raspberry Pi Pico and other rp2040 boards
|
||||
- Add support for custom SERCOM interface of Arduino SAMD devices. Force SDA SCL to use GPIO numeration for STM32 bug (https://www.mischianti.org/forums/topic/compatible-with-stm32duino/).
|
||||
- Force SDA SCL to use GPIO numeration (https://www.mischianti.org/forums/topic/cannot-set-sda-clk-on-esp8266/).
|
||||
- Fix the SDA SCL type #58 and add basic support for SAMD device.
|
||||
- 06/04/2022: v1.0.3 Fix package size
|
||||
|
||||
Tutorial:
|
||||
|
||||
To download. click the DOWNLOADS button in the top right corner, rename the uncompressed folder PCF8575. Check that the PCF8575 folder contains `PCF8575\\.cpp` and `PCF8575.h`. Place the DHT library folder your `<arduinosketchfolder>/libraries/` folder. You may need to create the libraries subfolder if its your first library. Restart the IDE.
|
||||
|
||||
# Reef complete PCF8575 PCF8575AP digital input and output expander with i2c bus.
|
||||
I try to simplify the use of this IC, with a minimal set of operation.
|
||||
|
||||
PCF8575 address map 0x20 default
|
||||
|
||||
Constructor:
|
||||
you must pas the address of i2c (to check the adress use this guide [I2cScanner](https://playground.arduino.cc/Main/I2cScanner))
|
||||
```cpp
|
||||
PCF8575(uint8_t address);
|
||||
```
|
||||
for esp8266 if you want specify SDA e SCL pin use this:
|
||||
|
||||
```cpp
|
||||
PCF8575(uint8_t address, uint8_t sda, uint8_t scl);
|
||||
```
|
||||
You must set input/output mode:
|
||||
```cpp
|
||||
pcf8575.pinMode(P0, OUTPUT);
|
||||
pcf8575.pinMode(P1, INPUT);
|
||||
pcf8575.pinMode(P2, INPUT);
|
||||
```
|
||||
|
||||
then IC as you can see in the image have 8 digital input/output:
|
||||
|
||||

|
||||
|
||||
So to read all analog input in one trasmission you can do (even if I use a 10millis debounce time to prevent too much read from i2c):
|
||||
```cpp
|
||||
PCF8575::DigitalInput di = PCF8575.digitalReadAll();
|
||||
Serial.print(di.p0);
|
||||
Serial.print(" - ");
|
||||
Serial.print(di.p1);
|
||||
Serial.print(" - ");
|
||||
Serial.print(di.p2);
|
||||
Serial.print(" - ");
|
||||
Serial.println(di.p3);
|
||||
```
|
||||
|
||||
To follow a request (you can see It on [issue #5](https://github.com/xreef/PCF8575_library/issues/5)) I create a define variable to work with low memori device, if you decomment this line on .h file of the library:
|
||||
|
||||
```cpp
|
||||
// #define PCF8575_LOW_MEMORY
|
||||
```
|
||||
|
||||
Enable low memory props and gain about 7byte of memory, and you must use the method to read all like so:
|
||||
|
||||
```cpp
|
||||
byte di = pcf8575.digitalReadAll();
|
||||
Serial.print("READ VALUE FROM PCF: ");
|
||||
Serial.println(di, BIN);
|
||||
```
|
||||
|
||||
where di is a byte like 11100011110001, so you must do a bitwise operation to get the data, operation that I already do in the "normal" mode, here an example:
|
||||
|
||||
```cpp
|
||||
p0 = ((di & bit(0))>0)?HIGH:LOW;
|
||||
p1 = ((di & bit(1))>0)?HIGH:LOW;
|
||||
p2 = ((di & bit(2))>0)?HIGH:LOW;
|
||||
p3 = ((di & bit(3))>0)?HIGH:LOW;
|
||||
p4 = ((di & bit(4))>0)?HIGH:LOW;
|
||||
p5 = ((di & bit(5))>0)?HIGH:LOW;
|
||||
p6 = ((di & bit(6))>0)?HIGH:LOW;
|
||||
p7 = ((di & bit(7))>0)?HIGH:LOW;
|
||||
```
|
||||
|
||||
|
||||
if you want read a single input:
|
||||
|
||||
```cpp
|
||||
int p1Digital = PCF8575.digitalRead(P1); // read P1
|
||||
```
|
||||
|
||||
If you want write a digital value you must do:
|
||||
```cpp
|
||||
PCF8575.digitalWrite(P1, HIGH);
|
||||
```
|
||||
or:
|
||||
```cpp
|
||||
PCF8575.digitalWrite(P1, LOW);
|
||||
```
|
||||
|
||||
You can also use interrupt pin:
|
||||
You must initialize the pin and the function to call when interrupt raised from PCF8575
|
||||
```cpp
|
||||
// Function interrupt
|
||||
void keyPressedOnPCF8575();
|
||||
|
||||
// Set i2c address
|
||||
PCF8575 pcf8575(0x39, ARDUINO_UNO_INTERRUPT_PIN, keyPressedOnPCF8575);
|
||||
```
|
||||
Remember you can't use Serial or Wire on interrupt function.
|
||||
|
||||
The better way is to set only a variable to read on loop:
|
||||
```cpp
|
||||
void keyPressedOnPCF8575(){
|
||||
// Interrupt called (No Serial no read no wire in this function, and DEBUG disabled on PCF library)
|
||||
keyPressed = true;
|
||||
}
|
||||
```
|
||||
|
||||
For the examples I use this wire schema on breadboard:
|
||||

|
||||
|
||||
[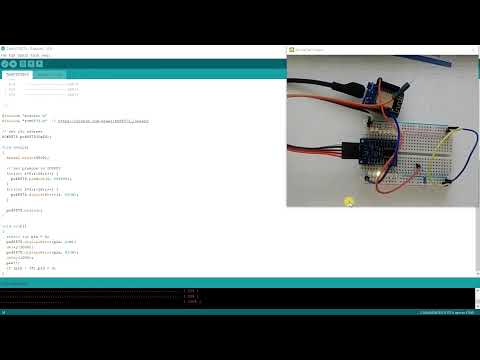](https://youtu.be/jWeHzBLeN6s "Test pcf8575")
|
30
lib/PCF8575 library/examples/blinkOnPin0/blinkOnPin0.ino
Normal file
@ -0,0 +1,30 @@
|
||||
/*
|
||||
Blink led on PIN0
|
||||
by Mischianti Renzo <http://www.mischianti.org>
|
||||
|
||||
https://www.mischianti.org/2019/01/02/pcf8575-i2c-digital-i-o-expander-fast-easy-usage/
|
||||
*/
|
||||
|
||||
#include "Arduino.h"
|
||||
#include "PCF8575.h"
|
||||
|
||||
// Set i2c address
|
||||
PCF8575 pcf8575(0x20);
|
||||
|
||||
void setup()
|
||||
{
|
||||
Serial.begin(115200);
|
||||
|
||||
// Set pinMode to OUTPUT
|
||||
pcf8575.pinMode(P0, OUTPUT);
|
||||
|
||||
pcf8575.begin();
|
||||
}
|
||||
|
||||
void loop()
|
||||
{
|
||||
pcf8575.digitalWrite(P0, HIGH);
|
||||
delay(1000);
|
||||
pcf8575.digitalWrite(P0, LOW);
|
||||
delay(1000);
|
||||
}
|
@ -0,0 +1,76 @@
|
||||
/*
|
||||
* PCF8575 GPIO Port Expand
|
||||
* http://nopnop2002.webcrow.jp/WeMos/WeMos-25.html
|
||||
*
|
||||
* PCF8575 ----- WeMos
|
||||
* A0 ----- GRD
|
||||
* A1 ----- GRD
|
||||
* A2 ----- GRD
|
||||
* VSS ----- GRD
|
||||
* VDD ----- 5V/3.3V
|
||||
* SDA ----- GPIO_4
|
||||
* SCL ----- GPIO_5
|
||||
* INT ----- GPIO_13
|
||||
*
|
||||
* P0 ----------------- BUTTON0
|
||||
* P1 ----------------- BUTTON1
|
||||
* P2 ----------------- BUTTON2
|
||||
* P3 ----------------- BUTTON3
|
||||
* P4 ----------------- BUTTON4
|
||||
* P5 ----------------- BUTTON5
|
||||
* P6 ----------------- BUTTON6
|
||||
* P7 ----------------- BUTTON7
|
||||
*
|
||||
*/
|
||||
|
||||
#include "Arduino.h"
|
||||
#include "PCF8575.h" // https://github.com/xreef/PCF8575_library
|
||||
|
||||
#define ESP8266_INTERRUPTED_PIN 13
|
||||
|
||||
// Set i2c address
|
||||
PCF8575 pcf8575(0x20);
|
||||
|
||||
// Function interrupt
|
||||
bool keyPressed = false;
|
||||
|
||||
void keyPressedOnPCF8575(){
|
||||
// Serial.println("keyPressedOnPCF8575");
|
||||
keyPressed = true;
|
||||
}
|
||||
|
||||
void setup()
|
||||
{
|
||||
Serial.begin(9600);
|
||||
pinMode(ESP8266_INTERRUPTED_PIN, INPUT_PULLUP);
|
||||
attachInterrupt(digitalPinToInterrupt(ESP8266_INTERRUPTED_PIN), keyPressedOnPCF8575, FALLING);
|
||||
|
||||
for(int i=0;i<8;i++) {
|
||||
pcf8575.pinMode(i, INPUT);
|
||||
}
|
||||
pcf8575.begin();
|
||||
}
|
||||
|
||||
void loop()
|
||||
{
|
||||
if (keyPressed){
|
||||
PCF8575::DigitalInput val = pcf8575.digitalReadAll();
|
||||
if (val.p0==HIGH) Serial.println("KEY0 PRESSED");
|
||||
if (val.p1==HIGH) Serial.println("KEY1 PRESSED");
|
||||
if (val.p2==HIGH) Serial.println("KEY2 PRESSED");
|
||||
if (val.p3==HIGH) Serial.println("KEY3 PRESSED");
|
||||
if (val.p4==HIGH) Serial.println("KEY4 PRESSED");
|
||||
if (val.p5==HIGH) Serial.println("KEY5 PRESSED");
|
||||
if (val.p6==HIGH) Serial.println("KEY6 PRESSED");
|
||||
if (val.p7==HIGH) Serial.println("KEY7 PRESSED");
|
||||
if (val.p8==HIGH) Serial.println("KEY8 PRESSED");
|
||||
if (val.p9==HIGH) Serial.println("KEY9 PRESSED");
|
||||
if (val.p10==HIGH) Serial.println("KEY10 PRESSED");
|
||||
if (val.p11==HIGH) Serial.println("KEY11 PRESSED");
|
||||
if (val.p12==HIGH) Serial.println("KEY12 PRESSED");
|
||||
if (val.p13==HIGH) Serial.println("KEY13 PRESSED");
|
||||
if (val.p14==HIGH) Serial.println("KEY14 PRESSED");
|
||||
if (val.p15==HIGH) Serial.println("KEY15 PRESSED");
|
||||
keyPressed= false;
|
||||
}
|
||||
}
|
@ -0,0 +1,28 @@
|
||||
/*
|
||||
KeyPressed on PIN1
|
||||
by Mischianti Renzo <http://www.mischianti.org>
|
||||
|
||||
https://www.mischianti.org/2019/01/02/pcf8575-i2c-digital-i-o-expander-fast-easy-usage/
|
||||
*/
|
||||
|
||||
#include "Arduino.h"
|
||||
#include "PCF8575.h"
|
||||
|
||||
// Set i2c address
|
||||
PCF8575 pcf8575(0x20);
|
||||
unsigned long timeElapsed;
|
||||
void setup()
|
||||
{
|
||||
Serial.begin(115200);
|
||||
|
||||
pcf8575.pinMode(P1, INPUT);
|
||||
|
||||
pcf8575.begin();
|
||||
}
|
||||
|
||||
void loop()
|
||||
{
|
||||
uint8_t val = pcf8575.digitalRead(P1);
|
||||
if (val==HIGH) Serial.println("KEY PRESSED");
|
||||
delay(50);
|
||||
}
|
@ -0,0 +1,35 @@
|
||||
/*
|
||||
KeyPressed async
|
||||
by Mischianti Renzo <http://www.mischianti.org>
|
||||
|
||||
https://www.mischianti.org/2019/01/02/pcf8575-i2c-digital-i-o-expander-fast-easy-usage/
|
||||
*/
|
||||
|
||||
#include "Arduino.h"
|
||||
#include "PCF8575.h"
|
||||
|
||||
// Set i2c address
|
||||
PCF8575 pcf8575(0x20);
|
||||
unsigned long timeElapsed;
|
||||
void setup()
|
||||
{
|
||||
Serial.begin(115200);
|
||||
|
||||
pcf8575.pinMode(P1, INPUT);
|
||||
pcf8575.begin();
|
||||
|
||||
|
||||
timeElapsed = millis();
|
||||
}
|
||||
|
||||
void loop()
|
||||
{
|
||||
// Read and store on buffer all input (pinMode) that are going HIGHT
|
||||
pcf8575.readBuffer();
|
||||
if (millis()>timeElapsed+2000){
|
||||
// read value on buffer than reset value for that pin
|
||||
uint8_t val = pcf8575.digitalRead(P1);
|
||||
if (val==HIGH) Serial.println("KEY PRESSED STORED ON BUFFER, NOW READED AND RESETTED.");
|
||||
timeElapsed = millis();
|
||||
}
|
||||
}
|
@ -0,0 +1,46 @@
|
||||
/*
|
||||
KeyPressed with interrupt
|
||||
by Mischianti Renzo <http://www.mischianti.org>
|
||||
|
||||
https://www.mischianti.org/2019/01/02/pcf8575-i2c-digital-i-o-expander-fast-easy-usage/
|
||||
*/
|
||||
|
||||
#include "Arduino.h"
|
||||
#include "PCF8575.h"
|
||||
|
||||
// For arduino uno only pin 1 and 2 are interrupted
|
||||
#define ARDUINO_UNO_INTERRUPTED_PIN 2
|
||||
|
||||
// Function interrupt
|
||||
void keyPressedOnPCF8575();
|
||||
|
||||
// Set i2c address
|
||||
PCF8575 pcf8575(0x39, ARDUINO_UNO_INTERRUPTED_PIN, keyPressedOnPCF8575);
|
||||
unsigned long timeElapsed;
|
||||
void setup()
|
||||
{
|
||||
Serial.begin(115200);
|
||||
|
||||
pcf8575.pinMode(P1, INPUT);
|
||||
pcf8575.begin();
|
||||
|
||||
|
||||
timeElapsed = millis();
|
||||
}
|
||||
|
||||
bool keyPressed = false;
|
||||
void loop()
|
||||
{
|
||||
if (keyPressed){
|
||||
uint8_t val = pcf8575.digitalRead(P1);
|
||||
Serial.print("READ VALUE FROM PCF ");
|
||||
Serial.println(val);
|
||||
keyPressed= false;
|
||||
}
|
||||
}
|
||||
|
||||
void keyPressedOnPCF8575(){
|
||||
// Interrupt called (No Serial no read no wire in this function, and DEBUG disabled on PCF library)
|
||||
keyPressed = true;
|
||||
|
||||
}
|